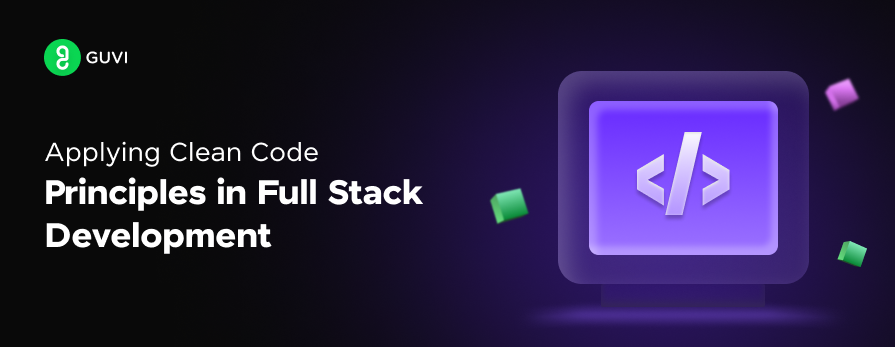
Applying Clean Code Principles in Full Stack Development
Oct 31, 2024 5 Min Read 2654 Views
(Last Updated)
The concept of “clean code” has become increasingly crucial, especially in full stack development. As developers, we’re tasked with creating complex systems that span multiple layers of technology, from user interfaces to databases and everything in between. The principles of clean code serve as a guiding light, helping us understand this complexity while maintaining code that is readable, maintainable, and efficient.
In this blog post, we will explore how clean code principles can be applied across the entire stack of a modern web application. We’ll get into best practices for frontend development, backend systems, database design, and even extend these principles to DevOps practices. By the end of this post, you’ll have a comprehensive understanding of how to implement clean code techniques in every aspect of your full stack projects.
Table of contents
- What is Clean Code?
- Why is Clean Code Important?
- Clean Code Fundamentals: The SOLID Principles
- Single Responsibility Principle (SRP)
- Open-Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
- Clean Code Principles in Full Stack Development
- Meaningful Variable and Function Names
- Keep Functions and Methods Short
- Comments and Documentation
- Consistent Formatting and Indentation
- DRY (Don't Repeat Yourself) Principle
- Use Meaningful Whitespace
- Error Handling
- Refactoring Techniques for Cleaner Code
- Identifying Code Smells
- Refactoring Patterns and Strategies
- Tools for Automated Refactoring
- Balancing Clean Code with Deadlines and Practicality
- When to Prioritize Clean Code vs. Quick Delivery
- Technical Debt Management
- Conclusion
- FAQs
- What is Clean Code, and why is it important in Full Stack Development?
- How do Clean Code principles enhance collaboration in a Full Stack team?
- What are some common Clean Code practices for back-end development?
- How can Clean Code principles improve front-end code, like HTML, CSS, and JavaScript?
- How can Full Stack developers implement Clean Code in complex projects?
What is Clean Code?
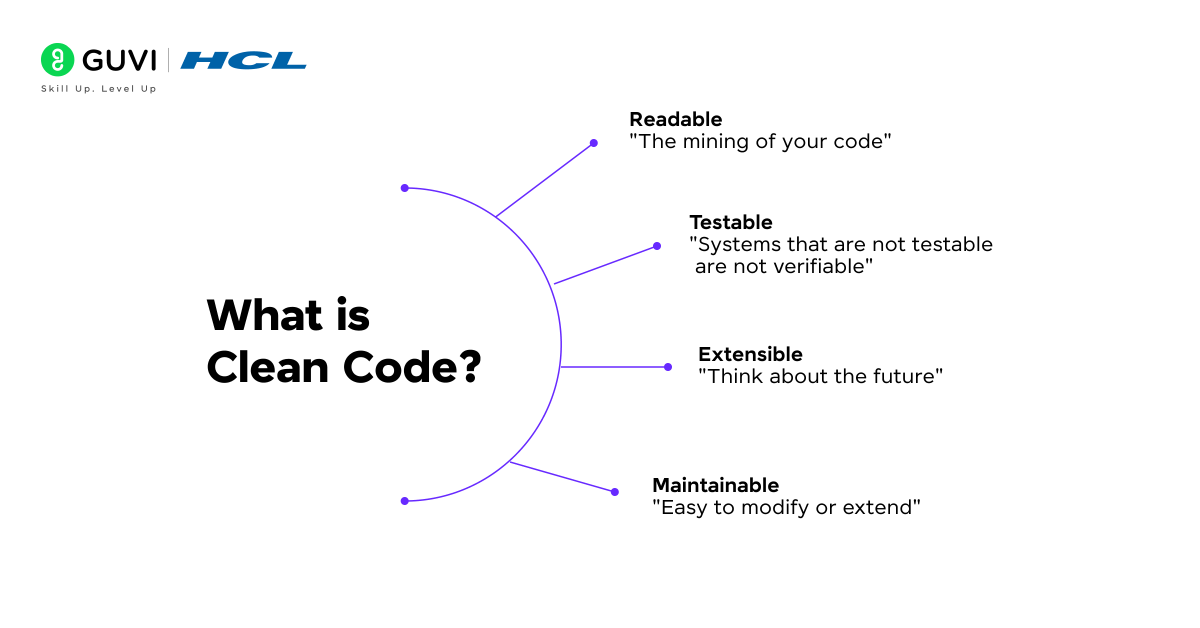
