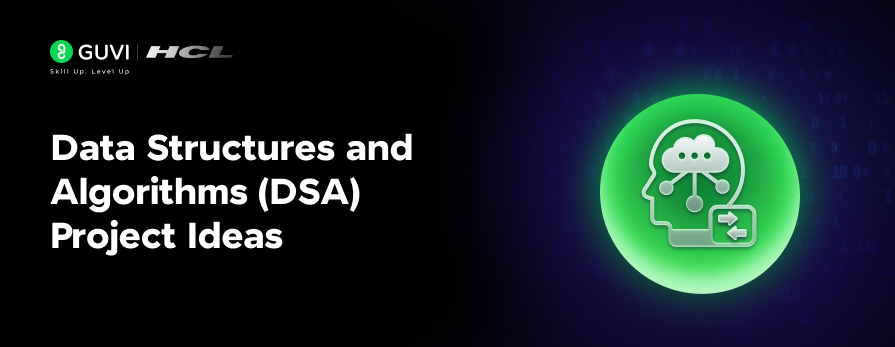
10 Important Data Structures and Algorithms Project Ideas
Dec 11, 2024 5 Min Read 12355 Views
(Last Updated)
Practicing data structures and algorithms (DSA) could push your coding skills to the next level and choosing the right project can be a game-changer, helping you apply theoretical concepts to real-world scenarios while building a solid foundation.
Whether you’re a beginner or more advanced, finding unique data structures and algorithms project ideas can be the perfect way to master DSA.
In this guide, we’ll cover top data structures and algorithms project ideas designed to sharpen your DSA knowledge. You’ll explore various DSA implementations, from beginner-friendly projects to more challenging ones.
Table of contents
- Top 10 Data Structure and Algorithms Project Ideas
- Social Media Friend Recommendation System
- Real-Time Stock Price Analysis
- Library Management System
- Movie Recommendation System Using Collaborative Filtering
- URL Shortener Service
- E-commerce Inventory Management System
- Data Compression Using Huffman Encoding
- Predictive Text Input Using Trie
- Job Scheduling Algorithm
- Chatbot with Real-Time Response Analysis
- Conclusion
- FAQs
- What are the easy Data Structure and Algorithms project ideas for beginners?
- Why are Data Structure and Algorithms projects important for beginners?
- What skills can beginners learn from Data Structure and Algorithms projects?
- Which Data Structure and Algorithms project is recommended for someone with no prior programming experience?
- How long does it typically take to complete a beginner-level Data Structure and Algorithms project?
Top 10 Data Structure and Algorithms Project Ideas
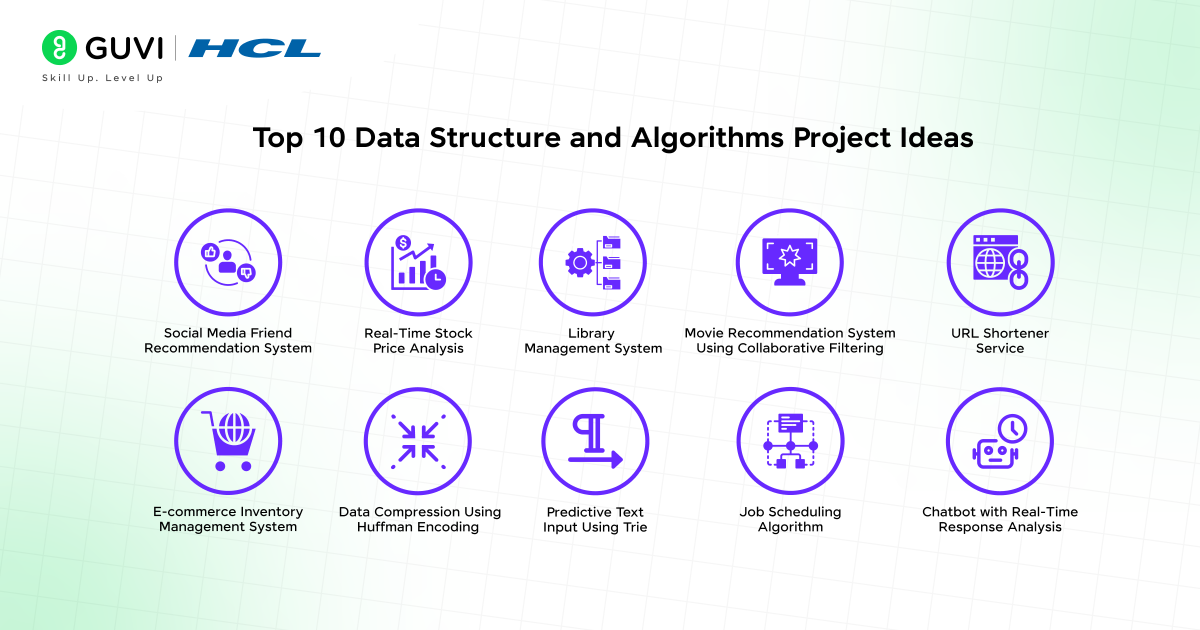
To make sure you don’t get stranded along the way, we’ve curated data structures and algorithms project ideas with varying complexities, learning outcomes, and programming languages to suit your skill level.
