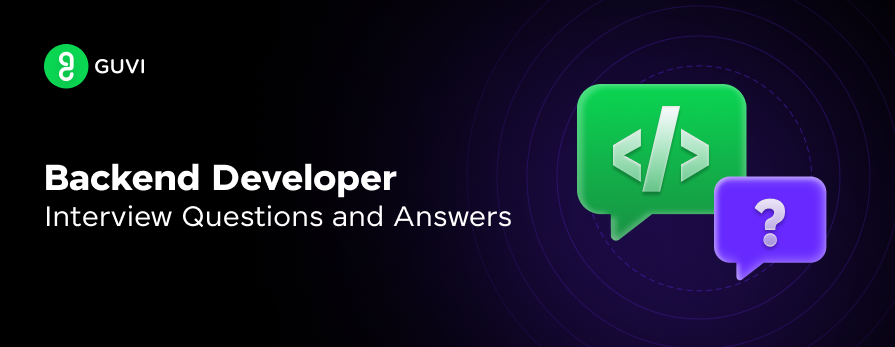
Backend Developer Interview Questions and Answers
Oct 04, 2024 7 Min Read 3533 Views
(Last Updated)
Backend development plays a critical role in building and maintaining the server-side logic that powers web applications. As companies increasingly rely on complex systems to manage data and provide seamless user experiences, the demand for skilled backend developers continues to grow.
Preparing for backend developer interviews requires a deep understanding of various concepts like databases, APIs, system design, and performance optimization, as well as proficiency in problem-solving and collaboration.
In this article, I will be putting forward answers to the most frequently asked backend developer interview questions and answers, covering technical areas such as database design, API integration, scalability, and system architecture.
As an added step of preparation, we will also discuss situational and behavioral questions, ensuring you’re ready for both the technical and soft skill evaluations.
Table of contents
- Introduction to Backend Development
- Top Backend Developer Interview Questions and Answers: Section-Wise
- SQL and Database Management
- API Design and Web Services
- Security
- System Design and Architecture
- Performance Optimization
- Scalability and Fault Tolerance
- Situational and Problem-Solving Questions
- HR and Behavioral Interview Questions
- Takeaways…
- FAQs
- How do I prepare for a backend developer interview?
- What is the skill of a backend developer?
- What is the main job of a backend developer?
- What are the 3 parts of backend development?
- Is SQL backend or frontend?
Introduction to Backend Development
Backend development is the backbone of any application, responsible for managing databases, servers, and the logic that drives the front end. Unlike frontend development, where the focus is on the user interface and experience, backend developers handle the behind-the-scenes functionality that users rely on without direct interaction.
A backend developer’s daily responsibilities typically include:
- Database Management: Handling CRUD operations (Create, Read, Update, Delete) and ensuring efficient database architecture.
- API Development: Designing, developing, and maintaining RESTful APIs that connect frontend interfaces with the database.
- Server Maintenance: Monitoring server performance, scaling server resources, and ensuring uptime during high traffic.
- Security Measures: Protecting applications from SQL injection, Cross-Site Scripting (XSS), and other vulnerabilities.
A solid backend developer must be proficient in the following key areas:
Skill | Description |
Programming Languages | Mastery of languages like Python, Java, Ruby, or Node.js is essential. Backend frameworks like Django, Spring, or Express.js are also critical. |
Database Knowledge | Familiarity with relational (e.g., MySQL, PostgreSQL) and NoSQL databases (e.g., MongoDB, Cassandra). |
API & HTTP Protocols | Understanding how to design and consume REST APIs, manage HTTP methods, and ensure stateless interactions. |
Version Control | Working knowledge of Git for versioning code and collaborating with teams. |
Security Best Practices | Understanding of database security and authentication methods (OAuth, JWT), encryption (HTTPS), and mitigation strategies against attacks like SQL injection. |
A typical day for a backend developer involves working with servers, optimizing databases, securing APIs, handling server-side logic, and communicating with front-end components to ensure seamless functionality.
Top Backend Developer Interview Questions and Answers: Section-Wise
In this guide, to ensure you gain a well-rounded understanding of the kind of questions that can be asked in these interviews, I have divided the range of questions into different sections from technical to situational and even HR.
We will be discussing a few questions and answers from all these areas for an elevated learning experience.