Clean code is characterized by its simplicity, readability, and maintainability. It’s code that is easy to understand, easy to change, and easy to extend. Robert C. Martin, in his seminal book “Clean Code: A Handbook of Agile Software Craftsmanship,” describes clean code as code that is focused, simple, and deliberate. It’s code that has been taken care of, where each function, class, and module tells a clear, cohesive story.
The importance of clean code cannot be overstated. In the short term, it might seem faster to write “quick and dirty” code to meet deadlines. However, this approach inevitably leads to technical debt, which can severely impede a project’s progress in the long run. Clean code, on the other hand, leads to:
- Improved readability and comprehension
- Easier maintenance and updates
- Faster onboarding of new team members
- Reduced bugs and easier debugging
- Enhanced scalability and flexibility
Why is Clean Code Important?
Clean code is not aesthetics; it’s actually a basic principle that goes a long way into impacting the quality, maintainability, and success of software projects. Here’s why clean code matters:
- Clarity: Clean code is well structured, uses meaningful names, and has eliminated all unnecessary complexity. This makes it easier for developers to understand and navigate their code.
- Modifiability: A clean code can be easily modified in terms of introducing a new feature or changing something that does not introduce bugs or unexpected side effects.
- Reduced Debugging Time: Clean code reduces the chance of bugs, which means less time spent while debugging the issue and solving it.
- Improved Communication: Clean code improves the communication of a team for better. The closer that it is to being understood by others, the better one can discuss changes, work together on new features, and resolve conflicts.
- Increased Efficiency: Consistent coding standards and practices reduce the time that needs to get a new developer up to speed on a project, thus improving efficiency and productivity.
- Future-Proofing: Clean code is easier to adapt to changing requirements or the latest technologies. It is easy to maintain and evolve the code base in the long run. Lower
- Technical Debt: Clean code prevents technical debt from surfacing in the first place, hence saving the organization in the long term run from increased development costs and low productivity.
