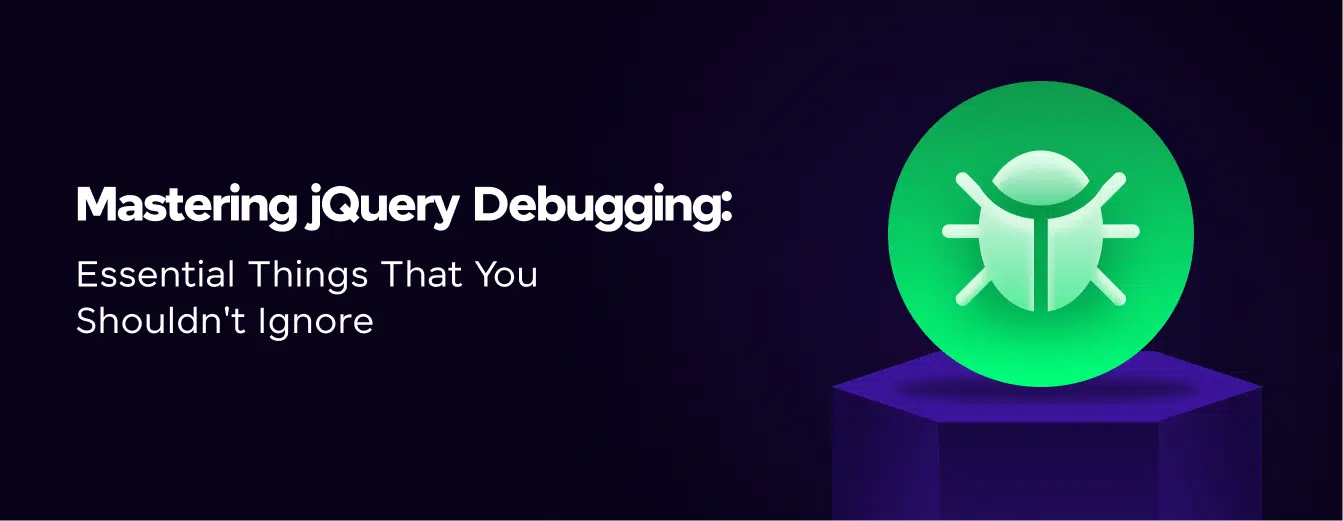
Mastering jQuery Debugging: Essential Things That You Shouldn’t Ignore
Apr 01, 2025 5 Min Read 3863 Views
(Last Updated)
When you are working with codes, it is an essential practice to understand how to debug those codes. You can’t solely rely on testers all the time.
Similarly, when you are working with jQuery, it is important to know the process of jQuery debugging and the fundamentals of it.
In this article, you will learn and understand everything related to jQuery debugging. So, without further delay, let us get started!
Table of contents
- Understanding jQuery Debugging
- Why Troubleshooting jQuery Code Matters?
- Common Headaches in jQuery Development
- Must-Have Tools for jQuery Debugging
- Browser Developer Tools
- jQuery-specific Debugging Tools
- How to Perform jQuery Debugging Like a Pro?
- Decode Error Messages
- Console Logs: Your Best Friend
- Breakpoints: Pause and Inspect
- Advanced Techniques for jQuery Debugging
- Event Handling Debugging
- AJAX Debugging
- Plugin Debugging
- Best Practices in jQuery Debugging
- Code Review and Refactoring
- Documentation and Comments
- Seeking Help and Resources
- Conclusion
- FAQs
- How can you identify jQuery errors in the console?
- What is jQuery Lint?
- How do you debug jQuery selectors?
- How can you debug jQuery animations?
