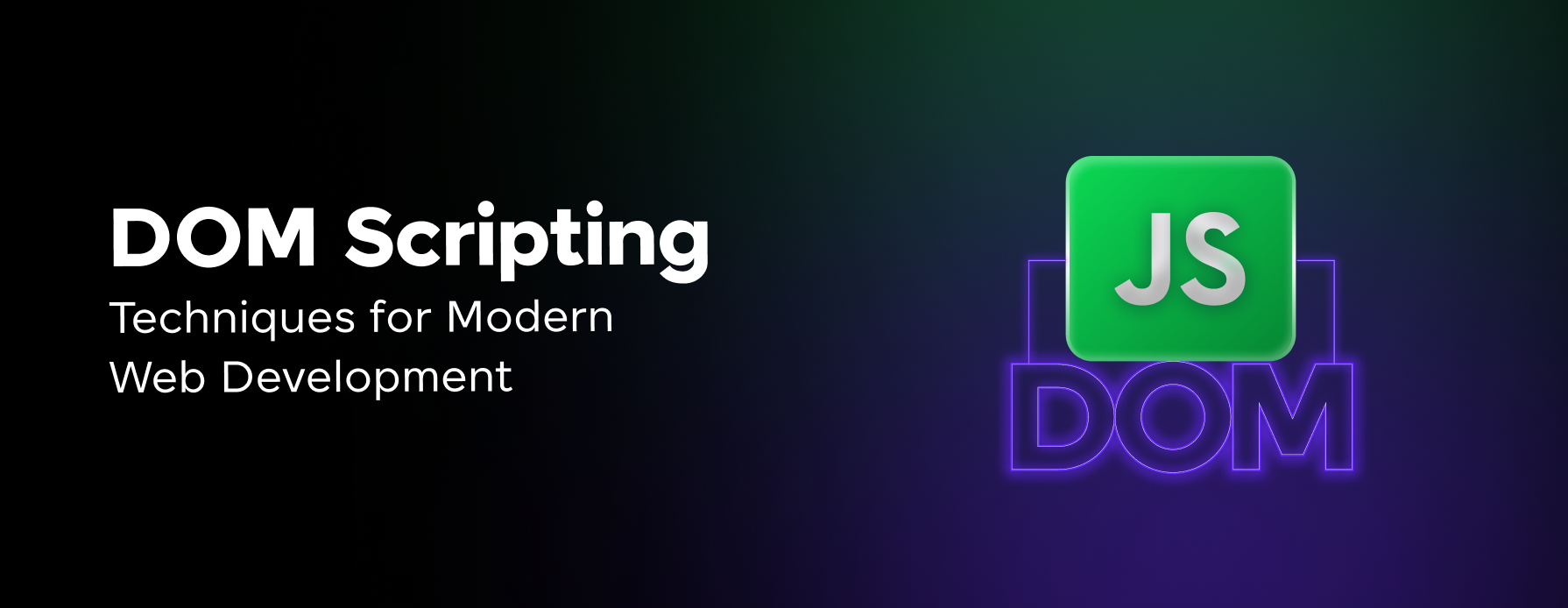
DOM Scripting Techniques for Modern Web Development
Sep 19, 2024 5 Min Read 1518 Views
(Last Updated)
Web development has indeed become one of the top technologies in the IT industry. Also, developers are learning and trying various innovative techniques to make it function effectively. One of the most prominent and vital steps developers take is working on DOM manipulation and DOM Scripting.
In this blog, we’ll be reading about what is DOM Scripting and some of the great DOM Scripting Techniques for Modern Web Development. Let’s learn the key concepts and the tricks you should know for DOM Scripting.
Table of contents
- Why DOM Scripting is a Game-Changer
- Why You Should Care About DOM Scripting
- Key DOM Scripting Concepts
- What is the Document Object Model (DOM)?
- Manipulating DOM Elements Dynamically
- Must-Know DOM Scripting Tricks
- a) Handling Events in DOM Scripting
- b) Navigating and Changing the DOM
- c) Asynchronous JavaScript and the DOM
- Advanced DOM Scripting Tips
- a) Speeding Up Your Site with DOM Tricks
- b) Making Sure Your Code Works Everywhere
- Best Practices for Clean, Fast Code
- Wrapping Up
- FAQs
- What are DOM methods in web technology?
- What is DOM scripting?
- Is DOM important in web development?
- What is the DOM manipulation technique?
Why DOM Scripting is a Game-Changer
DOM scripting is like having a magic wand for web development. The Document Object Model (DOM) lets you create, change, and remove elements from a webpage on the fly. This means your web apps can be super interactive and dynamic, making users go “Wow!” By getting the hang of DOM scripting, you can tweak web pages in real-time, reacting to user actions and updating content without needing a page to reload.