Clean Code Fundamentals: The SOLID Principles
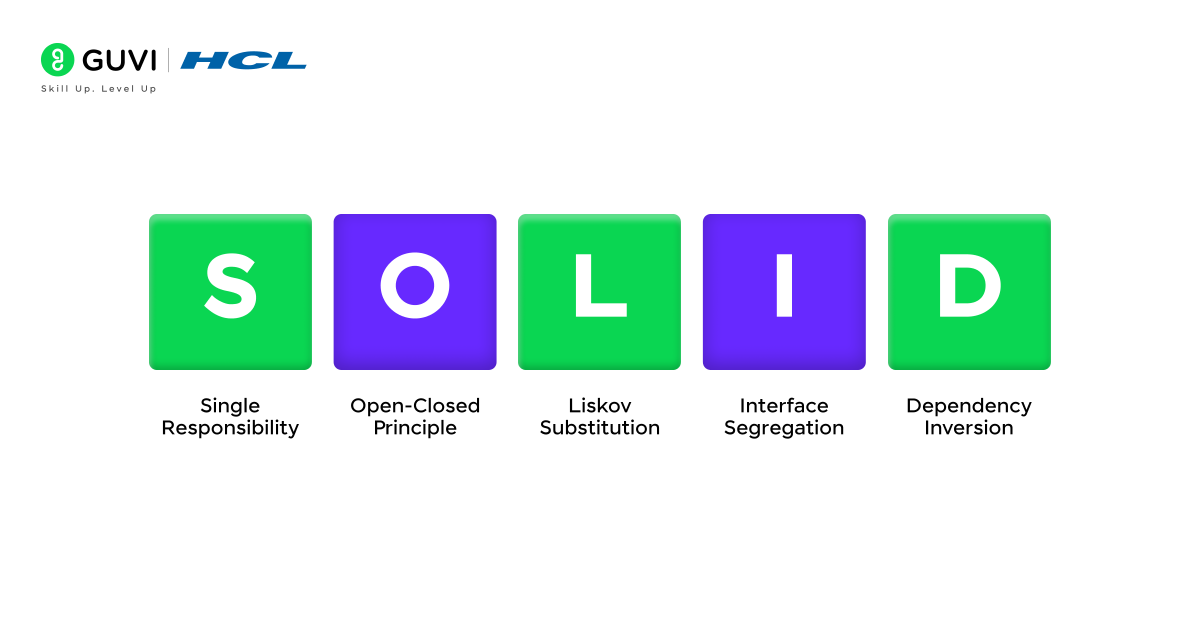
The SOLID principles are a set of design principles that promote code quality, maintainability, and extensibility. They were introduced by Robert C. Martin and have become a cornerstone of object-oriented programming.
Single Responsibility Principle (SRP)
A class should have a single responsibility and a reason to change. This means that a class should focus on a specific task or area of functionality and avoid taking on too many responsibilities. By adhering to the SRP, you can create smaller, more focused classes that are easier to understand, maintain, and test.
Open-Closed Principle (OCP)
Classes should be open for extension but closed for modification. This means that you can add new functionality to a class without changing its existing code. This is achieved through inheritance and polymorphism. By following the OCP, you can make your code more flexible and adaptable to change.
Liskov Substitution Principle (LSP)
Objects of a derived class should be substitutable for objects of the base class without affecting the correctness of the program. This ensures that subclasses adhere to the contract defined by the base class. By following the LSP, you can avoid unexpected behavior and maintain the integrity of your codebase.
Interface Segregation Principle (ISP)
Clients should not be forced to depend on interfaces they do not use. This means that large interfaces should be broken down into smaller, more specific interfaces. By following the ISP, you can reduce coupling between classes and improve the modularity of your code.
Dependency Inversion Principle (DIP)
High-level modules should not depend on low-level modules. Both should depend on abstractions. This means that your code should be designed to be independent of specific implementations. By following the DIP, you can make your code more flexible, testable, and easier to maintain.
Clean Code Principles in Full Stack Development
Clean code is not just about writing code that works; it’s about writing code that is readable, maintainable, and extensible. By following clean code principles, you can significantly improve the quality and efficiency of your software development projects.
Lets explore the key principles of clean code and provide practical examples to illustrate their application.
1. Meaningful Variable and Function Names
- Clarity: Choose names that accurately reflect the purpose of variables, functions, and classes. This makes the code easier to understand and maintain.
- Avoid Abbreviations: Use full words or clear abbreviations to avoid confusion.
- Consistency: Maintain consistent naming conventions throughout your codebase.
Example:
JavaScript
// Bad naming
let x = 5;
function doSomething(y) {
// ...
}
// Good naming
let totalScore = 5;
function calculateTotalPrice(items) {
// ...
}
2. Keep Functions and Methods Short
- Focus: Functions should have a single responsibility and be concise.
- Readability: Shorter functions are easier to understand and maintain.
- Testability: Smaller functions are easier to test individually.
Example:
JavaScript
// Long function
function processUserData(user) {
// Many lines of code...
}
// Refactored into smaller functions
function validateUserInput(userInput) {
// Validation logic...
}
function saveUserToDatabase(user) {
// Database operation...
}
3. Comments and Documentation
- Explain Purpose: Use comments to explain the purpose of complex code sections or algorithms.
- Document Public APIs: Provide clear documentation for public functions and classes.
- Avoid Redundant Comments: Don’t comment on obvious code. The code itself should be self-explanatory.
