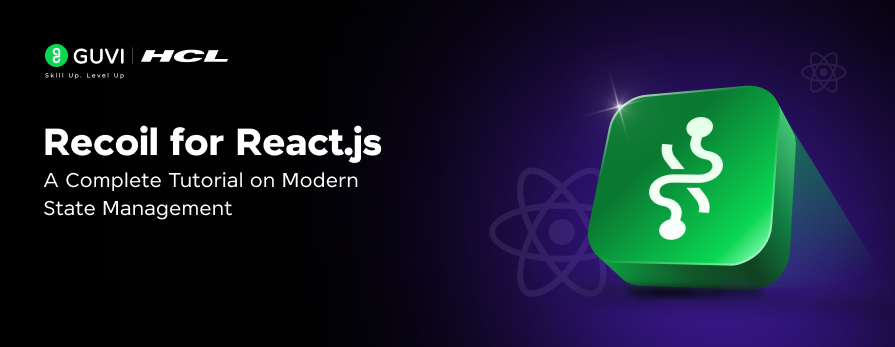
Recoil for ReactJS: A Complete Tutorial on Modern State Management
Dec 31, 2024 2 Min Read 2262 Views
(Last Updated)
You must already know about ReactJS which is a popular backend framework. There is a specific library called Recoil that helps in managing and sharing of components.
That is what we are going to see in this article, an in-depth analysis on Recoil for ReactJS. So, without further ado, let us get started!
Table of contents
- What is Recoil for ReactJS?
- Step-by-Step Guide to Install Recoil for ReactJS
- Step 1: Install Recoil
- Step 2: Set Up RecoilRoot
- Step 3: Create Atoms
- Step 4: Use Atoms in Components
- Step 5: Create a Component to Display the State
- Step 6: Combine Components in App
- Step 7: Run Your Application
- Additional Points: Choose based on your preferences
- Conclusion
What is Recoil for ReactJS?
Recoil is a state management library for React that provides a simple and efficient way to manage the global state. It allows you to share state across components without the need for prop drilling or complex context providers.
Prerequisites
Before you begin, ensure you have the following:
– Basic knowledge of React.js.
– Node.js and npm installed on your machine.
– A React project setup (you can create one using Create React App).
Step-by-Step Guide to Install Recoil for ReactJS
Step 1: Install Recoil
First, you need to install Recoil in your React project. Open your terminal and navigate to your project directory, then run the following command:
npm install recoil
or if you are using Yarn:
yarn add recoil
Step 2: Set Up RecoilRoot
Recoil requires a `RecoilRoot` component to be wrapped around your application. This component provides the context for Recoil state management.
Open your `src/index.js` (or `src/index.tsx` if you are using TypeScript) file and wrap your main application component with `RecoilRoot`:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { RecoilRoot } from 'recoil';
ReactDOM.render(
<RecoilRoot>
<App />
</RecoilRoot>,
document.getElementById('root')
);
Step 3: Create Atoms
Atoms are units of state in Recoil. You can think of them as pieces of state that can be read from and written to from any component.
Create a new file called `atoms.js` in your `src` directory:
// src/atoms.js
import { atom } from 'recoil';
export const textState = atom({
key: 'textState', // unique ID (with respect to other atoms/selectors)
default: '', // default value (aka initial value)
});
Step 4: Use Atoms in Components
Now that you have created an atom, you can use it in your components. You can read and write to the atom using the `useRecoilState` hook.
Here’s an example of a simple component that uses the `textState` atom:
// src/TextInput.js
import React from 'react';
import { useRecoilState } from 'recoil';
import { textState } from './atoms';
const TextInput = () => {
const [text, setText] = useRecoilState(textState);
const handleChange = (event) => {
setText(event.target.value);
};
return (
<div>
<input type="text" value={text} onChange={handleChange} />
<p>You typed: {text}</p>
</div>
);
};
export default TextInput;
Step 5: Create a Component to Display the State
You can create another component to display the current state of the `textState` atom:
const TextDisplay = () => {
const text = useRecoilValue(textState);
return <p>Current text: {text}</p>;
};
export default TextDisplay;
Step 6: Combine Components in App
Now, you can combine the `TextInput` and `TextDisplay` components in your main `App` component:
// src/App.js
import React from 'react';
import TextInput from './TextInput';
import TextDisplay from './TextDisplay';
const App = () => {
return (
<div>
<h1>Recoil Example</h1>
<TextInput />
<TextDisplay />
</div>
);
};
export default App;
Step 7: Run Your Application
Now that everything is set up, you can run your application to see Recoil in action. In your terminal, run:
npm start
or
yarn start
Open your browser and navigate to `http://localhost:3000`. You should see an input field where you can type text, and the current text will be displayed below it.
Additional Points: Choose based on your preferences
Redux :
For a more complex project, Redux might be the better choice due to its mature ecosystem, centralized state management, and extensive middleware support.
Recoil:
For a more complex project, Recoil might be the better choice due to its flexible atom-based state management, fine-grained control over re-renders, and seamless integration with React’s Concurrent Mode.
In case, you want to learn more about these frameworks and gain in-depth knowledge on full-stack development, consider enrolling for GUVI’s certified Full-stack Development Course that teaches you everything from scratch and make sure you master it!
Conclusion
Congratulations! You have successfully set up Recoil in your React.js project. You can now manage the global state easily without the complexity of prop drilling or context providers. Recoil also offers advanced features like selectors and asynchronous state management, which you can explore as you become more comfortable with the library.
Feel free to expand on this example by adding more atoms, selectors, and components to suit your application’s needs. Happy coding!
Did you enjoy this article?