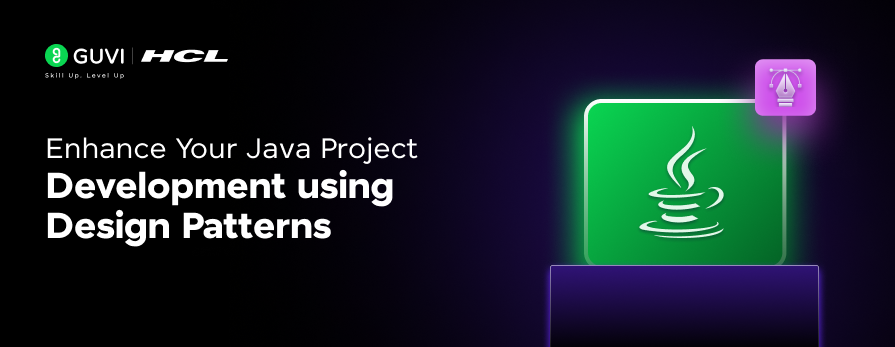
Enhance Your Java Project Development using Design Patterns
Oct 09, 2024 5 Min Read 177 Views
(Last Updated)
Design patterns are essential tools for enhancing the efficiency and scalability of your Java project development. They provide proven solutions to common software design problems, enabling you to build robust, flexible, and maintainable code.
In this article, we’ll explore how leveraging design patterns can significantly improve your Java projects by promoting best practices, reducing code redundancy, and simplifying complex structures.
Let’s dive into the world of design patterns and discover how they can enhance your Java project development!
Table of contents
- What Are Design Patterns?
- Key Characteristics of Design Patterns
- Types of Design Patterns in Java
- Creational Patterns
- Structural Patterns
- Behavioral Patterns
- When to Use Design Patterns?
- Best Practices for Implementing Design Patterns
- Conclusion
What Are Design Patterns?
Design patterns are proven solutions to common software design problems. They are not specific code implementations but rather templates or guidelines that can be adapted to fit various situations. By using design patterns, developers can create systems that are easier to understand, maintain, and extend in Java.
Key Characteristics of Design Patterns
- Reusability: Patterns can be reused across different projects, reducing the time spent on problem-solving.
- Maintainability: Code organized around design patterns is easier to modify and extend.
- Communication: Patterns provide a shared vocabulary for developers, making it easier to discuss design concepts.
- Best Practices: Patterns encapsulate best practices that have been tested in various scenarios.
Types of Design Patterns in Java
Design patterns are categorized into three main types: Creational, Structural, and Behavioral. Each category addresses different aspects of software design.
1. Creational Patterns
Creational patterns focus on object creation mechanisms, providing various ways to create objects while controlling their instantiation. Here are some of the most common creational patterns:
1. Singleton Pattern
- Definition: Ensures that a class has only one instance and provides a global point of access to it.
- Use Case: Useful for managing shared resources, such as configuration settings or logging.
Implementation Example:
java
public class Singleton {
private static Singleton instance;
private Singleton() {
// Private constructor to prevent instantiation
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
2. Factory Method Pattern
- Definition: Defines an interface for creating an object but allows subclasses to alter the type of objects that will be created.
- Use Case: Useful when a class cannot anticipate the type of objects it needs to create.
Implementation Example:
java
abstract class Product {
public abstract void use();
}
class ConcreteProductA extends Product {
@Override
public void use() {
System.out.println("Using Product A");
}
}
class ConcreteProductB extends Product {
@Override
public void use() {
System.out.println("Using Product B");
}
}
abstract class Creator {
public abstract Product factoryMethod();
}
class ConcreteCreatorA extends Creator {
@Override
public Product factoryMethod() {
return new ConcreteProductA();
}
}
class ConcreteCreatorB extends Creator {
@Override
public Product factoryMethod() {
return new ConcreteProductB();
}
}
3. Abstract Factory Pattern
- Definition: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Use Case: Useful for creating products that share a common theme.
Implementation Example:
java
interface GUIFactory {
Button createButton();
Checkbox createCheckbox();
}
class WinFactory implements GUIFactory {
public Button createButton() {
return new WinButton();
}
public Checkbox createCheckbox() {
return new WinCheckbox();
}
}
class MacFactory implements GUIFactory {
public Button createButton() {
return new MacButton();
}
public Checkbox createCheckbox() {
return new MacCheckbox();
}
}
4. Builder Pattern
- Definition: Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
- Use Case: Particularly helpful when an object requires many parameters.
