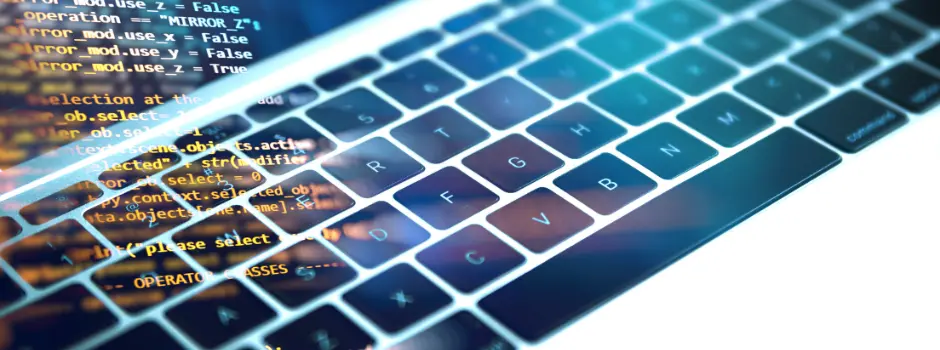
In Node.js, the Buffer
class, which is part of the global namespace, provides various methods for creating, reading, writing, and manipulating buffers. For example, you can convert between different encodings like UTF-8, Base64, and hexadecimal, or even slice and concatenate buffers efficiently.
Understanding buffers is essential for JavaScript developers working on backend systems or any application that requires robust data handling at the binary level.
In this blog, we’ll explore about Buffers in JavaScript, and its characteristics. You can also learn to create buffers, and access data through buffers. Let’s explore in-depth:
Table of contents
- What are Buffers in JavaScript?
- Characteristics of Buffers
- Creating buffers
- Accessing Buffer data
- Methods on Buffers
- Buffer pooling
- Summary
What are Buffers in JavaScript?
In Node.js, a buffer is a global object that offers a direct method of working with binary data. Buffers are significant because JavaScript traditionally lacked the built-in capability of dealing with raw binary data.
It is beneficial for managing raw data outside of the memory heap of the V8 JavaScript engine. It represents a fixed-length series of bytes. Buffers are implemented as a subclass of JavaScript’s Uint8Array, allowing them to use the underlying typed array capability while extending it with methods specific to binary data handling.
As a high-level language, JavaScript is built to handle data by default in UTF-16 string encoding. However, when dealing with I/O tasks, such as reading files or managing network data, the data happens to be in binary format. JavaScript can work with this binary data thanks to buffers.
Characteristics of Buffers
- Fixed Length: A buffer’s size cannot be altered after it has been created.
- Raw Memory Allocation: Buffers are allotted outside of the V8 heap, enabling effective binary data management.
- Global Availability: The Buffer class is globally available in Node.js, meaning it can be used without requiring an import statement.
- Support for Data Types: Strings, arrays, and other buffers can all be used for creating buffers.
Creating buffers
Buffer.alloc(size): Creates a buffer of a specified size (in bytes), filled with zeroes.
const buf = Buffer.alloc(10); // Creates a buffer of 10 bytes filled with zeroes console.log(buf); // <Buffer 00 00 00 00 00 00 00 00 00 00> |
Buffer.from(array): Creates a buffer from an array of bytes.
const buf = Buffer.from([20, 56, 89, 28]); console.log(buf); // <Buffer 14 38 59 1c> |
Buffer.from(string, [encoding]): Creates a buffer from a string, with optional encoding.
const buf = Buffer.from(‘Hello’); console.log(buf); // <Buffer 48 65 6c 6c 6f> |
Buffer.allocUnsafe(size): a buffer of a specified size is created, but it is not initialized, therefore it can contain outdated data.
const buf = Buffer.allocUnsafe(10); // Fast but may contain old data console.log(buf); // <Buffer random memory data> |
Accessing Buffer data
In a buffer, we retrieve individual bytes in the same way that you retrieve elements of an array.
const buf = Buffer.from(‘Hello’); console.log(buf[0]); // 72 (ASCII code for ‘H’) |
Modifying buffer data
buf[0] = 65; // Modifies the first byte to represent ‘A’ console.log(buf.toString()); // ‘Aello’ |
Methods on Buffers
Buffers come with a variety of useful methods for manipulating and interacting with binary data
buf.toString([encoding], [start], [end]): Converts the buffer data into a string. By default, the encoding is utf-8.
const buf = Buffer.from(‘Hello World’); console.log(buf.toString()); // ‘Hello World’ console.log(buf.toString(‘utf8’, 0, 5)); // ‘Hello’ |
buf.slice([start], [end]): Returns a new buffer that references the same memory as the original buffer, but is sliced from start to end.
const buf = Buffer.from(‘Hello World’); const slice = buf.slice(0, 5); console.log(slice.toString()); // ‘Hello’ |
buf.length: Returns the length (in bytes) of the buffer.
const buf = Buffer.from(‘Hello’); console.log(buf.length); // 5 |
Buffer pooling
Reusing pre-allocated memory buffers to enhance efficiency and lessen memory fragmentation during binary data processing is known as buffer pooling in Node.js. Node.js could allocate a bigger memory pool and give you smaller slices from it when you create a small buffer. Lowering the requirement for frequent memory allocations enhances performance. This method works especially well in high-performance applications where frequent memory allocation and deallocation might cause overhead and inefficiencies.
In case, you want to learn more about “buffers” and gain in-depth knowledge on full-stack development, consider enrolling for GUVI’s certified Full Stack Development Course that teaches you everything from scratch and make sure you master it!
Summary
- Buffers are used to store and manipulate binary data.
- Important in Node.js for handling I/O operations with raw data.
- Buffers have fixed sizes and can be created using Buffer.alloc() , Buffer.from() , etc.
- They offer a variety of methods for reading, writing, slicing, and manipulating data.
- Buffers are tightly integrated with streams, which allows efficient processing of large files or network data.
Did you enjoy this article?