Example:
JavaScript
// Bad comment
x = x + 1; // Increment x
// Good comment
// Calculate the total score
totalScore += 1;
4. Consistent Formatting and Indentation
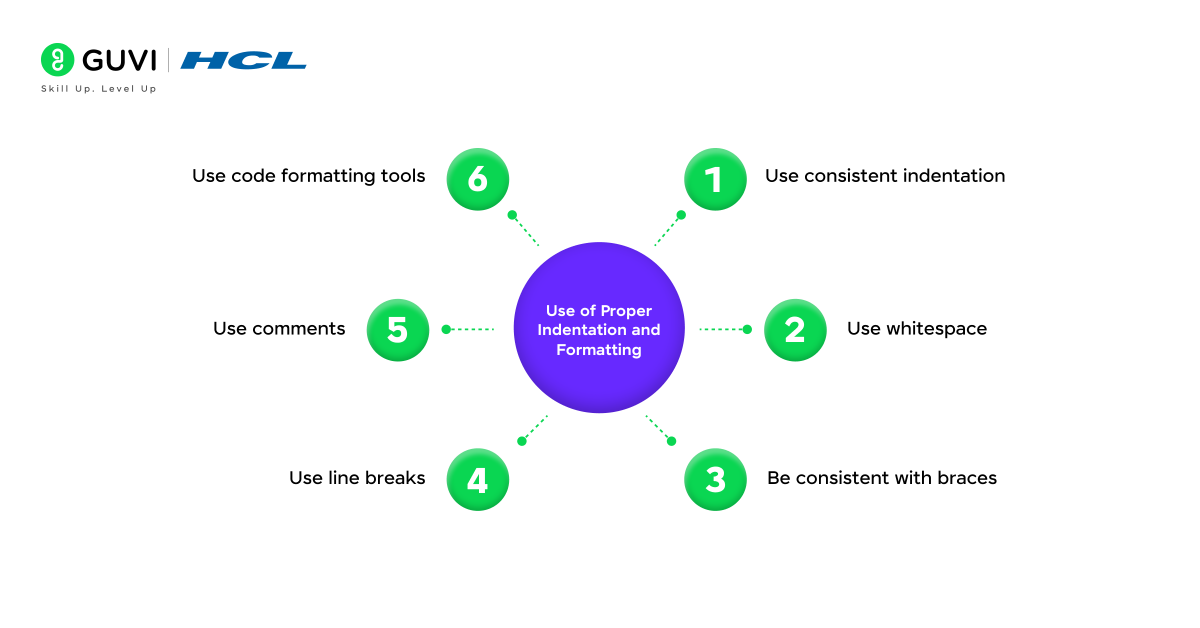
- Readability: Consistent formatting makes code easier to read and understand.
- Maintainability: Following a consistent style improves code maintainability and collaboration.
Example:
JavaScript
// Inconsistent formatting
if(condition){
doSomething();
} else {
doSomethingElse();
}
// Consistent formatting
if (condition) {
doSomething();
} else {
doSomethingElse();
}
5. DRY (Don’t Repeat Yourself) Principle
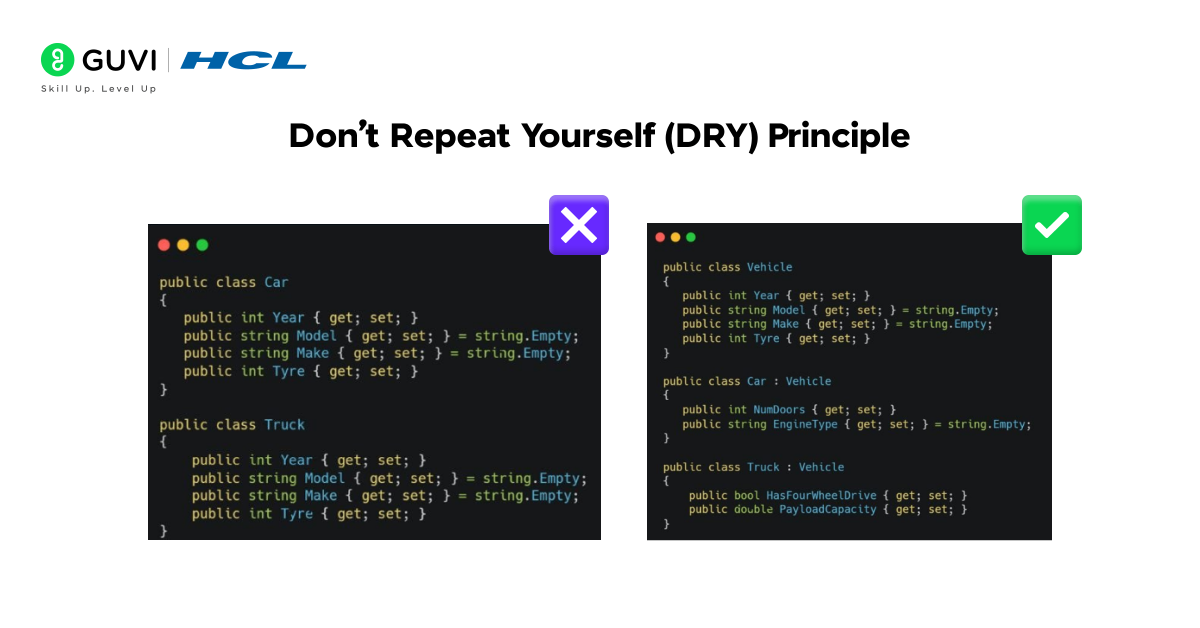
- Avoid Code Duplication: Extract common code into reusable functions or classes to reduce redundancy.
- Maintainability: DRY code is easier to maintain and modify.
- Consistency: Ensures consistent behavior across different parts of your codebase.
Example:
JavaScript
// Duplicated code
function calculateBookPrice(quantity, price) {
return quantity * price;
}
function calculateLaptopPrice(quantity, price) {
return quantity * price;
}
// Refactored code
function calculateItemPrice(quantity, price) {
return quantity * price;
}
6. Use Meaningful Whitespace
- Clarity: Use whitespace to visually separate logical sections of your code.
- Readability: Proper spacing makes code easier to scan and understand.
Example:
JavaScript
// Poor use of whitespace
const sum=function(a,b){return a+b;}
// Improved use of whitespace
const sum = function (a, b) {
return a + b;
};
7. Error Handling
- Anticipate Errors: Write code that anticipates potential errors and handles them gracefully.
- Informative Error Messages: Provide clear and helpful error messages to aid debugging.
- Error Logging: Log errors to track and troubleshoot issues.
Example:
JavaScript
try {
// Code that might throw an error