1. Social Media Friend Recommendation System
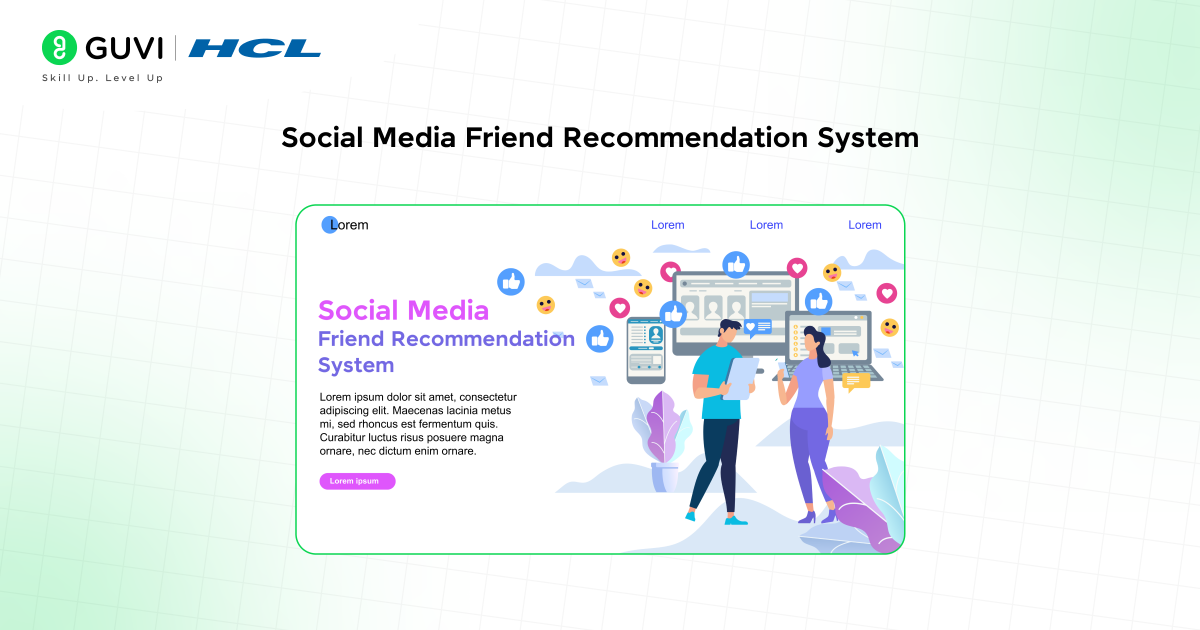
Social media platforms thrive on connections, and a friend recommendation system adds a personal touch by suggesting new people to connect with. This project involves implementing graph structures and algorithms that help find the shortest path between users and identify mutual connections and similar interests.
Time Taken: 1–2 weeks
Project Complexity: Intermediate
Learning Outcomes: This project will deepen your understanding of graph data structures, especially in terms of traversing nodes, calculating shortest paths, and analyzing relationship networks.
Features of the Project:
- Uses graph traversal and pathfinding algorithms
- Analyzes connections to provide friend recommendations
- Can be extended to include user preferences and interests
Programming Languages Used: Python, Java
Real-world Applications: This system replicates the recommendation engine behind platforms like Facebook and LinkedIn, where friend suggestions help users expand their network.
Source Code: Social Media Friend Recommendation System
2. Real-Time Stock Price Analysis
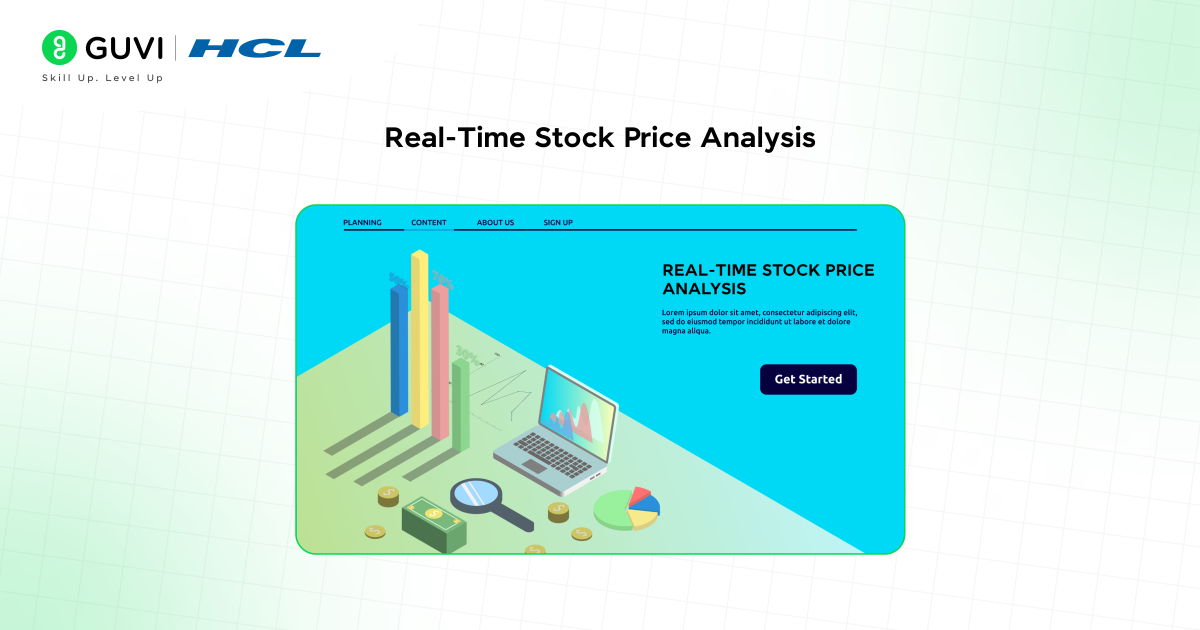
This project is designed to analyze real-time stock prices by processing incoming data, updating prices, and providing users with immediate feedback. Using heaps and sorting algorithms, you’ll create an application that efficiently tracks and displays live stock data, helping users monitor stock price trends.