Understanding jQuery Debugging
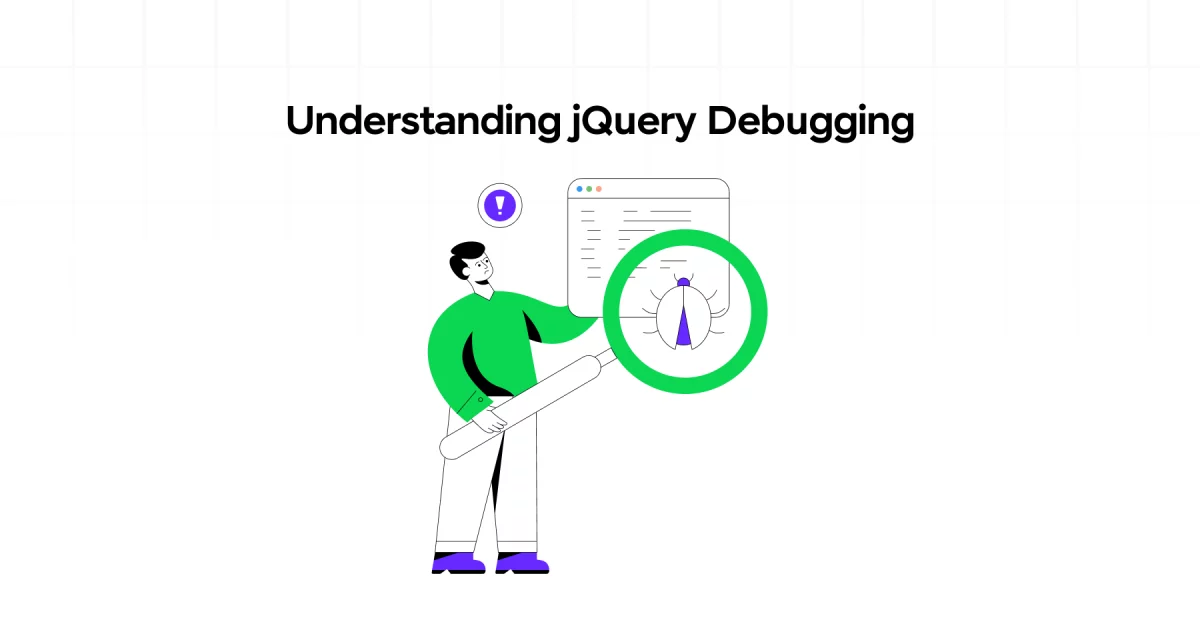
jQuery debugging is a must-have skill for any developer using this popular JavaScript library. By grasping the importance of troubleshooting and recognizing common hiccups, you can speed up your development process and boost your web app’s performance.
Why Troubleshooting jQuery Code Matters?
Fixing jQuery code isn’t just about squashing bugs, it’s about making sure your code runs smoothly, giving users a seamless experience.
Spotting and fixing errors early is the key concept of jQuery debugging which can prevent those annoying glitches that mess up your app. Plus, good jQuery debugging helps you write cleaner, more efficient code, making your life easier when you need to maintain or update your codebase.
Think of it this way: catching issues early saves you from bigger headaches down the road.
Common Headaches in jQuery Development
When you’re knee-deep in jQuery, you might run into a few common issues that can make jQuery debugging a pain. Here are some usual suspects:
- Syntax Errors: A tiny typo can stop your script dead in its tracks.
- Wrong Selectors: Using the wrong or too broad selectors can lead to unexpected results.
- Event Handling Woes: Messing up event handlers can cause conflicts or make events not fire at all.
- AJAX Troubles: Handling asynchronous requests can be tricky, leading to data retrieval and processing problems.
- Plugin Clashes: Using multiple jQuery plugins can sometimes cause compatibility issues.
Knowing these common pitfalls helps you dodge them. Getting a handle on these aspects will make you a better debugger and a more efficient developer.
Learn: jQuery Selectors: An Informative Guide On The Most Essential Framework
Must-Have Tools for jQuery Debugging
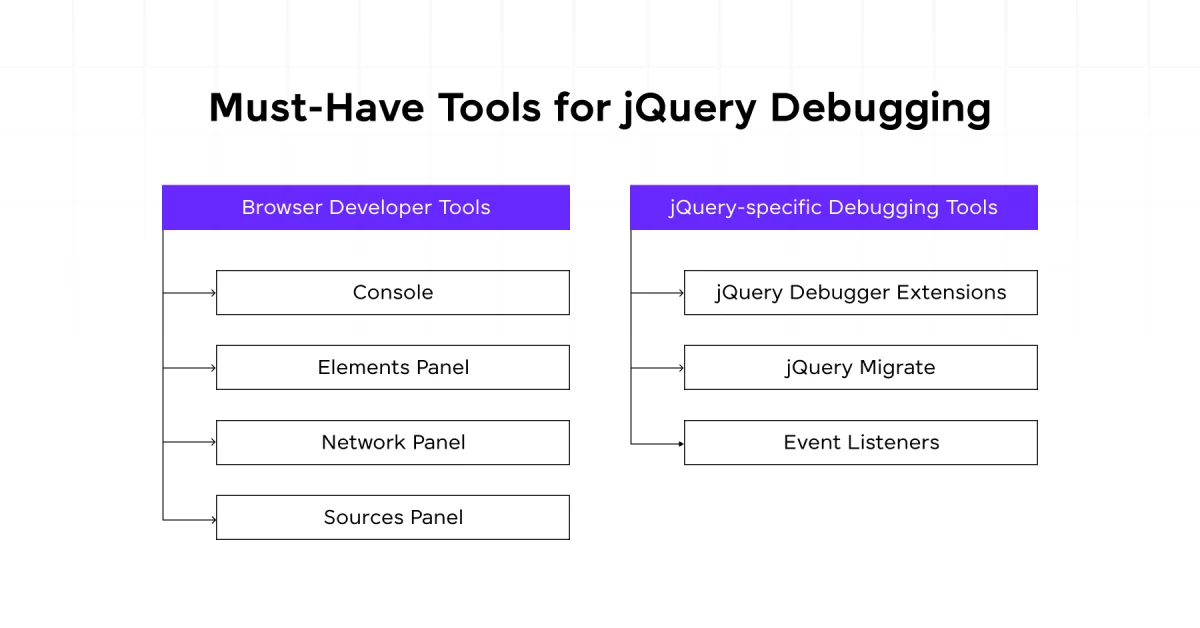
jQuery debugging can be as easy as it sounds if you have the right tools in your hands. Let’s check out some must-have tools that’ll help you in the jQuery debugging process.
