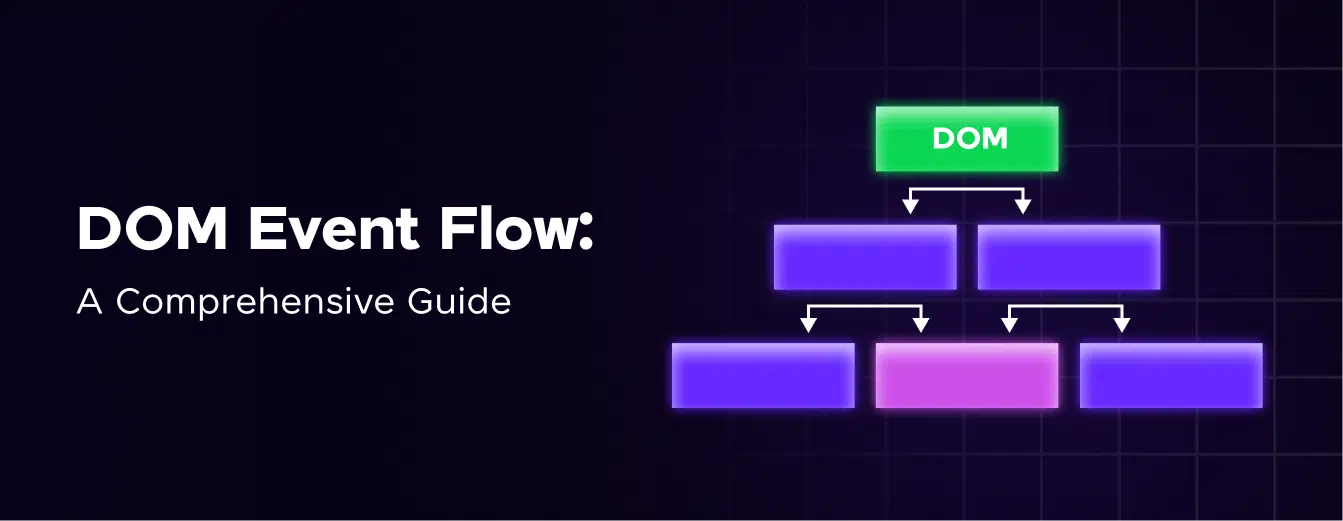
DOM Event Flow: A Comprehensive Guide
Jun 26, 2024 6 Min Read 284 Views
(Last Updated)
Learning how events propagate through the Document Object Model (DOM) is fundamental for every web developer aiming to create interactive and responsive web applications. DOM Event Flow refers to the sequence in which events are processed and handled from their origin to their destination within the DOM structure.
This concept is important because it dictates how events interact with elements on a webpage, influencing user interactions and application behavior.
In this blog post, we will explore the DOM Event Flow, exploring its phases—capturing, targeting, and bubbling—and discussing strategies for event handling, browser compatibility considerations, and more. By the end, you’ll have a clear understanding of how to use DOM Event Flow to create robust and efficient web applications. Let’s begin!
Table of contents
- What is DOM Event Flow?
- DOM Event Flow Explained
- Event Capturing Phase
- Target Phase
- Bubbling Phase
- Practical Examples of Event Flow
- Event Propagation Strategies
- Browser Compatibility and Cross-Browser Issues
- Conclusion
- FAQs
- What is the difference between event capturing and event bubbling in DOM Event Flow?
- How can I control event propagation in DOM Event Flow?
- Why is understanding DOM Event Flow important for web development?
What is DOM Event Flow?
DOM Event Flow, also known as Event Propagation, refers to the order in which events are received on the page when an event occurs. It describes the path an event takes through the HTML Document Object Model (DOM) after it is triggered.
There are two phases in the event flow:
- Capturing Phase: This phase starts from the window object and travels down through the DOM tree, triggering the event handlers registered for the capturing phase on each node it encounters along the way until it reaches the target element.