Time Taken: 2–3 weeks
Project Complexity: Advanced
Learning Outcomes: This project will teach you how to work with heaps and sorting algorithms for real-time data handling. Additionally, you’ll gain experience managing large datasets and learn techniques for effective data filtering and sorting.
Features of the Project:
- Real-time stock price updates and analysis
- Sorting and filtering for trend analysis
- Option to set price alerts for significant changes
Programming Languages Used: Python, JavaScript
Real-world Applications: The project is ideal for applications in stock market analysis, e-commerce price tracking, and any industry that relies on real-time data monitoring.
Source Code: Real-Time Stock Price Analysis
3. Library Management System
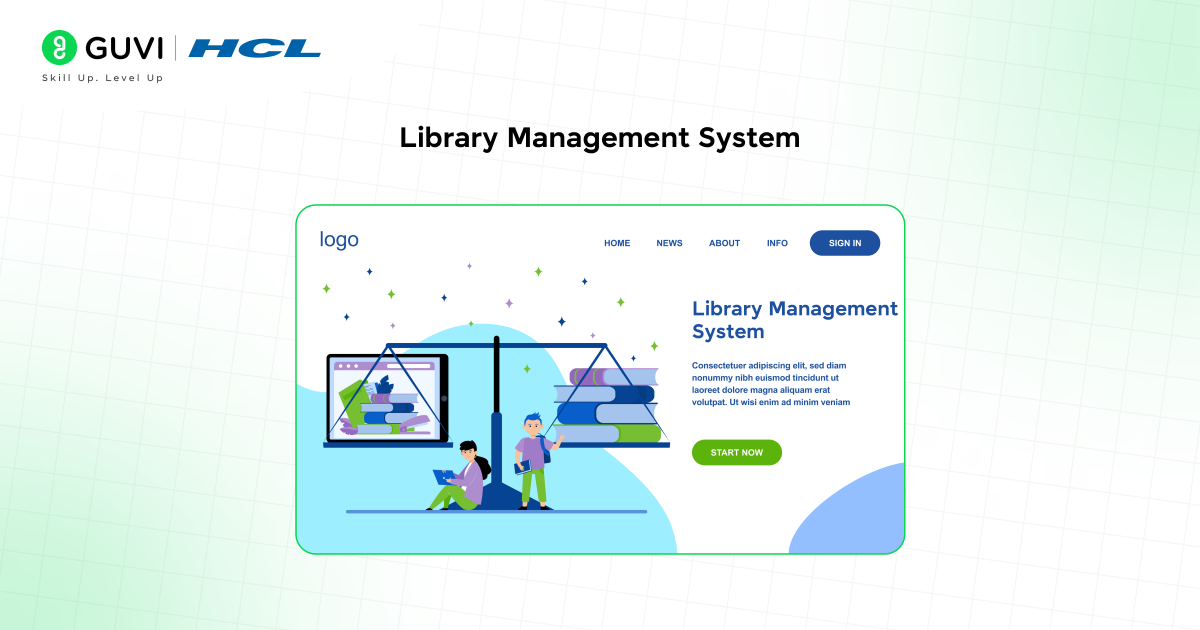
Libraries depend on systematic organization and an efficient way to track books and users. This project involves designing a library management system that handles book borrow and return actions using stacks and queues, making it a simple yet practical project for DSA practice.
Time Taken: 1 week
Project Complexity: Beginner
Learning Outcomes: You’ll gain foundational knowledge of stacks and queues, as well as their practical applications in organizing and managing data.