But before we go any further, it is important that you have a basic understanding of backend and full-stack development. If not, consider enrolling for a professionally certified online Full-stack Development Course that teaches you everything about backend frameworks and helps you get started as a developer.
Let us see some of the best tools for jQuery debugging:
Browser Developer Tools
Browser Developer Tools like jQuery debugger for Chrome are your best friend when it comes to fixing jQuery issues. These tools are built into most modern web browsers and offer a ton of features to help you debug.
Key Features:
- Console: The console is where you can log messages and errors. It’s super handy for checking the flow of your jQuery code and outputting values.
- Elements Panel: This panel lets you inspect and tweak the DOM in real-time. You can see how your jQuery code interacts with HTML elements, making it easier to spot problems.
- Network Panel: Great for keeping an eye on AJAX requests. You can see the status of each request, the data sent, and the response received. For more advanced AJAX debugging tricks, head over to our section on AJAX Debugging.
- Sources Panel: This panel allows you to set breakpoints, step through your code line by line, and watch expressions. It’s crucial for pinpointing where your jQuery code might be messing up.
jQuery-specific Debugging Tools
Besides browser developer tools, several jQuery-specific tools like jQuery Lint can make your life easier.
Key Features:
- jQuery Debugger Extensions: These browser extensions add jQuery-specific features to your developer tools. They help you visualize jQuery data objects, events, and more.
- jQuery Migrate: This plugin helps you find and fix compatibility issues between different jQuery versions. It logs warnings in the console for deprecated features.
- Event Listeners: Figuring out which events are bound to which elements can be tricky. Tools that visualize event listeners can help you debug event-handling issues more effectively.
Getting a good handle on these jQuery debugging tools will make troubleshooting jQuery code a lot easier.
Know More: Best JQuery Course Online with Certification
How to Perform jQuery Debugging Like a Pro?