- Bubbling Phase: After reaching the target element, the event propagates back up through the DOM tree, triggering the event handlers registered for the bubbling phase on each node it encounters along the way until it reaches the window object.
The capturing phase occurs first, followed by the target phase, and finally, the bubbling phase. Most events in the browser follow the bubbling phase, which means event handlers registered on parent elements will be triggered after the event handlers on the target element.
The event flow is important because it allows developers to control the order in which event handlers are executed and to handle events at different levels of the DOM hierarchy. For example, you can use the addEventListener method with the capture option set to true to register an event handler for the capturing phase or set it to false (or omit it) for the bubbling phase.
Understanding the DOM Event Flow is crucial for writing efficient and effective event-handling code, especially in complex web applications where multiple event handlers may be attached to different elements in the DOM.
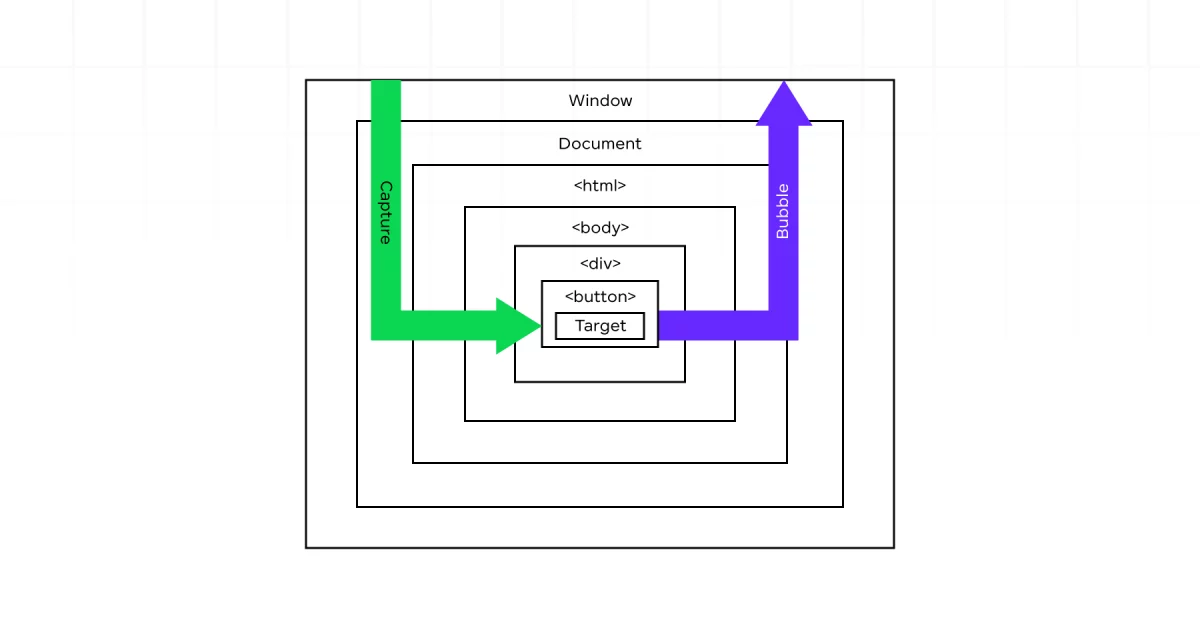
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Career Program with Placement Assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Additionally, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript self-paced certification course.
DOM Event Flow Explained
The DOM Event Flow consists of three distinct phases: the Event Capturing Phase, the Target Phase, and the Bubbling Phase. Understanding these phases is crucial for writing efficient and effective event-handling code, especially in complex web applications where multiple event handlers may be attached to different elements in the DOM.
1. Event Capturing Phase
The Event Capturing Phase is the first phase of the DOM Event Flow. It starts from the window object, which is the topmost node in the DOM tree, and travels down through the DOM tree, triggering the event handlers registered for the capturing phase on each node it encounters along the way until it reaches the target element.
During the capturing phase, the event first fires on the window object, then on the document object, followed by the HTML element, and so on, until it reaches the target element where the event was triggered.