Features of the Project:
- Tracks borrowed and available books
- Manages book borrow and return transactions
- Supports user and inventory management
Programming Languages Used: Java, C++
Real-world Applications: This project can be applied to small libraries, bookstores, or any scenario that requires organized inventory management.
Source Code: Library Management System
4. Movie Recommendation System Using Collaborative Filtering
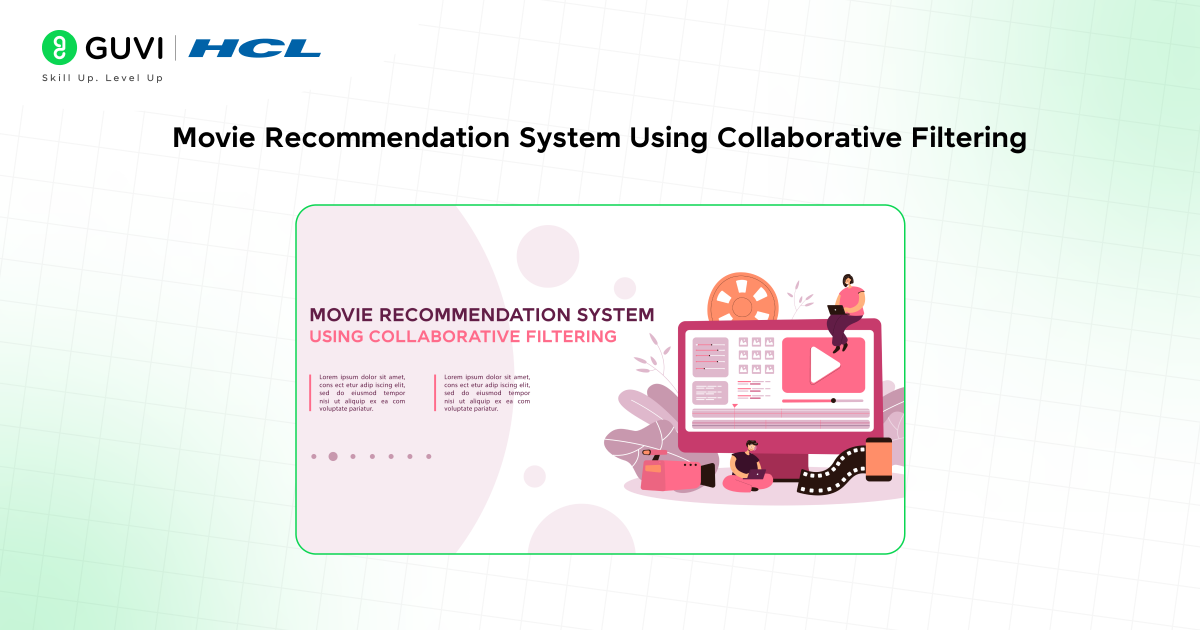
Recommendation systems are everywhere, from Netflix to e-commerce sites. This project uses collaborative filtering and DSA principles like hash maps and arrays to develop a system that recommends movies based on user preferences and past behaviors.
Time Taken: 1–2 weeks
Project Complexity: Intermediate
Learning Outcomes: This project enhances your understanding of collaborative filtering, arrays, and hash maps while teaching you how to implement a basic recommendation engine.
Features of the Project:
- Recommends movies based on user interests
- Incorporates collaborative filtering techniques
- Scalable to include more recommendation parameters
Programming Languages Used: Python
Real-world Applications: Similar to recommendation engines used by platforms like Netflix and Amazon, this system personalized content recommendations, enhancing user engagement.
Source Code: Movie Recommendation System
5. URL Shortener Service
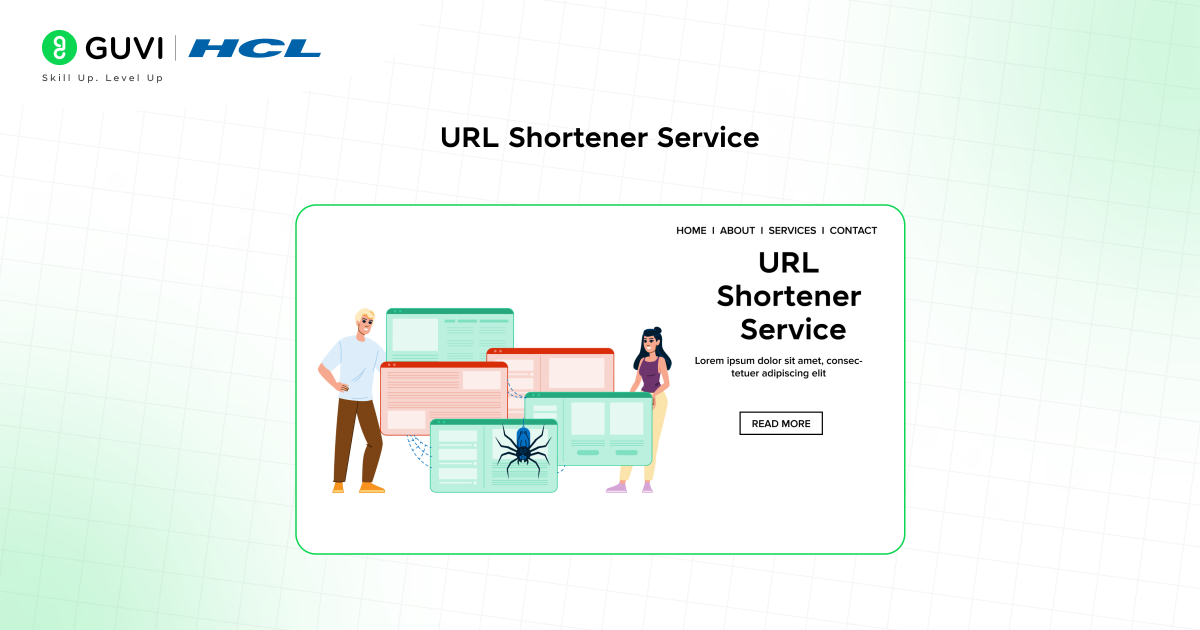
With the popularity of platforms like Twitter, URL shorteners have become essential. This project will help you build a URL shortener service, which takes long URLs and converts them into shorter, easily shareable links using hash functions and stack operations.