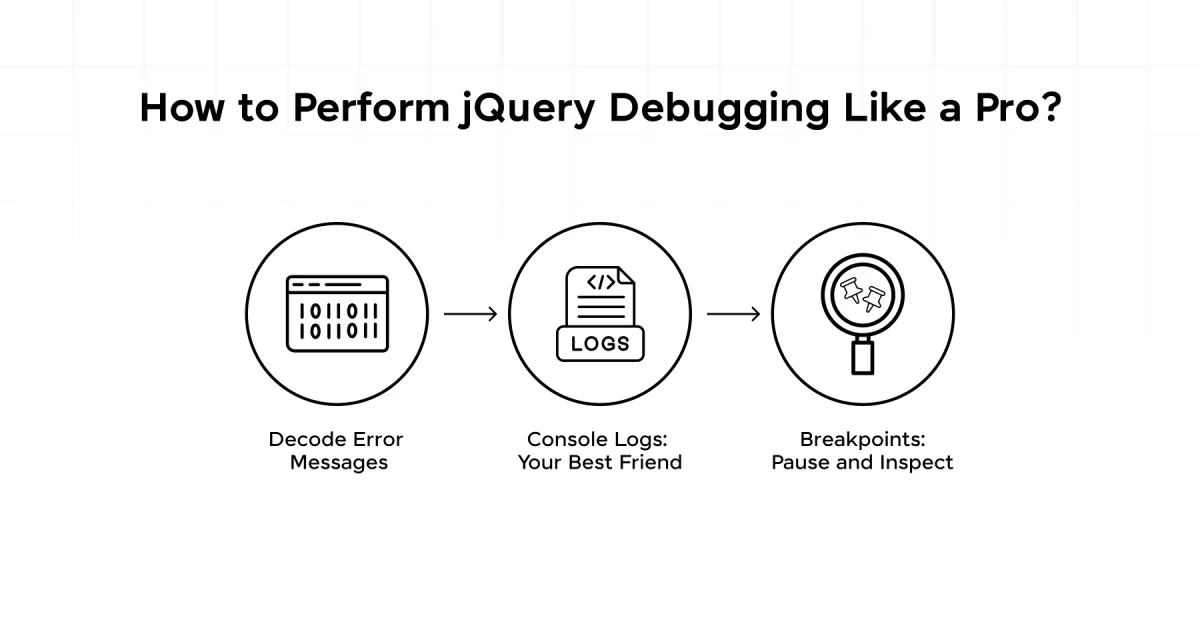
Now that you understand and know the tools of jQuery debugging, it is time to put that to use. Here are some solid tips to help you on your jQuery debugging journey without pulling your hair out.
1. Decode Error Messages
Error messages are like your code’s way of waving a red flag. They tell you what’s wrong and where to look. Here’s a quick cheat sheet for common jQuery errors:
Error Message | What It Means | How to Fix It |
---|---|---|
$ is not defined | jQuery isn’t loaded | Make sure you’ve included the jQuery library |
Uncaught TypeError: undefined is not a function | Wrong function call | Double-check your function names and syntax |
Syntax Error | Typo in your code | Look for typos and syntax mistakes |
Also Read: jQuery DOM Manipulation: Learn Everything About This On-demand Technique
2. Console Logs: Your Best Friend
Console logs are like breadcrumbs that help you trace your code’s path. Use console.log()
to print out values and messages to the browser’s console. This way, you can see what’s happening at different points in your code.
Here’s a quick example:
$(document).ready(function() {
console.log("Document is ready!");
var element = $('#myElement');
console.log("Element:", element);
});
This trick helps you keep tabs on variable values, function outputs, and the state of the DOM.
3. Breakpoints: Pause and Inspect
Breakpoints let you hit the pause button on your code. You can inspect variables, see the call stack, and step through your code one line at a time.
How to Set Breakpoints:
- Open Developer Tools (hit F12 or right-click and select “Inspect”).
- Go to the “Sources” tab.
- Find your jQuery file.
- Click on the line number where you want to pause.
Breakpoints give you a close-up view of what’s happening in your code. Using these simple strategies can make jQuery debugging a lot easier.
Explore More: Migrating from jQuery to Vanilla JavaScript for Modern Web Development
Advanced Techniques for jQuery Debugging
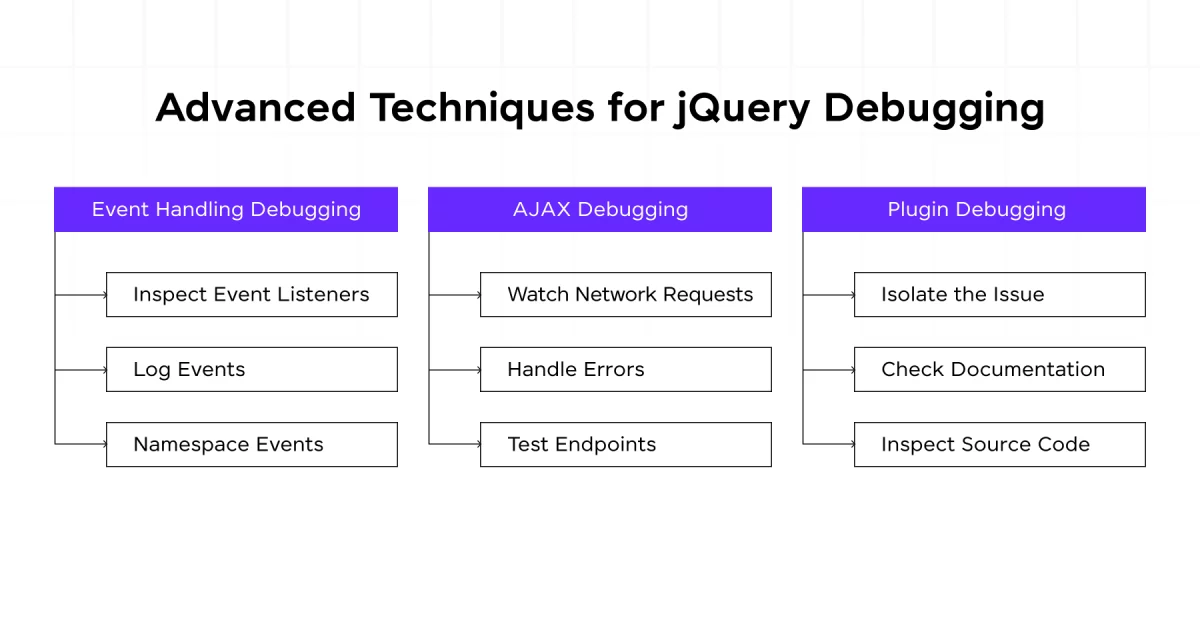
The basics are done and we instilled you with the foundational knowledge for jQuery debugging. It is now time to get the hang of advanced jQuery debugging techniques that can seriously up your game in squashing bugs quickly. We’re talking event handling, AJAX, and plugin debugging.