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Course with Placement Assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Additionally, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript certification course.
Feature | Why |
---|---|
Real-Time Updates | Makes users happy with instant changes |
Dynamic Content | Keeps things lively and engaging |
User Interaction | Quick responses to user actions |
Want to dive deeper into the DOM? Check out the document object model guide.
Why You Should Care About DOM Scripting
Getting good at DOM scripting isn’t just about making your web apps look cool. It’s about making them work better and easier to maintain.
- Better User Experience: With DOM scripting, you can update the user interface on the spot, giving users a smooth and responsive experience. This is a must for single-page apps (SPAs).
- Speedy Data Handling: By tweaking DOM elements directly, you cut down on server requests, making your apps faster and more efficient.
- Works Everywhere: Modern DOM scripting ensures your web apps run smoothly across different browsers.
- Easy to Maintain: Clean and well-structured DOM scripts make your code easier to manage and update, saving you time and headaches.
- More Interactive: DOM scripting lets you add fun stuff like forms, pop-ups, and dynamic content updates, making your site more engaging.
Benefit | What It Means |
---|---|
Better User Experience | Instant updates without reloading the page |
Speedy Data Handling | Fewer server requests, faster performance |
Works Everywhere | Consistent behavior across browsers |
Easy to Maintain | Simplifies code updates and management |
More Interactive | Adds fun and engaging elements |
By mastering these techniques, you’ll level up your web development game, making you a pro at building modern, interactive web apps.
Do read: DOM Event Flow: A Comprehensive Guide
Key DOM Scripting Concepts
What is the Document Object Model (DOM)?
The Document Object Model (DOM) is like the backstage pass to your web documents. It’s a programming interface that lets you mess around with the structure, content, and style of your web pages. Think of it as a tree of objects where each branch and leaf represents parts of your HTML or XML document. This tree structure lets you tweak your web pages on the fly, making it a must-know for any web developer.
The DOM starts at the root (the document) and branches out to elements like <html>
, <head>
, and <body>
. Each tag, attribute, and text snippet is a node in this tree.
Here’s a simple HTML document:
<!DOCTYPE html>
<html>
<head>
<title>Sample Document</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a sample paragraph.</p>
</body>
</html>
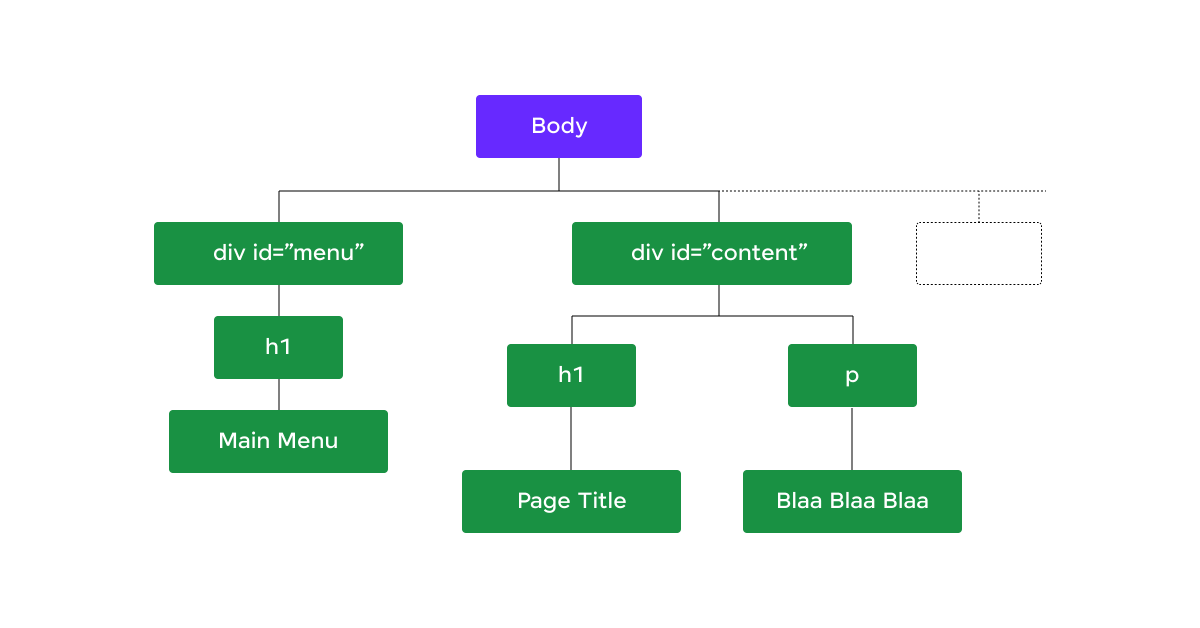
And its DOM tree looks like this:
+ Document
+ html
+ head
+ title
- "Sample Document"
+ body
+ h1
- "Hello, World!"
