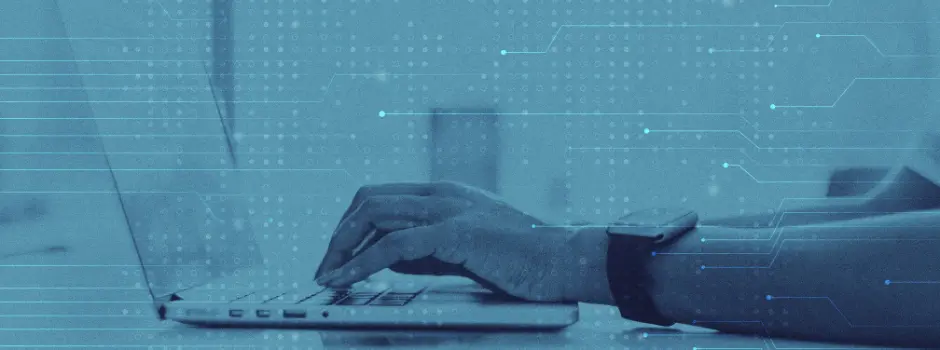
The Asynchronous IO Revolution : How Python is Changing the Game
Mar 07, 2025 5 Min Read 1062 Views
(Last Updated)
In the world of programming, efficiency and performance are paramount. Traditional synchronous programming models can often bottleneck any applications especially when dealing with tasks that involve waiting for external resources like I/O operations, network requests, or database queries. This is where asynchronous programming comes into play.
Asynchronous programming allows multiple tasks to be executed concurrently without blocking the main thread of execution. Instead of waiting for a task to finish before moving on to the next, the program can switch to other tasks making efficient use of system resources. This is particularly beneficial for I/O-bound applications where the CPU is often idle while waiting for external resources.
Table of contents
- Python's Asynchronous Capabilities
- Key Benefits of Asynchronous Programming in Python
- Python Async IO : Where Does It Fit In ?
- I/O-Bound Applications:
- Concurrency Without Threads:
- Integration with Other Libraries:
- Asynchronous Programming Patterns:
- When Not to Use asyncio:
- The asyncio Package and async/await
- Conclusion
Python’s Asynchronous Capabilities
Python, a versatile and popular language, has embraced asynchronous programming through the asyncio module. This module provides a high-performance framework for writing asynchronous code in Python. It introduces concepts like coroutines, event loops, and futures, which are essential for understanding asynchronous programming in Python.
Coroutines
Coroutines are functions that can be paused and resumed at arbitrary points. In the context of asynchronous programming, they are used to represent asynchronous tasks. When a coroutine encounters an asynchronous operation, it yields control back to the event loop, allowing other tasks to be executed. When the asynchronous operation completes, the coroutine is resumed with the result.
Event Loops
The event loop is the heart of asynchronous programming in Python. It is responsible for managing the execution of coroutines and handling asynchronous events. It continuously checks for completed asynchronous operations and resumes the corresponding coroutines.
Futures
Futures are objects that represent the result of an asynchronous operation. They provide a way to get the result of a coroutine or asynchronous task once it has completed.
Key Benefits of Asynchronous Programming in Python
- Improved Performance: Asynchronous programming can significantly enhance the performance of I/O-bound applications by allowing the program to handle multiple tasks concurrently.
- Scalability: Asynchronous programs can handle a larger number of concurrent connections or requests without compromising performance.
- Responsiveness: By avoiding blocking operations, asynchronous programs can maintain a responsive user interface, even under heavy load.
- Efficient Resource Utilization: Asynchronous programming can make better use of system resources by allowing the program to switch between tasks while waiting for external resources.
Python Async IO : Where Does It Fit In ?
Python’s asyncio module provides a framework for writing asynchronous code, a paradigm that allows multiple tasks to run concurrently without blocking each other. This is particularly useful for I/O-bound tasks, such as network requests or file operations.
