
What is DOM in Web Development?
Sep 19, 2024 4 Min Read 1678 Views
(Last Updated)
The Document Object Model, or DOM for short, is a big deal in web development. If you want to get good at front-end stuff, you gotta know the DOM. Think of it like a family tree for your web page, where each branch and leaf is a part of the document. This setup lets you mess around with the content and structure of your web pages on the fly.
Let’s discuss DOM in Web Development in-depth:
Table of contents
- Why Bother with the DOM in Web Development?
- What's the Deal with DOM?
- How It’s Built
- How DOM Works in Web Development
- DOM Manipulation
- Event Handling with DOM
- Boost Your Web Development with the DOM
- Dynamic Web Content
- Interactive User Interfaces
- Common Mistakes to Avoid
- Wrap Up
Why Bother with the DOM in Web Development?
Knowing the DOM isn’t just about being able to say you know it; it’s about using it to make cool, interactive web apps. When you get how the DOM works, you can change stuff on your page without making the whole thing reload, handle events like a pro, and build interfaces that users will love.
Being good at DOM manipulation is a must for modern web development. Skills like DOM manipulation and event handling are basics every developer should have down pat. Plus, understanding the DOM tree structure can help you make your sites faster and fix bugs easier.
Mastering the DOM sets you up to build solid, responsive, and user-friendly web apps. It’s the first step to diving into more advanced stuff like frameworks and libraries that lean heavily on DOM manipulation.
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Course with Placement Assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Additionally, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript certification course.
What’s the Deal with DOM?
The Document Object Model (DOM) is like the backstage crew of a theater production. It’s not a programming language, but it’s what makes the magic happen behind the scenes. When you open a webpage, your browser creates a model of that page, known as the DOM. This model is a tree structure where each node represents a part of the document (like an element or text). Without the DOM, the web would be as exciting as watching paint dry.
How It’s Built
Think of the DOM as a family tree. At the top, you’ve got the document object, the big boss. From there, it branches out into child nodes, forming a tree-like structure.
HTML Document
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a paragraph.</p>
</body>
</html>
Corresponding DOM Structure
- document
- html
- head
- title
- “Page Title”
- body
- h1
- “Hello, World!”
- p
- “This is a paragraph.”
In this tree, each element is a node. The html
element is the parent of both the head
and body
elements. The body
element is the parent of the h1
and p
elements. It’s like a family reunion where everyone’s connected.
Read: DOM Nodes: Understanding and Manipulating the Document Object Model
Here’s a quick table to break it down:
Node Type | Description |
---|---|
document | The root of the DOM tree |
html | The root element of the HTML document |
head | Contains meta-information about the document |
body | Contains the content of the document |
h1, p | Elements within the body containing text |
By getting the hang of the DOM’s structure, you can navigate, access, and tweak elements to make your web content pop.
How DOM Works in Web Development
Getting a grip on how the Document Object Model (DOM) works is a game-changer for web development. Let’s break it down into two main parts: DOM manipulation and event handling.
DOM Manipulation
DOM manipulation is all about tweaking the structure, content, or style of a web page on the fly using JavaScript. This is how you make your web pages come alive and respond to user actions. Think of it as the magic wand that lets you add, remove, or change elements, attributes, and styles.
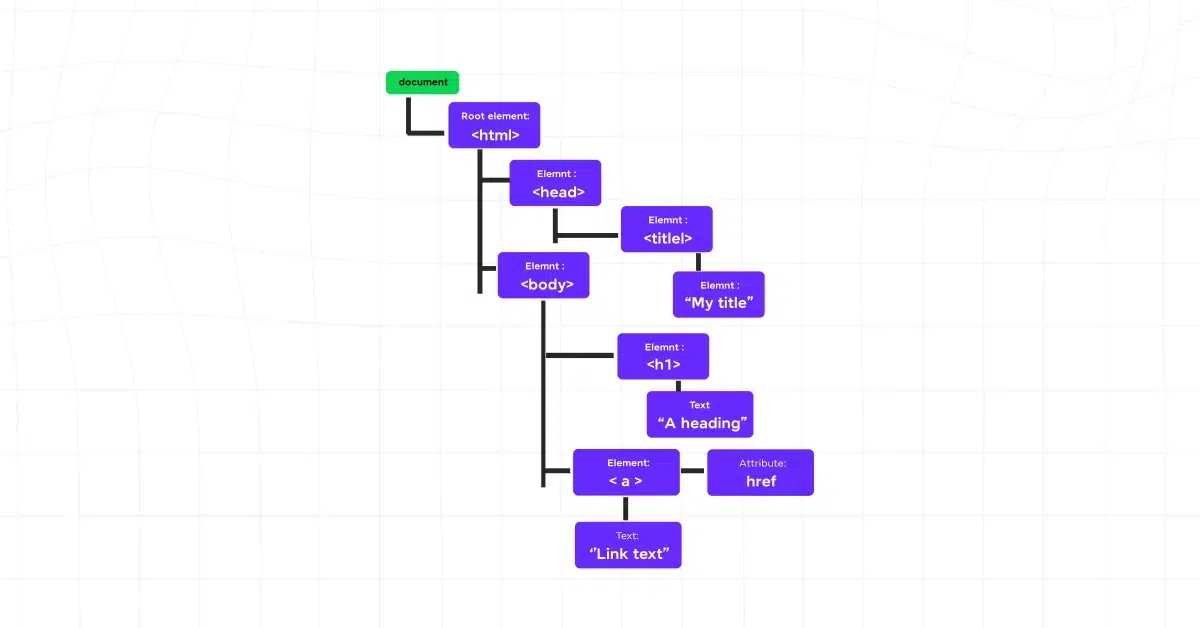
Here are some handy methods for DOM manipulation:
getElementById(id)
: Grabs an element by its ID.getElementsByClassName(class)
: Fetches elements by their class name.querySelector(selector)
: Snags the first element that matches a CSS selector.querySelectorAll(selector)
: Collects all elements that match a CSS selector.createElement(tag)
: Whips up a new HTML element.appendChild(node)
: Adds a new child node to an element.removeChild(node)
: Kicks out a child node from an element.setAttribute(name, value)
: Sets an attribute on an element.classList.add(className)
: Adds a class to an element.classList.remove(className)
: Removes a class from an element.
