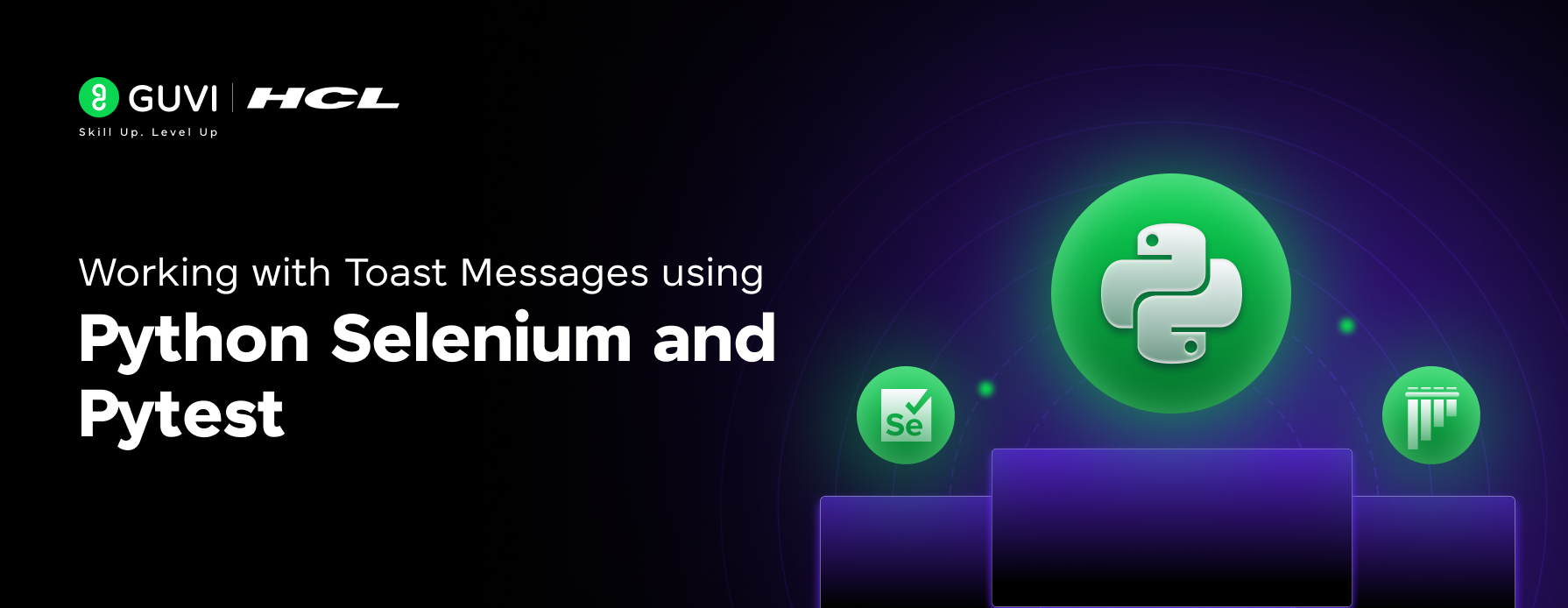
Working with Toast Messages using Python Selenium and Pytest
Oct 24, 2024 3 Min Read 716 Views
(Last Updated)
In the intricate world of user and interface, every interaction counts. A well-designed web application is not just about aesthetics but also it is about fluid communication as well. Enter the Toast Message, a seemingly small element that packs a powerful punch in conveying essential information to users.
A toast message is a brief non-intrusive notification that appears at the bottom or at the top of a web page for a very short duration.
It is quite interesting to learn so let us now see more about toast messages in this article!
Table of contents
- Introduction to Toast Messages
- Prerequisites
- The Automation Testing Code
- The Output
- Conclusion
Introduction to Toast Messages
Unlike traditional alert boxes, which disrupt the user’s flow, toast messages maintain focus on the current task while providing timely feedback. This simple, small yet subtle approach enhances user experience by avoiding interruptions and allowing users to continue their interactions seamlessly and hassle-free.
Toast messages are versatile tools with a wide range of applications. They can confirm successful actions such as saving a document or submitting a form. They can provide subtle guidance, like suggesting alternative options or prompting for further actions.
Additionally, toasts can inform users about errors or unexpected events without causing alarm. By delivering concise and relevant information, toasts empower users to make informed decisions and progress efficiently.
The effectiveness of toast messages lies in their brevity and clarity. A well-crafted toast conveys its message quickly and disappears before becoming intrusive. The design should complement the overall aesthetic of the application, ensuring consistency and visual appeal.
Furthermore, the placement of the toast message is crucial. Displaying it at the bottom of the screen allows users to focus on the main content while still being aware of the notification.
In conclusion, toast messages are indispensable components of modern web applications. Their ability to provide timely feedback without disrupting the user experience makes them invaluable for enhancing usability and user satisfaction.
