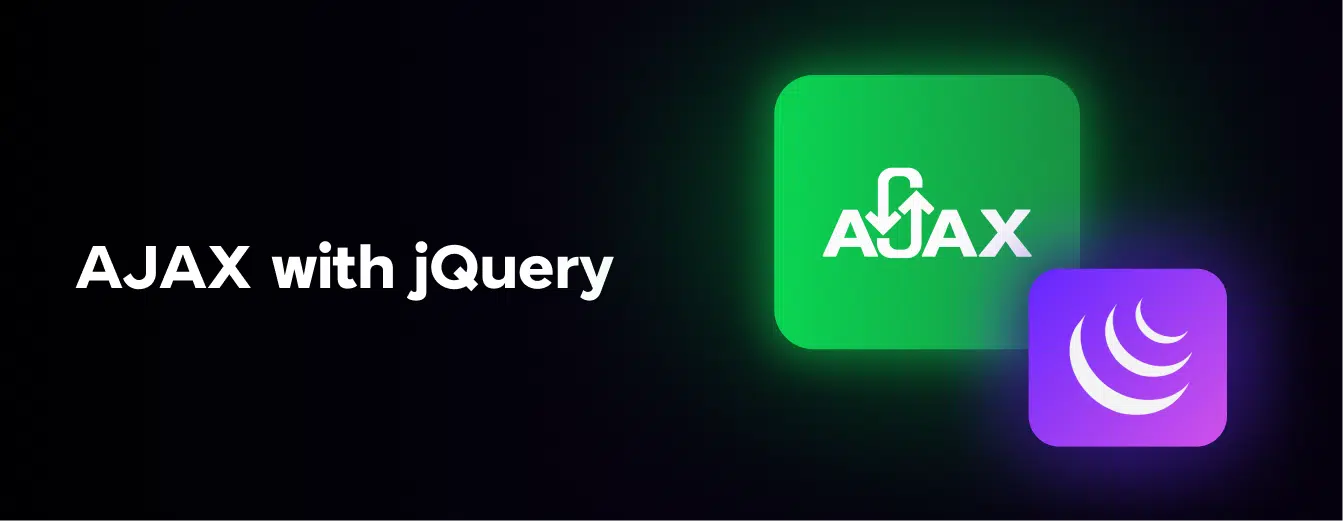
AJAX with jQuery: A Comprehensive Guide for Dynamic Web Development
Mar 13, 2025 8 Min Read 5637 Views
(Last Updated)
In today’s digital world, users expect web applications to be dynamic and responsive. AJAX (Asynchronous JavaScript and XML) is key to achieving this, allowing for real-time updates without constant page reloads. However, AJAX can be complex to implement due to its syntax and cross-browser issues.
This is where jQuery comes in. As a powerful JavaScript library, jQuery simplifies AJAX, making it easier and more efficient to use. In this blog, we’ll explore the basics of AJAX and how jQuery enhances its functionality.
This blog will equip you with the skills to create dynamic and interactive web applications using AJAX with jQuery.
Table of contents
- What is AJAX?
- What is jQuery?
- AJAX with jQuery
- Setting Up Your Environment
- Basics of AJAX with jQuery
- Handling Different Request Types
- Working with JSON Data
- Handling Responses
- Chaining AJAX Calls
- Using jQuery's Convenience Methods
- Error Handling and Debugging
- Security Considerations
- Conclusion
- FAQs
- What is AJAX and how does jQuery simplify its implementation?
- How can I handle AJAX errors effectively using jQuery?
- What are the security considerations when using AJAX with jQuery?
What is AJAX?
AJAX (Asynchronous JavaScript and XML) is a set of web development techniques that allows web applications to transfer data between a web browser and a server asynchronously, without requiring the entire web page to be reloaded. It enables the creation of dynamic and responsive web applications that can update content seamlessly without disrupting the user experience.
The core component of AJAX is the XMLHttpRequest object (or its modern equivalents like the Fetch API), which allows JavaScript to send HTTP requests to a web server and retrieve data from the server after the page has loaded. This data can be formatted as XML, JSON, HTML, or plain text, and can be used to update specific sections of a web page without refreshing the entire page.
