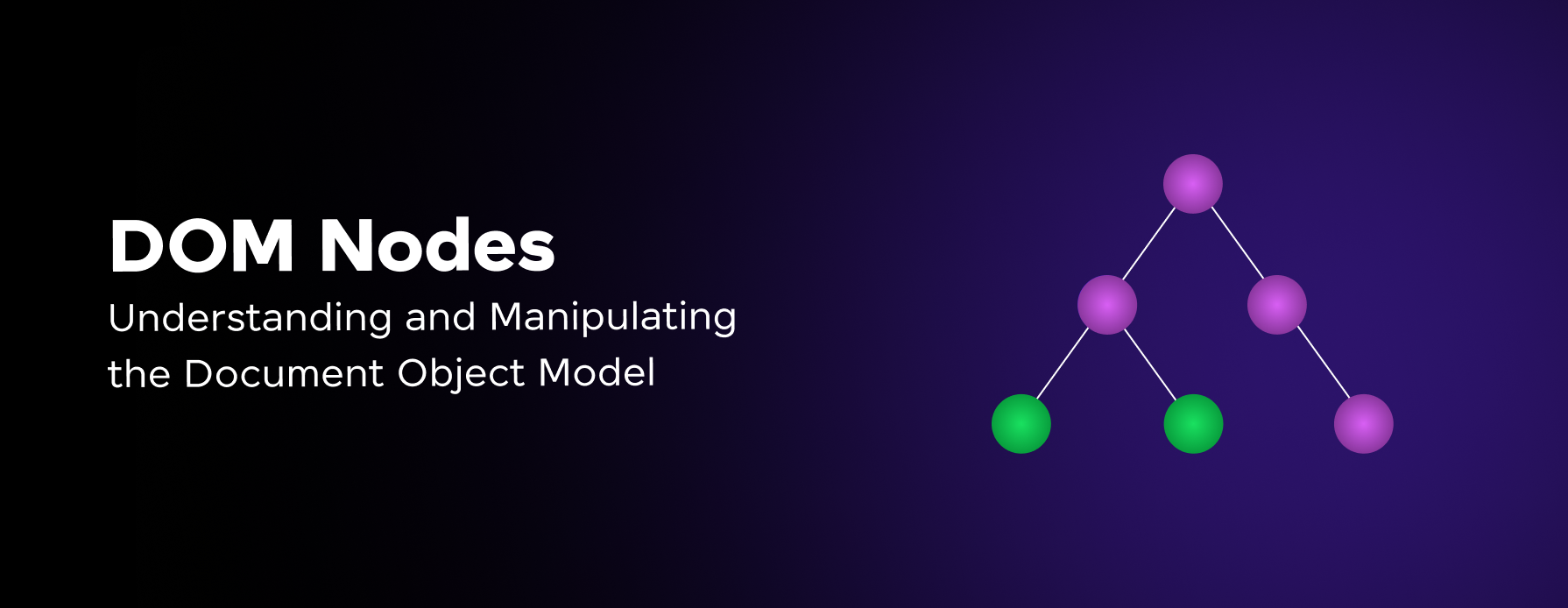
DOM Nodes: Understanding and Manipulating the Document Object Model
Sep 19, 2024 7 Min Read 1930 Views
(Last Updated)
In web development, the Document Object Model (DOM) serves as the foundation upon which interactive and dynamic web pages are built. At its core are DOM nodes, essential building blocks that represent elements, text, comments, and more within an HTML document. Understanding DOM nodes is fundamental to manipulating and interacting with web content programmatically.
In this blog, we will explore DOM nodes, from their types and properties to traversal and manipulation. Learning DOM nodes will help you to create more responsive, efficient, and interactive web applications. By the end of this blog, you’ll be equipped with the knowledge to navigate the DOM with confidence to enhance user experiences and streamline development processes. Let’s begin!
Table of contents
- What are DOM Nodes?
- DOM Nodes: A Comprehensive Guide
- Different Types of DOM Nodes
- Traversing the DOM
- Manipulating DOM Nodes
- Node Properties and Methods
- Practical Applications of DOM Nodes
- Best Practices for Working with DOM Nodes
- Conclusion
- FAQs
- What are DOM nodes?
