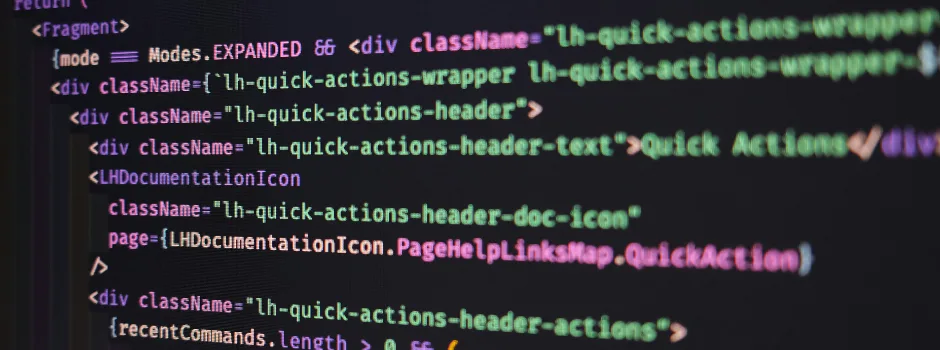
Asynchronous operations are essential in JavaScript, allowing developers to manage tasks like network requests and timers without blocking code execution. This capability is crucial for maintaining a smooth user experience in web applications. By utilizing callbacks, promises, and async/await syntax, developers can effectively handle asynchrony and enhance application performance.
Table of contents
- Asynchronous Operations in JavaScript
- Single Thread and Logical Processes
- How Asynchronous Operations Work
- Asynchronous Operations in Browsers
- Event Loop and Callbacks
- Asynchronous Operations in Node.js
- Event Loop and Thread Pool
- Conclusion
Asynchronous Operations in JavaScript
JavaScript is essentially a single-threaded language, meaning it runs programs sequentially, line by line. But thanks to an arrangement called the event loop, it can effectively manage asynchronous operations. Because of this, JavaScript can handle things like timers, network requests, and user interactions without interfering with the main thread of operation.
Single Thread and Logical Processes
- Single Logical Process: Single-threaded programming in JavaScript refers to the execution of all code within a single logical process. It avoids problems like race situations that can occur in multi-threaded settings because only one piece of code can be run at a time. The run-to-completion semantics enforce this, requiring each job (such as a function’s execution) to conclude before starting a new one.
- Event Loop Mechanism: Despite only having one thread, JavaScript can manage asynchronous tasks by using tools like the event loop. An asynchronous job (such as a network request) is started and then sent to either the thread pool of Node.js or the Web APIs provided by the browser. After execution, the outcomes are queued up for the main thread to execute without stalling it.
- Concurrency through Asynchronous Programming: JavaScript accomplishes concurrency by enabling asynchronous task execution rather than the use of multiple threads. This implies that other activities can continue running while one waits for an activity (like fetching data) to finish.
How Asynchronous Operations Work
JavaScript provides a number of constructs for managing asynchronous operations, including:
- Callbacks: Functions that are passed as arguments to other functions and executed after some operation completes.
- Promises: Objects that stand in for an asynchronous operation’s ultimate success (or failure) and the value that results from it. Using .then(), promises can be chained, adding callbacks to the microtask queue.
- Async/Await: Syntactic sugar on promises that enables synchronous code to be written in an asynchronous fashion. While permitting other operations to continue, the await keyword halts execution until the promise resolves.
Asynchronous Operations in Browsers
Event Loop and Callbacks
The Event Loop and Web APIs are the main means by which asynchronous operations are managed in a browser. The following is a summary of the procedure:
Call Stack: The call stack is a data structure that uses the last-in, first-out (LIFO) principle to track function calls. A function is placed onto the stack upon call, then it is removed from the stack upon return. This maintains track of the function that is running at the moment.
Callback Queue (Task Queue): When the call stack is cleared, messages (callbacks) prepared for execution are stored in the callback queue. This queue is used to hold the callback functions for any asynchronous operations (such as timers and network requests) that are executed.
Event Loop: The event loop keeps checking to see if there is nothing on the call stack. If so, it moves the callbacks in First-in, First-out (FIFO) manner into the call stack so that it can be executed after pulling it from the callback queue. This mechanism, despite only using one thread, enables JavaScript to carry out non-blocking actions.
Microtask Queue: Promises and their callbacks (i.e., .then() handlers) are stored in this queue. Due to its greater priority than the callback queue, the event loop will process all microtasks before proceeding with the callback queue tasks when the call stack is empty.
In case, you want to learn more about “asynchronous operations” and gain in-depth knowledge on full-stack development, consider enrolling for GUVI’s certified Full-stack Development Course that teaches you everything from scratch and make sure you master it!
Asynchronous Operations in Node.js
Event Loop and Thread Pool
An event loop is used by Node.js as well, however its architecture is very different
Call Stack: Node.js implements a Call Stack for synchronous code execution, just like browsers do.
Thread Pool: For I/O tasks that are too demanding for the Event Loop, Node.js has a Thread Pool that is run by libuv. This makes it possible for Node.js to execute non-blocking I/O operations efficiently.
Event Queue: An event queue holds the callback for an asynchronous operation, such as reading a file, once it has finished.
Event Loop: When the Call Stack is empty, the Event Loop pushes callbacks from the Event Queue in a First-in, First-out (FIFO) manner to the call stack for execution.
Feature | Browser | Node.js |
Environment | Runs in a web environment with Web APIs | Runs on server-side with libuv |
Thread Management | Single-threaded with Web APIs | Single-threaded with Thread Pool |
Event Handling | Uses Callback Queue and Event Loop | Uses Event Queue and Event Loop |
API Availability | Limited to browser-specific APIs | Includes file system access, networking, etc. |
Conclusion
In summary, mastering asynchronous operations is vital for building responsive JavaScript applications. By leveraging callbacks, promises, and async/await, developers can ensure efficient code execution and create seamless user experiences. As the demand for interactive web applications grows, these skills will remain indispensable in the JavaScript landscape.
Did you enjoy this article?