Here’s a basic overview of how AJAX works:
- Event Handling: An event occurs on the web page, such as a user clicking a button or submitting a form.
- XMLHttpRequest Object Creation: The JavaScript code creates an XMLHttpRequest object (or uses the Fetch API) to facilitate the asynchronous data transfer.
- Sending a Request: The XMLHttpRequest object sends an HTTP request to the server, typically using methods like GET or POST.
- Server Processing: The server processes the request and prepares the response data.
- Response Handling: Once the response is received from the server, a callback function in JavaScript handles the response and updates the page content accordingly, without reloading the entire page.
AJAX allows web applications to be more responsive and efficient by reducing the need for full-page refreshes and providing a smoother user experience. It is widely used in modern web development for features like auto-complete suggestions, real-time form validations, live updates (e.g., news feeds, chat applications), and dynamic user interfaces.
Ready to unlock your potential? Enroll in GUVI’s Full Stack Development Course now to master the skills needed for a successful tech career.
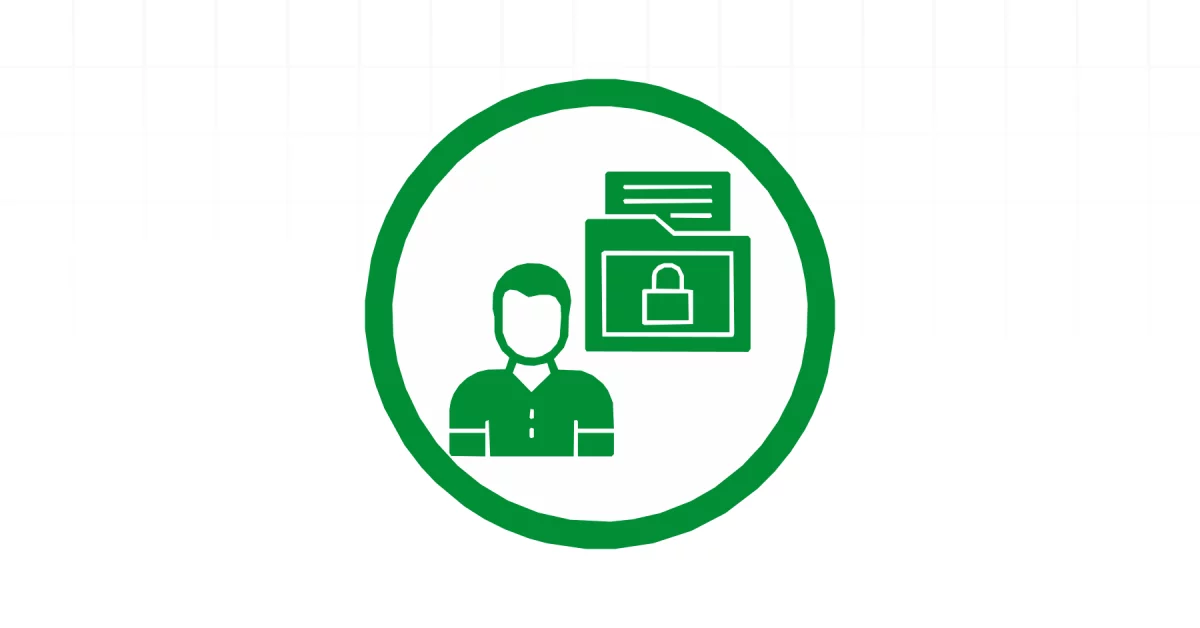
Now that we’ve covered the basics of AJAX, let’s explore another essential tool for web developers: jQuery.
What is jQuery?
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies things like HTML document traversal and manipulation, event handling, animation, and Ajax with an easy-to-use API that works across many browsers.
Some key features and advantages of jQuery include:
- DOM Manipulation: jQuery simplifies client-side scripting of HTML by providing a convenient syntax for traversing and modifying the Document Object Model (DOM).
- Event Handling: jQuery offers a straightforward way to attach event handlers to DOM elements, simplifying tasks like creating interactive web pages.
- AJAX: jQuery provides a clean and simple API for making AJAX requests, handling responses, and updating page content dynamically.