- How do you access DOM nodes using JavaScript?
- What are some common operations on DOM nodes?
What are DOM Nodes?
In the Document Object Model (DOM), nodes are the fundamental building blocks that represent different parts of a web document. A node can be an element, attribute, text, comment, or even the document itself. Understanding the concept of DOM nodes is crucial for manipulating and traversing the structure of a web page using JavaScript.
Here are the different types of nodes in the DOM:
- Document Node: This is the root node of the entire document, representing the web page itself. It serves as the entry point for accessing all other nodes.
- Element Node: These nodes represent HTML elements, such as <div>, <p>, <a>, and so on. They form the structure of the document and can contain child nodes (including other element nodes, text nodes, or attribute nodes).
- Attribute Node: These nodes represent the attributes of an HTML element, such as id, class, src, or any custom attribute. Attribute nodes are considered child nodes of the element node they belong to.
- Text Node: These nodes represent the textual content within an element. Any visible text in a web document is represented as a text node.
- Comment Node: These nodes represent HTML comments within the document. While they are part of the DOM, they are generally ignored by browsers and are mainly used for documentation or debugging purposes.
- Document Type Node: This node represents the Document Type Definition (DTD) of the document, which specifies the rules and syntax for the markup language being used (e.g., HTML or XML).
Each node in the DOM has properties and methods that allow you to access and manipulate its content, structure, and behavior. For example, you can retrieve the text content of a text node, modify the attributes of an element node, or traverse the parent-child relationship between nodes.
Working with DOM nodes is essential for tasks such as creating, modifying, or removing elements dynamically, handling user events, and manipulating the content and structure of a web page. JavaScript provides various methods and properties to interact with DOM nodes, such as getElementById(), getElementsByTagName(), createElement(), appendChild(), and many more.
Also Explore: How To Boost DOM Rendering Performance Like a Pro
Here’s a simple example that demonstrates how to access and manipulate DOM nodes using JavaScript:
// Get an element node by its ID
const heading = document.getElementById('myHeading');
// Access the text content of a text node
console.log(heading.firstChild.nodeValue); // Output: Existing text
// Create a new text node
const newText = document.createTextNode('New Text');
// Replace the existing text node with the new one
heading.replaceChild(newText, heading.firstChild);
