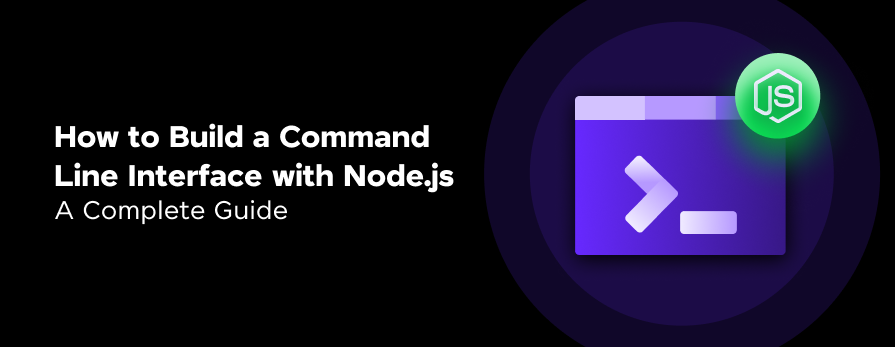
How to Build a Command Line Interface with Node.js: A Complete Guide
Dec 31, 2024 3 Min Read 3287 Views
(Last Updated)
In software development, efficiency and productivity are important. Command Line Interfaces (CLI) have long been the trusted tool for developers to streamline tasks, and automate processes. In Node.js, this potent capability reaches new heights, empowering developers to build robust, customizable, and user-friendly CLI applications with ease.
In this blog, we will learn how to build a Command Line Interface with Node.js. We’ll explore CLI development, from setting up your environment to designing intuitive user experiences, and equip you with the tools and knowledge needed to create CLI applications that elevate your development workflow. Let’s get started!
Table of contents
- What is CLI?
- How to Build a Command Line Interface with Node.js
- Project Setup
- Initialize npm Project
- Install Packages
- Create the Main Script (index.js)
- Write the Code (index.js)
- Run the Application
- Get ready to see the output!
- Output
- Conclusion
- FAQs
- What are the advantages of building a Command Line Interface (CLI) with Node.js?
- How can I handle user input and arguments effectively in a Node.js CLI application?
- What are some best practices for testing and publishing a Node.js CLI application?
What is CLI?
CLI stands for Command Line Interface. It is a text-based interface used to interact with a computer’s operating system or software applications by entering commands as text input. The CLI provides a way to execute tasks, navigate directories, manage files and directories, and run programs or scripts.
In contrast to a Graphical User Interface (GUI), which uses visual elements like windows, icons, and menus, the CLI requires the user to type in specific commands and follow a structured syntax. This makes it more efficient and powerful for advanced users, system administrators, and developers who need to perform complex or repetitive tasks.
Examples of CLIs include:
- Terminal or Console on Unix-based systems (Linux, macOS)
- Command Prompt (cmd.exe) or PowerShell on Windows
- Bash (Bourne Again SHell) or other shell environments
- Python or Ruby interactive shells for programming languages
CLIs are often used for tasks such as system administration, scripting, automation, file management, version control, and software development. While they may have a steeper learning curve compared to GUIs, CLIs offer more flexibility, control, and efficiency for advanced users.
Curious about web development but unsure where to begin? GUVI’s Full Stack Development course offers a risk-free way to explore the exciting possibilities of full-stack development. Explore the curriculum, and the interactive learning format, and experience the supportive learning environment. Enroll now!
Having understood the fundamentals of Command Line Interface (CLI), let’s learn – how to build a CLI with Node.js.
How to Build a Command Line Interface with Node.js
Ever felt intimidated by command-line interfaces (CLIs)? Don’t worry! Building a simple CLI tool with Node.js is easier than you think. Thanks to a wealth of open-source packages, you can add features like color, animation, and user input with ease.
In this tutorial, we’ll guide you through creating a basic CLI tool using Node.js. Let’s begin!
Steps to Create a Node.js CLI
1. Project Setup
- Create a new folder for your project.
- Open your preferred IDE (like Visual Studio Code) within that folder.
Also Read: 10 Best Node.js Libraries and Packages
2. Initialize npm Project
Open a terminal inside your project directory and run npm init -y. This quickly creates an empty npm project without manual configuration.
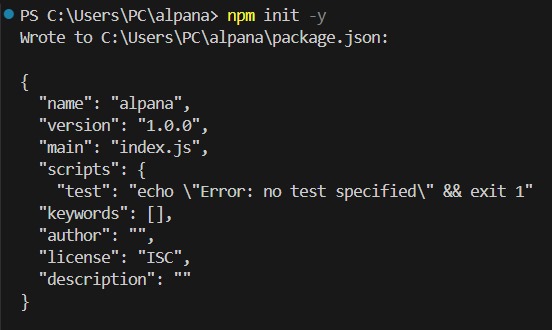
Also Read: How to Install Nodejs on Windows?: A Step-by-Step Guide
3. Install Packages
We’ll use some helpful open-source packages:
- figlet: Generates ASCII art text for a creative welcome message.