- Animations: jQuery comes with built-in animation capabilities, allowing developers to create simple and complex animations with minimal code.
- Cross-Browser Compatibility: jQuery abstracts away cross-browser inconsistencies, enabling developers to write code that works consistently across different browsers and versions.
- Plug-in Ecosystem: jQuery has a vast ecosystem of plugins and extensions that provide additional functionality, ranging from form validation to image sliders and more.
- Chaining: jQuery supports method chaining, which allows you to string multiple operations together in a single statement, resulting in more concise and readable code.
- Selectors: jQuery provides a powerful and concise syntax for selecting DOM elements, similar to CSS selectors, making it easy to target specific elements on a page.
While jQuery was once found everywhere in web development, with the advancement of modern browsers and the rise of newer JavaScript standards and libraries, its usage has declined in recent years. However, jQuery is still widely used, especially in older web applications and projects, and it continues to be maintained and supported by its active community.
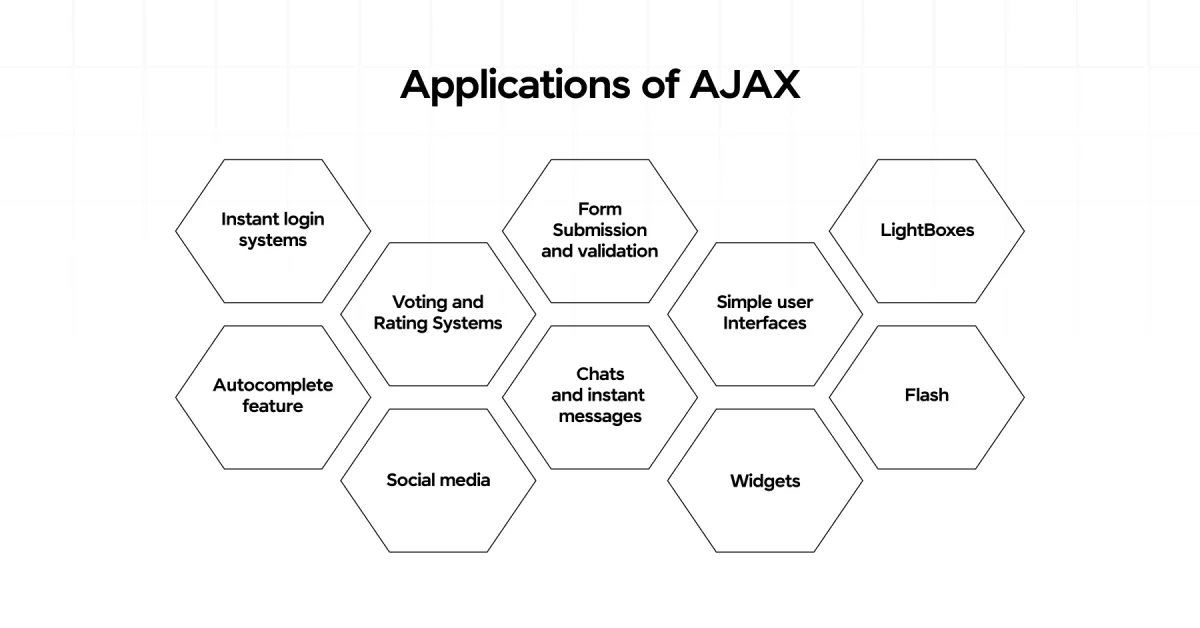
Now that we have a solid understanding of what jQuery is and how it simplifies JavaScript programming, let’s explore one of its most powerful features: using AJAX with jQuery.
AJAX with jQuery
Let’s explore AJAX with jQuery, covering everything from setting up your environment to handling different request types, working with JSON data, chaining AJAX calls, and more.
1. Setting Up Your Environment
Before getting into AJAX with jQuery, let’s ensure we have the necessary setup in place. First, you’ll need to include the jQuery library in your web project. You can either download the library from the official jQuery website (https://jquery.com/) or include it via a Content Delivery Network (CDN) link, such as:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Once you’ve included the jQuery library, you’re ready to start using its powerful capabilities, including AJAX functionality.