In this example, we first retrieve an element node (<h1 id=”myHeading”>Existing text</h1>) using getElementById(). We then access the text content of the text node (child node of the element node) using firstChild.nodeValue. Next, we create a new text node with the string “New Text” and replace the existing text node with the new one using replaceChild().
Understanding DOM nodes is a fundamental concept in web development, as it allows you to dynamically manipulate and interact with the structure and content of web pages, enabling the creation of rich and interactive user experiences.
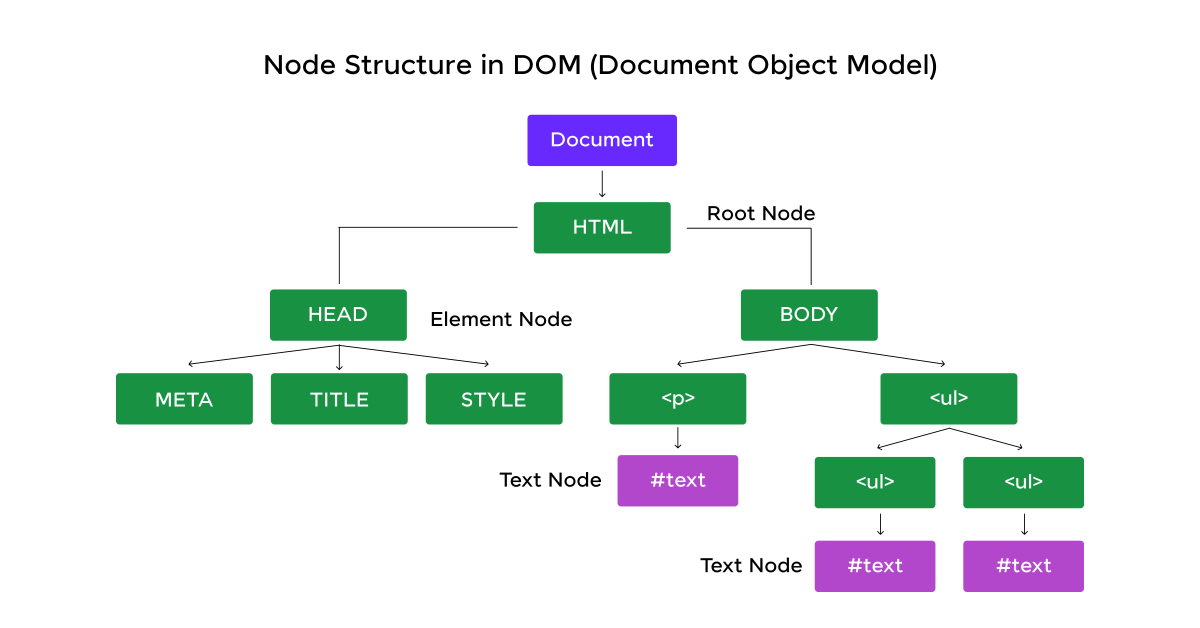
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Course with Placement Assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Additionally, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript certification course.
DOM Nodes: A Comprehensive Guide
In web development, the Document Object Model (DOM) plays a crucial role in representing and manipulating the structure and content of web pages. At the heart of the DOM lies the concept of nodes, which are the fundamental building blocks that make up the entire document tree. Understanding DOM nodes is essential for creating dynamic and interactive web experiences.
Different Types of DOM Nodes
The DOM specification defines several types of nodes, each serving a specific purpose in representing the various elements and components of a web document. Let’s explore the different node types:
a. Element Node: These nodes represent HTML elements, such as <div>, <p>, <a>, and so on. They form the backbone of the document structure and can contain child nodes, including other element nodes, text nodes, or attribute nodes.
b. Text Node: Text nodes represent the textual content within an element. Any visible text in a web document is encapsulated within a text node, which is a child node of its parent element node.
c. Attribute Node: These nodes represent the attributes of an HTML element, such as id, class, src, or any custom attribute. Attribute nodes are considered child nodes of the element node they belong to.
d. Comment Node: Comment nodes represent HTML comments within the document. While they are part of the DOM, they are generally ignored by browsers and are mainly used for documentation or debugging purposes.
e. Document Node: This is the root node of the entire document, representing the web page itself. It serves as the entry point for accessing all other nodes.
f. Document Type Node: This node represents the Document Type Definition (DTD) of the document, which specifies the rules and syntax for the markup language being used (e.g., HTML or XML).
g. Document Fragment Node: A document fragment is a lightweight object that serves as a temporary container for nodes. It is useful when performing complex DOM manipulations, as it allows you to create and modify a subtree of nodes without affecting the live document.