It’s important to note that the capturing phase is not widely used in modern web development. Most event handlers are registered for the bubbling phase, which is the default behavior in most browsers.
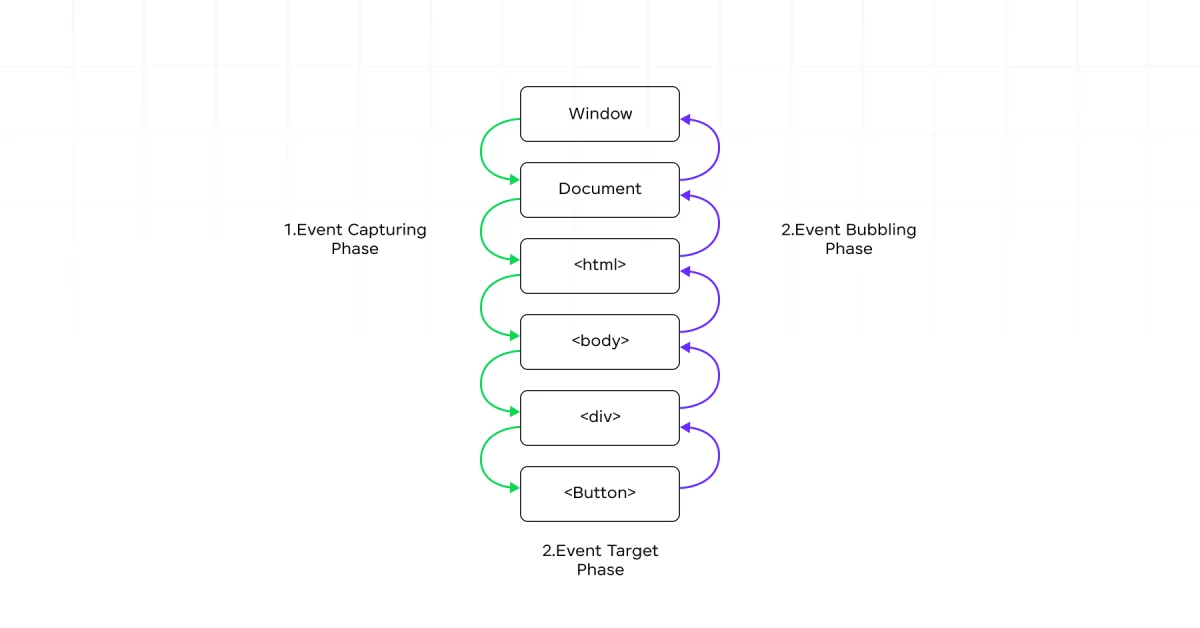
Also Read: How to Master DOM in Selenium for Beginners
2. Target Phase
The Target Phase is the second phase of the DOM Event Flow, and it occurs immediately after the capturing phase. In this phase, the event is dispatched to the target element, which is the element where the event was triggered.
During the target phase, the event handlers registered on the target element for the specific event type are executed. This phase is often overlooked because it is a single point in the event flow, but it is essential for handling events directly on the target element.
Also Read: What is DOM manipulation? Common Tasks and Elements [2024]
3. Bubbling Phase
The Bubbling Phase is the third and final phase of the DOM Event Flow. It occurs immediately after the target phase and is the most commonly used phase for event handling in modern web development.
In the bubbling phase, the event propagates back up through the DOM tree, triggering the event handlers registered for the bubbling phase on each node it encounters along the way until it reaches the window object.
The bubbling phase starts from the target element and works its way up through the parent elements, triggering event handlers registered on each parent element in the DOM hierarchy, until it reaches the topmost node (the window object).
During the bubbling phase, event handlers registered on parent elements have the opportunity to handle the event, allowing for more flexible and modular event handling in complex applications.