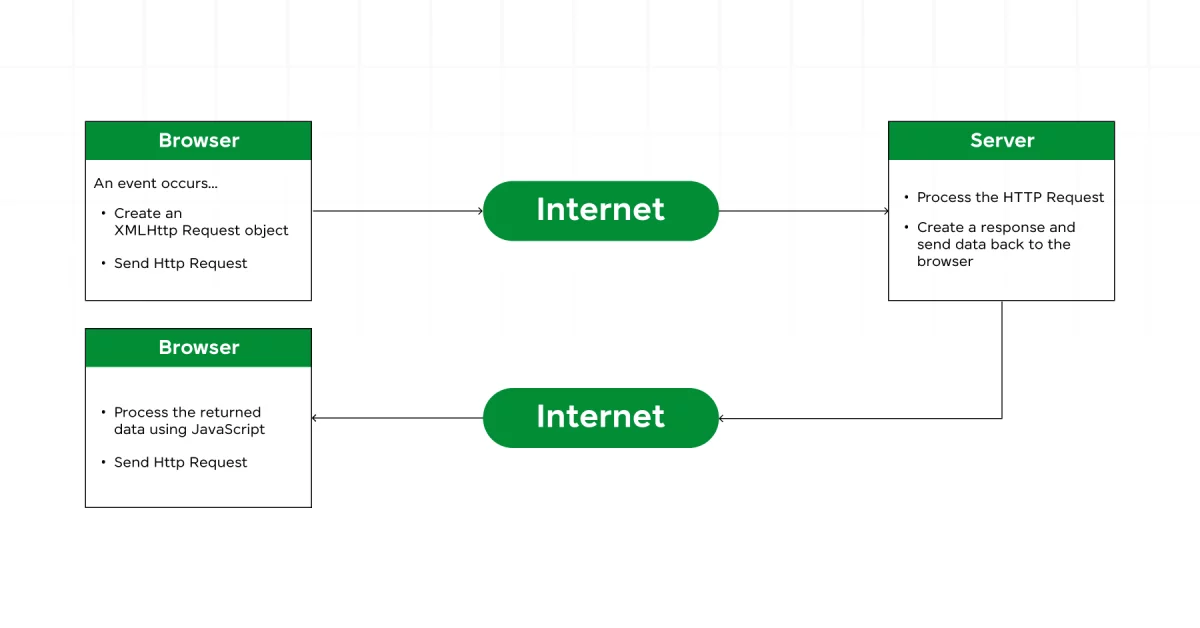
2. Basics of AJAX with jQuery
At its core, AJAX in jQuery revolves around the $.ajax() function, which provides a simple and consistent interface for making asynchronous HTTP requests. Here’s a basic example of how to use it:
$.ajax({
url: 'https://api.example.com/data',
method: 'GET',
success: function(response) {
// Handle the successful response
console.log(response);
},
error: function(xhr, status, error) {
// Handle the error
console.error(error);
}
});
In this example, we’re making a GET request to the specified URL (https://api.example.com/data). The success callback function is executed if the request is successful, allowing you to handle the response data. The error callback function is called if an error occurs during the request, giving you the opportunity to handle the error appropriately.
jQuery’s $.ajax() function accepts a configuration object with various options that control the behavior of the AJAX request. Some commonly used options include:
- URL: The URL to which the request is sent.
- method (or type): The HTTP request method (e.g., GET, POST, PUT, DELETE).
- data: The data to be sent with the request (for POST, PUT, and DELETE requests).
- headers: Additional headers to be included in the request.
- success: The callback function to be executed when the request is successful.
- error: The callback function to be executed when the request encounters an error.
Also Read: Real-world Web Development Applications
3. Handling Different Request Types
While the previous example demonstrated a GET request, jQuery’s $.ajax() function supports various HTTP request methods, allowing you to handle different types of operations. Here’s an example of how to make a POST request with jQuery:
$.ajax({
url: 'https://api.example.com/data',
method: 'POST',
data: { name: 'John Doe', email: 'john@example.com' },
success: function(response) {
// Handle the successful response
console.log(response);
},
error: function(xhr, status, error) {
// Handle the error
console.error(error);
}
});
In this case, we’re sending data (an object with name and email properties) along with the POST request. The data option can accept various data formats, including JSON, Form URL-encoded data, and more.
