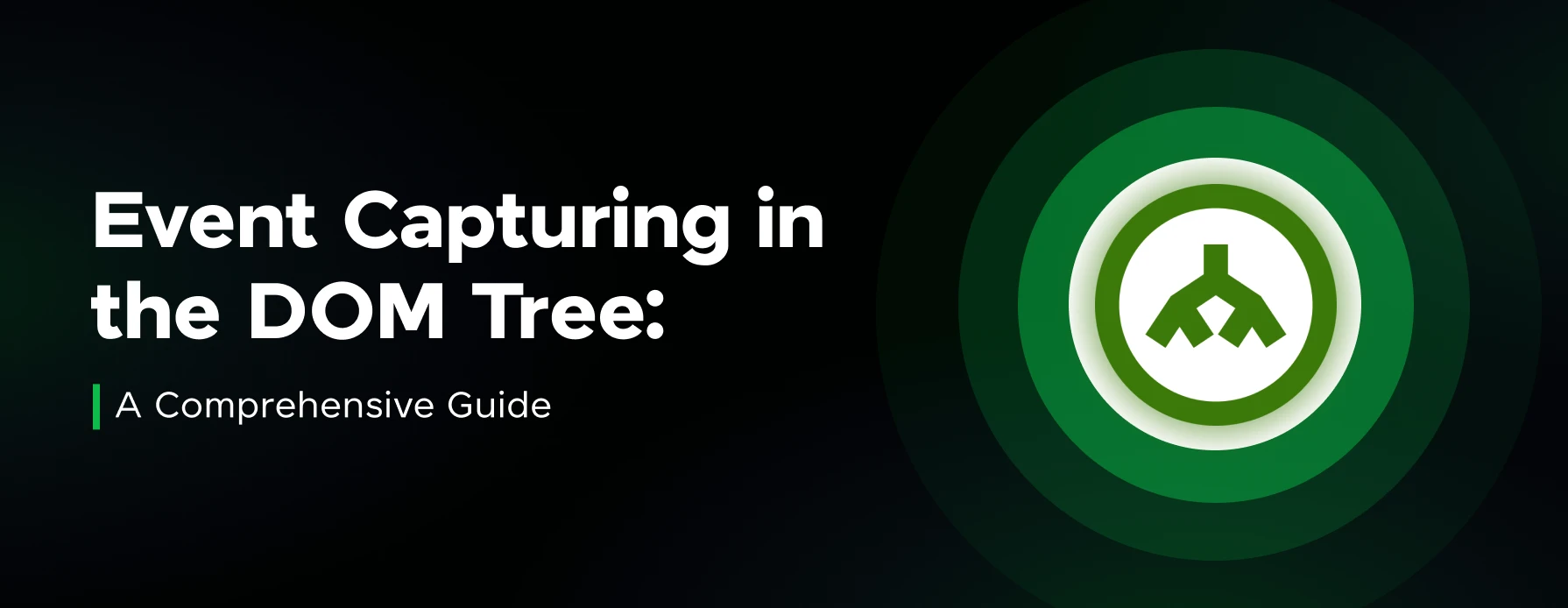
Event Capturing in the DOM Tree: A Comprehensive Guide
Sep 19, 2024 5 Min Read 1504 Views
(Last Updated)
In web development, understanding how events propagate through the Document Object Model (DOM) is important for creating dynamic and responsive user interfaces. When a user interacts with a webpage—whether by clicking a button, typing into a form, or hovering over an image—these interactions trigger events that travel through the DOM in a specific order.
This blog post focuses on the first phase of event propagation: event capturing. Event capturing, also known as the capturing phase, is a less commonly discussed aspect of event handling compared to event bubbling. However, mastering event capturing can provide web developers with a powerful tool for controlling event flow, especially in complex web applications.
In this blog, we will explore event capturing. By the end of this post, you’ll have a solid understanding of how to use event capturing to enhance your web development projects, ensuring a more controlled and efficient user experience.
Table of contents
- What is Event Flow in the DOM?
- Event Capturing in the DOM Tree
- What is Event Capturing?