- inquirer: Simplifies user input prompts in the terminal.
- gradients-string: Adds colorful flair to your output.
You can learn more about each package by visiting their official documentation.
Let’s install some open-sourced npm packages required for this project:
npm install figlet
npm install inquirer
npm install gradient-string
Also Read: Node.JS as Backend: Everything You Need to Know About this Popular Framework
4. Create the Main Script (index.js)
Create a file named index.js inside your project folder.
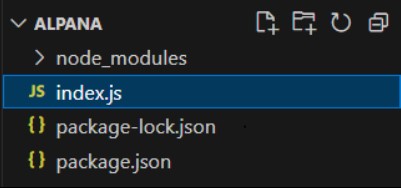
Also Read: NodeJS or Python: Which Backend Technology is Best Suited?
5. Write the Code (index.js)
We’ll create a single function called greet to welcome the user.
Inside greet:
- Use figlet to display a cool ASCII art “Meghana CLI” message.
- Utilize inquirer to prompt the user for their name and store it in a variable.
- Employ gradients-string to create a colorful greeting message personalized with the user’s name.
Now, let’s write the following code inside the index.js file:
import figlet from "figlet";
import inquirer from "inquirer";
import gradient from "gradient-string";
// Declare a variable to store the user's name
let userName;
const greet = async () => {
// Displaying Meghana CLI
figlet('Meghana CLI', function (err, data) {
console.log(data)
});
// Wait for 2secs
await new Promise(resolve => setTimeout(resolve, 2000));
// Ask the user's name
const { name } = await inquirer.prompt({
type: "input",
name: "name",
message: "Enter your name?"
});
// Set the user's name
userName = name;
// Print the welcome message
const msg = `Hello ${userName}!`;
figlet(msg, (err, data) => {
console.log(gradient.pastel.multiline(data));
});
}
// Call the askName function
greet();
Also Read: Top Technologies to Learn for a JavaScript Backend Developer

6. Run the Application
In your terminal, type node index.js to execute the script.
Get ready to see the output!
Output
Here’s the final output:
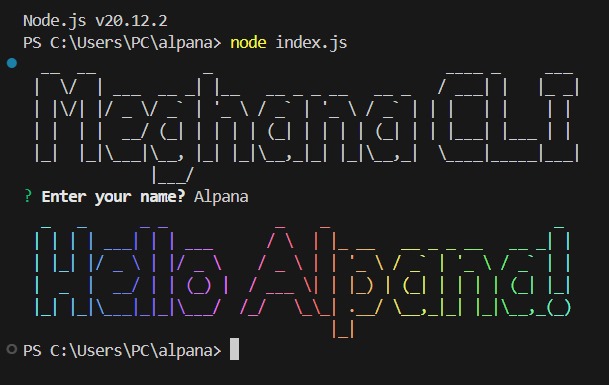
Enroll now and gain the in-demand skills to design, develop, and deploy full-stack web applications. GUVI’s Full Stack Development course provides a comprehensive curriculum, expert instruction, and a supportive learning community to empower you to become a web developer. Don’t miss this opportunity to upskill and transform your career – enroll today!
Conclusion
Command Line Interfaces remain a fundamental tool for developers, sysadmins, and power users alike. Node.js empowers developers to create CLI applications that are fast, efficient, and feature-rich. CLI development with Node.js bridges the gap between traditional terminal-based workflows and modern development practices, enabling seamless integration with web technologies and APIs.
Mastering CLI development with Node.js equips developers with the skills needed to thrive in a fast-paced, command-driven world.
Also Read: Salary of a NodeJs Developer in Famous 10 Companies in India
FAQs
What are the advantages of building a Command Line Interface (CLI) with Node.js?
Building a Command Line Interface (CLI) with Node.js brings several advantages. Firstly, Node.js’s asynchronous nature enables efficient handling of I/O operations, making it well-suited for CLI development where responsiveness is important.
Additionally, Node.js boasts a vast ecosystem of npm packages, offering a rich selection of libraries for tasks such as argument parsing, input/output handling, and color formatting.
How can I handle user input and arguments effectively in a Node.js CLI application?
Effectively handling user input and arguments is essential for a seamless CLI experience. In Node.js, libraries like yargs provide an intuitive solution for parsing command-line arguments and options.
By defining commands and options using yargs’ fluent API, developers can easily access parsed arguments in their application logic.
What are some best practices for testing and publishing a Node.js CLI application?
To ensure reliability and maintainability, developers should write comprehensive unit tests for individual commands and functionality using popular testing frameworks like Jest or Mocha. Automating testing procedures helps in validating consistent behavior across different environments and edge cases.
Did you enjoy this article?