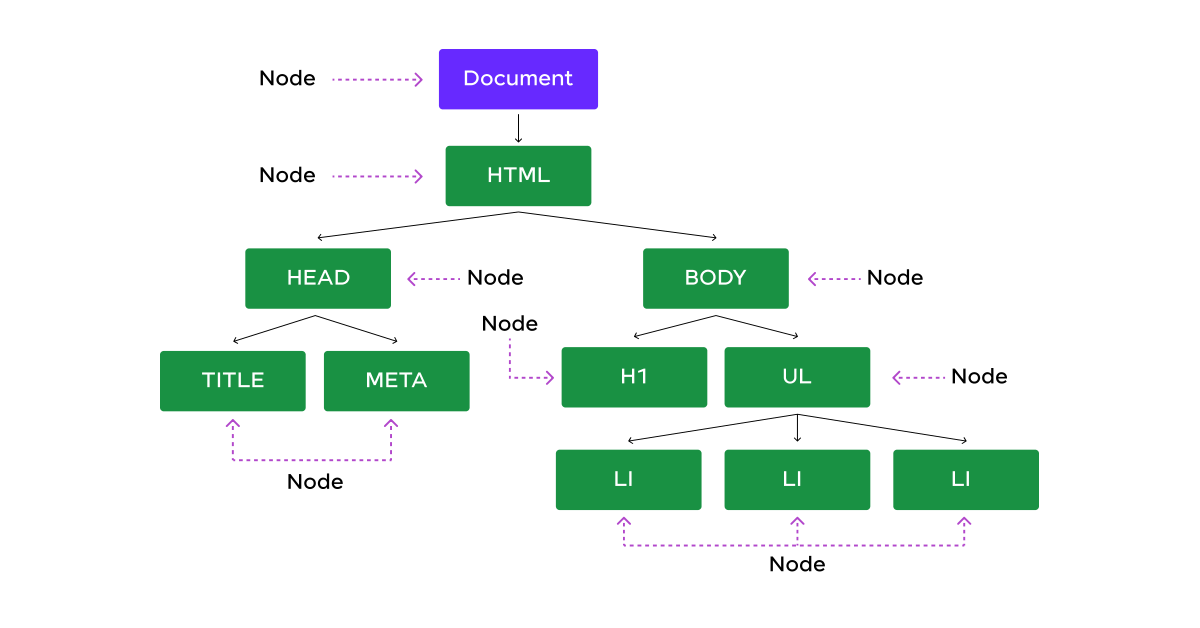
Also Read: How to Master DOM in Selenium for Beginners
Traversing the DOM
Once you understand the different types of nodes, the next step is learning how to navigate and traverse the DOM tree. The DOM provides several properties and methods that allow you to access and manipulate nodes in relation to their parent, child, and sibling nodes.
a. Accessing Parent Nodes: You can access the parent node of a given node using the parentNode property or the parentElement property (for element nodes).
b. Accessing Child Nodes: To access the child nodes of a given node, you can use the childNodes property (returns a live NodeList of all child nodes, including text and comment nodes) or the children property (returns an HTMLCollection of only the element child nodes).
c. Accessing Sibling Nodes: The nextSibling and previousSibling properties allow you to access the next and previous sibling nodes, respectively, at the same level in the DOM tree. For element nodes, you can use nextElementSibling and previousElementSibling to skip over text and comment nodes.
d. Accessing the First and Last Child: The firstChild and lastChild properties provide direct access to the first and last child nodes of a given node, respectively.
e. DOM Traversal Methods: In addition to the properties mentioned above, the DOM also provides methods like getElementsByTagName(), getElementsByClassName(), and querySelector()/querySelectorAll() to traverse and select nodes based on specific criteria.
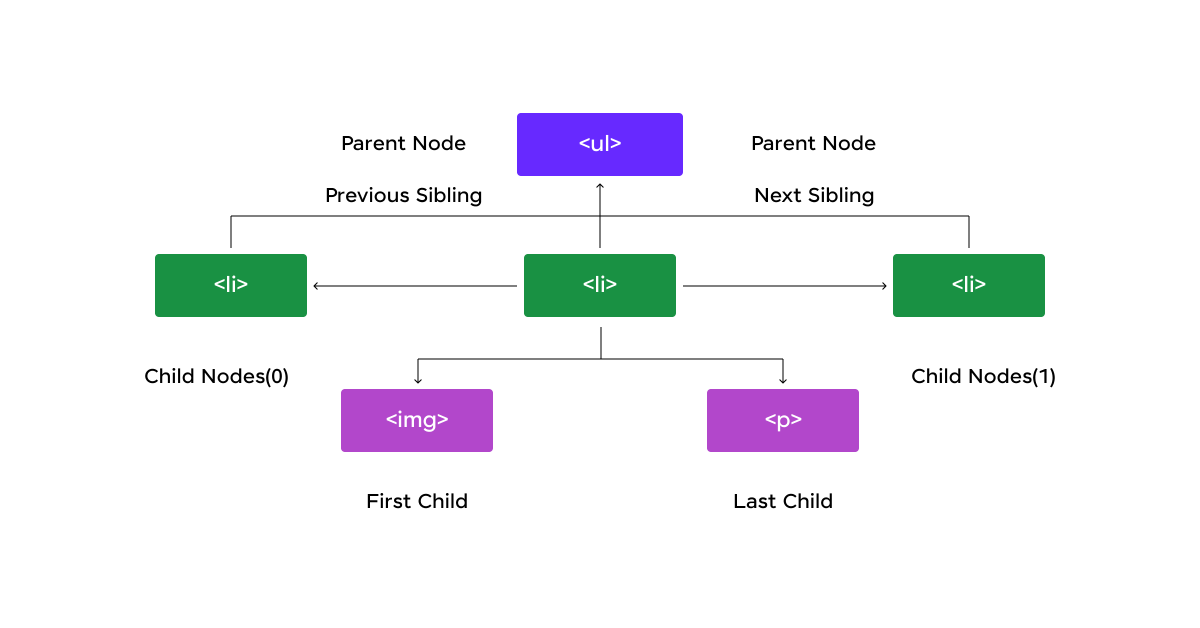
Also Read: DOM Event Flow: A Comprehensive Guide
Manipulating DOM Nodes
One of the primary purposes of working with DOM nodes is to manipulate the structure and content of a web page dynamically. The DOM provides several methods for creating, inserting, modifying, and removing nodes.