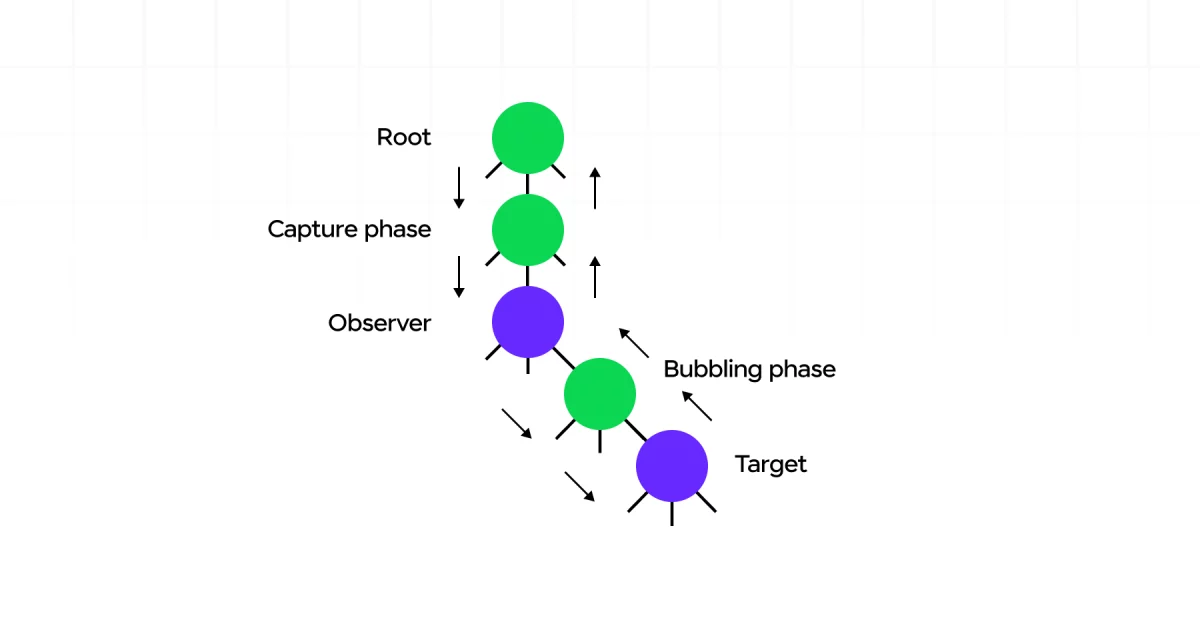
Also Read: Event Bubbling in DOM: An Interesting Technique To Know About [2024]
4. Practical Examples of Event Flow
To better understand the DOM Event Flow, let’s consider a practical example. Imagine an HTML structure with nested elements, such as a button inside a div inside a section:
<section id="parent">
<div id="child">
<button id="target">Click me</button>
</div>
</section>
When a user clicks the button, the event flow proceeds as follows:
- Capturing Phase:
- The click event is first dispatched to the window object.
- The event then travels down through the document object and the HTML element.
- If there are any event handlers registered for the capturing phase on the section, div, or button elements, they will be executed in this order.
- Target Phase: 4. The click event is dispatched to the target element (the button). 5. Any event handlers registered directly on the button for the click event are executed.
- Bubbling Phase: 6. The event starts bubbling up from the button. 7. If there are any event handlers registered for the bubbling phase on the div element, they are executed. 8. If there are any event handlers registered for the bubbling phase on the section element, they are executed. 9. Finally, if there are any event handlers registered for the bubbling phase on the document or window objects, they are executed.
By understanding the DOM Event Flow, developers can leverage event-handling strategies that fit their application’s needs. For example, if an event needs to be handled consistently across multiple elements, registering the event handler on a common parent element during the bubbling phase can be more efficient than attaching the same handler to each element.