Time Taken: 1–2 weeks
Project Complexity: Intermediate
Learning Outcomes: You’ll learn how to use hash functions, manage URL data with stacks, and understand how to handle data storage for quick retrieval.
Features of the Project:
- Converts long URLs to short, unique identifiers
- Supports URL tracking and expiration
- Provides analytics on URL usage
Programming Languages Used: JavaScript, Python
Real-world Applications: This is commonly used in social media platforms and marketing campaigns, where it’s essential to have easily shareable and trackable links.
Source Code: URL Shortener Service
6. E-commerce Inventory Management System
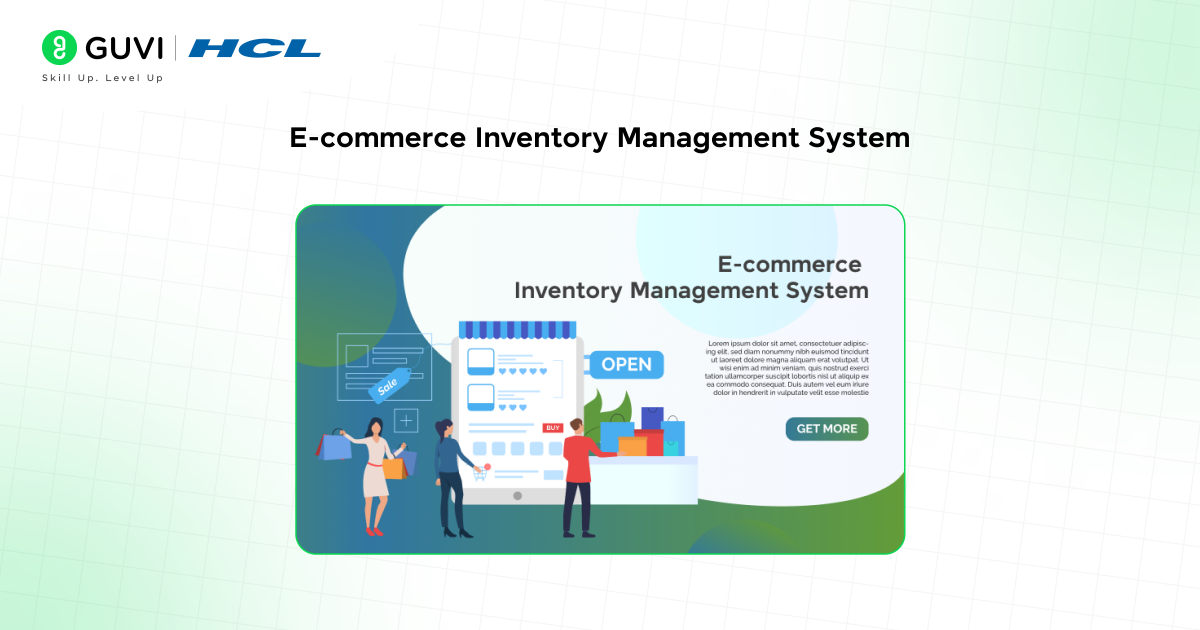
Managing inventory is a crucial part of any e-commerce platform, and this project helps you build a system that organizes, tracks and updates inventory levels efficiently.
By implementing binary search trees and hashing, you’ll create a system that manages products and stock levels, making it easy to search and update inventory.
Time Taken: 2–3 weeks
Project Complexity: Advanced
Learning Outcomes: This project will strengthen your understanding of binary search trees, hashing, and efficient data storage. You’ll learn how to manage large datasets and perform quick lookups to enhance inventory management.
Features of the Project:
- Keeps track of product quantities and categories
- Efficiently searches for items and updates stock levels
- Can be extended with additional features like reorder alerts
Programming Languages Used: C++, Python
Real-world Applications: Ideal for e-commerce platforms, retail, and warehouse management where inventory must be kept updated in real-time.