For more juicy details and cool tricks, check out our article on DOM manipulation.
Event Handling with DOM
Event handling is what makes your web pages interactive. Events are things like clicks, key presses, or mouse movements. By using event listeners, you can run specific code when these events happen.
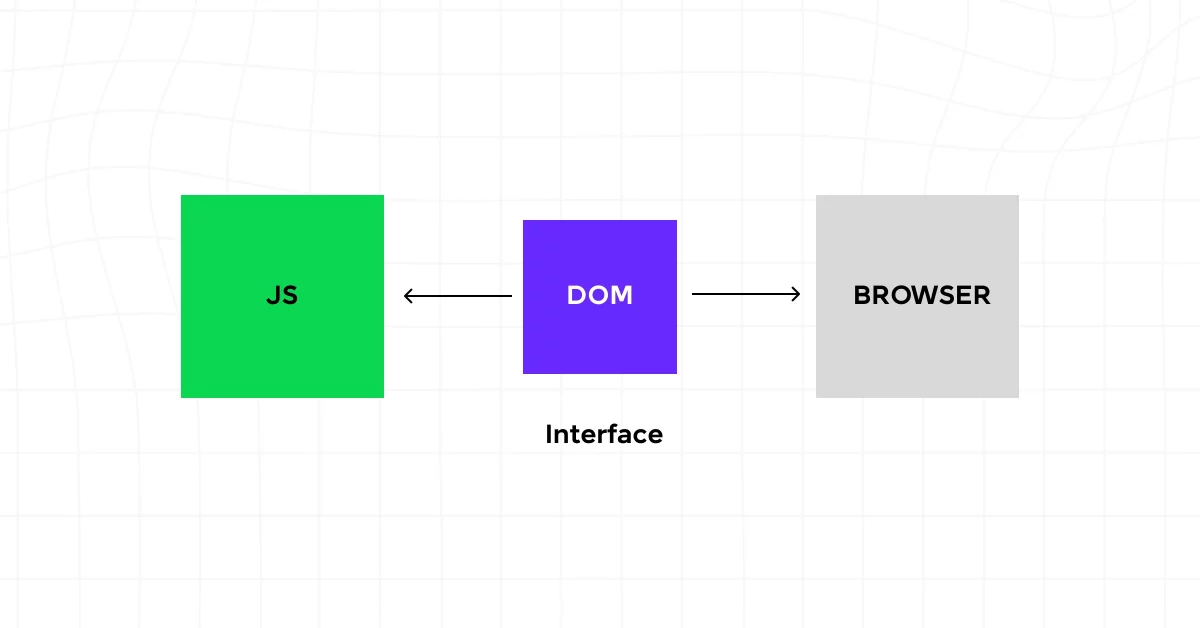
Here are some common events in the DOM:
click
: Fires when an element is clicked.mouseover
: Fires when the mouse pointer hovers over an element.mouseout
: Fires when the mouse pointer leaves an element.keydown
: Fires when a key is pressed.keyup
: Fires when a key is released.
To handle events, you use the addEventListener
method:
document.getElementById('myButton').addEventListener('click', function() {
alert('Button clicked!');
});
This snippet attaches a click event listener to a button with the ID myButton
. When the button gets clicked, an alert pops up.
Boost Your Web Development with the DOM
Using the Document Object Model (DOM) can take your web projects to the next level. By getting the hang of the DOM, you can make your web pages come alive with dynamic content and interactive features that keep users hooked.