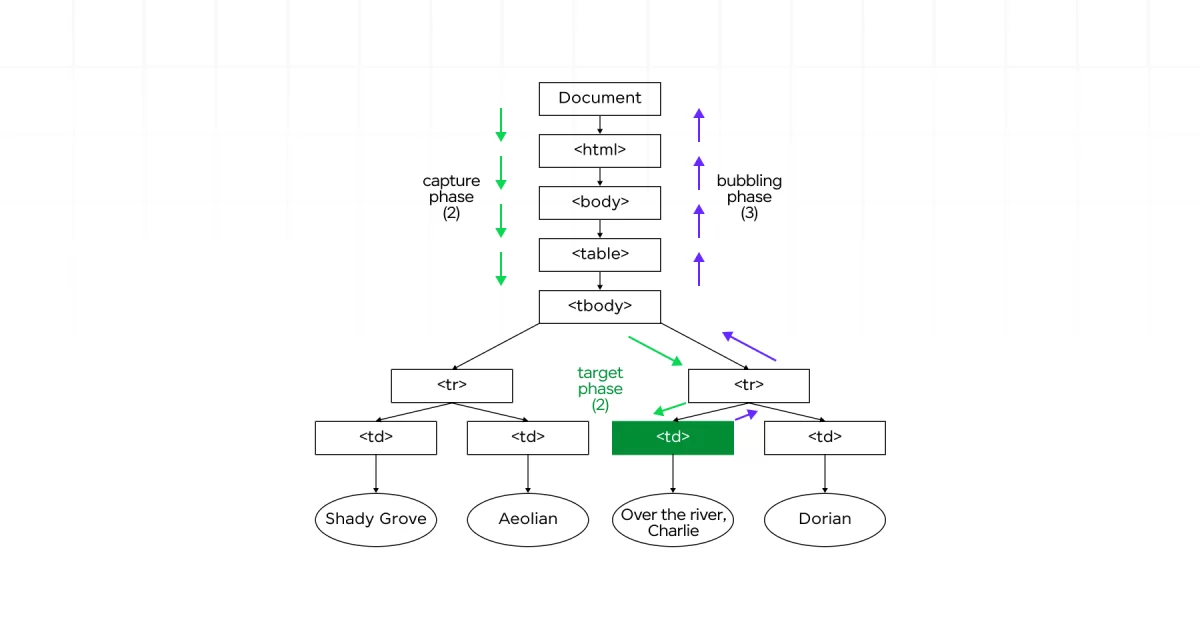
Also Read: A Comprehensive Guide On Virtual DOM: Learn Everything About It [2024]
5. Event Propagation Strategies
Depending on the requirements of a web application, developers can choose different event propagation strategies to handle events effectively. Here are some common strategies:
a. Event Capturing: While not commonly used, the event capturing phase can be useful in certain scenarios. Developers can register event handlers for the capturing phase by passing the capture option as true when using the addEventListener method:
element.addEventListener('click', handleClick, true); // true for capturing phase
This approach can be beneficial when you want to handle an event before it reaches the target element, such as implementing custom event behavior or modifying the event object before it is processed by other handlers.
b. Event Bubbling (Default): The bubbling phase is the most widely used event propagation strategy. By default, event handlers are registered for the bubbling phase when using the addEventListener method without specifying the capture option:
element.addEventListener('click', handleClick); // Default is bubbling phase
This strategy allows for more flexible and modular event handling, as event handlers can be registered on parent elements to handle events triggered by their child elements. It promotes code reusability and maintainability, especially in complex applications with nested DOM structures.
c. Event Delegation: Event delegation is a powerful technique that leverages the bubbling phase of the DOM Event Flow. Instead of attaching event handlers to multiple child elements, a single event handler is attached to a parent element. When an event bubbles up from a child element, the parent element’s event handler is invoked, and the target element is identified based on the event’s properties (e.g., event.target).
Event delegation can significantly improve performance and reduce memory usage, especially in scenarios where elements are dynamically added or removed from the DOM. It also simplifies event-handling logic by centralizing it in a single event handler.