1. SQL and Database Management
Question 1: What is the difference between SQL and NoSQL databases?
Answer:
- SQL (Structured Query Language) databases are relational, with structured schemas, and they store data in tables (e.g., MySQL, PostgreSQL).
- NoSQL databases are non-relational and can handle unstructured or semi-structured data (e.g., MongoDB, Cassandra).
Question 2: How do you optimize a slow SQL query?
Answer:
- Add indexes to frequently queried columns.
- Use EXPLAIN to analyze query execution plans.
- Avoid selecting all columns (SELECT *), and instead query only the needed fields.
Question 3: What are transactions in SQL?
Answer:
A transaction is a sequence of operations executed as a single unit. It guarantees ACID properties (Atomicity, Consistency, Isolation, Durability) to ensure data integrity.
Question 4: How do you handle database migrations in a live system?
Answer:
- Use database migration tools (like Liquibase or Flyway) to automate changes.
- Apply changes in small, incremental batches.
- Backup the database before significant changes.
Question 5: What is the difference between JOIN and UNION in SQL?
Answer:
- JOIN combines rows from two or more tables based on a related column.
- UNION combines results from two queries into a single result set, eliminating duplicates.
2. API Design and Web Services
Question 1: What are RESTful APIs, and why are they used?
Answer:
REST (Representational State Transfer) is an architectural style used for designing networked applications. It uses HTTP methods like GET, POST, PUT, and DELETE to perform CRUD operations on resources.
Question 2: What is the difference between REST and SOAP?
Answer:
- REST is lightweight, stateless, and uses JSON for data exchange.
- SOAP (Simple Object Access Protocol) is more protocol-driven and uses XML, typically requiring more overhead.
Question 3: How does GraphQL differ from REST?
Answer:
GraphQL allows clients to query specific data fields, reducing over-fetching or under-fetching data, whereas REST APIs return fixed data structures.
Question 4: How do you handle versioning in REST APIs?
Answer:
You can handle versioning by:
- Including versioning in the URL (/api/v1/resource).
- Using headers (Accept: application/vnd.myapi.v1+json).
Question 5: What are the advantages of using WebSockets over HTTP?
Answer:
WebSockets provide full-duplex communication, allowing data to be sent in both directions simultaneously, making it ideal for real-time applications like chat or stock market updates.
3. Security
Question 1: How do you prevent SQL injection?
Answer:
- Use parameterized queries or prepared statements.
- Validate and sanitize all user inputs.
- Use ORM frameworks that abstract SQL.
Question 2: What is Cross-Site Request Forgery (CSRF), and how do you prevent it?
Answer:
CSRF is an attack where unauthorized commands are sent from a trusted user’s browser. Prevention techniques include using anti-CSRF tokens and implementing SameSite cookie policies.
Question 3: How do you implement secure authentication in APIs?
Answer:
- Use token-based authentication (e.g., JWT).
- Implement OAuth 2.0 for third-party access.
- Ensure communication is secured with SSL/TLS encryption.
Question 4: What is HTTPS, and why is it important?
Answer:
HTTPS is the secure version of HTTP, encrypting data between client and server using SSL/TLS, protecting against eavesdropping and man-in-the-middle attacks.
Question 5: What is a rate limiter, and why is it important?
Answer:
A rate limiter restricts the number of requests a client can make in a specific time period. It protects the server from DoS attacks and excessive API usage.
4. System Design and Architecture
Question 1: What is the CAP theorem, and how does it apply to distributed systems?
Answer:
The CAP theorem states that in a distributed system, you can only guarantee two of the following three:
- Consistency: All nodes see the same data.
- Availability: System always responds to requests.
- Partition Tolerance: The system continues to function even with network partitions.
Question 2: What are microservices, and how do they compare to monolithic architecture?
Answer:
Microservices are small, independent services that work together, whereas monolithic architecture involves one large application.
Question 3: What is the difference between horizontal and vertical scaling in backend systems?
Answer:
- Horizontal scaling involves adding more servers to distribute the load across multiple machines, which is ideal for handling large-scale applications.
- Vertical scaling increases the resources of a single server (e.g., adding more RAM, CPU), but it has physical limitations in terms of how much one machine can scale.
Question 4: What are the benefits of using a message queue in microservices architecture?