Source Code: E-commerce Inventory Management System
7. Data Compression Using Huffman Encoding
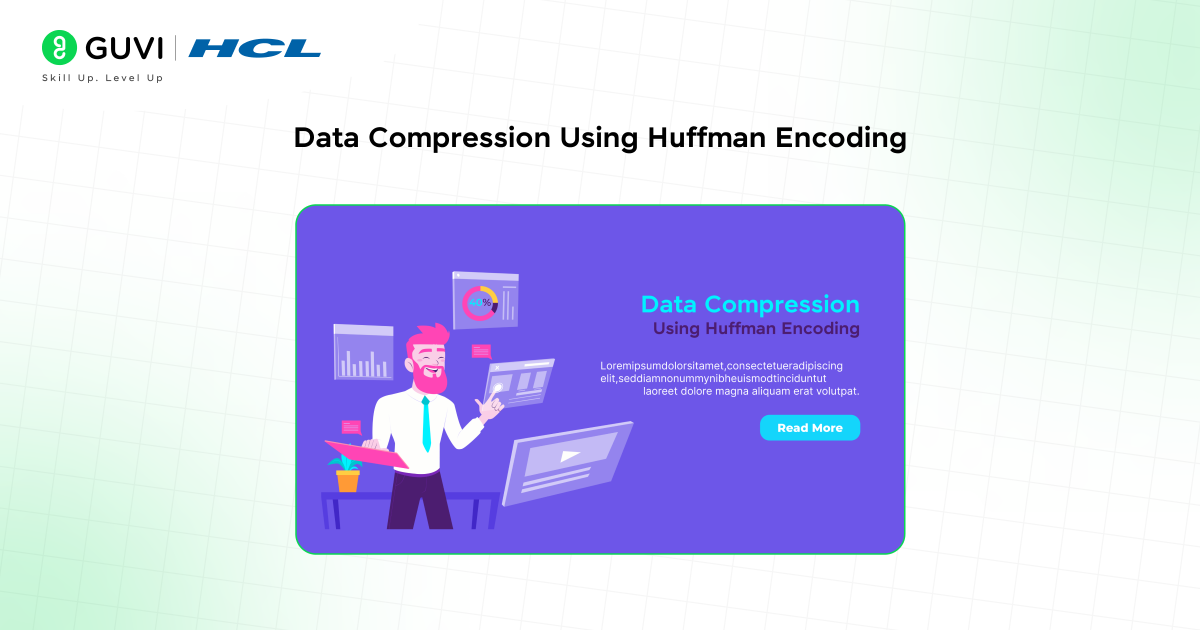
Data compression is essential for saving storage and improving transfer times. This project involves implementing Huffman encoding, a tree-based compression algorithm that reduces data size.
You’ll develop a tool that compresses and decompresses text files, making them smaller and easier to store or transfer.
Time Taken: 1–2 weeks
Project Complexity: Advanced
Learning Outcomes: Through this project, you’ll gain an understanding of tree structures, encoding algorithms, and the principles behind data compression.
Features of the Project:
- Compresses text files using Huffman encoding
- Supports file decompression to the original size
- Optimizes storage usage and transfer speed
Programming Languages Used: C++, Python
Real-world Applications: Widely used in file compression (e.g., ZIP files) and image compression for reduced storage requirements.
Source Code: Data Compression Using Huffman Encoding
8. Predictive Text Input Using Trie
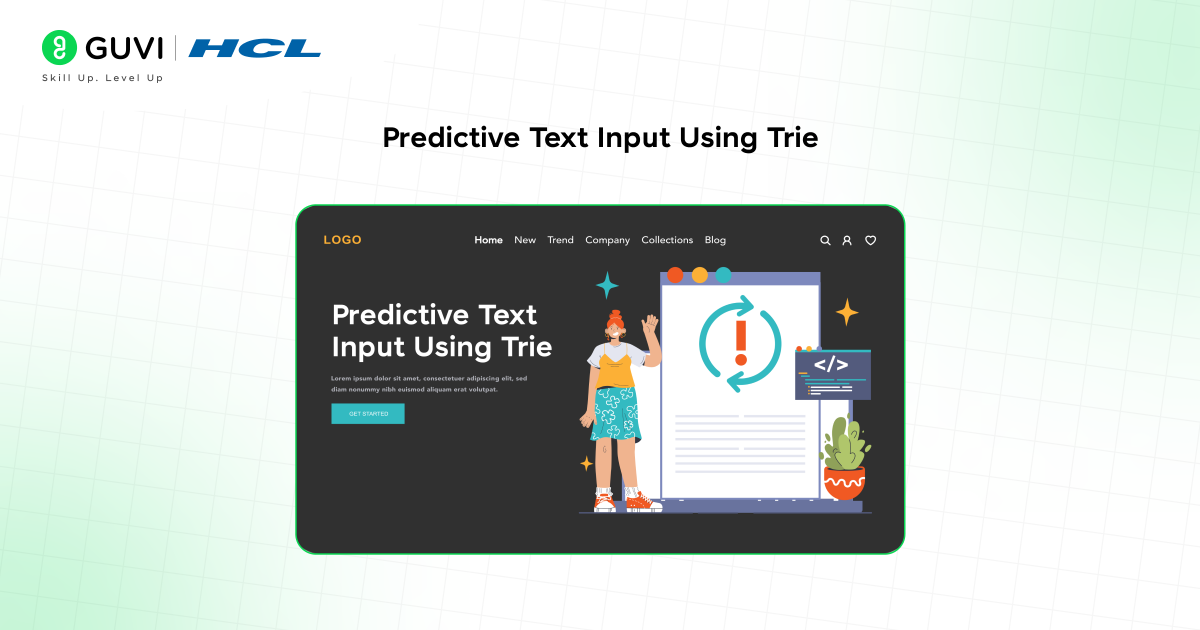
Predictive text input is a feature in search engines, text editors, and messaging apps. This project involves building a predictive text input system using the Trie data structure, enabling fast data retrieval based on the user’s input. Tries are ideal for this project due to their efficiency in storing and searching strings.
