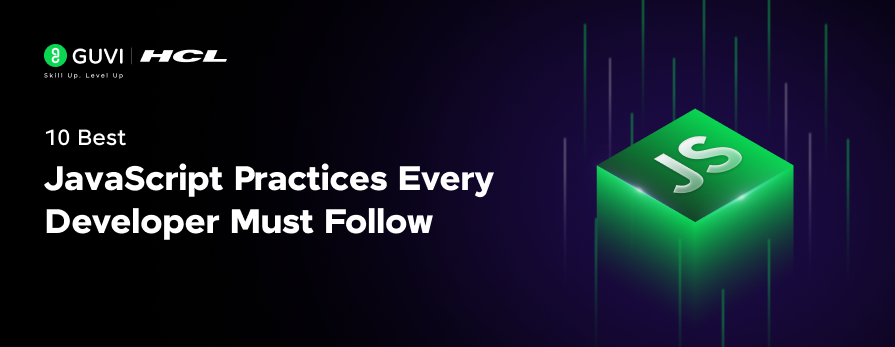
10 Best JavaScript Practices Every Developer Must Follow
Mar 03, 2025 5 Min Read 3814 Views
(Last Updated)
Are you writing JavaScript code that is clean, efficient, and easy to maintain? Or do you often find yourself debugging messy code, struggling with unexpected errors, or facing performance issues?
JavaScript is a powerful and widely used language, but writing high-quality JavaScript requires more than just knowing the syntax. To create robust applications, developers must follow best practices that improve readability, maintainability, and performance.
In this article, we’ll explore 10 essential JavaScript practices that every developer should follow to write better, cleaner, and more optimized code. So, without further ado, let us get started.
Table of contents
- 10 Essential JavaScript Practices
- Use Strict Mode
- Declare Variables Properly
- Keep Code Modular with Functions
- Avoid Polluting the Global Namespace
- Use Descriptive Naming Conventions
- Handle Errors Gracefully
- Optimize Performance
- Avoid Callback Hell by Using Promises and Async/Await
- Use Template Literals Instead of String Concatenation
- Use ES6+ Features to Write Cleaner Code
- Conclusion
- FAQs
- What is the purpose of using 'use strict' in JavaScript?
- Why should I prefer 'let' and 'const' over 'var' for variable declarations?
- How can I avoid callback hell in JavaScript?
- What are template literals, and when should I use them?
- Why is it important to minimize the use of global variables in JavaScript?
10 Essential JavaScript Practices
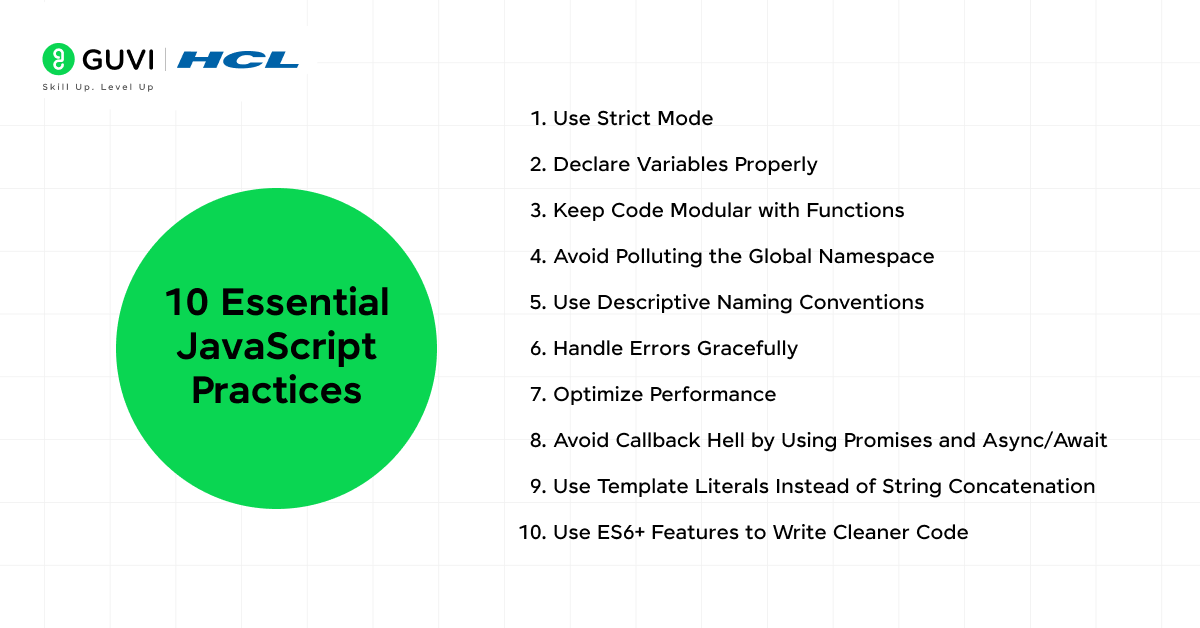
JavaScript is one of the most widely used programming languages in web development. Following best practices ensures better performance, reduces bugs, and makes collaboration easier.
