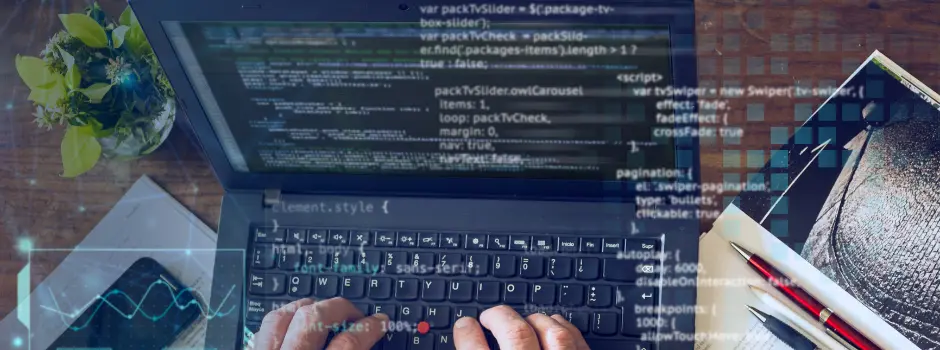
Event Delegation and Bubbling in JavaScript
Dec 31, 2024 3 Min Read 761 Views
(Last Updated)
Event delegation leverages bubbling by allowing you to attach a single event listener to a parent element rather than individual child elements. This technique is especially beneficial when dealing with dynamic content or a large number of similar elements. By using event delegation, you can reduce memory usage, simplify your code, and improve performance by avoiding repetitive event listener attachments.
In this blog, we’ll explore Event Delegation and Bubbling in JavaScript. We will learn how event bubbling works, discuss the principles of event delegation, and provide examples to demonstrate their practical application. Whether you’re new to JavaScript or looking to refine your skills, mastering these concepts is essential for building efficient and interactive web applications.
Table of contents
- Event Delegation
- Advantages of Event Delegation
- Disadvantages of Event Delegation
- Event Bubbling
- Conclusion
Event Delegation
JavaScript’s Event Delegation approach lets you manage events more effectively by having one event listener assigned to a parent element rather than having separate listeners attached to each of the child components. When working with a large number of elements or elements that are generated dynamically, this is quite helpful.
Event delegation makes use of the DOM’s event propagation— especially, event bubbling. An event on an element can be captured at a higher level in the DOM hierarchy since it bubbles up through its ancestors, such as parents and grandparents.
Example:
<ul id=”parentList”> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> <script> document.getElementById(‘parentList’) .addEventListener(‘click’, function (event) { if (event.target.tagName === ‘LI’) { alert(‘You clicked on ‘ + event.target.textContent); } }); </script> |
- You add a click event listener to the <ul> element (parent), rather than to each <li> element.
- An event is bubbled up to the <ul>, where it is processed and caught when a list item (<li>) is clicked.
Advantages of Event Delegation
- Improved Performance:
- Reduced Memory Usage: A parent element has a single event listener linked to it rather than several listeners attached to each of the child components. Memory is saved in this way, particularly when handling a lot of child items.
- Efficient DOM Management: Web pages with several interactive elements can run much better when there are fewer event listeners in the DOM thanks to event delegation.
- Handles Dynamic Content: You don’t have to manually attach event listeners to newly added child components when they are introduced dynamically (for example, by appending content using JavaScript). Events from these additional elements will be automatically captured by the parent’s event listener.
- Consistent Behavior Across Browsers: By utilizing the common event propagation mechanism, event delegation makes sure that your event-handling code functions properly in all modern browsers.
Disadvantages of Event Delegation
- Potential for Unintended Event Handling:
- Capturing Unwanted Events: You may need to add further logic to filter out undesirable event targets if the parent element has multiple child elements that aren’t meant to handle events. This may make things more complicated and maybe introduce bugs.
- Incorrect Targeting: You may need to inspect the event.target and take appropriate action if a child element that isn’t relevant triggers the event, which will add overhead to your function.
- Event Propagation Issues:
- Event Bubbling Complexity: Because event delegation depends on bubbling, problems may arise if the same event is being handled by other event listeners higher up in the DOM hierarchy. To avoid conflicts, you might need to utilize event.stopPropagation() or extra logic, which would need more complex event management.
- Delayed Event Handling: When opposed to connecting event listeners directly to child elements, there may occasionally be a tiny delay because the event bubbles up to the parent before being processed.
- Not Suitable for All Event Types:
- Non-Bubbling Events: Certain events—such as blur, focus, mouseleave, and mouseenter —don’t bubble, which means a parent element cannot assign them. You still need to attach event listeners to the elements directly in these circumstances.
- Low-Frequency Events: Events that happen occasionally, like a single button click or form submission, may not gain much from event delegation in terms of efficiency and may even add needless complexity.
Event Bubbling
The process known as Event Bubbling occurs when an event that starts on a child element starts to bubble up to its parent elements and so on until it reaches the root element (document). It is JavaScript’s default method of event propagation. Event propagation refers to the way events move through the DOM when an event is fired.
Event propagation has three phases:
- Capturing phase (Trickling): Beginning in the window, the event proceeds to the target element via the DOM tree.
- Target phase: The event reaches the target element where it was originally initiated.
- Bubbling phase: After reaching the target, the event bubbles up from the target element to the window and propagates across all ancestor elements.
In JavaScript, events propagate by default during the bubbling phase; however, you can alternatively decide to handle events during the capturing phase.
Example:
<div id=“outer”> <div id=“inner”> <button id=“btn”>Click Me</button> </div> </div> <script> document.getElementById(‘outer’).addEventListener(‘click’, function () { console.log(‘Outer div clicked’); }); document.getElementById(‘inner’).addEventListener(‘click’, function () { console.log(‘Inner div clicked’); }); document.getElementById(‘btn’).addEventListener(‘click’, function () { console.log(‘Button clicked’); }); </script> |
If you click the button, you’ll see the following in the console:
Button clicked Inner div clicked Outer div clicked |
This is because the event bubbles from the button to its parent (#inner) and grandparent (#outer) elements. You can stop event bubbling using event.stopPropagation(). This prevents the event from propagating to ancestor elements.
document.getElementById(‘btn’).addEventListener(‘click’, function (event) { event.stopPropagation(); // Stop the event from bubbling console.log(‘Button clicked’); }); |
Under this circumstance, the event won’t bubble up to the parent components; instead, only “Button clicked” will show up in the console.
Conclusion
Event delegation and bubbling are indispensable concepts for writing efficient JavaScript code. By understanding event bubbling, you can grasp how events propagate through the DOM hierarchy, enabling advanced interaction handling.
Did you enjoy this article?