Time Taken: 1 week
Project Complexity: Intermediate
Learning Outcomes: This project will teach you about the Trie data structure and its applications in predictive text and autocomplete functionality.
Features of the Project:
- Predicts and suggests text based on partial input
- Supports dynamic text updates and additions
- Highly efficient for large word datasets
Programming Languages Used: Python, Java
Real-world Applications: Commonly used in search bars, mobile keyboards, and code editors to improve typing speed and accuracy.
Source Code: Predictive Text Input Using Trie
9. Job Scheduling Algorithm
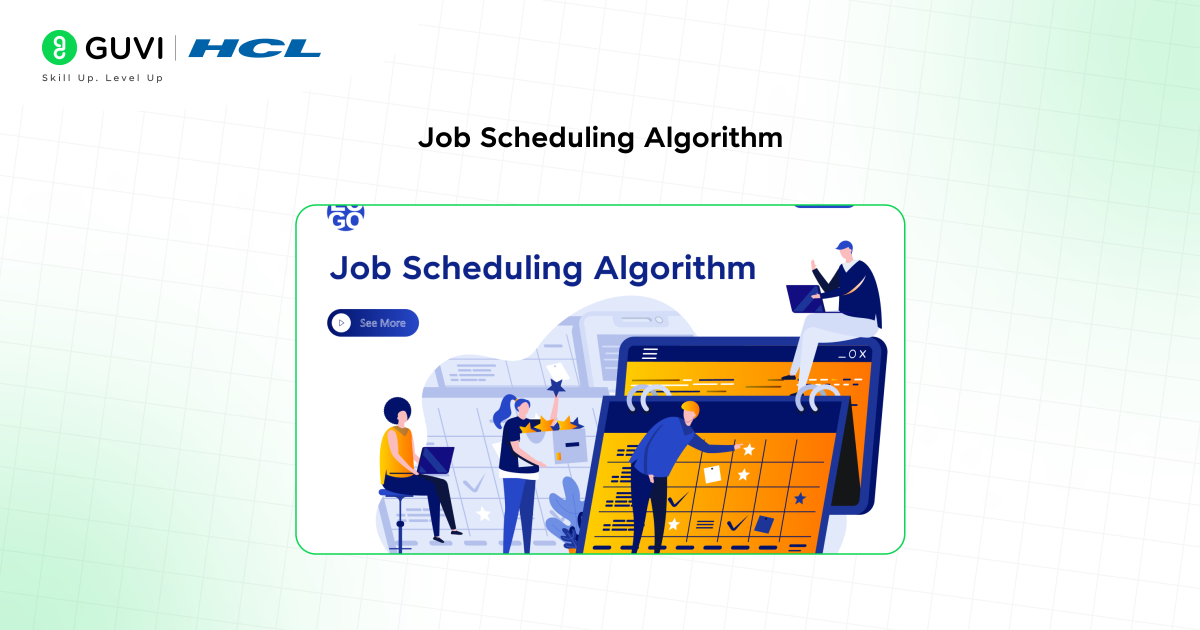
Efficient task management is crucial in both operating systems and cloud applications. This project involves creating a job scheduling algorithm that assigns priorities and resources to various tasks using priority queues and sorting techniques, ensuring that tasks are completed in an optimized order.
Time Taken: 1–2 weeks
Project Complexity: Intermediate
Learning Outcomes: You’ll understand the importance of scheduling algorithms, priority queues, and efficient resource management in applications.
Features of the Project:
- Prioritizes tasks based on deadlines or resource needs
- Implements sorting and priority queue algorithms
- Scalable to handle various types of tasks and priorities
Programming Languages Used: C
Real-world Applications: Used in operating systems, cloud computing, and applications that require optimal resource allocation.