If you’re already familiar with JavaScript basics, this guide will help you refine your coding style with best practices that every developer should follow.
1. Use Strict Mode
What is Strict Mode?
Strict mode in JavaScript is a way to enforce stricter rules and catch common errors that might otherwise go unnoticed. It helps in writing secure and optimized code by eliminating silent errors.
Why Use Strict Mode?
- Prevents the use of undeclared variables.
- Eliminates this coercion in functions (making it undefined instead of the global object).
- Restricts the use of reserved keywords for future versions of JavaScript.
How to Enable Strict Mode
To enable strict mode, simply include ‘use strict’; at the beginning of your script or within a function.
javascript
'use strict';
function example() {
let message = "Hello, World!";
console.log(message);
}
example();
Using strict mode helps avoid accidental mistakes and makes debugging easier.
Related blog: Tips and Tricks for JavaScript Debugging Skills
2. Declare Variables Properly
Why Is Variable Declaration Important?
JavaScript allows variable declaration using var, let, and const, but each behaves differently:
- var: Function-scoped, which can lead to accidental overwrites due to hoisting.
- let: Block-scoped, preventing unintended overwriting.
- const: Block-scoped, but its value cannot be reassigned after initialization.
Best Practice: Use let and const Instead of var
javascript
const MAX_USERS = 100; // Constant value that won’t change
let userCount = 5; // Can be reassigned
// Avoid this:
var total = 10; // Function-scoped and can lead to unexpected results
Key Benefits:
- Prevents accidental global variable creation.
- Ensures predictable scoping.
- Improves readability and maintainability.
3. Keep Code Modular with Functions
Why Should You Write Modular Code?
Instead of writing large chunks of code in one function or file, it’s best to break them down into smaller, reusable functions. This makes your code more readable, easier to debug, and maintainable.
Example of Modular Code
javascript
// A function to calculate area
function calculateArea(width, height) {
return width * height;
}
// Another function to display the result
function displayArea(width, height) {
console.log(`The area is ${calculateArea(width, height)}`);
}
displayArea(5, 10);
Advantages of Modular Functions:
- Reusability: Functions can be used multiple times in different parts of the application.
- Better Debugging: Issues are easier to track when functions have a single responsibility.
- Improved Collaboration: Code is easier for teams to understand and modify.
4. Avoid Polluting the Global Namespace
What Is the Global Namespace?
In JavaScript, variables and functions declared without var, let, or const are automatically added to the global namespace (or global scope). This can lead to conflicts and unpredictable behavior, especially in large applications.
Best Practices to Avoid Global Pollution
1. Use Function Encapsulation
Wrap your code inside functions to create local scopes.
javascript
function myModule() {
let privateVar = "I am private";
console.log(privateVar);
}
myModule();
2. Use Immediately Invoked Function Expressions (IIFE)
This pattern prevents variables from leaking into the global scope.
javascript
(function() {
let privateData = "Encapsulated Data";
console.log(privateData);
})();
3. Use Modules (ES6 Imports/Exports)
Modular programming using ES6 modules ensures better code organization.
javascript
// In file user.js
export function getUser() {
return { name: "John Doe" };
}
// In another file
import { getUser } from "./user.js";
console.log(getUser());
Benefits:
- Prevents Variable Overwriting: Reduces risk of name conflicts.
- Improves Code Organization: Helps separate different functionalities.
- Enhances Security: Keeps sensitive data out of the global scope.
5. Use Descriptive Naming Conventions
Why Are Naming Conventions Important?
Poorly named variables and functions make code difficult to understand. Proper naming enhances readability, maintainability, and debugging.
Best Practices for Naming Variables & Functions:
1. Use meaningful names
Instead of:
javascript
let x = 10;
Use:
javascript
let itemCount = 10;
2. Follow CamelCase for Variables and Functions
javascript
let userName = "JohnDoe"; // Good
function getUserDetails() { ... } // Good
3. Use Uppercase for Constants
javascript
const MAX_USERS = 100;
4. Prefix Boolean Variables with is, has, or can
javascript
let isUserLoggedIn = true;
let hasAdminPrivileges = false;
Benefits:
- Makes code self-explanatory.
- Helps avoid confusion when working with multiple developers.
- Improves long-term maintainability.
6. Handle Errors Gracefully
Why Is Error Handling Important?
Ignoring errors can lead to application crashes and bad user experiences. Instead of letting errors break the application, handle them gracefully.
Best Practices:
1. Use Try-Catch Blocks
javascript
try {
let result = riskyOperation();
console.log(result);
} catch (error) {
console.error("An error occurred:", error.message);
}
2. Use Default Values to Prevent Errors
javascript
function getUserName(user) {
return user?.name || "Guest";
}
console.log(getUserName(null)); // Outputs: Guest
3. Validate Inputs Before Processing
javascript
function divide(a, b) {
if (b === 0) {
throw new Error("Cannot divide by zero.");
}
return a / b;
}
try {
console.log(divide(10, 2)); // Works fine
console.log(divide(10, 0)); // Throws an error
} catch (error) {
console.error(error.message);
}
Benefits:
- Prevents application crashes.