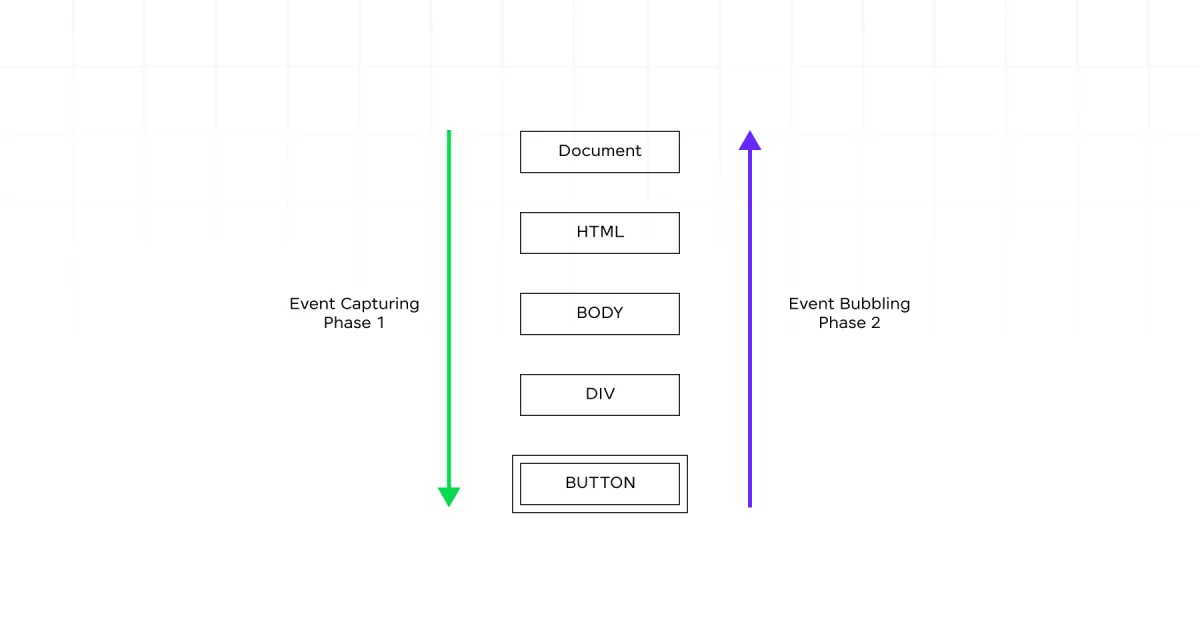
Also Read: What are Events in JavaScript? A Complete Guide
6. Browser Compatibility and Cross-Browser Issues
While the DOM Event Flow is a standardized concept, there are some cross-browser compatibility issues and quirks that developers should be aware of:
a. Legacy Event Model (Internet Explorer < 9): In older versions of Internet Explorer (prior to version 9), the event model was different from the W3C standard. Instead of using the addEventListener method, developers had to rely on the attachEvent and detachEvent methods, which did not support the capturing phase.
b. Event Propagation in Internet Explorer: In Internet Explorer, the event propagation order was different from the standard. The bubbling phase occurred before the capturing phase, which was the opposite of the W3C standard.
c. Event Object Compatibility: The properties and methods available on the event object may vary across different browsers. For example, the stopPropagation method used to stop event propagation may not work consistently across all browsers.
d. Vendor Prefixes: Some browsers may require vendor prefixes for certain event-related properties or methods, such as addEventListener or stopPropagation. Developers should be aware of these prefixes and ensure cross-browser compatibility by using feature detection or polyfills.
To mitigate cross-browser issues, developers can follow best practices, such as using feature detection, polyfills, or libraries like jQuery, which provide a consistent and cross-browser-compatible API for event handling.
