
30 Software Developer Interview Questions and Answers
Nov 22, 2024 5 Min Read 80 Views
(Last Updated)
The world of software development is highly competitive and preparing for a software developer interview can be a daunting task, especially with the vast array of topics and questions that might come your way.
To help you navigate this process, we’ve compiled a comprehensive list of 30 software developer interview questions and answers, categorized by experience level: Fresher, Intermediate, and Advanced.
Table of contents
- Top 30 Software Developer Interview Questions and Answers
- Fresher Level
- Intermediate Level
- Advanced Level
- Conclusion
Top 30 Software Developer Interview Questions and Answers

Below is a list of 30 comprehensive software developer questions and answers that deemed fit for freshers to experienced professionals who’s looking to upskill their career. Let us have a look at them!
Fresher Level
1. What is the difference between == and === in JavaScript?
JavaScript’s == performs type conversion before comparing, while === compares both value and type directly. For instance, 5 == “5” is true, but 5 === “5” is false.
2. Explain object-oriented programming (OOP) concepts.
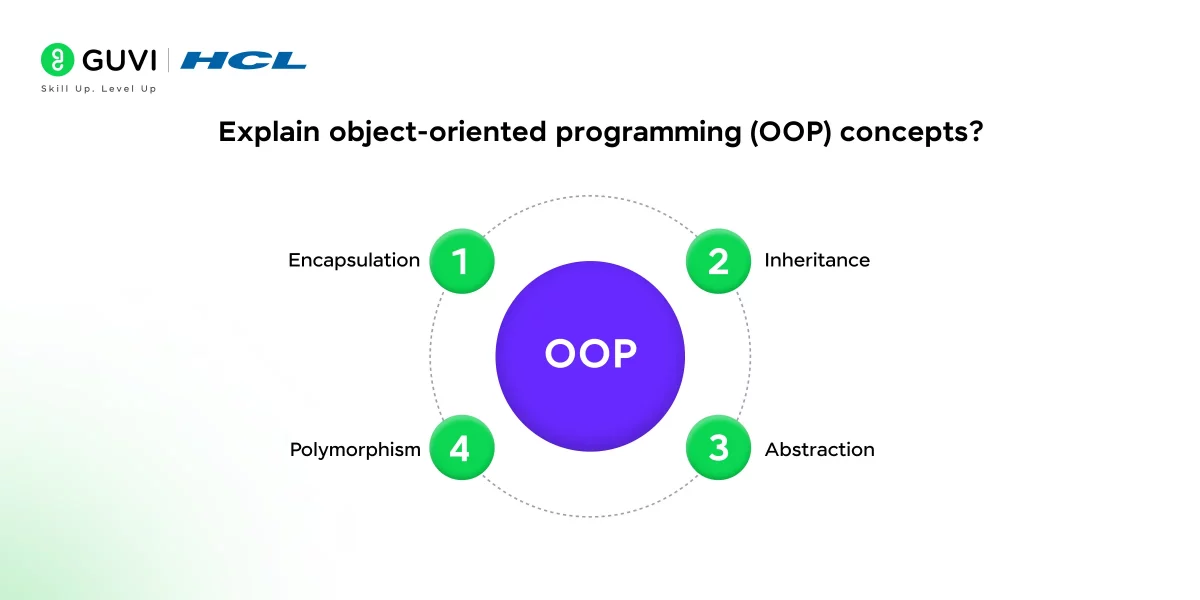
OOP is a programming paradigm based on four key principles: Encapsulation, Inheritance, Polymorphism, and Abstraction. These help create modular, reusable code and structure data as objects.
3. Write a function in Python to reverse a string.
def reverse_string(s):
return s[::-1]
print(reverse_string("Hello"))
This function uses Python’s slicing technique to reverse a string.
4. What is a data structure, and why is it important?
Data structures organize data efficiently, making it easier to access, modify, and store. Common data structures include arrays, lists, stacks, queues, and trees, each designed for specific use cases.
5. Can you explain the concept of an algorithm?
An algorithm is a step-by-step procedure to solve a problem or perform a task. Algorithms are crucial in programming, as they determine the efficiency and speed of your code.
6. Describe the differences between a stack and a queue.

A stack operates in a “last in, first out” (LIFO) manner, while a queue follows “first in, first out” (FIFO). For instance, adding books to a stack works from the top, while people joining a queue are served in order.
7. How does version control work, and why is it important?
Version control manages changes to code, allowing multiple developers to collaborate effectively. Git is a popular tool that records revisions and tracks who made changes.
8. Write a function in JavaScript to check if a number is even.
javascript
function isEven(num) {
return num % 2 === 0;
}
console.log(isEven(4)); // true
console.log(isEven(7)); // false
9. What is recursion, and can you give an example?
Recursion is a function calling itself to solve a problem. For example:
python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
print(factorial(5)) # 120
10. Explain the difference between an array and a linked list.
Arrays store data in contiguous memory locations, allowing for direct access via index. Linked lists, on the other hand, use nodes that contain data and pointers, making them more flexible for dynamic data insertion.
