
30 Brilliant Game Developer Interview Questions and Answers
Nov 14, 2024 6 Min Read 74 Views
(Last Updated)
After the lockdown, one of the prominent fields that is growing exponentially, especially in India is the gaming industry. The role of a game developer is a high-demand one at the moment and in order to crack the interview, you need to master the game developer interview questions and answers.
When preparing for a game development interview, it’s essential to cover a mix of foundational knowledge, practical coding skills, and industry-standard techniques.
Below is a comprehensive list of game developer interview questions and answers tailored to help you feel confident for game developer roles at three experience levels: fresher, intermediate, and advanced. So, without further ado let us get started!
Table of contents
- Top 30 Game Developer Interview Questions and Answers
- Fresher Level
- Intermediate Level
- Advanced Level
- Conclusion
Top 30 Game Developer Interview Questions and Answers
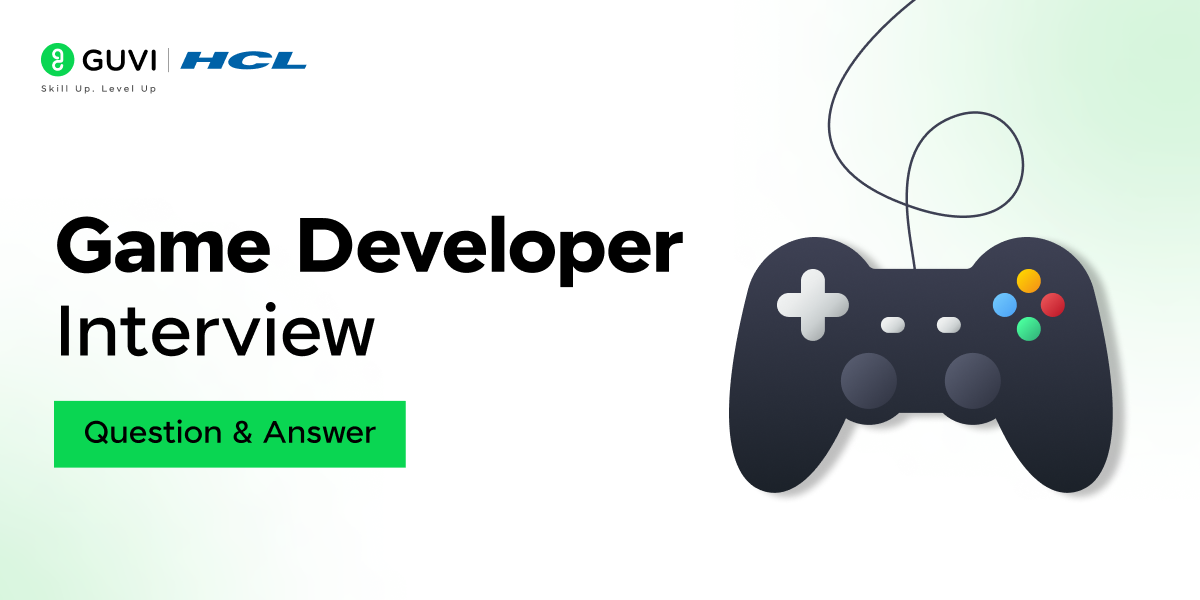
Here are 30 game developer interview questions designed to equip you with the essentials.
Fresher Level
These questions assess basic knowledge of game development principles, key terms, and general programming skills required for a successful game development career.