Here’s a comprehensive breakdown of where asyncio fits in:
1. I/O-Bound Applications:
- Web Servers and APIs: asyncio is well-suited for building high-performance web servers and APIs that can handle many concurrent connections without blocking.
- Network Applications: It’s ideal for applications that need to interact with other systems over the network, such as clients for messaging protocols or distributed systems.
- File I/O: asyncio can efficiently handle file I/O operations, especially when dealing with a large number of files or when reading and writing data in chunks.
2. Concurrency Without Threads:
- Avoiding Global Interpreter Lock (GIL): Unlike traditional threading in Python, asyncio doesn’t suffer from the GIL, which limits the execution of multiple threads to a single core. This makes it more efficient for I/O-bound tasks.
- Simpler Programming Model: asyncio’s event-driven model simplifies the programming of concurrent tasks compared to using threads, reducing the complexity of dealing with synchronization and deadlocks.
3. Integration with Other Libraries:
- Web Frameworks: Many popular web frameworks like Django and Flask have integrations with asyncio, allowing you to build asynchronous web applications.
- Database Libraries: Some database libraries, such as aiopg for PostgreSQL, provide asynchronous interfaces that can be used with asyncio.
- Network Libraries: Libraries like aiohttp and aiosqlite offer asynchronous versions of HTTP and SQLite, respectively.
4. Asynchronous Programming Patterns:
- Coroutines: The core building block of asyncio is the coroutine, a special type of function that can be paused and resumed at specific points.
- Event Loops: The event loop is responsible for managing the execution of coroutines and handling events like network connections and timers.
- Futures: Futures represent the result of an asynchronous operation and can be used to wait for the result or handle exceptions.
5. When Not to Use asyncio:
- CPU-Bound Tasks: If your application is primarily CPU-bound (e.g., intensive calculations), asyncio might not provide significant performance benefits. In such cases, using multiple threads might be more appropriate.
- Simple Sequential Tasks: For simple, sequential tasks that don’t involve I/O operations, using asyncio can introduce unnecessary complexity.
The asyncio Package and async/await
Python’s asynchronous programming paradigm, introduced in version 3.4, is a powerful tool for handling non-blocking operations efficiently. The asyncio package, along with the async and await keywords, provides a structured approach to defining, constructing, and executing asynchronous code.
The async keyword is used to declare a function as asynchronous, indicating that it can be paused during execution to allow other tasks to proceed. The await keyword is employed within asynchronous functions to pause execution until a specific asynchronous operation completes. This mechanism enables efficient resource utilization and prevents blocking the entire application while waiting for time-consuming tasks to finish.
Async IO, or asynchronous input/output, is a programming paradigm that allows programs to handle multiple tasks concurrently without blocking the main thread. At the core of async IO are coroutines specialized functions that can pause their execution and yield control to other coroutines. This allows for efficient non-blocking operations, especially when dealing with tasks like network requests or file I/O.
To understand coroutines, think of them as functions that can take a break and resume later, much like a generator function. However, unlike generators, coroutines can interact with other coroutines and the main event loop to achieve asynchronous behavior.
To get started with async IO, let’s dive into a simple “Hello World” example. This program will demonstrate the basic principles of coroutines and how they work within the async IO framework.
Here, is a simple code in Synchronized way of Python programming:-
import time
class SumanNumberCounter:
def count(self):
print(“One”)
time.sleep(1)
print(“Two”)
def main():
counter = SumanNumberCounter()
for i in range(3):
counter.count()
if __name__ == “__main__”:
s = time.perf_counter()
main()
elapsed = time.perf_counter() – s
print(f”Code executed in {elapsed:0.2f} seconds.”)
Here is what happened during code execution :-
- We created a SumanNumberCounter class.
- The count function is now a method defined within the SumanNumberCounter class.
- In the main function, we create an instance of the SumanNumberCounter class using counter = SumanNumberCounter().
- We then call the count method on the object counter three times.