Answer:
Message queues (e.g., RabbitMQ, Kafka) decouple services, allowing them to communicate asynchronously. Benefits include:
- Fault tolerance: If a service is down, the message is stored in the queue until it’s processed.
- Scalability: Message queues help balance loads between services.
Question 5: How do you design a highly available and fault-tolerant system?
Answer:
A highly available and fault-tolerant system requires:
- Replication: Data is replicated across multiple servers or locations to prevent data loss.
- Load balancing: Traffic is distributed across servers to prevent overloading any one server.
- Auto-scaling: Automatically adding or removing servers based on demand.
Would you like to learn not just backend but also frontend development and build your career as a full-stack developer?
Then GUVI’s Zen Class Full Stack Development Course which offers a comprehensive program designed to transform beginners into skilled developers will be the best resource for you.
It covers key technologies like HTML, CSS, JavaScript, React, Node.js, and more, focusing on hands-on learning and real-world projects. Key benefits include personalized mentorship, industry-grade projects, and job placement assistance.
5. Performance Optimization
Question 1: How do you improve the performance of a backend application?
Answer:
- Database query optimization: Use indexes, avoid unnecessary joins, and limit data retrieval.
- Caching: Store frequently accessed data in memory (e.g., Redis, Memcached) to reduce database load.
- Lazy loading: Load resources only when they are required to reduce memory consumption.
Question 2: How does a Content Delivery Network (CDN) improve application performance?
Answer:
A CDN stores copies of static content (images, CSS, JS files) on geographically distributed servers. This reduces latency by serving content from a server closest to the user.
Question 3: What is database sharding, and when should you use it?
Answer:
Sharding is a database partitioning technique where a large database is split into smaller, more manageable pieces, called shards. It’s used to improve scalability in distributed systems, especially when dealing with large datasets.
Question 4: How do you handle high traffic and ensure smooth performance under load?
Answer:
- Load balancers: Distribute traffic across multiple servers to avoid overloading.
- Auto-scaling: Automatically add more servers during peak traffic times.
- Asynchronous processing: Offload time-consuming tasks to background jobs.
Question 5: What is the purpose of using a reverse proxy server?
Answer:
A reverse proxy acts as an intermediary for requests from clients seeking resources from backend servers. It improves:
- Security: Hides the identity of backend servers.
- Load distribution: Balances incoming traffic across multiple servers.
- Caching: Stores frequently requested content to improve response times.
6. Scalability and Fault Tolerance
Question 1: What is eventual consistency, and how does it apply to distributed systems?
Answer:
Eventual consistency is a model used in distributed systems where updates to the system will eventually propagate to all nodes, but not immediately. This is common in systems that prioritize availability over immediate consistency (e.g., in CAP theorem).
Question 2: How do you design a system to handle millions of users?
Answer:
To handle millions of users, consider:
- Horizontal scaling: Add more servers to handle increased traffic.
- Database partitioning (sharding): Split the database into smaller chunks to reduce load.
- Caching: Use in-memory caches (e.g., Redis, Memcached) to store frequently accessed data.
- Load balancers: Distribute traffic across multiple servers.
Question 3: What is the role of a microservices architecture in scaling applications?
Answer:
Microservices break down applications into independent, loosely coupled services. Each service can be scaled individually based on its demand, making it easier to manage and scale large applications.
Question 4: How do you handle failover in a distributed system?
Answer:
- Use replication to ensure data redundancy across multiple servers.
- Implement automatic failover mechanisms where a backup server takes over if the primary one fails.
- Health checks: Constantly monitor system health and trigger failover if a service is down.
Question 5: What is the difference between synchronous and asynchronous communication in microservices?
Answer:
- Synchronous communication involves real-time communication where services wait for a response (e.g., REST APIs).
- Asynchronous communication allows services to send messages and process responses later, typically using message queues or event-driven architectures.
7. Situational and Problem-Solving Questions
Situational and problem-solving questions assess how you think on your feet and handle real-world backend challenges. These questions often don’t have a single correct answer but focus on your approach to problem-solving, decision-making, and troubleshooting.
Question 1: How would you handle a sudden increase in traffic to your backend services?
Answer:
- Short-term: Increase capacity by scaling horizontally using auto-scaling techniques and load balancers to distribute traffic.