- How Event Capturing Works
- Use Cases for Event Capturing
- Event Capturing vs. Event Bubbling
- Combining Event Capturing with Event Bubbling
- Common Pitfalls and Best Practices
- Advanced Topics
- Conclusion
- FAQs
- What is the difference between event capturing and event bubbling?
- How do I enable event capturing for an event listener in JavaScript?
- When should I use event capturing instead of event bubbling?
What is Event Flow in the DOM?
Event Flow in the DOM (Document Object Model) refers to the order in which events are propagated or flow through the HTML elements in a web page. When an event occurs, such as a user clicking a button or hovering over an element, the event needs to be transmitted to the appropriate target element and any other elements that may be interested in receiving that event.
The event flow in the DOM follows a specific path, which can be categorized into two main phases:
- Capturing Phase: In this phase, the event starts at the top-level element (typically the window object) and travels down through the ancestor elements of the target element. During this phase, any event handlers registered in the capturing phase are triggered in reverse order, from the top-level element to the target element’s parent.
- Bubbling Phase: After the capturing phase, the event reaches the target element itself, and any event handlers registered directly on the target element are triggered. Then, the event bubbles up through the ancestor elements, triggering any event handlers registered in the bubbling phase, from the target element’s parent up to the top-level element.
Most events in the DOM follow the bubbling phase, which means that if an event handler is not explicitly set to capture the event, it will be triggered during the bubbling phase. However, some events, like the focus event, do not bubble at all.
Event handlers can be registered to listen for events during either the capturing phase or the bubbling phase, depending on the desired behavior. By default, event handlers are registered for the bubbling phase.
Understanding the event flow in the DOM is crucial for managing event propagation, preventing unwanted event handling, and ensuring that events are handled correctly in complex web applications.
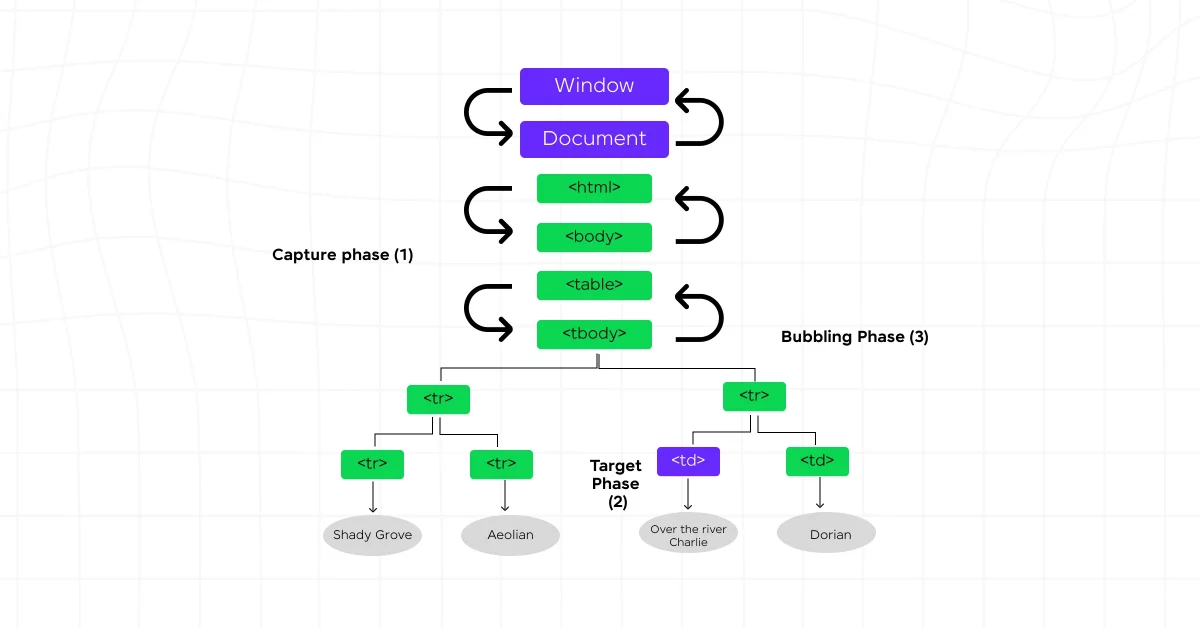
Also Explore: jQuery DOM Manipulation: Learn Everything About This On-demand Technique
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Course with Placement Assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Additionally, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript certification course.