1. What is the Game Loop, and why is it important?
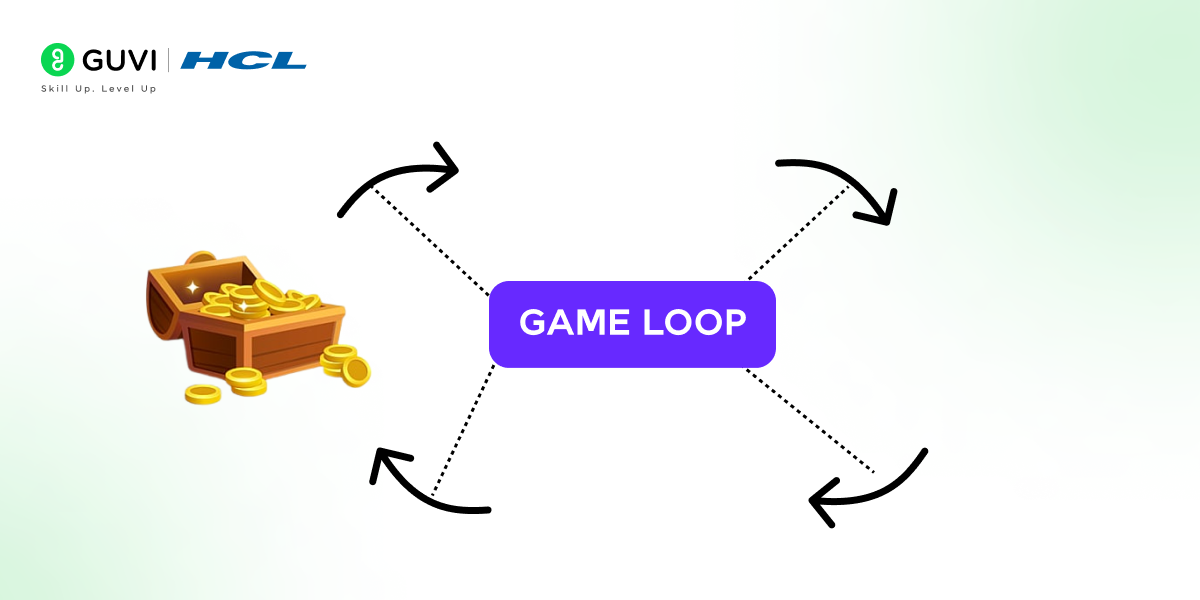
The game loop is a fundamental concept in game development that runs continuously to update the game state and render frames on the screen. It’s essential because it manages the real-time interaction between user inputs, physics, and graphics, making the game responsive and engaging.
2. Explain the concept of Frames Per Second (FPS). Why does it matter?
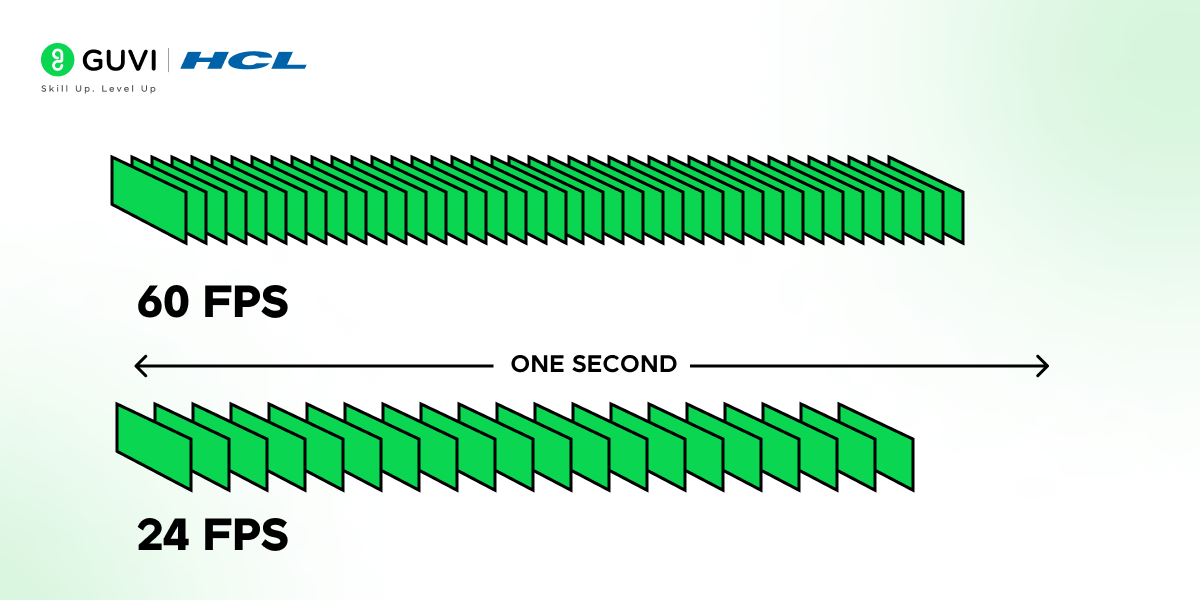
FPS is a measure of how many frames are rendered per second in a game. It matters because higher FPS ensures smoother motion and a better user experience, while lower FPS can make games feel laggy and unresponsive.