- Long-term: Investigate bottlenecks, optimize database queries, implement caching mechanisms, and review your system’s architecture for improvements.
Question 2: A production API service is down, and users are affected. How would you troubleshoot the issue?
Answer:
- Check logs for errors or warnings.
- Verify the health of servers and databases.
- Ensure network connectivity between services is functional.
- Roll back recent deployments if the issue is linked to new changes.
- Communicate with affected users about downtime while working on a resolution.
Question 3: How would you approach designing a system that supports millions of users simultaneously?
Answer:
- Use horizontal scaling to add servers as needed.
- Employ caching to reduce database load.
- Partition data using sharding to improve performance.
- Use a content delivery network (CDN) to serve static resources globally.
- Implement load balancing to manage traffic efficiently.
Question 4: How would you handle data consistency in a distributed system?
Answer:
- Implement eventual consistency for less critical data to ensure high availability.
- Use ACID-compliant transactions for critical operations.
- Design the system with CAP theorem in mind, prioritizing based on the application needs.
Question 5: You notice that the backend services are slowing down over time. How would you diagnose and fix the issue?
Answer:
- Analyze performance metrics (e.g., CPU, memory usage, latency).
- Optimize database queries and look for locking or blocking issues.
- Review server logs for memory leaks or frequent errors.
- Implement profiling tools to find slow methods or inefficient code.
- Introduce caching layers where necessary.
8. HR and Behavioral Interview Questions
HR and behavioral questions help interviewers gauge your soft skills, work ethic, and cultural fit within the company. While these aren’t technical, they’re equally important for securing a backend developer position.
Question 1: Can you describe a time when you faced a challenging problem as a backend developer and how you resolved it?
Answer:
- Situation: Explain the challenge (e.g., a system outage or performance degradation).
- Task: What was your responsibility?
- Action: Detail the steps you took (e.g., diagnostics, collaboration with team members, deployment of fixes).
- Result: Highlight the positive outcome (e.g., restored service, improved performance).
Question 2: How do you prioritize tasks when managing multiple projects simultaneously?
Answer:
- Use project management tools like JIRA to track tasks.
- Break down tasks into priority levels (e.g., critical vs. non-critical).
- Communicate with stakeholders to align priorities.
- Focus on tasks that have the highest impact on the project or team.
Question 3: How do you handle working under pressure or tight deadlines?
Answer:
- Stay organized: Break tasks into smaller, manageable pieces.
- Communicate proactively with the team to manage expectations.
- Use time management techniques like the Pomodoro technique to stay focused.
- If needed, ask for support from the team to meet deadlines.
Question 4: Have you ever worked in a team where there was conflict? How did you handle it?
Answer:
- Listen to both sides of the argument.
- Facilitate a calm discussion to understand the root of the conflict.
- Focus on finding a solution that aligns with the team’s goals.
- Ensure that after resolving the conflict, there is no lingering tension.
Question 5: Why do you want to work as a backend developer at this company?
Answer:
- Research the company’s mission and projects to tailor your answer.
- Mention any technologies they use that you are excited to work with.
- Highlight how your skills and experience align with the role’s requirements.
- Express interest in growth opportunities and contributing to impactful projects.
Takeaways…
Preparing for a backend developer interview requires not only understanding the core technical skills but also being adept at problem-solving and adapting to real-world challenges.
By mastering key concepts such as API design, database management, and system architecture, you can showcase your technical prowess and approach interviews with confidence.
I hope this in-depth discussion of all kinds of backend interview questions and answers has helped you in your learning journey, do let us know in the comments section if you have any doubts.
FAQs
1. How do I prepare for a backend developer interview?
Focus on mastering data structures, algorithms, database design, and core backend technologies like APIs, server-side languages, and security protocols.
2. What is the skill of a backend developer?
A backend developer is skilled in:
a) Server-side Programming
b) Database Management
c) API Development
d) Handling Server Infrastructure
3. What is the main job of a backend developer?
The main job is to build and maintain the server-side logic, databases, and APIs that power the front end of an application.
4. What are the 3 parts of backend development?
The three parts are:
a) Server Logic
b) Database Management
c) API Integration.
5. Is SQL backend or frontend?
SQL is a backend technology used for database management.
Did you enjoy this article?