Here, is a simple code in ASynchronized way of Python programming:-
import asyncio
import time
class SumanAsycCounter:
def __init__(self):
self.count = 1
async def print_and_wait(self):
print(self.count)
self.count += 1
await asyncio.sleep(1)
async def main():
tasks = []
for i in range(3):
counter = SumanAsycCounter()
tasks.append(counter.print_and_wait())
await asyncio.gather(*tasks)
if __name__ == “__main__”:
s = time.perf_counter()
asyncio.run(main())
elapsed = time.perf_counter() – s
print(f”Code Executed in {elapsed:0.2f} seconds.”)
Here is what happened during code execution :-
- asyncio: This module provides facilities for writing asynchronous code in Python. It’s used to manage concurrent tasks efficiently.
- time: This module provides functions for working with time. Here, time.perf_counter() is used to measure the execution time of the asynchronous code.
Class name: SumanAsycCounter indicates its purpose: an asynchronous counter.
Constructor (__init__ method):
- Initializes an instance attribute self.count to 1. This attribute will be used to keep track of the counter value.
Asynchronous method (async def print_and_wait):
- This method will be called asynchronously (explained later).
- print(self.count): Prints the current value of the counter.
- self.count += 1: Increments the counter value by 1.
- await asyncio.sleep(1): This line pauses the execution of the current task asynchronously for 1 second. Other tasks can continue running while this task is paused.
- async def main(): Declares an asynchronous function named main.
- tasks = []: Creates an empty list to store references to the asynchronous tasks.
- Loop (for i in range(3)):
- Creates three instances of SumanAsycCounter (one for each iteration).
- Calls the counter.print_and_wait() method for each instance and appends it to the tasks list. Here, the method calls are scheduled, but they don’t necessarily execute immediately because they are asynchronous.
- await asyncio.gather(*tasks):
- This line uses the asyncio.gather function to wait for all the tasks in the tasks list to finish executing. It’s asynchronous, meaning other tasks can still run while waiting.
Conditional Execution:
Python
- if __name__ == “__main__”:
- s = time.perf_counter()
- asyncio.run(main())
- elapsed = time.perf_counter() – s
- print(f”Code Executed in {elapsed:0.2f} seconds.”)
Use code with super caution.
- This block ensures the code inside it runs only when the script is executed directly (not imported as a module).
- s = time.perf_counter(): Starts a timer to measure execution time.
- asyncio.run(main()): This line calls the asyncio.run function, which takes over the event loop and runs the asynchronous code defined in the main function. This effectively starts the asynchronous execution of the tasks.
- elapsed = time.perf_counter() – s: Stops the timer and calculates the elapsed time.
- print(f”Code Executed in {elapsed:0.2f} seconds.”): Prints the time it took for the code to execute.
This code demonstrates the use of asynchronous programming in Python Course to create three independent counters that print their values sequentially with a 1-second delay between each print. Asynchronous programming allows the program to run these tasks concurrently, potentially improving performance in situations where tasks can be paused or waiting for external events (like I/O).
Conclusion
The asynchronous I/O revolution in Python has fundamentally transformed the landscape of concurrent programming. By introducing the asyncio library and the async and await keywords, Python has made it easier to write efficient, non-blocking code. This shift is particularly impactful for I/O-bound applications, such as web servers and real-time data processing systems, where traditional synchronous methods often lead to performance bottlenecks.
Asynchronous I/O allows multiple tasks to run concurrently without the overhead of multithreading or multiprocessing. The asyncio library provides a robust framework for managing asynchronous operations, with features like event loops and coroutines that facilitate cooperative multitasking. The clear and concise syntax of async and await has lowered the barrier to entry, enabling more developers to harness the power of asynchronous programming.
This paradigm shift has also spurred the development of high-performance frameworks like FastAPI and Sanic, which leverage asynchronous I/O to handle numerous simultaneous connections efficiently. Additionally, a growing ecosystem of asynchronous libraries, such as aiohttp and aiomysql, supports the development of fully asynchronous applications.
Did you enjoy this article?