You can also perform other HTTP request methods like PUT and DELETE by simply specifying the appropriate method option in the configuration object.
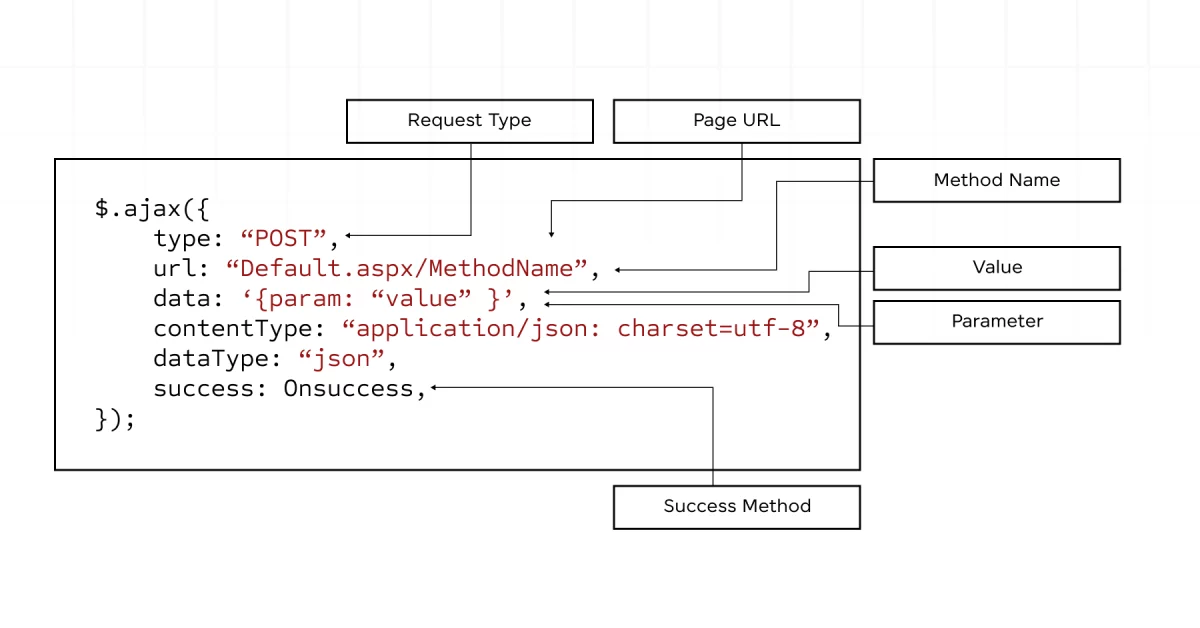
4. Working with JSON Data
Many modern web APIs return data in JSON (JavaScript Object Notation) format, which is lightweight and easily parsable by JavaScript. jQuery provides built-in support for handling JSON data in AJAX requests. Here’s an example of how to work with JSON data:
$.ajax({
url: 'https://api.example.com/users',
method: 'GET',
dataType: 'json',
success: function(users) {
// The response is automatically parsed as JSON
users.forEach(function(user) {
console.log(user.name);
});
},
error: function(xhr, status, error) {
// Handle the error
console.error(error);
}
});
In this example, we’re setting the dataType option to ‘json’, which instructs jQuery to automatically parse the response as JSON. This means that the success callback function will receive the parsed JSON data as an object or array, depending on the response format.
Also, Read Top ReactJS Interview Questions and Answers! [Part-2]
5. Handling Responses
jQuery’s $.ajax() function provides various ways to handle the response data, depending on your needs. In addition to the success and error callbacks, you can also use the done, fail, and always methods to handle the response.
$.ajax({
url: 'https://api.example.com/data',
method: 'GET'
})
.done(function(response) {
// Handle the successful response
console.log(response);
})
.fail(function(xhr, status, error) {
// Handle the error
console.error(error);
})
.always(function() {
// This code will always be executed, regardless of success or failure
console.log('Request completed');
});
The done method is similar to the success callback, while the fail method is analogous to the error callback. The always method is executed regardless of whether the request was successful or not, allowing you to perform cleanup tasks or update the UI accordingly.