} catch (error) {
console.error("An error occurred:", error.message);
}
By following these principles, you can write code that is not only functional but also maintainable, scalable, and easy to work with. Clean code is an investment that pays off in the long run, leading to improved project outcomes and developer satisfaction.
Refactoring Techniques for Cleaner Code
Identifying Code Smells
Code smells are indicators of potential problems in your code. Some common code smells include:
- Duplicated Code
- Long Method
- Large Class
- Too Many Parameters
- Divergent Change
- Shotgun Surgery
- Feature Envy
- Data Clumps
Refactoring Patterns and Strategies
- Extract Method: Break down long methods into smaller, more focused methods.
- Extract Class: Split large classes into smaller, more cohesive classes.
- Replace Conditional with Polymorphism: Use polymorphism to handle complex conditional logic.
- Introduce Parameter Object: Group related parameters into a single object.
- Remove Middle Man: Eliminate unnecessary delegating methods.
Tools for Automated Refactoring
- IDE Refactoring Tools: Most modern IDEs offer built-in refactoring tools.
- Linters: Use linters like ESLint for JavaScript to identify and automatically fix code style issues.
- Static Analysis Tools: Tools like SonarQube can help identify complex code that might need refactoring.
Balancing Clean Code with Deadlines and Practicality
When to Prioritize Clean Code vs. Quick Delivery
- Understand the Context: Consider the project stage, team size, and long-term maintenance needs.
- Minimal Viable Cleanliness: Aim for a basic level of cleanliness even under tight deadlines.
- Refactor Opportunistically: Take opportunities to clean up code during feature additions or bug fixes.
- Communicate Trade-offs: Clearly communicate the implications of technical debt to stakeholders.