Also Read: jQuery DOM Manipulation: Learn Everything About This On-demand Technique [2024]
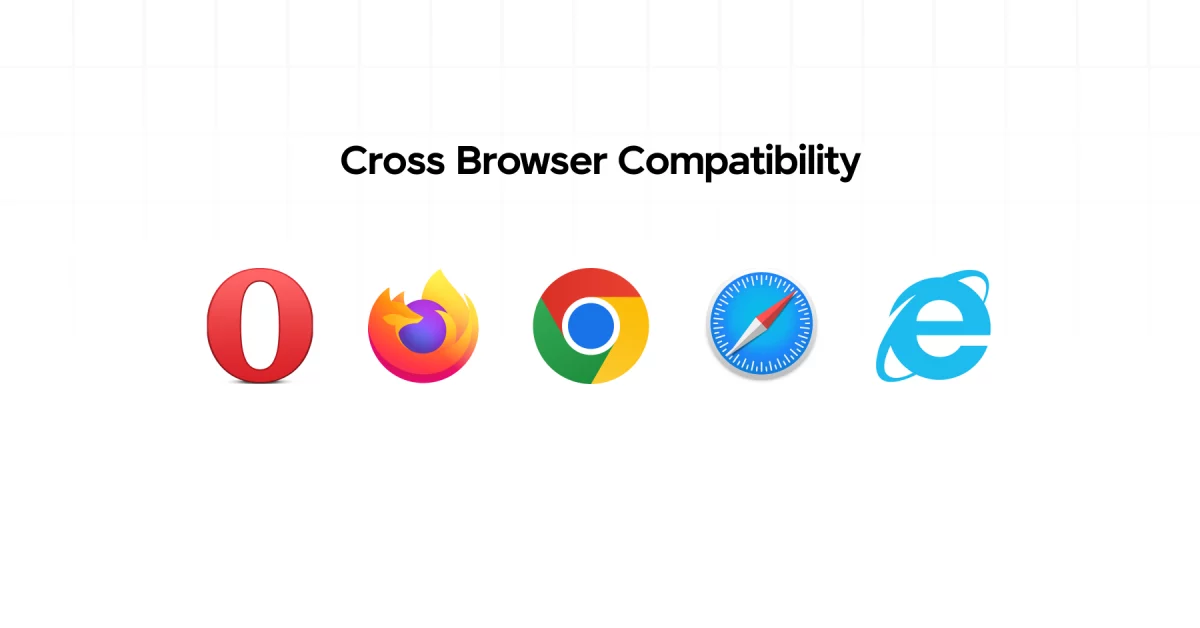
Kickstart your Full Stack Development journey by enrolling in GUVI’s Certified Full Stack Development Career Program with Placement Assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements. Alternatively, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript self-paced course.
Conclusion
The DOM Event Flow is a fundamental concept in JavaScript that governs the order in which events are received and processed within the browser’s rendering engine. Understanding the Event Capturing Phase, Target Phase, and Bubbling Phase is crucial for writing efficient and effective event-handling code, especially in complex web applications with nested DOM structures.
By leveraging different event propagation strategies, such as event capturing, event bubbling, and event delegation, developers can optimize event handling, improve performance, and enhance code reusability and maintainability.
While the DOM Event Flow is a standardized concept, developers should be aware of potential cross-browser compatibility issues and quirks, and take appropriate measures to ensure consistent behavior across different browsers.
Overall, mastering the DOM Event Flow and its intricacies is essential for building robust and responsive web applications that provide a seamless user experience.
Also Read: 10 Best Frontend Development Frameworks
FAQs
What is the difference between event capturing and event bubbling in DOM Event Flow?
Event capturing and event bubbling are two phases of DOM Event Flow that determine the order in which event handlers are executed. During event capturing, events propagate from the root of the DOM tree down to the target element.
In contrast, during event bubbling, events propagate from the target element back up through its ancestors to the root of the DOM tree.
How can I control event propagation in DOM Event Flow?
You can control event propagation using methods like event.stopPropagation() and event.preventDefault() in JavaScript. stopPropagation() prevents further propagation of the current event in both capturing and bubbling phases, ensuring it doesn’t reach parent or descendant elements. preventDefault() prevents the default action associated with the event, such as submitting a form or following a link.
Why is understanding DOM Event Flow important for web development?
Understanding DOM Event Flow is crucial because it enables developers to create interactive and responsive web applications. By knowing how events propagate through the DOM tree—from capturing to target to bubbling phases—developers can effectively handle user interactions, implement event delegation for efficient event management, and troubleshoot issues related to event handling.
Did you enjoy this article?