Intermediate Level
11. How would you improve the performance of a slow query in SQL?
Optimizations could include indexing relevant columns, using joins effectively, avoiding SELECT *, and removing unnecessary computations within the query.
12. What are design patterns? Give an example.
Design patterns are reusable solutions to common programming problems. For example, the Singleton pattern restricts instantiation of a class to one object, commonly used for database connections.
13. Write a function in JavaScript to find the maximum element in an array.
javascript
Copy code
function findMax(arr) {
return Math.max(...arr);
}
console.log(findMax([3, 5, 1, 8])); // 8
14. Explain the importance of Big O notation.
Big O notation describes an algorithm’s efficiency based on input size, helping developers predict runtime and optimize performance.
15. Describe the purpose of RESTful APIs.
RESTful APIs provide a standardized way to communicate between client and server via HTTP methods like GET, POST, PUT, and DELETE. This makes it easier to create scalable, modular applications.
16. What is dependency injection, and why is it used?
Dependency injection is a technique where dependencies are passed into a class, rather than being hard-coded. This makes testing and maintenance easier by allowing dependencies to be swapped or mocked.
17. Explain the difference between synchronous and asynchronous programming.
In synchronous programming, tasks execute one after another. In asynchronous programming, tasks can run in parallel, allowing other operations to continue without waiting for each task to complete.
18. Implement a function to check if a number is prime.
Python
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
# Example usage
print(is_prime(7)) # Output: True
19. Write a function to find the maximum value in a list of integers.
Python
def find_max(numbers):
if not numbers:
return None # Return None for an empty list
max_value = numbers[0]
for num in numbers:
if num > max_value:
max_value = num
return max_value
# Example usage
print(find_max([1, 5, 3, 9, 2])) # Output: 9
20. Implement a function to sort an array using the Bubble Sort algorithm.
Python
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
print(bubble_sort([64, 34, 25, 12, 22, 11, 90])) # Output: [11, 12, 22, 25, 34, 64, 90]
Advanced Level
21. What is multithreading, and why is it used?
Multithreading allows concurrent execution of multiple parts of a program to improve performance, especially in CPU-intensive tasks.
22. Explain the difference between optimistic and pessimistic locking in databases.
Optimistic locking assumes conflicts are rare, checking for changes before committing. Pessimistic locking assumes conflicts are frequent, locking records early to prevent them.
23. Write a function in Python to implement a binary search algorithm.
Python
def binary_search(arr, target):
low, high = 0, len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
print(binary_search([1, 2, 3, 4, 5], 4)) # Output: 3
24. Describe a time when you optimized an application. What was your approach?
You might discuss techniques like lazy loading, caching, code splitting, or database indexing—whatever best suits your experience.
25. What are microservices, and what benefits do they offer?
Microservices divide an application into independent services, each focusing on specific functions, enhancing scalability and flexibility for large applications.
26. Write a function to find the longest substring without repeating characters in a given string.
Python
def longest_unique_substring(s):
char_map = {}
start = max_length = 0
for end in range(len(s)):
if s[end] in char_map:
start = max(start, char_map[s[end]] + 1)
char_map[s[end]] = end
max_length = max(max_length, end - start + 1)
return max_length
# Example usage
print(longest_unique_substring("abcabcbb")) # Output: 3 ("abc")
27. Implement a function to perform binary search on a sorted list.
Python
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Element not found
# Example usage
print(binary_search([1, 2, 3, 4, 5, 6], 4)) # Output: 3
28. Write code to implement an LRU (Least Recently Used) cache in Python.
Python
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity):
self.cache = OrderedDict()
self.capacity = capacity
def get(self, key):
if key in self.cache:
value = self.cache.pop(key)
self.cache[key] = value
return value
return -1
def put(self, key, value):
if key in self.cache:
self.cache.pop(key)
elif len(self.cache) >= self.capacity:
self.cache.popitem(last=False)
self.cache[key] = value
29. What is a monolithic application, and how does it differ from microservices?
A monolithic application has tightly integrated components, while microservices split functionality into independent services, making updates easier but adding complexity to deployment.
30. Write a function to detect cycles in a linked list.
Python
class ListNode:
def __init__(self, x):
self.val = x
self.next = None
def has_cycle(head):
slow, fast = head, head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
if slow == fast:
return True
return False
# Example usage
# Create a linked list with a cycle for testing
node1 = ListNode(1)
node2 = ListNode(2)
node1.next = node2
node2.next = node1
print(has_cycle(node1)) # Output: True
These 30 software developer interview questions and answers help you crack any interview with ease and give you the confidence to appear with a strong will!
In case you want to learn more about development and programming languages, consider enrolling in GUVI’s Full Stack Developer online course that teaches you everything from scratch and equips you with all the necessary knowledge!
Conclusion
In conclusion, by preparing these questions across different levels, you can boost your confidence and showcase your knowledge effectively.
Remember, practice makes perfect, so take time to understand the concepts and try coding solutions on your own to reinforce your learning. Good luck with your interview preparation!
Did you enjoy this article?