+ p
- "This is a sample paragraph."
A Comprehensive Guide On Virtual DOM is a must to know when you’re working with DOM Scripting.
Manipulating DOM Elements Dynamically
Playing around with DOM elements lets you create web pages that react and change on the go. You can add, tweak, or remove elements and attributes, change content, and adjust styles based on user actions or other triggers.
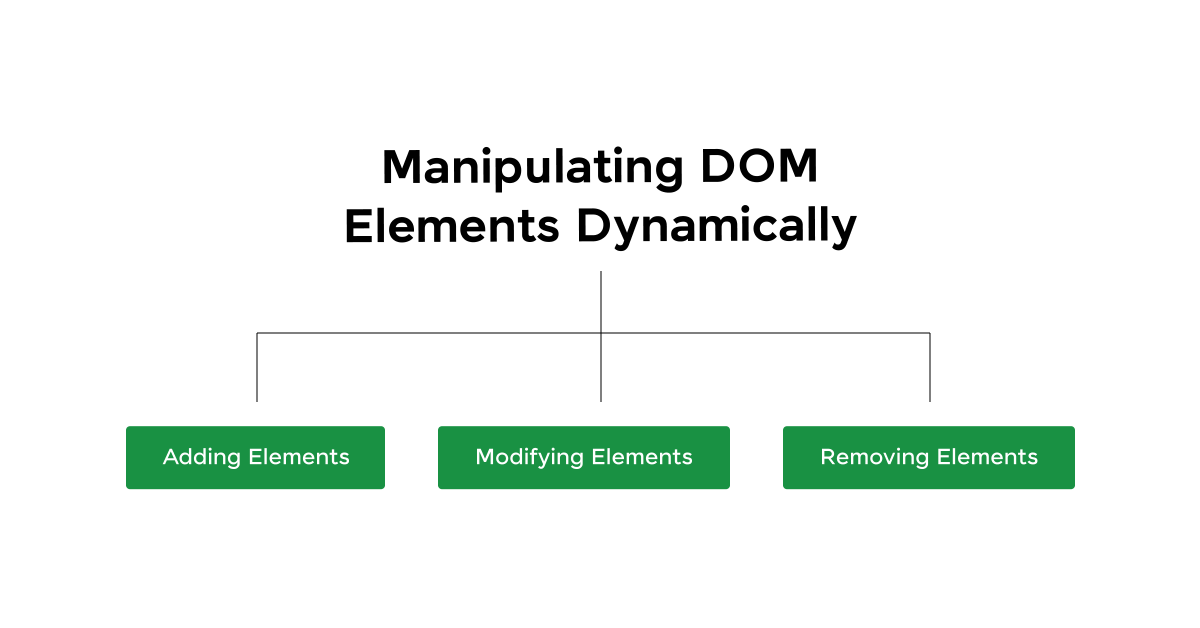
a) Adding Elements
To add new elements, you can use methods like createElement
, appendChild
, and insertBefore
. Here’s how you can add a new paragraph to the body:
// Create a new paragraph element
let newParagraph = document.createElement('p');
// Set the text content of the paragraph
newParagraph.textContent = 'This is a dynamically added paragraph.';
// Append the paragraph to the body
document.body.appendChild(newParagraph);
b) Modifying Elements
To change existing elements, use properties like innerHTML
, textContent
, and style
. Here’s an example of changing the text and style of a heading:
// Select the element to be modified
let heading = document.querySelector('h1');
// Change the text content
heading.textContent = 'Welcome to DOM Scripting!';
// Change the style
heading.style.color = 'blue';
heading.style.fontSize = '24px';
c) Removing Elements
To remove elements, use the removeChild
method. Here’s how to remove a paragraph:
// Select the paragraph to be removed
let paragraphToRemove = document.querySelector('p');
// Remove the paragraph from its parent
paragraphToRemove.parentElement.removeChild(paragraphToRemove);
For more detailed examples and techniques, check out our guide on DOM manipulation in JavaScript.
Mastering these techniques is a game-changer for web development. They let you build responsive, interactive, and user-friendly web apps.