1. Event Handling Debugging
Event handling is a big deal in jQuery, but it can be a pain to debug. Here’s how you can make it easier:
- Inspect Event Listeners: Open your browser’s developer tools and check out the event listeners on your elements. This helps you see where events are attached and if they’re working right.
- Log Events: Stick some console logs in your event handlers to see when and how events are firing. This gives you a peek into the event flow and any weird stuff going on.
- Namespace Events: Use event namespacing to keep your event listeners organized. This avoids conflicts and makes debugging specific events a breeze.
2. AJAX Debugging
AJAX is awesome but can be tricky to debug. Here’s how to handle it:
- Watch Network Requests: Use the Network tab in your browser’s developer tools to keep an eye on AJAX requests and responses. You can check headers, payloads, and responses to spot issues.
- Handle Errors: Add error handling in your AJAX calls to catch and log errors. This helps you find problems with server responses or connectivity.
- Test Endpoints: Use tools like Postman to test your API endpoints separately. This ensures they’re working and returning the right data.
AJAX Debugging Strategy | What It Does |
---|---|
Watch Network Requests | Inspect AJAX requests and responses in browser tools |
Handle Errors | Capture and log errors in your AJAX calls |
Test Endpoints | Verify API endpoints with tools like Postman |
3. Plugin Debugging
jQuery plugins are great for adding features but can also bring bugs. Here’s how to debug them:
- Isolate the Issue: Turn off other plugins and scripts to see if the problem is with the plugin. This helps you focus on the plugin itself.
- Check Documentation: Look at the plugin’s documentation for known issues, settings, and troubleshooting tips. This can give you clues and solutions.
- Inspect Source Code: Dive into the plugin’s source code to understand how it works and find bugs. Add console logs to trace the execution flow and spot problems.
jQuery Debugging plugins means isolating the issue, checking docs, and inspecting the code. By using these advanced jQuery debugging techniques, you’ll get better at troubleshooting and fixing jQuery issues.