6. Chaining AJAX Calls
One of the powerful features of jQuery’s AJAX implementation is the ability to chain AJAX calls. This means you can execute multiple AJAX requests sequentially, where the next request depends on the result of the previous one. Chaining can be particularly useful when working with APIs that require multiple requests to fetch or update data.
Here’s an example of how to chain AJAX calls in jQuery:
$.ajax({
url: 'https://api.example.com/users',
method: 'GET',
dataType: 'json'
})
.done(function(users) {
// Handle the list of users
console.log(users);
// Chain another AJAX request for a specific user
var userId = users[0].id;
return $.ajax({
url: `https://api.example.com/users/${userId}`,
method: 'GET',
dataType: 'json'
});
})
.done(function(user) {
// Handle the details of the specific user
console.log(user);
})
.fail(function(xhr, status, error) {
// Handle any errors that occurred in the chain
console.error(error);
});
In this example, we’re first making a GET request to fetch a list of users. In the done callback, we’re handling the list of users and then chaining another AJAX request to fetch the details of a specific user based on the user’s ID. The second AJAX request is executed only after the first one is successful, and its response is handled in the next callback.
Chaining AJAX calls can make your code more modular and easier to maintain, as each AJAX request is handled separately while still being part of a larger workflow.
7. Using jQuery’s Convenience Methods
While the $.ajax() function provides a comprehensive and flexible interface for making AJAX requests, jQuery also offers several convenient methods that simplify common AJAX operations. These methods are shorthand versions of the $.ajax() function and can make your code more concise and readable.
Some of the commonly used convenience methods include:
- $.get(): Performs a GET request.
- $.post(): Performs a POST request.
- $.getJSON(): Performs a GET request and automatically parses the response as JSON.
These convenience methods can make your code more readable and easier to understand, especially for simple AJAX operations. However, for more complex scenarios or when you need greater control over the request configuration, the $.ajax() function is the preferred choice.
Also Read: ReactJS Tutorial: Crafting a ToDo List Application in 5 Simple Steps (Code Included)
8. Error Handling and Debugging
While AJAX requests can greatly enhance the user experience of your web applications, they can also introduce new challenges when it comes to error handling and debugging. jQuery provides several tools and techniques to help you identify and resolve issues related to AJAX requests.
One of the primary ways to handle errors in AJAX requests is through the error callback function or the fail method. These allow you to capture and handle any errors that occur during the request, such as network errors, server errors, or validation errors.
$.ajax({
url: 'https://api.example.com/data',
method: 'GET'
})
.done(function(response) {
// Handle the successful response
console.log(response);
})
.fail(function(xhr, status, error) {
// Handle the error
console.error(error);
// You can also inspect the xhr object for more information
console.log('Status:', status);
console.log('Response:', xhr.responseText);
});
In the example above, we’re logging the error message, status code, and the response text from the server in the fail callback. This information can be invaluable for debugging and understanding the root cause of the error.
Another useful tool for debugging AJAX requests is the browser’s developer tools. Most modern browsers provide a Network panel or tab that allows you to inspect AJAX requests and responses in detail. You can view the request headers, response headers, and response data, which can help you identify issues related to data formatting, authentication, or server-side errors.