- Improves debugging.
- Enhances user experience by providing meaningful error messages.
7. Optimize Performance
Why Should You Optimize JavaScript Code?
Efficient code execution ensures fast load times and smooth user interactions. Poorly optimized JavaScript can slow down applications, leading to a bad user experience.
Performance Optimization Techniques:
1. Minimize DOM Manipulations
javascript
// Bad (Causes multiple reflows)
document.getElementById("title").innerHTML = "Hello";
document.getElementById("title").style.color = "red";
// Better (Batch updates)
let title = document.getElementById("title");
title.innerHTML = "Hello";
title.style.color = "red";
2. Use Debouncing for Event Listeners
javascript
function debounce(func, delay) {
let timeout;
return function(...args) {
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(this, args), delay);
};
}
window.addEventListener("resize", debounce(() => {
console.log("Window resized!");
}, 300));
Benefits:
- Enhances user experience with smooth performance.
- Reduces CPU and memory usage.
- Prevents unnecessary function executions.
8. Avoid Callback Hell by Using Promises and Async/Await
What Is Callback Hell?
Callback hell occurs when multiple nested callbacks make the code difficult to read and maintain. This often happens in asynchronous operations like API calls, database queries, or file system operations.
Example of Callback Hell:
javascript
getUser(1, function(user) {
getOrders(user.id, function(orders) {
processOrders(orders, function(result) {
console.log(result);
});
});
});
The nested structure makes it hard to debug and understand.
Solution: Use Promises and Async/Await
Using Promises
Promises provide a cleaner way to handle asynchronous operations.
javascript
getUser(1)
.then(user => getOrders(user.id))
.then(orders => processOrders(orders))
.then(result => console.log(result))
.catch(error => console.error(error));
Using Async/Await
Async/Await makes asynchronous code look synchronous, improving readability.
javascript
async function fetchData() {
try {
const user = await getUser(1);
const orders = await getOrders(user.id);
const result = await processOrders(orders);
console.log(result);
} catch (error) {
console.error(error);
}
}
fetchData();
Benefits:
- Improves code readability
- Simplifies error handling
- Makes debugging easier
9. Use Template Literals Instead of String Concatenation
What Are Template Literals?
Template literals (introduced in ES6) allow embedding variables and expressions inside strings using backticks (`) instead of the traditional string concatenation (+ operator).
Why Should You Use Template Literals?
- Better Readability
- Easier String Formatting
- Supports Multi-Line Strings Without \n
Best Practice: Using Template Literals
javascript
const name = "John";
const age = 25;
console.log(`My name is ${name} and I am ${age} years old.`);
Benefits:
- Improves readability
- Reduces unnecessary concatenation
- Simplifies handling of multi-line strings
10. Use ES6+ Features to Write Cleaner Code
Why Use Modern JavaScript (ES6+)?
JavaScript has evolved significantly over the years. The introduction of ES6 (ECMAScript 2015) and later versions brought several powerful features that improved code quality.
Best ES6+ Features Every Developer Should Use
1. Default Parameters
Instead of checking for undefined values, use default function parameters.
javascript
function greet(name = "Guest") {
console.log(`Hello, ${name}!`);
}
greet(); // Output: Hello, Guest!
greet("Alice"); // Output: Hello, Alice!
2. Arrow Functions
Arrow functions provide a concise way to write functions and automatically bind this.
javascript
const add = (a, b) => a + b;
console.log(add(5, 3)); // Output: 8
Benefits:
- Improves code readability and maintainability
- Reduces boilerplate code
- Enhances performance with modern syntax
In case you want to learn more about JavaScript and how it impacts Full Stack Development, consider enrolling in GUVI’s Full Stack Developer online course that teaches you everything from scratch and equips you with all the necessary knowledge!e
Conclusion
Writing clean, efficient, and maintainable JavaScript is not just about knowing the syntax—it’s about following best practices that enhance readability, reduce bugs, and optimize performance.
By following these above best practices, you’ll not only write better JavaScript but also improve the maintainability, performance, and scalability of your applications.
FAQs
Enabling strict mode with ‘use strict’; enforces stricter parsing and error handling in your JavaScript code, helping to catch common coding mistakes and prevent the use of undeclared variables.
let and const provide block-level scoping, reducing the risk of variable hoisting issues and accidental global variable creation, leading to more predictable and maintainable code.
To prevent callback hell, use Promises and the async/await syntax, which allow for writing cleaner and more readable asynchronous code by avoiding deeply nested callbacks.
Template literals, introduced in ES6, are string literals enclosed by backticks (`) that allow embedded expressions and multi-line strings, making string interpolation and formatting more convenient and readable.
Reducing global variables helps prevent naming collisions and potential overwriting by other scripts, leading to more modular and maintainable code.
Did you enjoy this article?