3. What is the difference between a Sprite and a Texture?
A sprite is an image or a 2D animation integrated into a game’s scene. A texture, on the other hand, is a flat image applied to a 3D model to give it color and detail. Sprites are usually used in 2D games, while textures are more common in 3D games.
4. Write a simple code to move a character forward in Unity using C#.
csharp
public class PlayerMovement : MonoBehaviour
{
public float speed = 5f;
void Update()
{
transform.Translate(Vector3.forward * speed * Time.deltaTime);
}
}
5. What are physics engines, and can you name a few used in game development?
A physics engine simulates physical systems within games, like collision detection, rigid body dynamics, and fluid simulation. Common physics engines include Unity’s built-in physics, Box2D, Havok, and PhysX.
Intermediate Level
These questions are designed to gauge your understanding of more advanced concepts and problem-solving abilities in game development.
6. Explain the difference between Object Pooling and Garbage Collection.
Object pooling is a design pattern that reuses game objects instead of creating and destroying them repeatedly. Garbage collection, however, is the automated process of freeing up memory by removing objects that are no longer in use. Object pooling helps reduce performance overhead caused by garbage collection in resource-intensive games.
7. What is a Shader, and why is it important in game development?
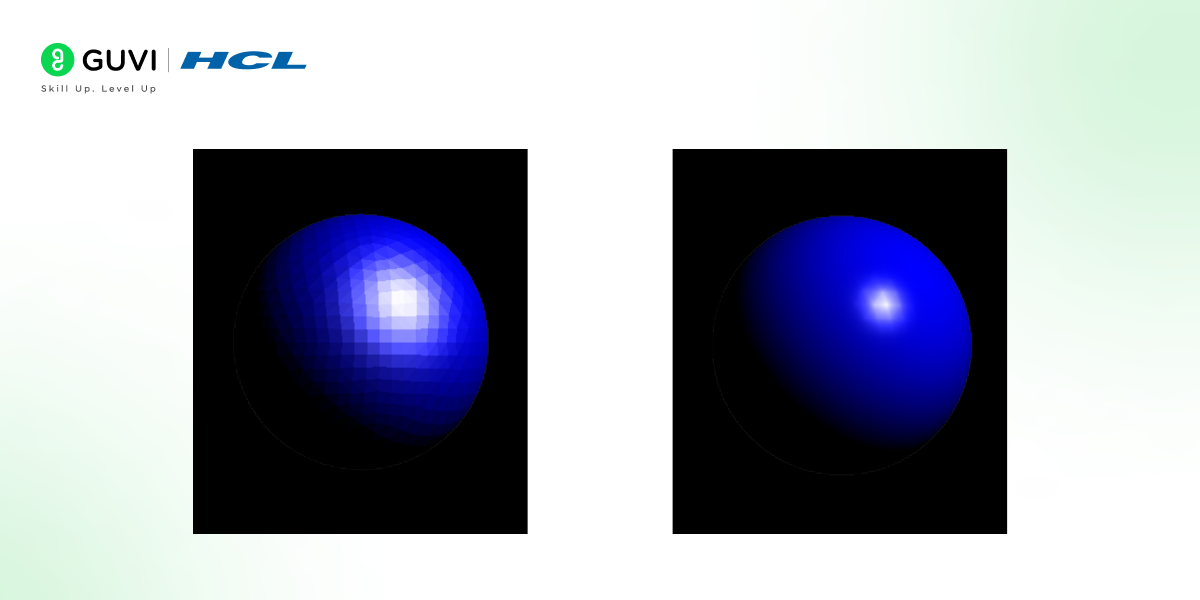
A shader is a type of program used to control the appearance of graphics, typically for 3D models. Shaders are crucial because they allow developers to create complex visual effects, such as lighting, shadows, and textures, to enhance the realism of the game.