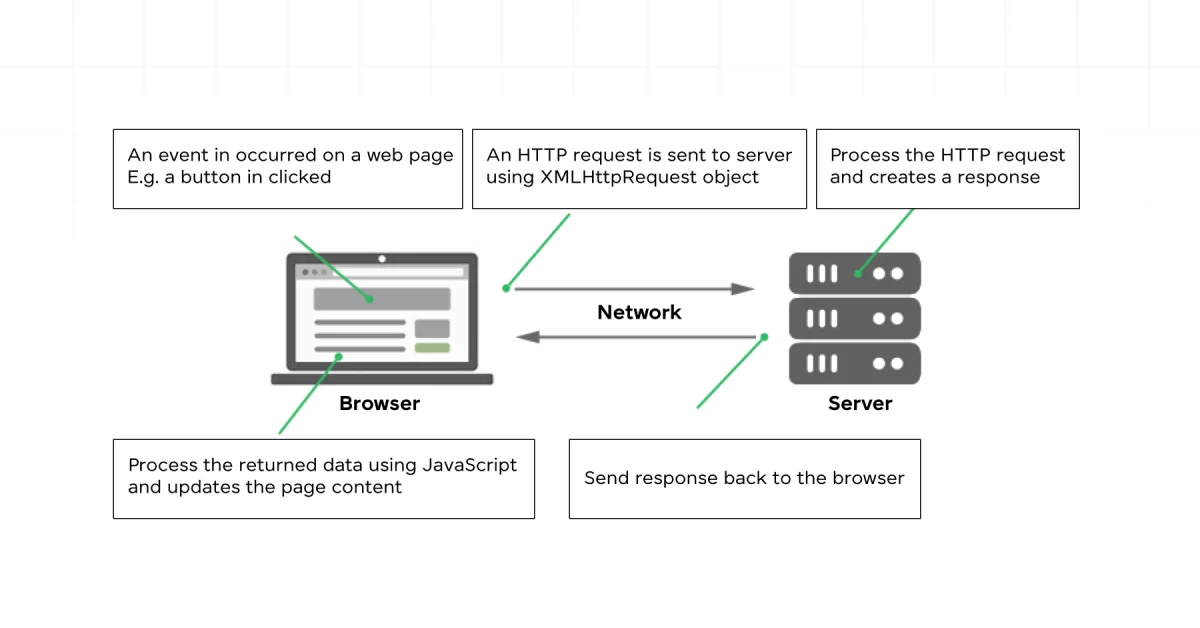
Also Explore: Best Tips and Tricks for JavaScript Debugging Skills
Additionally, jQuery provides the $.ajaxSetup() method, which allows you to configure global AJAX settings and options. This can be useful for setting up global error handling, setting default headers, or enabling cross-domain requests (if needed).
$.ajaxSetup({
error: function(xhr, status, error) {
// Global error handling
console.error('AJAX Error:', error);
}
});
In this example, we’re setting up a global error handler for all AJAX requests using $.ajaxSetup(). This can be particularly useful in larger applications where you want consistent error handling across different AJAX requests.
9. Security Considerations
When working with AJAX and jQuery, it’s important to consider security implications to protect your web applications from potential vulnerabilities. Here are some important security considerations to keep in mind:
- Cross-Site Scripting (XSS): XSS vulnerabilities can occur when user-provided data is not properly sanitized or encoded before being rendered in the browser. jQuery provides methods like $.fn.text() and $.fn.html() that can help prevent XSS by automatically encoding the data before inserting it into the DOM.
- Cross-Site Request Forgery (CSRF): CSRF attacks can occur when an authenticated user’s browser is tricked into performing unwanted actions on a trusted website. To mitigate CSRF risks, you should always include CSRF tokens in your AJAX requests and validate them on the server side.
- Same-Origin Policy: By default, AJAX requests are subject to the same-origin policy, which means they can only communicate with the same origin (domain, protocol, and port) as the web page that initiated the request. If you need to make cross-origin requests, you’ll need to enable CORS (Cross-Origin Resource Sharing) on the server or use techniques like JSONP (JSON with Padding) or a proxy server.
- Sensitive Data Handling: When working with sensitive data, such as authentication credentials or personal information, it’s essential to transmit and store the data securely. Always use HTTPS (SSL/TLS) to encrypt the communication between the client and server, and follow best practices for secure data storage and handling on the server side.
- Third-Party Libraries and Dependencies: When using third-party libraries or dependencies, ensure that you’re using the latest versions and keep them up-to-date. Outdated libraries can introduce security vulnerabilities, so it’s important to stay vigilant and monitor for updates and security advisories.
- Input Validation and Sanitization: Always validate and sanitize user input, both on the client side and server side, to prevent injection attacks, such as SQL injection or code injection.
By following these security best practices and staying up-to-date with the latest security advisories and recommendations, you can help protect your web applications from potential vulnerabilities and ensure a secure and reliable user experience.
Transform your future with expert training in full-stack development. Join GUVI’s Full Stack Development Course today and start building cutting-edge web applications! Enroll now!