Must-Know DOM Scripting Tricks
Getting the hang of DOM scripting is a game-changer for web development. These tricks help you build interactive and lively web apps.
a) Handling Events in DOM Scripting
Event handling is all about making your website respond to user actions like clicks, key presses, and mouse moves. By attaching event listeners to elements, you can trigger specific functions when these actions happen. Here are some common events:
Event Type | What It Does |
---|---|
click | Fires when an element is clicked |
mouseover | Fires when the mouse hovers over an element |
keydown | Fires when a key is pressed down |
submit | Fires when a form is submitted |
Example of adding an event listener:
document.getElementById('myButton').addEventListener('click', function() {
alert('Button clicked!');
});
Also Know: Event Bubbling in DOM
b) Navigating and Changing the DOM
DOM traversal and manipulation let you move around the DOM tree and change its structure or content. This includes adding, removing, or tweaking elements and attributes. Key methods for DOM traversal include:
Method | What It Does |
---|---|
getElementById() | Selects an element by its ID |
getElementsByClassName() | Selects elements by their class name |
querySelector() | Selects the first element that matches a CSS selector |
querySelectorAll() | Selects all elements that match a CSS selector |
Example of manipulating an element:
let element = document.getElementById('myElement');
element.innerHTML = 'New content';
element.style.color = 'blue';
c) Asynchronous JavaScript and the DOM
Asynchronous JavaScript lets you do tasks without freezing the main thread. This is super handy for things like fetching data from a server. Using methods like fetch
or XMLHttpRequest
, you can update the DOM with the data you get.
Example of fetching data and updating the DOM:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
document.getElementById('dataContainer').innerHTML = data.value;
});
Knowing how to work with asynchronous JavaScript is key for making dynamic and responsive web apps. For more info, visit our article on dom manipulation in jQuery.
By mastering these DOM scripting tricks, you’ll be ready to create web experiences that are both engaging and interactive.
Advanced DOM Scripting Tips
a) Speeding Up Your Site with DOM Tricks
Want your site to load faster and run smoother? Here are some tricks to make your DOM scripting more efficient:
- Cut Down on DOM Access: Grabbing elements from the DOM over and over is like running to the store for every single item on your grocery list. Instead, grab everything you need in one trip and store them in variables.
- Group DOM Changes: Instead of making a bunch of little changes, do them all at once. This way, your browser doesn’t have to keep redrawing the page.
- Use Document Fragments: Adding a bunch of elements? Stick them in a document fragment first, then add the whole fragment to the DOM. This keeps the browser from getting bogged down with too many updates.
Strategy | Why It Works |
---|---|
Cut Down on DOM Access | Saves time and resources |
Group DOM Changes | Reduces the number of redraws |
Use Document Fragments | Minimizes reflows |
b) Making Sure Your Code Works Everywhere
Different browsers can be like different people—what works for one might not work for another. Here’s how to keep everyone happy:
- Check Features, Not Browsers: Instead of asking, “Are you Chrome?” ask, “Can you do this?” This way, you know if a feature is supported.
- Polyfills: Think of polyfills as backup plans for older browsers. They add missing features so everyone gets the same experience.
- Vendor Prefixes: Some CSS properties need a little extra help to work in all browsers. Adding vendor prefixes can make sure your styles look good everywhere.
Technique | Why It Matters |
---|---|
Check Features | Ensures functionality across browsers |
Polyfills | Adds missing features for older browsers |
Vendor Prefixes | Makes CSS work consistently |
Also Read: How to Master DOM in Selenium for Beginners
Best Practices for Clean, Fast Code
Want your code to be easy to read, maintain, and scale? Follow these best practices:
- Keep Things Separate: Your HTML, CSS, and JavaScript should each have their own space. This makes your code easier to read and manage.
- No Inline Scripts: Put your JavaScript in external files. This keeps your HTML clean and your scripts easier to update.
- Event Delegation: Instead of adding event listeners to a bunch of elements, add one to their common parent. This saves memory and speeds things up.
Best Practice | Why It’s Good |
---|---|
Keep Things Separate | Makes code easier to read and manage |
No Inline Scripts | Keeps HTML clean, scripts easy to update |
Event Delegation | Saves memory, speeds up performance |
Kickstart your Full Stack Development journey by enrolling in GUVI’s certified Full Stack Development Course with Placement Assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements. Alternatively, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript course.
Wrapping Up
Now that you know all about DOM Scripting Techniques, you’re all ready to explore more on web development and get yourself one more step ahead in this web development journey. You’ll not just learn the DOM Scripting Techniques, but also some of the best practices you must follow to build scalable, robust, and flexible web applications.
FAQs
What are DOM methods in web technology?
DOM (document object model) represents a document with a logical tree, where each branch of the tree ends in a node, and each node contains objects. Using DOM, you can change the document’s structure, style, or content.
What is DOM scripting?
DOM Scripting is using JavaScript to manipulate the DOM inside a web page, either during page load or in response to user events, like clicking a button or selecting text.
Is DOM important in web development?
Yes, DOM is an important element in web development where JavaScript accesses elements and performs manipulation of the HTML element.
Without DOM, JavaScript won’t be able to do it.
What is the DOM manipulation technique?
JavaScript DOM manipulation allows you to modify the website content, alter the structure, and style directly with JavaScript.
Did you enjoy this article?