Technical Debt Management
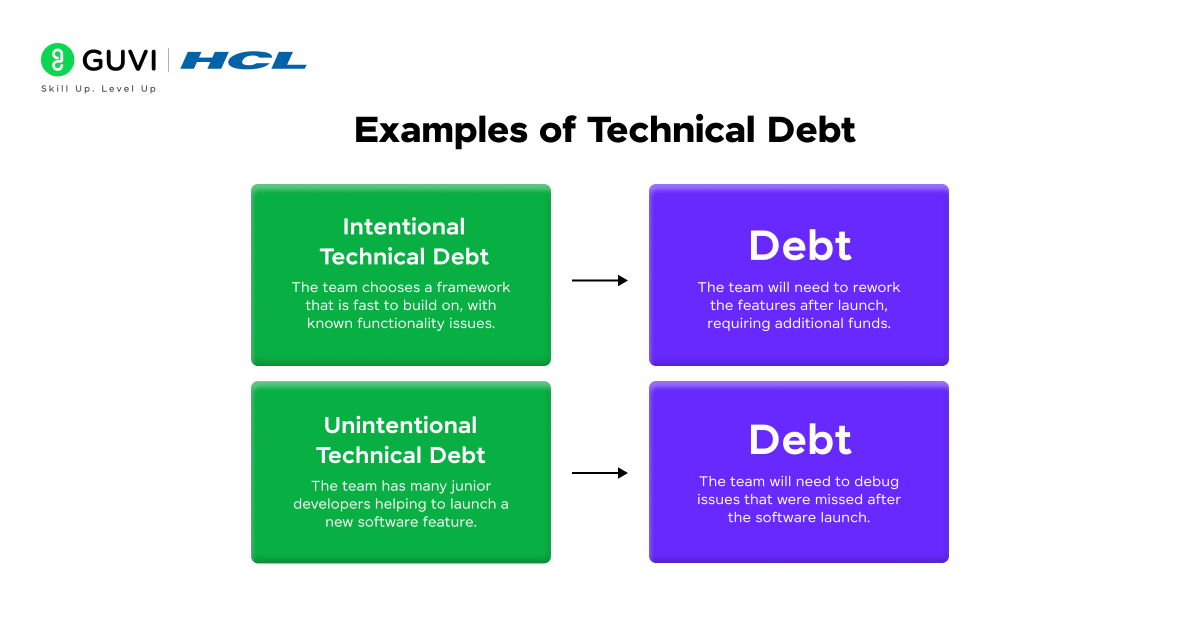
- Track Technical Debt: Use tools or maintain a backlog to track areas needing improvement.
- Regular Refactoring Sprints: Allocate time for regular code cleanup and refactoring.
- Boy Scout Rule: Leave the code a little better than you found it with each change.
- Measure and Communicate: Use metrics to show the impact of technical debt and the benefits of addressing it.
If you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript certification course.
Conclusion
Embrace clean code not as a set of rigid rules, but as a mindset that values clarity, simplicity, and craftsmanship. By doing so, you’ll not only improve your own full stack development skills but also contribute to a culture of excellence in your development team and the wider software community.
As you continue your journey in full stack development, keep refining your approach to clean code. Stay curious, keep learning, and always strive to leave the codebase better than you found it. Your future self and your teammates will thank you for it.
FAQs
What is Clean Code, and why is it important in Full Stack Development?
Clean Code refers to writing code that is simple, readable, and maintainable. It’s important in full stack development because it ensures better collaboration, faster debugging, and scalability of both front-end and back-end systems.
How do Clean Code principles enhance collaboration in a Full Stack team?
Clean Code promotes clear and consistent coding practices, making it easier for team members to understand each other’s code. This reduces miscommunication and helps speed up code reviews and collaborative development.
What are some common Clean Code practices for back-end development?
Key practices include meaningful naming conventions, avoiding long functions, keeping functions single-purpose, and ensuring modularity for easy testing and debugging.
How can Clean Code principles improve front-end code, like HTML, CSS, and JavaScript?
For front-end development, Clean Code principles help maintain structured and well-commented HTML, CSS, and JavaScript. This includes following consistent naming conventions for classes/IDs, minimizing inline styles, and organizing code to enhance readability and maintainability.
How can Full Stack developers implement Clean Code in complex projects?
Developers should prioritize refactoring, use design patterns, maintain clear documentation, and follow a consistent coding style. Adopting tools like linters, code formatters, and code reviews also helps to ensure Clean Code throughout complex projects.
Did you enjoy this article?