Learn More: 6 Emerging Programming Languages for Backend Development
Best Practices in jQuery Debugging
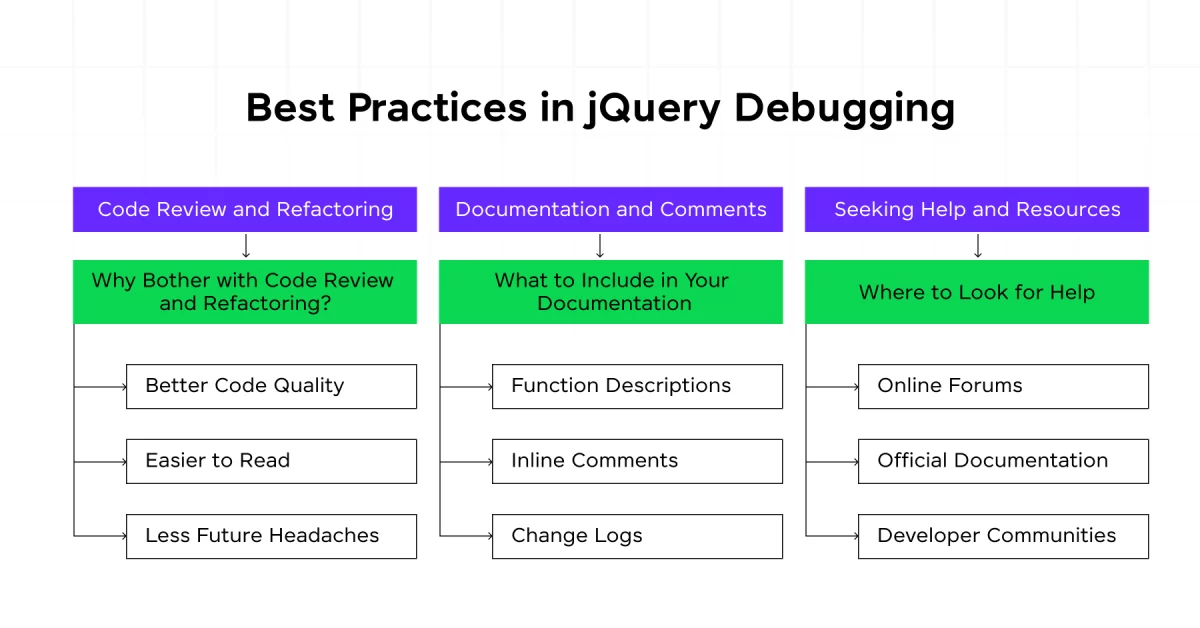
Now, there are certain aspects to any technique that will help us efficiently use it. Similarly, best practices in jQuery debugging can boost your debugging skills and make your code shine. Here’s how to do it right:
1. Code Review and Refactoring
Regularly checking and cleaning up your code is like giving your car a regular service. It keeps things running smoothly and helps you spot issues before they become big problems.
Refactoring means tweaking your code to make it cleaner and easier to read without changing what it does. This makes future fixes a breeze.
Why Bother with Code Review and Refactoring?
- Better Code Quality: Sniffs out bugs and sloppy code.
- Easier to Read: Makes your code a piece of cake to understand.
- Less Future Headaches: Cuts down on the time you’ll spend fixing stuff later.
2. Documentation and Comments
Writing clear documentation and comments is like leaving breadcrumbs for yourself and others. It helps everyone understand what your code is doing and why, making it simpler to fix when things go wrong.
What to Include in Your Documentation:
- Function Descriptions: A quick note on what each function does.
- Inline Comments: Little hints next to tricky parts of your code.
- Change Logs: A diary of what you’ve changed and why.
Example of Inline Comments:
// This function toggles the visibility of an element
function toggleVisibility(selector) {
$(selector).toggle(); // jQuery method to show/hide elements
}
3. Seeking Help and Resources
Sometimes, you need a little help from your friends—or the internet. There are tons of resources out there where you can find answers and get advice from other developers.
Where to Look for Help:
- Online Forums: Sites like Stack Overflow are gold mines of info from seasoned developers.
- Official Documentation: The jQuery documentation is your go-to for detailed info on methods and best practices.
- Developer Communities: Join groups on social media or forums to get quick answers and share your own tips.
By sticking to these practices, you’ll make your jQuery troubleshooting smoother and become a better coder.
If you want to learn more about jQuery debugging in full-stack development, then consider enrolling in GUVI’s Certified Full Stack Development Course which not only gives you theoretical knowledge but also practical knowledge with the help of real-world projects.
Also Read: Master Backend Development With JavaScript | Become a Pro
Conclusion
In conclusion, mastering jQuery debugging is an essential skill for any full-stack developer working with this powerful library. Effective jQuery debugging can save time, reduce frustration, and improve the quality of your code.
As you continue to refine your debugging skills, you’ll find that overcoming complex challenges becomes more manageable, leading to smoother and more successful development projects.
Also, Explore More Advanced Debugging Techniques For Full Stack Development
FAQs
You can identify jQuery errors by checking the browser’s developer console, where error messages and stack traces provide clues about the issues.
jQuery Lint is a tool that automatically checks your jQuery code for common errors and potential issues, helping you catch problems early in the development process.
You can debug jQuery selectors by using the browser’s console to test selectors, checking if they correctly match the intended elements, and optimizing them for performance.
You can debug jQuery animations by checking the timing and duration of animations, ensuring the correct elements are being animated, and using the .stop() method to control animations.
Did you enjoy this article?