8. Write a Unity C# script to detect collisions between two objects.
csharp
public class CollisionDetection : MonoBehaviour
{
void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.tag == "Enemy")
{
Debug.Log("Collision with enemy detected!");
}
}
}
9. How does the MVC (Model-View-Controller) pattern apply to game development?
The MVC pattern separates data (Model), the game’s visual interface (View), and user inputs (Controller). In games, this pattern allows for organized code and easy modifications, as each component can be independently changed without affecting the others.
10. What is the Level of Detail (LOD), and why is it used?
LOD is a technique that decreases the complexity of 3D models as they move farther from the camera. This optimization helps maintain high FPS by reducing unnecessary detail for objects that are less visible, preserving resources for rendering other game elements.
Advanced Level
These questions cover complex technical and design challenges to assess your problem-solving, optimization, and programming expertise in game development.
11. Describe the Entity-Component-System (ECS) architecture and its benefits in game development.
ECS is a design pattern where entities represent game objects, components contain data, and systems define the game logic. This architecture decouples data from behavior, making it easier to manage and optimize large-scale games by improving performance and code reusability.
12. What is Inverse Kinematics (IK), and how does it apply to game character animations?
Inverse kinematics is the process of calculating joint positions in character models to reach a specific point, often used to create realistic character animations. For example, when a character reaches for an object, IK adjusts the arm position accordingly.
13. How do you approach optimization in a game with performance issues?
Optimization typically involves profiling the game to identify bottlenecks, reducing draw calls, using level-of-detail models, managing memory usage, and implementing efficient algorithms. Specific optimizations might include culling unseen objects, using object pooling, and optimizing physics calculations.
14. Write a script in Unity C# to create a coroutine that pauses an action for 2 seconds before resuming.
csharp
using System.Collections;
using UnityEngine;
public class CoroutineExample : MonoBehaviour
{
void Start()
{
StartCoroutine(PauseAndResume());
}
IEnumerator PauseAndResume()
{
Debug.Log("Pausing...");
yield return new WaitForSeconds(2);
Debug.Log("Resuming!");
}
}
15. Explain how raycasting works in Unity and its practical applications.
Raycasting is a technique that casts an invisible ray from a point in a specified direction to detect objects along the ray’s path. In Unity, raycasting is often used to detect obstacles, implement shooting mechanics, and determine the line of sight for AI characters.
16. How would you implement memory management in a game with high resource demands?
Memory management involves reducing memory footprint by unloading unused assets, using asset bundles, pooling objects, and minimizing texture resolution. Profiling tools are essential to monitor memory usage and avoid leaks, especially in resource-constrained platforms like mobile devices.
17. Describe Procedural Generation and provide examples of its use in games.
Procedural generation is the creation of game content algorithmically rather than manually, often used for levels, environments, or textures. This approach is seen in games like Minecraft, where landscapes are generated dynamically, and No Man’s Sky, which features procedurally generated planets.
18. How do you manage multi-threading in game development?
Multi-threading enables games to perform tasks concurrently, such as AI processing or background loading, without affecting the main thread’s performance. In Unity, the Job System and Burst Compiler can be used for safe and efficient multi-threading. Synchronization is essential to avoid race conditions and maintain stability.
19. Explain the role of AI in NPC behavior and give an example of how it can be implemented.
AI in games allows NPCs to exhibit intelligent behaviors, enhancing the player’s immersion. This can be implemented using decision trees, state machines, or behavior trees. For instance, in a stealth game, an enemy AI might patrol an area, detect player presence, and switch states to “alert” or “chase” accordingly.
20. Write code to implement a finite state machine (FSM) for a simple enemy AI in Unity.
csharp
public class EnemyAI : MonoBehaviour
{
private enum State { Patrol, Chase, Attack }
private State currentState = State.Patrol;
void Update()
{
switch (currentState)
{
case State.Patrol:
Patrol();
break;
case State.Chase:
Chase();
break;
case State.Attack:
Attack();
break;
}
}
void Patrol() { /* Patrol logic */ }
void Chase() { /* Chase logic */ }
void Attack() { /* Attack logic */ }
}
21. Describe how multiplayer synchronization works and the challenges associated with it.
Multiplayer synchronization keeps all players’ game states consistent across different devices. Challenges include handling network latency, ensuring real-time updates, and managing desynchronization issues. Techniques like client prediction and server reconciliation are used to provide a smooth multiplayer experience.