Event Capturing in the DOM Tree
In the world of web development, understanding the behavior of events and how they propagate through the Document Object Model (DOM) is crucial for building interactive and responsive applications. While most developers are familiar with the concept of event bubbling, event capturing is often overlooked or misunderstood.
In this comprehensive blog post, we’ll dive deep into the intricacies of event capturing, explore its workings, use cases, and how it relates to event bubbling.
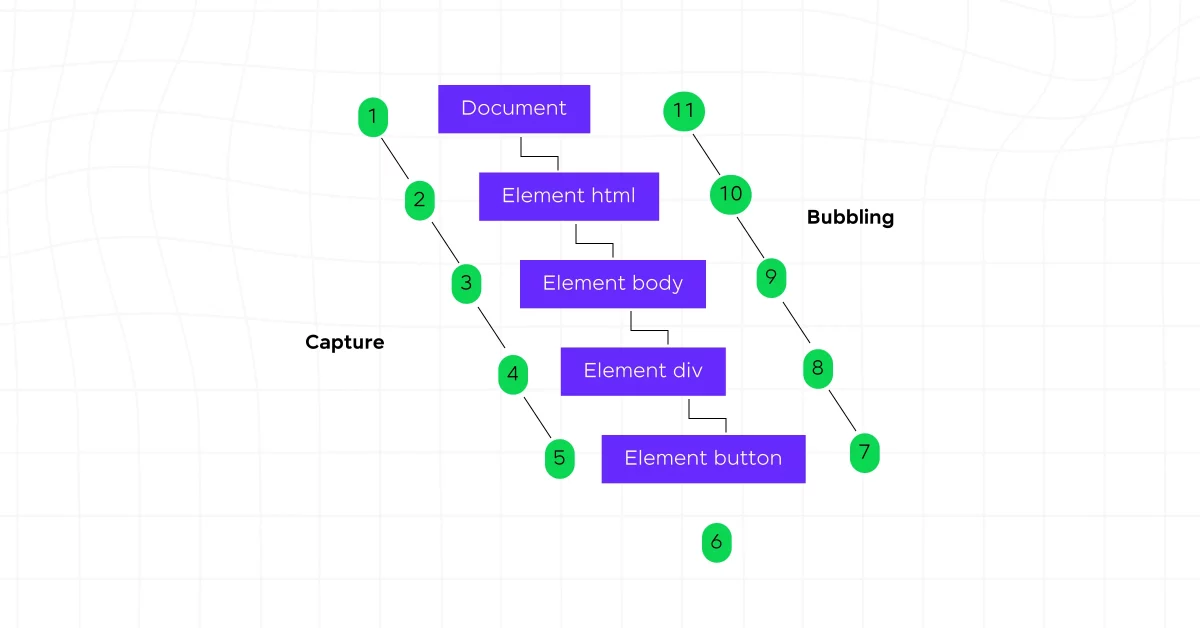
1. What is Event Capturing?
Event capturing is one of the two phases that occur during event propagation in the DOM tree. It is the first phase, where an event starts at the top of the DOM tree (typically the window object) and travels down through the ancestor elements of the target element until it reaches the target itself.
During the event-capturing phase, any event handlers registered to capture events will be triggered in reverse order, from the top-level element to the target element’s parent. This is in contrast to the more commonly used event bubbling phase, where event handlers are triggered from the target element up to the top-level element.