Implementation Example:
java
class Product {
private String part1;
private String part2;
public void setPart1(String part1) {
this.part1 = part1;
}
public void setPart2(String part2) {
this.part2 = part2;
}
}
class Builder {
private Product product;
public Builder() {
product = new Product();
}
public Builder buildPart1(String part1) {
product.setPart1(part1);
return this;
}
public Builder buildPart2(String part2) {
product.setPart2(part2);
return this;
}
public Product build() {
return product;
}
}
5. Prototype Pattern
- Definition: Creates new objects by copying an existing object, known as the prototype.
- Use Case: Useful when object creation is costly in terms of time or resources.
Implementation Example:
java
abstract class Prototype {
public abstract Prototype clone();
}
class ConcretePrototype extends Prototype {
private String field;
public ConcretePrototype(String field) {
this.field = field;
}
@Override
public Prototype clone() {
return new ConcretePrototype(field);
}
}
2. Structural Patterns
Structural patterns deal with the composition of classes and objects, focusing on how they can work together to form larger structures. Here are some common structural patterns:
1. Adapter Pattern
- Definition: Allows incompatible interfaces to work together by acting as a bridge between two incompatible interfaces.
- Use Case: Useful when integrating new features into existing systems.
Implementation Example:
java
interface Target {
void request();
}
class Adaptee {
public void specificRequest() {
System.out.println("Specific request");
}
}
class Adapter implements Target {
private Adaptee adaptee;
public Adapter(Adaptee adaptee) {
this.adaptee = adaptee;
}
@Override
public void request() {
adaptee.specificRequest();
}
}
2. Decorator Pattern
- Definition: Adds new functionality to an existing object without altering its structure.
- Use Case: Useful for adhering to the Single Responsibility Principle by allowing behavior to be added dynamically.
Implementation Example:
java
interface Coffee {
String getDescription();
double cost();
}
class SimpleCoffee implements Coffee {
public String getDescription() {
return "Simple coffee";
}
public double cost() {
return 1.0;
}
}
abstract class CoffeeDecorator implements Coffee {
protected Coffee coffee;
public CoffeeDecorator(Coffee coffee) {
this.coffee = coffee;
}
}
class MilkDecorator extends CoffeeDecorator {
public MilkDecorator(Coffee coffee) {
super(coffee);
}
public String getDescription() {
return coffee.getDescription() + ", with milk";
}
public double cost() {
return coffee.cost() + 0.5;
}
}
3. Facade Pattern
- Definition: Provides a simplified interface to a complex subsystem, making it easier to use.
- Use Case: Useful for hiding the complexities of a system.
Implementation Example:
java
class SubsystemA {
public void operationA() {
System.out.println("Operation A");
}
}
class SubsystemB {
public void operationB() {
System.out.println("Operation B");
}
}
class Facade {
private SubsystemA subsystemA;
private SubsystemB subsystemB;
public Facade() {
subsystemA = new SubsystemA();
subsystemB = new SubsystemB();
}
public void operation() {
subsystemA.operationA();
subsystemB.operationB();
}
}
4. Composite Pattern
- Definition: Allows clients to treat individual objects and compositions of objects uniformly.
- Use Case: Useful for building tree structures.
Implementation Example:
java
interface Component {
void operation();
}
class Leaf implements Component {
public void operation() {
System.out.println("Leaf operation");
}
}
class Composite implements Component {
private List<Component> children = new ArrayList<>();
public void add(Component component) {
children.add(component);
}
public void operation() {
for (Component child : children) {
child.operation();
}
}
}
5. Proxy Pattern
- Definition: Provides a surrogate or placeholder for another object to control access to it.
- Use Case: Useful for lazy initialization, access control, and logging.
Implementation Example:
java
interface Image {
void display();
}
class RealImage implements Image {
private String filename;
public RealImage(String filename) {
this.filename = filename;
loadImageFromDisk();
}
private void loadImageFromDisk() {
System.out.println("Loading " + filename);
}
public void display() {
System.out.println("Displaying " + filename);
}
}
class ProxyImage implements Image {
private RealImage realImage;
private String filename;
public ProxyImage(String filename) {
this.filename = filename;
}
public void display() {
if (realImage == null) {
realImage = new RealImage(filename);
}
realImage.display();
}
}
3. Behavioral Patterns
Behavioral patterns focus on communication between objects, defining how they interact and collaborate. Here are some common behavioral patterns:
1. Observer Pattern
- Definition: Defines a one-to-many dependency between objects, allowing one object to notify multiple observers of state changes.