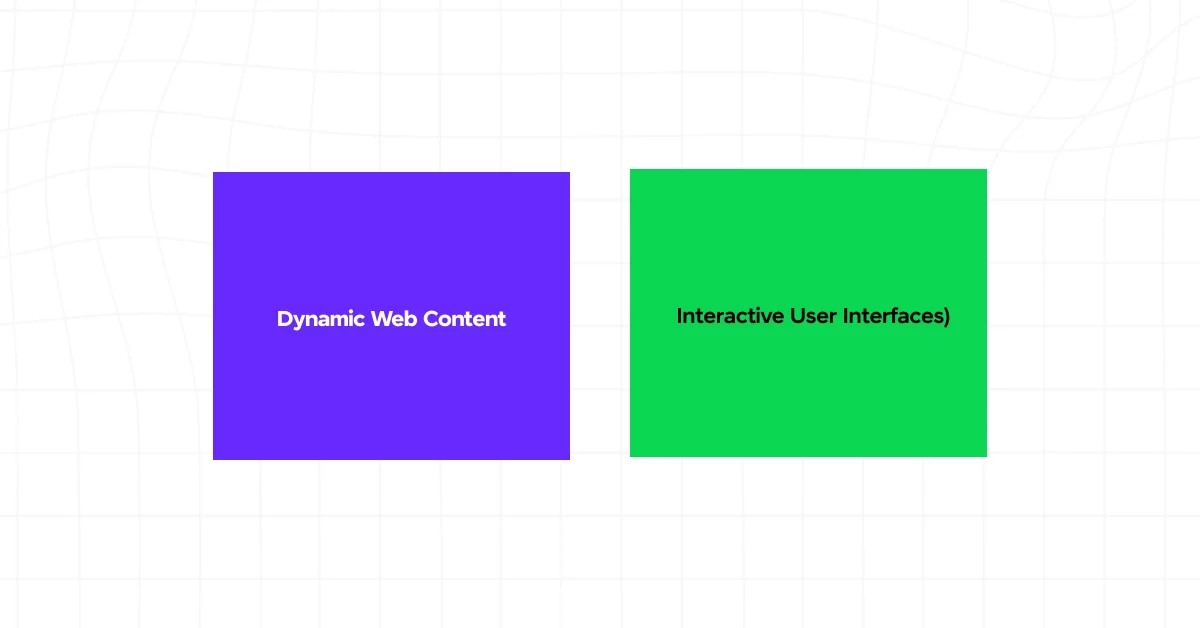
1. Dynamic Web Content
Dynamic web content means your web pages can change on the fly without needing a reload. The DOM lets you tweak HTML and CSS in real time, so your content can react to user actions, server updates, and more.
Here’s what you can do with DOM manipulation:
- Add, remove, or change HTML elements
- Adjust styles and classes
- Update element content
Imagine a live news feed where new articles pop up as they’re published, no page refresh needed. This keeps users engaged and makes for a smoother experience.
Want to dive deeper into DOM tricks? Check out our DOM manipulation guide.
2. Interactive User Interfaces
Modern web apps need snappy, interactive user interfaces (UIs). The DOM is your best buddy here, making your UIs responsive and fun to use. By handling events in the DOM, you can make your app react to user inputs, boosting usability.
Some cool interactive features you can build with the DOM include:
- Form validation and submission
- Dropdown menus and navigation bars
- Modal windows and pop-ups
Event handling in the DOM lets you catch and respond to user actions like clicks, key presses, and mouse movements. You can set up event listeners to trigger specific functions when something happens.
Picture a shopping cart app where items can be added or removed on the spot. With DOM events, you can update the cart in real-time as users interact with it.
Event Type | Description | Example Usage |
---|---|---|
Click | Triggered when an element is clicked | Adding items to a cart |
Keypress | Triggered when a key is pressed | Form input validation |
Mouseover | Triggered when the mouse is over an element | Dropdown menu activation |
Also Explore: Virtual DOM and Shadow DOM: Understanding the Key Differences and Benefits
Common Mistakes to Avoid
Steering clear of common mistakes can make your web apps faster and easier to maintain. Here are some pitfalls to dodge:
- Ignoring Performance: Too many unnecessary DOM manipulations can make your app sluggish. Always keep performance in mind and optimize your code.
- Inline Styles and Event Handlers: Avoid inline styles and event handlers. They clutter your HTML and are harder to maintain. Use external CSS and add event listeners with JavaScript.
- Global Variables for DOM Elements: Don’t use global variables for DOM elements. They can cause conflicts and make debugging a nightmare.
- Overusing Libraries: Libraries can make DOM manipulation easier, but don’t overdo it. Too many libraries can bloat your code. Learn the native DOM API and use libraries wisely.
- Skipping Event Delegation: Not using event delegation can lead to too many event listeners, which hurts performance. Always consider event delegation for handling events on multiple child elements.
Common Mistake | Impact |
---|---|
Ignoring Performance | Sluggish user experience |
Inline Styles and Handlers | Harder maintenance |
Global Variables | Debugging conflicts |
Overusing Libraries | Bloated code |
Ignoring Event Delegation | Degraded performance |
Kickstart your Full Stack Development journey by enrolling in GUVI’s certified Full Stack Development Course with Placement Assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements. Alternatively, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript course.
Wrap Up
By sticking to these best practices and avoiding common mistakes, you’ll get a grip on DOM techniques and boost your web development skills. Now that you know basic details about DOM in web development, implement these and work on some great web development projects.
Did you enjoy this article?