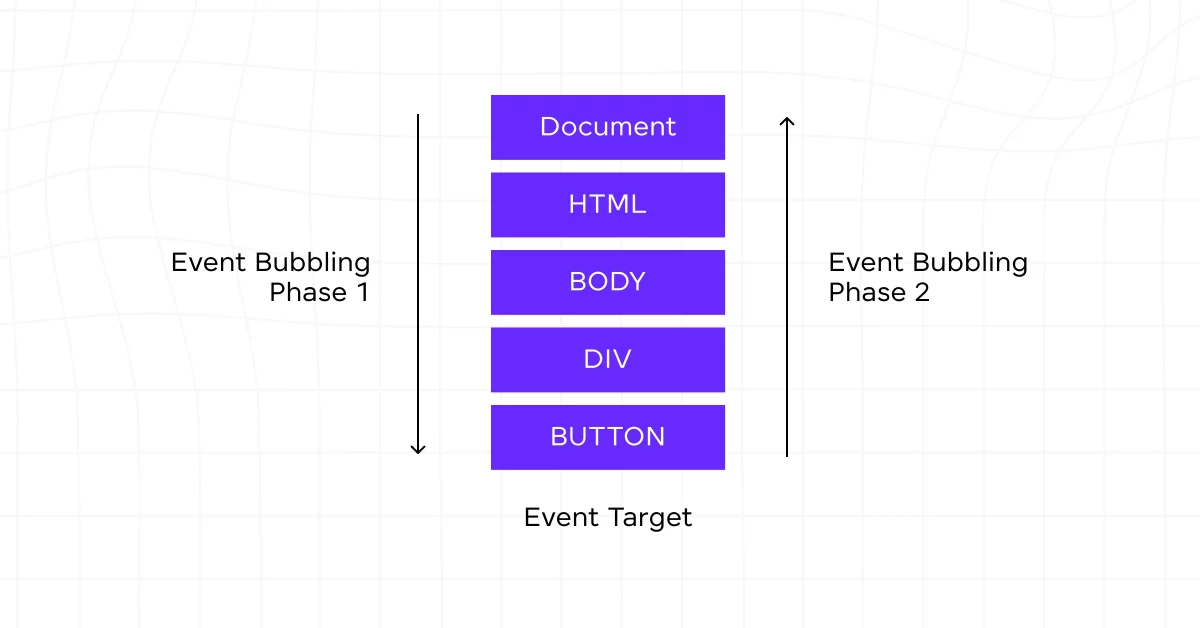
Also Read: What are Events in JavaScript? A Complete Guide
2. How Event Capturing Works
To better understand event capturing, let’s consider a simple example. Imagine a web page with a nested structure of HTML elements, such as a button inside a div inside the body:
<body>
<div>
<button>Click me</button>
</div>
</body>
When a user clicks the button, the event-capturing phase follows this order:
- The event starts at the window object.
- The event travels to the document object.
- The event travels to the <html> element.
- The event travels to the <body> element.
- The event travels to the <div> element.
- The event finally reaches the <button> element (the target element).
At each step, if any event handlers are registered to capture the event, they will be executed in the order specified above. It’s important to note that the event-capturing phase occurs before the event-bubbling phase, which follows a reverse order, starting from the target element and going up to the top-level element.