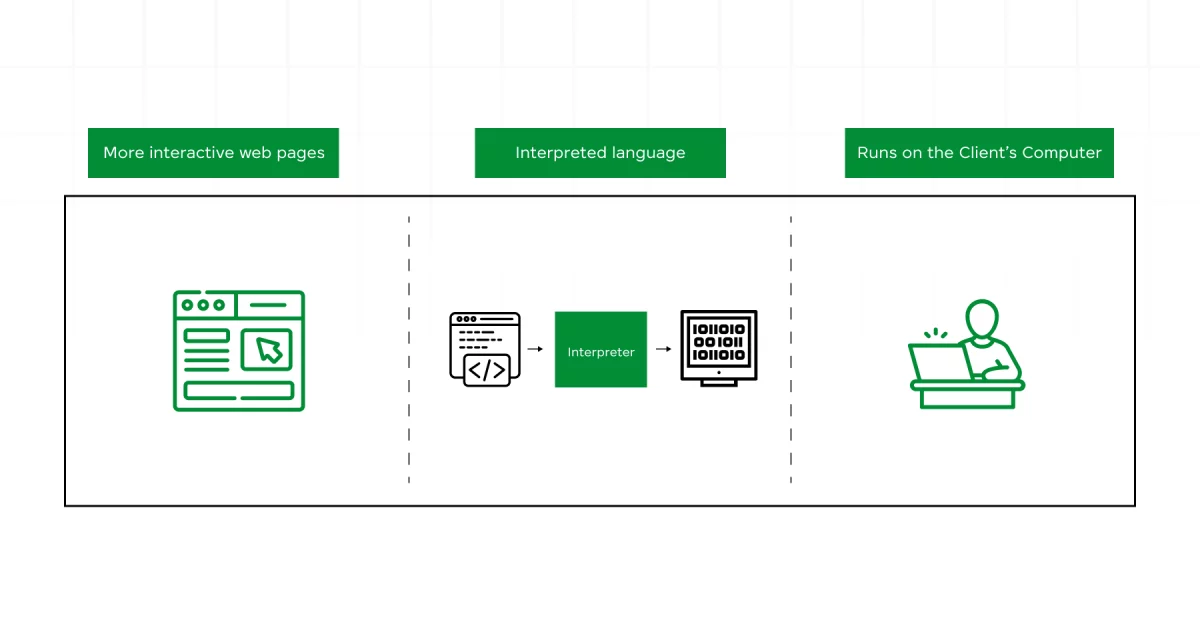
Also Read: Best Web Development Projects for All Techies
Conclusion
AJAX with jQuery is a powerful combination that enables developers to create dynamic and responsive web applications.
While jQuery has been a dominant force in the JavaScript ecosystem for many years, it’s important to note that the web development landscape is constantly evolving. With the rise of modern JavaScript frameworks and libraries like React, Angular, and Vue.js, the usage of jQuery has declined in recent years.
However, jQuery’s AJAX functionality remains relevant and valuable, particularly for older web applications or projects that rely on jQuery.
Mastering AJAX with jQuery can greatly enhance your web development skills and enable you to create rich and engaging user experiences. By using the power of jQuery’s AJAX capabilities, you can build responsive and efficient web applications that seamlessly integrate with server-side APIs and deliver a smooth and seamless user experience.
FAQs
AJAX (Asynchronous JavaScript and XML) enables web pages to update content dynamically by exchanging data with a server asynchronously, without reloading the entire page. jQuery simplifies AJAX by offering intuitive methods like $.ajax(), $.get(), $.post(), and $.getJSON().
Handling AJAX errors effectively ensures smooth user experiences in web applications. jQuery provides an error callback in its AJAX methods ($.ajax(), $.get(), $.post(), etc.) to manage error conditions. This callback captures details such as HTTP error codes, network issues, or server-side errors (xhr, status, error).
Security is critical when implementing AJAX to prevent vulnerabilities like Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF). Best practices include validating and sanitizing user inputs on the server side to prevent injection attacks. Ensure all AJAX requests are made over HTTPS to encrypt data transmission and protect against data interception.
Did you enjoy this article?