By understanding the nuances of toast message design and implementation, developers can create applications that are both efficient and enjoyable for users.
Would you like to know how to implement toast messages in a specific web development framework?
Prerequisites
For carrying out the automation testing using Python for Toast Messages, we need a web application. For this example, I am using the demo URL https://sumantoastmessage.netlify.app/
We need PyCharm, VSCode, or any other code editor for the same.
Also, for the project we need to have Python Selenium, Python WebDriver Manager, and Pytest framework installed on our machine.
The project structure will comprise of
PageObjects : This folder will contain all the automation code inside the Python file “toast_messages.py”
TestCodes : This folder will contain the Pytest file “test_toast_messages.py” to generate the Pytest test-case report in HTML format.
Reports : This will contain the Pytest-generated HTML test-case report
The Automation Testing Code
Since we have created our project structure we are going to start coding for your project. To do so, we will write our Python Selenium automation scripts in the “toast_messages.py”. Let us now see the code and understand it in detail:-
""""
File Name : toast_messages.py
Program : Capturing & Generating Toast Messages using Python Selenium & Pytest
"""
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
# Python Class to hold the Locators
class Toast_Locators:
success_toast_message = "//div[@class='column']/span[text()='Success: This is a success toast.']"
error_toast_message = "//div[@class='column']//span[text()='Error: This is an error toast.']"
# Python Class for Automation Testing
class Suman_Toast_Messages(Toast_Locators):
def __init__(self, url):
self.url = url
self.driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
# Click the Success Button
def click_button(self):
self.driver.maximize_window()
self.driver.get(self.url)
self.driver.implicitly_wait(10)
self.driver.find_element(by=By.ID, value="success").click()
return True
# Verify whether the success toast message is visible or not
def toast_visible(self):
if self.driver.find_element(by=By.XPATH, value=self.success_toast_message).is_displayed():
return True
else:
return False
# Extract the Success Toast Message Text using Python Selenium
def extract_toast_text(self):
if self.toast_visible():
text_message = self.driver.find_element(by=By.XPATH, value=self.success_toast_message).text
print(text_message)
return text_message
else:
print("ERROR : Toast Message not found !")
The entire code has been written using Python’s Object Oriented Programming Methodology (OOPS). The need to use the OOPS is so that we cannot only make use of the Page Object Model (POM) but also make our automation testing reusable.
Import the Python modules like Selenium, WebDriver Manager, By and Service into the code. Here, we are using the Python Selenium WebDriver Manager to manage our WebDriver so that the driver can be downloaded and installed by the Python itself.
Create a Python Class called “Toast_Locators” which will handle all the XPATHs necessary for your automation.
Now, create the main Python Class “Suman_Toast_Messages” which will inherit the “Toast_Locator” class. In this class, we will create a constructor method which will have the URL of the web application and the driver object as well.
Now, the “click_button” method will maximize the Chrome browser window, run the web application using the implicit wait and will find the “Success” button and click it. The method code will return True.
The “toast_visible” method will return True when the “Success Message” is displayed on the screen, else it will return False.
The “extract_toast_message” will return a text message and will extract the text else it will throw an error message on the console.
Now, when the automation code is ready it is time to write our Pytest Test-Cases. The code is given as below:-
"""
File Name : test_toast_message.py
Program : Pytest program to validate the Toast Message automation
"""
import pytest
from PageObjects.toast_messages import Suman_Toast_Messages
url = "https://sumantoastmessage.netlify.app/"
suman = Suman_Toast_Messages(url)
def test_click_button():
assert suman.click_button() == True
print("SUCCESS : Automation started")
def test_toast_visible():
assert suman.toast_visible() == True
print("SUCCESS : success toast message is visible")
def test_extract_toast_text():
assert suman.extract_toast_text() == "Success: This is a success toast."
In the following code, we have to import the PageObjects module into our Pytest file. We have three test cases which are as follows:-
Test_click_button : This test case will assert and validate the click done on the “Success” button.
Test_Toast_Visisble : This test case will assert and validate the visibility of the “Success” message.
Test_Extract_Toast_Text : This test case will assert and validate the extracted text from the web application and validate with the user-given input.
The Output
When you will run the Pytest file “test_toast_messages.py” which is present inside the “TestCases” folder using the command pytest -v -s –capture=sys –html=../Reports/toast_messages.html test_toast_messages.py
You will see a Test-Case report in HTML format has been created inside the Reports folder.
The detailed Pytest report is given as below
In case you want to learn more about automation testing with Selenium, consider enrolling for GUVI’s certified Selenium Automation Testing Course online course that teaches you everything from scratch and also provides you with an industry-grade certificate!
Conclusion
In conclusion, we can see that working with toast messages and automating and testing them is important work for any automation tester and with the help of Python, the entire process is super easy and hassle-free.
Did you enjoy this article?