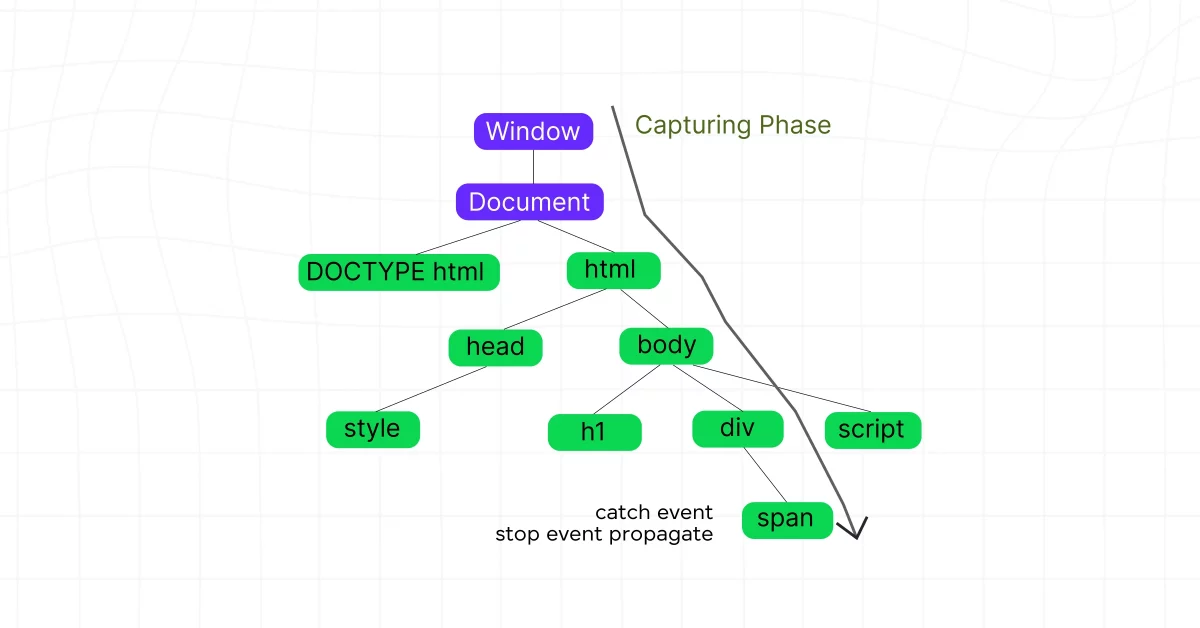
Also Read: What is DOM manipulation? Common Tasks and Elements
3. Use Cases for Event Capturing
While event capturing is not as widely used as event bubbling, there are certain scenarios where it can be beneficial:
- Event Delegation: Event capturing can be useful for event delegation, where a single event handler is attached to a parent element to handle events from its child elements. This can help optimize performance and reduce memory usage, especially in scenarios with dynamically generated or updated content.
- Event Interception: By capturing events at a higher level in the DOM tree, you can intercept and handle events before they reach the target element. This can be useful for implementing custom event handling logic, such as preventing certain events from reaching the target or modifying the event object before it is propagated.
- Accessibility and User Experience: Event capturing can be leveraged to enhance accessibility and user experience. For example, you could capture events at a higher level to provide custom keyboard navigation or implement focus management techniques.
Also Read: AJAX with jQuery: A Comprehensive Guide for Dynamic Web Development
4. Event Capturing vs. Event Bubbling
Event bubbling is the more commonly used and understood phase of event propagation. During the bubbling phase, an event starts at the target element and travels up through its ancestor elements until it reaches the top-level element (window). Event handlers registered for the bubbling phase are triggered in this order, from the target element to the top-level element.
While both phases serve different purposes, event bubbling is generally preferred for most use cases due to its natural flow and ease of understanding. However, event capturing can be a powerful tool in specific scenarios where you need to intercept or handle events at a higher level in the DOM tree.
5. Combining Event Capturing with Event Bubbling
It’s important to note that event capturing and event bubbling are not mutually exclusive. In fact, you can combine them to achieve the desired event-handling behavior. When an event occurs, it first goes through the capturing phase, then the event bubbling phase.
This allows you to register event handlers for both phases, providing flexibility in how you handle events. For example, you could capture an event at a higher level to perform some initial processing, then let the event bubble down to the target element for additional handling.
6. Common Pitfalls and Best Practices
While event capturing can be a powerful tool, it’s essential to be aware of potential pitfalls and follow best practices to ensure your code is maintainable and efficient:
- Performance Considerations: Event capturing can potentially impact performance, especially if you have many event handlers registered at higher levels in the DOM tree. It’s crucial to optimize your code and use event capturing judiciously.
- Readability and Maintainability: Event capturing can make your code more complex and harder to reason about, especially if you’re combining it with event bubbling. Always strive for clear and well-documented code to ensure long-term maintainability.
- Browser Compatibility: While modern browsers support event capturing, it’s essential to consider browser compatibility when using advanced event handling techniques, especially for older browser versions or less common browsers.