22. How would you handle the challenge of rendering a large open-world environment?
Rendering large open worlds requires optimizations such as level-of-detail, occlusion culling, and streaming assets based on the player’s location. Additionally, spatial partitioning techniques like quadtrees or octrees can help manage and load portions of the environment dynamically.
23. What is the difference between forward rendering and deferred rendering, and when would you choose one over the other?
Forward rendering renders each object with all of its lights individually, which can be resource-intensive but provides better control over lighting on a per-object basis. Deferred rendering, on the other hand, handles lighting calculations in a separate pass, allowing for more dynamic lights in the scene at a lower cost.
Deferred rendering is often chosen for complex scenes with multiple lights, while forward rendering can be better for simpler scenes with fewer light sources.
24. How do you implement a particle system, and what are its common applications in game development?
A particle system simulates effects like fire, smoke, rain, or explosions by rendering many small, animated particles. In Unity, particle systems can be created using the Particle System component, which allows for control over particle size, color, movement, and lifespan. Particle systems enhance visual effects and improve game realism.
25. Explain the role of a NavMesh in AI pathfinding and how it is implemented in Unity.
A NavMesh, or Navigation Mesh, is a data structure used for AI navigation in games. It defines walkable areas, allowing AI agents to navigate around obstacles and follow paths. In Unity, NavMesh can be generated within the scene, and agents can be assigned with a NavMeshAgent component to follow the mesh and reach specific destinations.
26. How do you use quaternions to handle 3D rotations, and why are they preferred over Euler angles?
Quaternions are used in 3D rotations to represent orientations and rotations without the issues of gimbal lock, which can occur with Euler angles. Quaternions allow smooth, continuous rotations and are computationally efficient, making them ideal for 3D games where complex rotations are needed.
27. Write a Unity C# script to instantiate multiple enemies at random positions within a defined range.
csharp
using UnityEngine;
public class EnemySpawner : MonoBehaviour
{
public GameObject enemyPrefab;
public int numberOfEnemies = 5;
public float spawnRange = 10f;
void Start()
{
for (int i = 0; i < numberOfEnemies; i++)
{
Vector3 spawnPosition = new Vector3(
Random.Range(-spawnRange, spawnRange),
0,
Random.Range(-spawnRange, spawnRange)
);
Instantiate(enemyPrefab, spawnPosition, Quaternion.identity);
}
}
}
28. What is serialization in Unity, and why is it important for game development?
Serialization is the process of converting objects into a format that can be easily saved and loaded. In Unity, serialization is crucial for saving game states, settings, or user data. Unity supports serialization for fields marked with [SerializeField] or for classes that implement the ISerializable interface.
29. Explain the concept of state machines in game AI and provide an example of its application.
State machines are used to manage the different states of an AI character, such as Idle, Patrol, Attack, and Flee. Each state has its specific behavior and transitions to other states based on conditions. For instance, an enemy AI might transition from Patrol to Attack when it detects a player nearby, providing a structured way to control AI behavior.
30. Describe the process of implementing multiplayer networking in Unity and the challenges involved.
Implementing multiplayer networking in Unity involves synchronizing game states across multiple players over a network. Unity’s Netcode or third-party solutions like Photon or Mirror can be used. Challenges include managing latency, maintaining consistent game states, handling disconnects, and ensuring fair play in competitive environments.
If you want to learn more about Unity and game development, consider enrolling for GUVI’s Unity Online Course which teaches everything you need and will also provide an industry-grade certificate!
Conclusion
In conclusion, whether you’re just starting out or tackling advanced concepts, being well-prepared with these game developer interview questions and answers enables you to answer both theoretical knowledge and hands-on coding practice that will make a difference.
Remember, interviews often test not just your technical abilities but also your problem-solving skills, adaptability, and creativity in game development.
Did you enjoy this article?