a. Creating Nodes: You can create new nodes using methods like createElement() (for creating element nodes), createTextNode() (for creating text nodes), and createComment() (for creating comment nodes).
b. Inserting Nodes: Once you have created a node, you can insert it into the DOM tree using methods like appendChild() (adds a node as the last child of a parent node), insertBefore() (inserts a node before a specified reference node), or replaceChild() (replaces an existing child node with a new node).
c. Modifying Nodes: You can modify the content and attributes of existing nodes using various properties and methods. For example, you can change the text content of a text node using the nodeValue property, modify the attributes of an element node using methods like setAttribute() and removeAttribute(), or update the styles of an element using the style property.
d. Removing Nodes: To remove a node from the DOM tree, you can use the removeChild() method on the parent node, passing the child node you want to remove as an argument.
e. Cloning Nodes: The cloneNode() method allows you to create a copy of an existing node, optionally including or excluding its child nodes.
Also Read: jQuery DOM Manipulation: Learn Everything About This On-demand Technique
Node Properties and Methods
Each type of DOM node comes with its own set of properties and methods that provide information about the node and allow you to manipulate it. Here are some commonly used properties and methods:
a. Node Properties
- nodeType: Returns an integer representing the type of the node (e.g., 1 for element nodes, 3 for text nodes, etc.).
- nodeName: Returns the name of the node (e.g., the tag name for element nodes, ‘#text’ for text nodes, etc.).
- nodeValue: Represents the value of the node (e.g., the text content for text nodes, the attribute value for attribute nodes).
- childNodes: Returns a live NodeList of all child nodes.
- parentNode: Returns the parent node of the current node.
b. Node Methods
- appendChild(newNode): Adds a new child node to the end of the node’s child node list.
- insertBefore(newNode, referenceNode): Inserts a new child node before a specified reference node.
- removeChild(node): Removes a child node from the node’s child node list.
- replaceChild(newNode, oldNode): Replaces an existing child node with a new node.
- cloneNode(deep): Creates a copy of the node. If deep is set to true, it also clones all descendant nodes.
