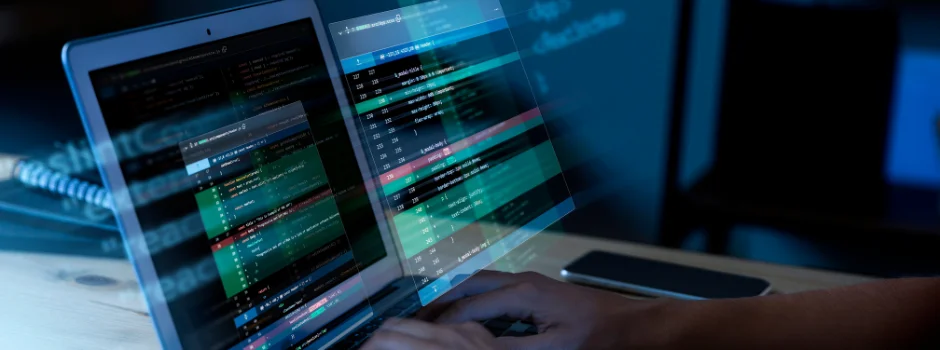
Debouncing and Throttling in JavaScript: A Beginner’s Guide
Jan 30, 2025 2 Min Read 461 Views
(Last Updated)
JavaScript applications often need to handle high-frequency events like scrolling, resizing, and user input efficiently to maintain smooth performance. Without optimization, these events can lead to excessive function calls, causing lag and performance issues.
Two common techniques for controlling the execution frequency of functions are Debouncing and Throttling. While both methods help optimize event handling, they serve distinct purposes and are used in different scenarios.
This article explores how debouncing and throttling work, their use cases, and practical implementations in JavaScript.
Table of contents
- What is Debouncing?
- What is Throttling?
- Examples in Action
- Debouncing Search Input
- Throttling Scroll Event
- Conclusion
What is Debouncing?
Debouncing in JavaScript makes sure that a function isn’t called immediately one after the other. Rather, it postpones the function’s execution until after a certain amount of time has passed since its previous invocation.
When you wish to minimize the number of times a function is executed, this can help. It makes sure the function is only called once following a sequence of immediate invocations.
Use Cases:
- Search input suggestions: Only invoke the search API after the user has finished/stopped typing.
- Window resizing: Executing the resizing logic after the user has finished/stopped resizing the window.
- Button clicks: Prevent double clicks on a button.
Example:
function debounce(inputFunction, delay) {
let timeoutId;
return function() {
clearTimeout(timeoutId); // Reset the timer every time the function is called
timeoutId = setTimeout(() => {
inputFunction(); // Call the actual function after the delay
}, delay);
};
}
// Example usage
const handleResize = debounce(() => {
console.log('Resized!');
}, 500);
window.addEventListener('resize', handleResize);
In this example:
- debounce() is a higher-order function that takes a function (inputFunction) and a delay (in milliseconds).
- If the user continuously resizes the window, the (handleResize) function will only be executed 500ms after the last resize event.
What is Throttling?
The purpose of throttling is to limit the number of times a function can be called within a given time frame. Unlike debouncing, throttling does not delay the execution of the function but restricts the number of times it can be called.
When you want to make sure that a function executes at consistent intervals throughout an ongoing action, this is helpful.
Use Cases:
- Scrolling events: Execute scroll-related logic (such as infinite scroll and lazy loading) periodically while the user scrolls.
- Mouse movement tracking: Track mouse position at regular intervals for performance-sensitive tasks.
- API rate limiting: Limit the number of API calls made over a period of time.
Example:
function throttle(inputFunction, limit) {
let lastCall = 0;
return function() {
const now = Date.now();
if (now - lastCall >= limit) {
lastCall = now;
inputFunction(); // Execute the function if time limit has passed
}
};
}
// Example usage
const handleScroll = throttle(() => {
console.log('Scrolled!');
}, 1000);
window.addEventListener('scroll', handleScroll);
In this example:
- throttle() is a higher-order function that takes a function (inputFunction) and a limit (in milliseconds).
- The (handleScroll) function will only be executed once every 1000ms, even if the user continues to scroll.
Aspect | Debouncing | Throttling |
Function execution | The function is executed only after a defined duration of inactivity. | The function is executed at regular intervals, regardless of how many times the event is triggered. |
Use case | Suitable in situations where the outcome matters (such as when an API request is sent after the user has finished typing). | Suitable for situations requiring recurring updates (such as adjusting the scroll position when the user scrolls). |
Delay type | Delay the execution process till the end of the specified delay time. | irrespective of how frequently the event is triggered, performs the function at each designated delay interval. |
Examples in Action
1. Debouncing Search Input
<input type="text" id="search-box" placeholder="Search...">
<script>
const input = document.getElementById('search-box');
const fetchSearchResults = debounce(() => {
console.log('Fetching search results...');
}, 500);
input.addEventListener('input', fetchSearchResults);
</script>
In this example, the fetchSearchResults function is only triggered 500 ms after the user stops typing, preventing the function from being executed on every keystroke.
2. Throttling Scroll Event
<script>
const fetchMoreContent = throttle(() => {
console.log('Loading more content...');
}, 2000);
window.addEventListener('scroll', fetchMoreContent);
</script>
In this example, even if the user scrolls continuously, fetchMoreContent will only be called once every two seconds. This is how Debouncing and Throttling works in JavaScript.
In case, you want to learn more about “Debouncing and Throttling in JavaScript” and gain in-depth knowledge on full-stack development, consider enrolling for GUVI’s certified Full-stack Development Course that teaches you everything from scratch with an industry-grade certificate!
Conclusion
In conclusion, both debouncing and throttling are essential techniques for improving JavaScript performance, especially when dealing with frequent event triggers.
Debouncing ensures a function executes only after a period of inactivity, making it ideal for search inputs and resize events. Throttling, on the other hand, ensures a function executes at regular intervals, making it suitable for scroll tracking and API rate limiting.
Understanding when and how to use these strategies can significantly enhance web application efficiency, ensuring smoother user experiences and optimized resource usage.
Did you enjoy this article?