- Event Order and Precedence: When combining event capturing and event bubbling, it’s important to understand the order in which event handlers are executed and how to manage event precedence to achieve the desired behavior.
- Event Delegation: While event capturing can be used for event delegation, event bubbling is often preferred for its simplicity and efficiency. Carefully evaluate the trade-offs before deciding on the appropriate approach.
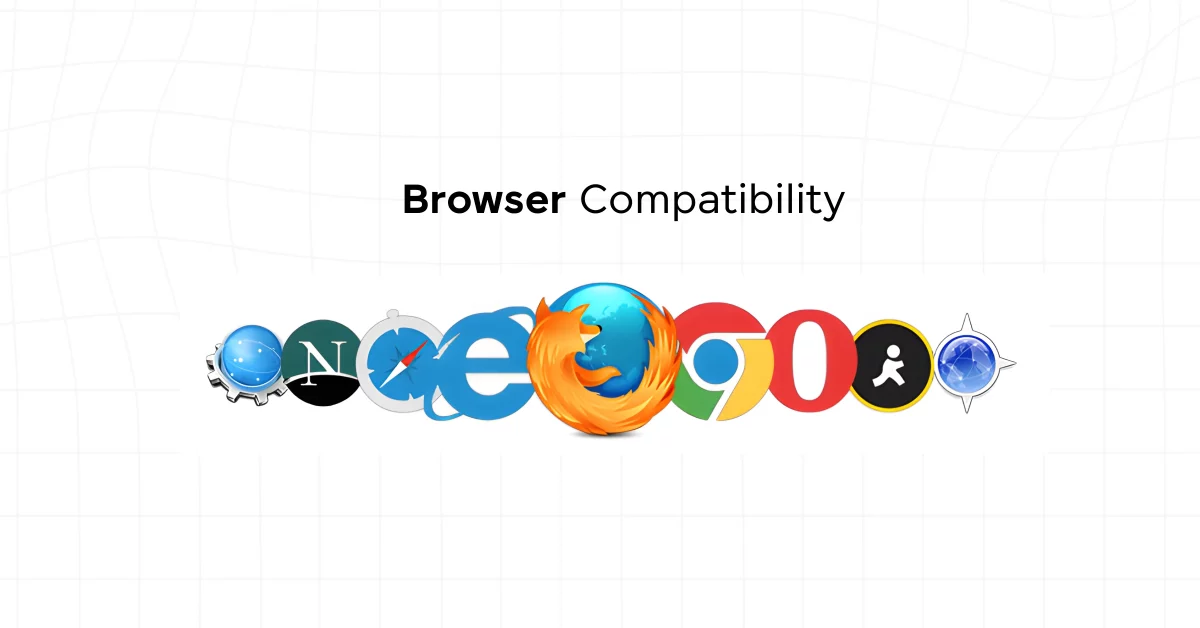
Also Read: What is jQuery? A Key Concept You Should Know
7. Advanced Topics
As you delve deeper into event capturing and event handling in the DOM, you may encounter more advanced topics and techniques:
- Shadow DOM and Event Capturing: With the introduction of the Shadow DOM, event-capturing behavior can become more complex. Understanding how events propagate within and across shadow boundaries is crucial for building robust web components.
- Custom Events and Event Capturing: JavaScript allows you to create and dispatch custom events, which can also be captured or bubbled. Understanding the propagation of custom events is essential for building modular and extensible applications.
- Accessibility and Event Capturing: Event capturing can play a vital role in enhancing accessibility features, such as keyboard navigation, focus management, and assistive technology integration. Exploring accessibility best practices and guidelines can help you build more inclusive and user-friendly applications.
- Performance Optimization and Event Capturing: While event capturing can impact performance, there are techniques and strategies you can employ to optimize event handling, such as leveraging event delegation, debouncing, and throttling.
Event capturing is a powerful and often overlooked aspect of event handling in the DOM. While it may not be as commonly used as event bubbling, understanding event capturing can open up new possibilities for building robust and advanced web applications.
By mastering event capturing, you’ll have a deeper understanding of event propagation and be better equipped to tackle complex event-handling scenarios.