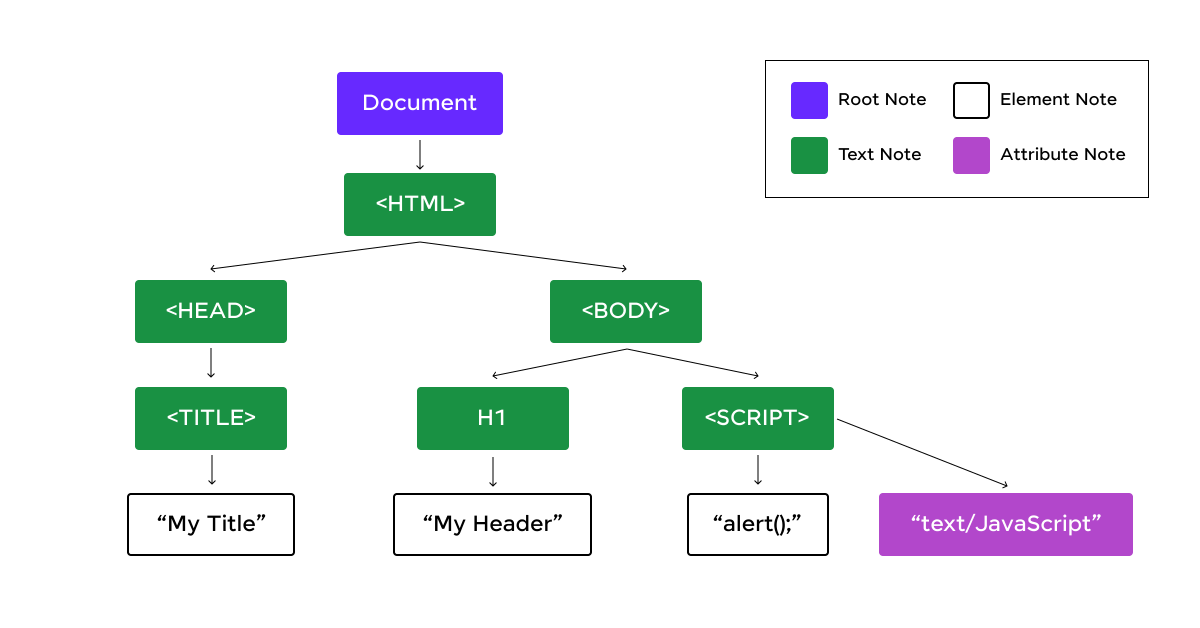
Also Read: 6 Essential Prerequisites for Learning AngularJS
Practical Applications of DOM Nodes
Understanding DOM nodes is essential for building dynamic and interactive web applications. Here are some practical applications where working with DOM nodes is crucial:
a. Dynamic Content Updates: By manipulating DOM nodes, you can update the content of a web page on the fly, such as displaying real-time data, updating user interfaces, or implementing infinite scrolling.
b. Form Validation: DOM nodes play a significant role in form validation. You can access and validate user input by traversing and manipulating the nodes associated with form elements.
c. User Interactions: Handling user interactions like clicks, hovers, and keyboard events often involves accessing and manipulating DOM nodes to respond to user actions and update the interface accordingly.
d. Dynamic Element Creation: With the ability to create and insert new nodes into the DOM, you can dynamically generate and render new elements on the web page, such as creating dropdown menus, modal windows, or custom components.
e. Web Accessibility: Proper manipulation of DOM nodes is crucial for ensuring web accessibility. By modifying attributes, adding labels, and managing focus, you can enhance the accessibility of your web applications for users with disabilities.
f. Data Visualization: When creating data visualization tools or chart libraries, you often need to generate and manipulate DOM nodes to render visual elements based on data.
g. Web Scraping and Data Extraction: DOM nodes are essential for web scraping and data extraction tasks, where you need to traverse the document tree and extract specific information from various nodes.