- Use Case: Particularly useful in event-driven programming.
Implementation Example:
java
interface Observer {
void update(String message);
}
class ConcreteObserver implements Observer {
private String name;
public ConcreteObserver(String name) {
this.name = name;
}
public void update(String message) {
System.out.println(name + " received message: " + message);
}
}
class Subject {
private List<Observer> observers = new ArrayList<>();
public void addObserver(Observer observer) {
observers.add(observer);
}
public void notifyObservers(String message) {
for (Observer observer : observers) {
observer.update(message);
}
}
}
2. Strategy Pattern
- Definition: Enables selecting an algorithm’s behavior at runtime.
- Use Case: Allows the behavior of a class to be defined by different strategies.
Implementation Example:
java
interface Strategy {
int execute(int a, int b);
}
class AddStrategy implements Strategy {
public int execute(int a, int b) {
return a + b;
}
}
class SubtractStrategy implements Strategy {
public int execute(int a, int b) {
return a - b;
}
}
class Context {
private Strategy strategy;
public Context(Strategy strategy) {
this.strategy = strategy;
}
public int executeStrategy(int a, int b) {
return strategy.execute(a, b);
}
}
3. Command Pattern
- Definition: Encapsulates a request as an object, allowing for parameterization of clients with queues, requests, and operations.
- Use Case: Useful for implementing undo operations.
Implementation Example:
java
interface Command {
void execute();
}
class Light {
public void turnOn() {
System.out.println("Light is ON");
}
public void turnOff() {
System.out.println("Light is OFF");
}
}
class LightOnCommand implements Command {
private Light light;
public LightOnCommand(Light light) {
this.light = light;
}
public void execute() {
light.turnOn();
}
}
class LightOffCommand implements Command {
private Light light;
public LightOffCommand(Light light) {
this.light = light;
}
public void execute() {
light.turnOff();
}
}
class RemoteControl {
private Command command;
public void setCommand(Command command) {
this.command = command;
}
public void pressButton() {
command.execute();
}
}
4. State Pattern
- Definition: Allows an object to alter its behavior when its internal state changes.
- Use Case: Useful for managing state transitions.
Implementation Example:
java
interface State {
void handle();
}
class ConcreteStateA implements State {
public void handle() {
System.out.println("Handling state A");
}
}
class ConcreteStateB implements State {
public void handle() {
System.out.println("Handling state B");
}
}
class Context {
private State state;
public void setState(State state) {
this.state = state;
}
public void request() {
state.handle();
}
}
5. Template Method Pattern
- Definition: Defines the skeleton of an algorithm in a method, deferring some steps to subclasses.
- Use Case: Allows subclasses to redefine certain steps of an algorithm without changing its structure.
Implementation Example:
java
abstract class AbstractClass {
public final void templateMethod() {
stepOne();
stepTwo();
stepThree();
}
protected abstract void stepOne();
protected abstract void stepTwo();
private void stepThree() {
System.out.println("Step three executed");
}
}
class ConcreteClass extends AbstractClass {
protected void stepOne() {
System.out.println("Step one executed");
}
protected void stepTwo() {
System.out.println("Step two executed");
}
}
When to Use Design Patterns?
Design patterns should be considered when:
- You encounter recurring problems in software design.
- You need to improve code reusability and maintainability.
- You want to enhance communication within a development team.
- You anticipate the need for scalability and flexibility in your software.
Best Practices for Implementing Design Patterns
- Understand the Problem: Before applying a design pattern, ensure you fully understand the problem you are trying to solve.
- Choose the Right Pattern: Select the design pattern that best fits the problem context. Avoid using patterns just for the sake of it.
- Keep It Simple: Avoid over-engineering. Implement patterns only when they add value to your design.
- Document Your Patterns: Clearly document the patterns you use in your codebase to facilitate understanding among team members.
- Refactor When Necessary: As your application evolves, revisit and refactor your design patterns to ensure they still fit the current context.
In case, you want to learn more about Java Full stack development and how to become one, consider enrolling for GUVI’s Certified Java Full-stack Developer Course that teaches you everything from scratch and make sure you master it!
Conclusion
Design patterns in Java are invaluable tools that help developers create robust, maintainable, and scalable software. By understanding and applying these patterns, you can streamline your coding processes, improve collaboration, and produce high-quality applications.
As you continue your journey in software development, familiarize yourself with these patterns to enhance your coding practices and problem-solving skills. Design patterns are not just theoretical concepts; they are practical solutions that can significantly improve the quality of your software projects.
Did you enjoy this article?