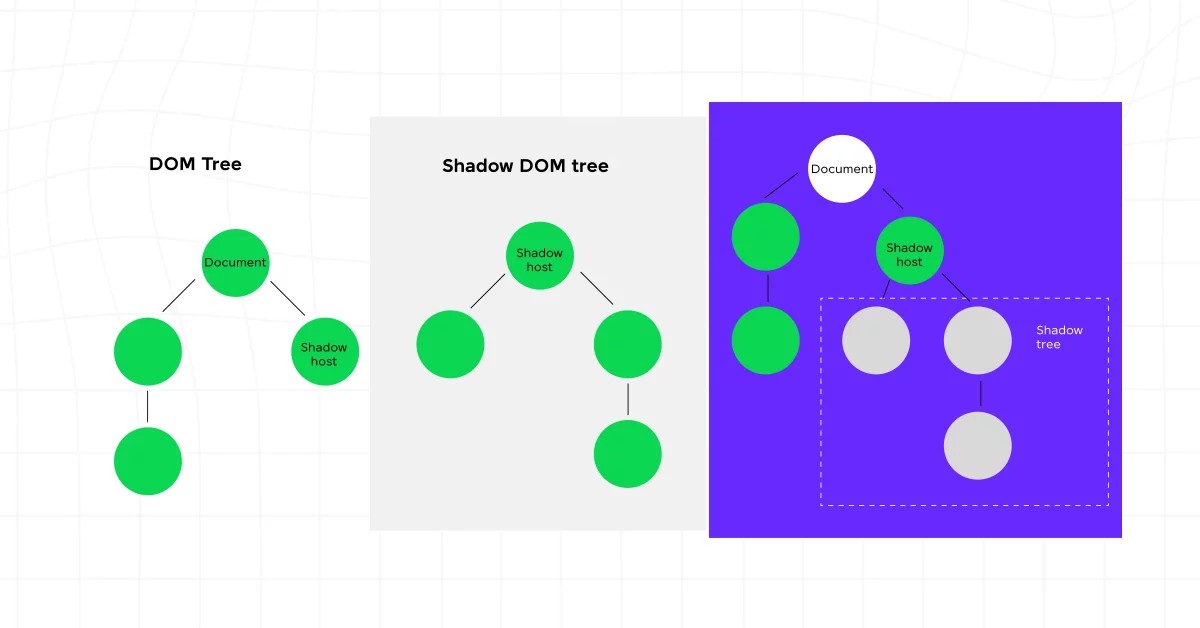
Kickstart your Full Stack Development journey by enrolling in GUVI’s certified Full Stack Development Course with Placement Assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements. Alternatively, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript course.
Conclusion
Mastering event capturing not only gives you more control over event handling but also allows you to create more efficient and predictable interactions in your web applications. By understanding when and how to use event capturing, you can ensure that your event handlers are executed in the desired order, leading to more maintainable and robust code.
Experiment with event capturing in your projects. Try implementing it in various contexts to see how it affects your event handling and overall application behavior. As you become more comfortable with this concept, you’ll find that it opens up new possibilities for managing events in innovative and effective ways.
Also Explore: A Comprehensive Guide On Virtual DOM: Learn Everything About It
FAQs
What is the difference between event capturing and event bubbling?
Event capturing, the first phase of event propagation, starts from the root and travels to the target element, allowing parent elements to handle events first. Event bubbling, the second phase, travels from the target element back up to the root, letting child elements handle events before their parents.
How do I enable event capturing for an event listener in JavaScript?
To enable event capturing, set the useCapture parameter to true when adding the event listener. For example: document.addEventListener(‘click’, function(event) { console.log(‘Capturing phase:’, event.target); }, true);. This ensures the event listener triggers during the capturing phase.
When should I use event capturing instead of event bubbling?
Use event capturing in scenarios such as handling events in nested elements, preventing unwanted propagation, and managing complex user interfaces. While event bubbling is more common, capturing provides better control over event flow in these cases, leading to more maintainable applications.
Did you enjoy this article?