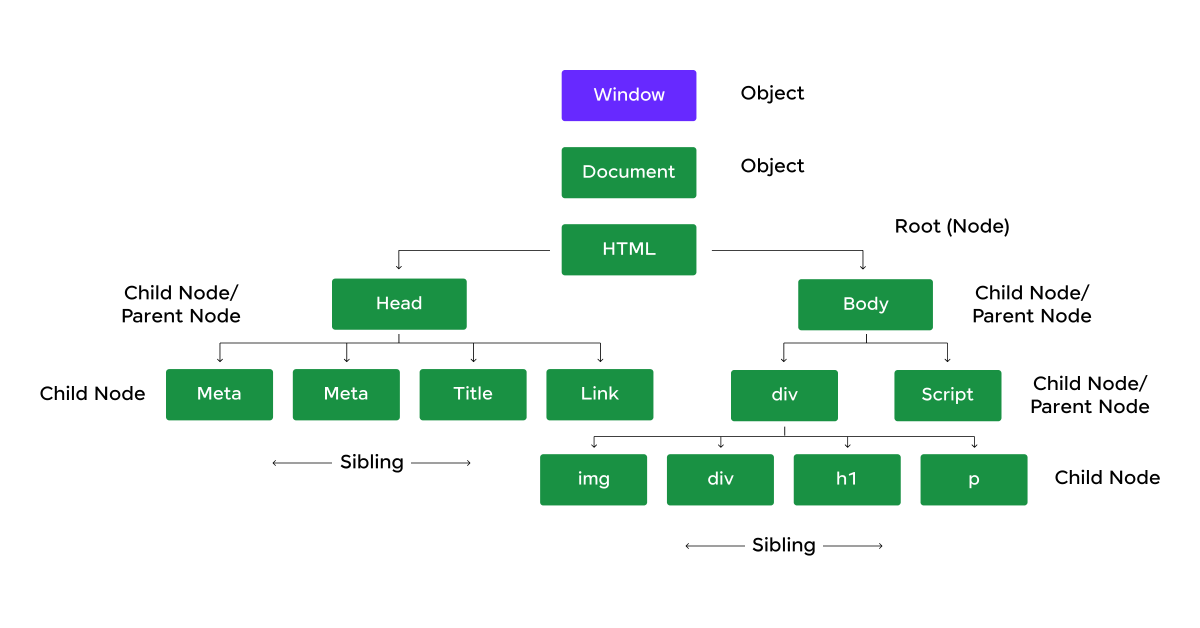
Also Read: Event Bubbling in DOM: An Interesting Technique To Know About
Best Practices for Working with DOM Nodes
While working with DOM nodes is powerful and versatile, it’s important to follow best practices to ensure efficient, maintainable, and performant code:
a. Minimize DOM Manipulations: DOM operations can be expensive, especially when dealing with large or complex document trees. Minimize the number of DOM manipulation by batching updates and avoiding unnecessary updates or reflows.
b. Use Efficient Selectors: When querying or selecting nodes, use efficient selectors like getElementById() or querySelector() instead of resource-intensive methods like getElementsByTagName() or querySelectorAll(), which can potentially return large NodeLists.
c. Cache Node References: If you need to perform multiple operations on the same node, cache a reference to that node instead of querying the DOM repeatedly. This can significantly improve performance.
d. Utilize Document Fragments: When creating or manipulating a large number of nodes, use document fragments to batch the operations and minimize reflows and repaints. This can significantly improve performance.
e. Event Delegation: Instead of attaching event listeners to individual nodes, consider using event delegation, where you attach a single event listener to a parent node and handle events based on event bubbling or capturing. This approach reduces memory usage and improves maintainability.
f. Avoid Excessive DOM Traversal: Traversing the DOM tree can be an expensive operation, especially for large documents. Try to minimize the amount of traversal needed by caching relevant nodes or using more efficient selection methods.
g. Separate Concerns: Maintain a clear separation between DOM manipulation logic and application logic. This promotes code reusability, testability, and maintainability.
h. Use Virtual DOM Libraries: For complex applications or user interfaces with frequent updates, consider using virtual DOM libraries like React, Vue, or Svelte. These libraries provide an abstraction layer that optimizes DOM updates and minimizes the number of actual DOM manipulations.
i. Leverage Browser DevTools: Modern browsers offer powerful developer tools that can help you inspect, debug, and optimize your DOM operations. Familiarize yourself with the available tools and techniques for profiling and analyzing DOM performance.
j. Test and Monitor: Implement comprehensive testing strategies, including unit tests and end-to-end tests, to ensure the correctness and reliability of your DOM manipulations. Additionally, monitor your application’s performance in production environments to identify and address potential bottlenecks related to DOM operations.
By following these best practices, you can ensure that your code is efficient, maintainable, and provides a smooth user experience, even when working with complex DOM structures and frequent updates.
DOM nodes are the fundamental building blocks of web documents, and understanding how to traverse, manipulate, and work with them is essential for creating dynamic and interactive web applications. By mastering the concepts and techniques covered in this comprehensive guide, you’ll be well-equipped to leverage the power of the DOM and build robust and performant web experiences.