Source Code: Job Scheduling Algorithm
10. Chatbot with Real-Time Response Analysis
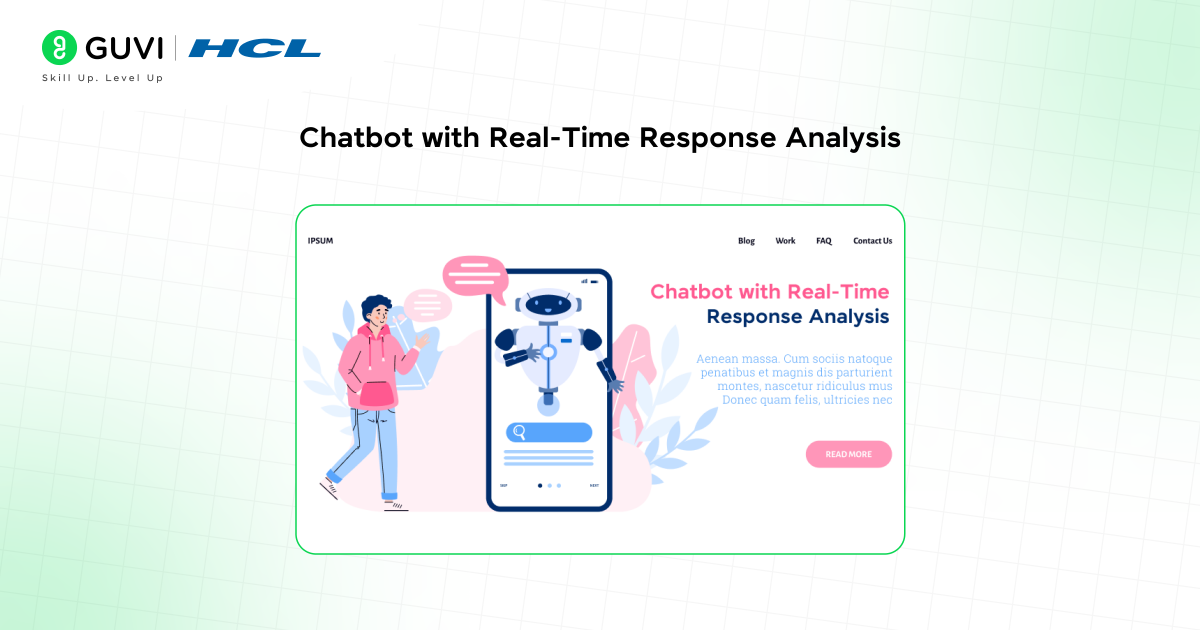
Chatbots are increasingly popular in customer service, and this project lets you create a bot with real-time response capabilities.
Using tree structures, priority queues, and search algorithms, you’ll build a chatbot that can analyze and respond to user queries instantly, offering relevant suggestions and support.
Time Taken: 2–3 weeks
Project Complexity: Advanced
Learning Outcomes: This project will enhance your understanding of real-time search algorithms, response prioritization, and the application of trees in building decision-making systems.
Features of the Project:
- Real-time query handling and response generation
- Learns and improves responses over time
- Can be extended with a knowledge base or FAQ integration
Programming Languages Used: Python, JavaScript
Real-world Applications: Used in customer support, e-commerce, and social media to provide quick and automated assistance.
Source Code: Chatbot with Real-Time Response Analysis
These data structures and algorithms project ideas will give you a strong understanding of what each project entails and what you can expect to learn as you progress.
Conclusion
In conclusion, tackling data structure and algorithms projects is one of the best ways to improve your programming skills. Not only do these projects solidify your understanding of essential concepts, but they also prepare you for real-world coding challenges.
Whether you’re aiming to refine your DSA skills for job interviews or to develop better software solutions, these project ideas cover various levels of complexity and real-world relevance.
FAQs
1. What are the easy Data Structure and Algorithms project ideas for beginners?
For beginners, projects like a Library Management System or Predictive Text Input using Trie are great options. They involve basic data structures like queues, stacks, and trees and help you grasp DSA essentials.
2. Why are Data Structure and Algorithms projects important for beginners?
DSA projects provide practical experience, helping you understand the importance of efficiency in code and how to apply algorithms in real-life scenarios, preparing you for technical interviews and career growth.
3. What skills can beginners learn from Data Structure and Algorithms projects?
You’ll learn to design efficient algorithms, optimize code, and gain a better understanding of data storage, retrieval, and manipulation. These projects also strengthen problem-solving skills.
4. Which Data Structure and Algorithms project is recommended for someone with no prior programming experience?
The Library Management System project is ideal for beginners without much coding experience. It’s a manageable introduction to data structures like queues and stacks in a practical setting.
5. How long does it typically take to complete a beginner-level Data Structure and Algorithms project?
Beginner projects generally take around one week to complete. With dedication and focus, you’ll have plenty of time to understand and apply the core concepts effectively.
Did you enjoy this article?