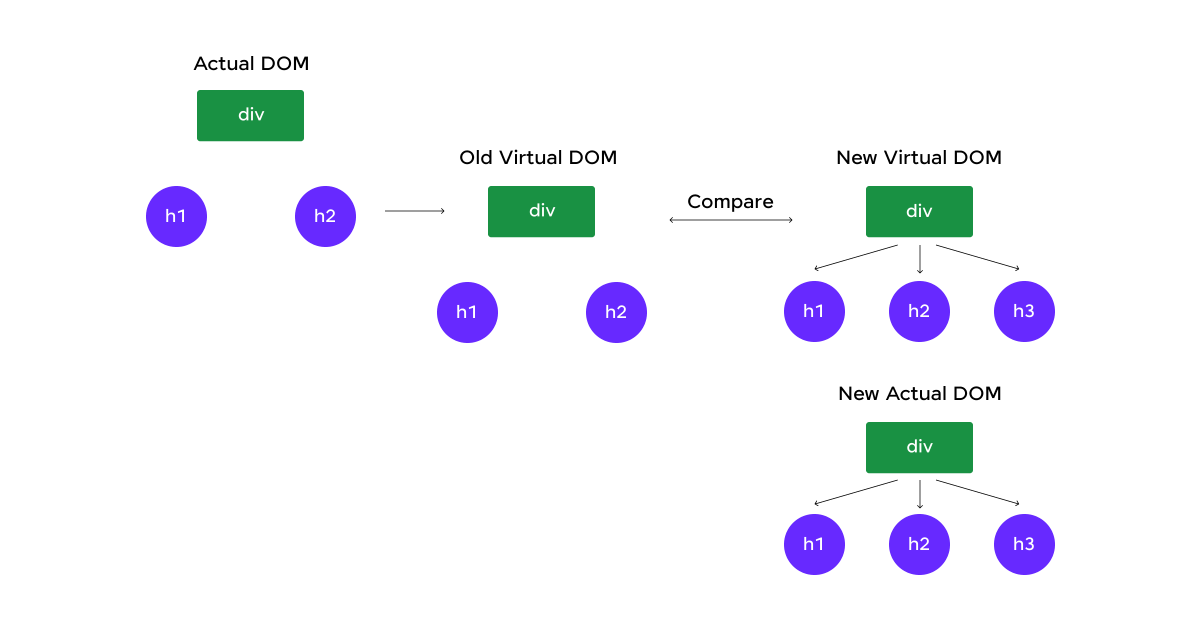
Kickstart your Full Stack Development journey by enrolling in GUVI’s certified Full Stack Development Course with Placement Assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements. Alternatively, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript course.
Conclusion
Learning DOM nodes opens doors to endless possibilities in web development. A solid grasp of DOM nodes empowers you to create robust, scalable, and interactive digital experiences. Keep exploring, keep experimenting, and continue pushing the boundaries of what’s possible with DOM nodes.
Must Explore: Event Capturing in the DOM Tree: A Comprehensive Guide
FAQs
What are DOM nodes?
DOM nodes are fundamental components of a web page’s structure, representing elements, attributes, and text content in a hierarchical tree. Each HTML or XML element, like <div> or <p>, is a node that JavaScript can manipulate dynamically. Understanding DOM nodes is essential for interactive web development as they form the foundation for accessing and modifying webpage content.
How do you access DOM nodes using JavaScript?
Accessing DOM nodes in JavaScript involves methods like getElementById for selecting elements by ID, querySelector and querySelectorAll for CSS selector-based queries, and navigating relationships between nodes using properties like childNodes and parentNode.
Once accessed, nodes can be modified in terms of content, attributes, styles, and structure, enabling dynamic updates on web pages.
What are some common operations on DOM nodes?
Common operations include updating text or HTML content, modifying attributes (e.g., src, href), adjusting styles through the style property, and manipulating the DOM structure by adding or removing nodes dynamically. Event handling is also crucial for responding to user interactions like clicks or input changes.
These operations are foundational for creating responsive and interactive web applications and enhancing user experience through real-time updates and interactive features.
Did you enjoy this article?