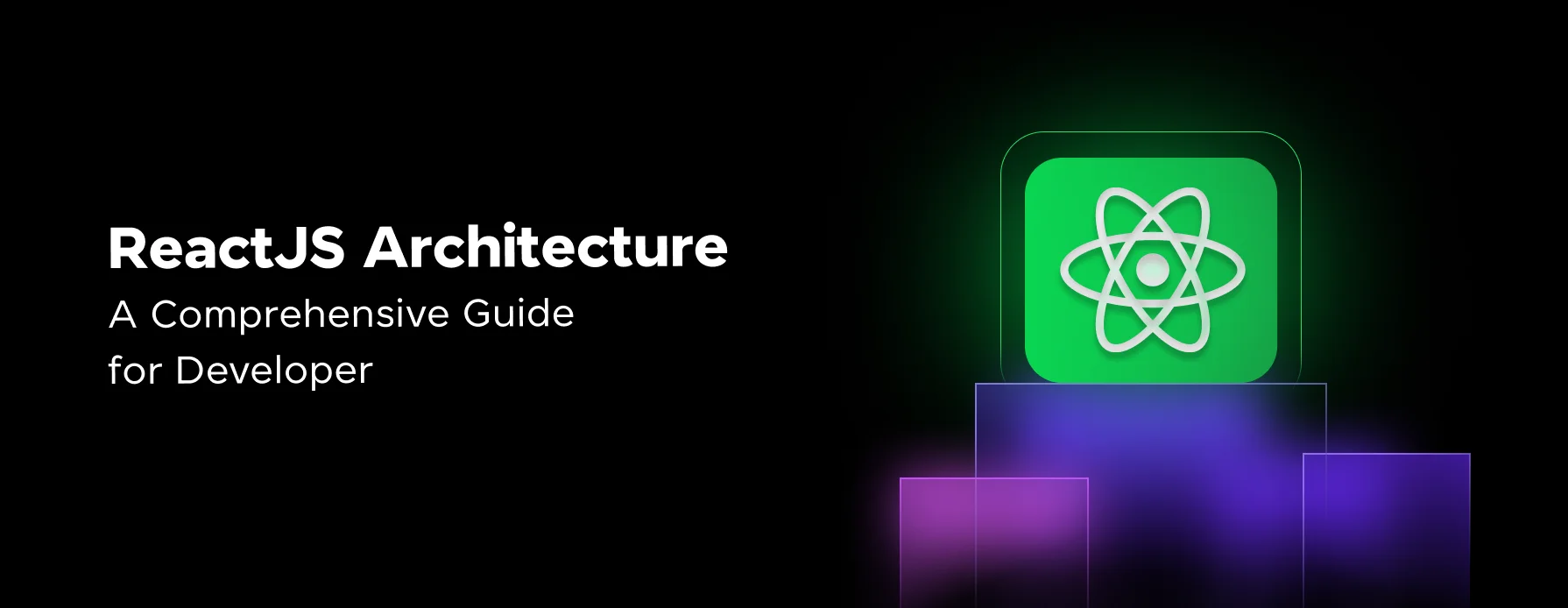
ReactJS Architecture: A Comprehensive Guide For Developers [2024]
Jun 18, 2024 6 Min Read 552 Views
(Last Updated)
The world of full-stack development is vast and consists of a lot of libraries and frameworks that help you make outstanding websites with ease. One such important framework is ReactJS. If you are someone new to this, no worries, you will learn everything about ReactJS architecture in this article.
By the end of this article, you will be gaining invaluable knowledge about ReactJS architecture that gives you the freedom and flexibility to work on the backend.
So, without further ado, let’s get started.
Table of contents
- What is ReactJS Architecture?
- Understanding ReactJS Architecture from Scratch
- Component-Based Architecture
- State Management
- Unidirectional Data Flow
- Advanced Concepts of ReactJS Architecture
- Context API
- Redux
- Code Splitting and Lazy Loading
- Higher-Order Components (HOCs)
- Common Issues and Best Practices in ReactJS Architecture
- Common Issues in ReactJS Architecture
- Best Practices in ReactJS Architecture
- Conclusion
- FAQs
- What is the purpose of the useEffect hook in React?
- What are some common performance optimization techniques in ReactJS?
- What are some best practices for organizing files in a React project?
- How does the useContext hook work in React?
What is ReactJS Architecture?
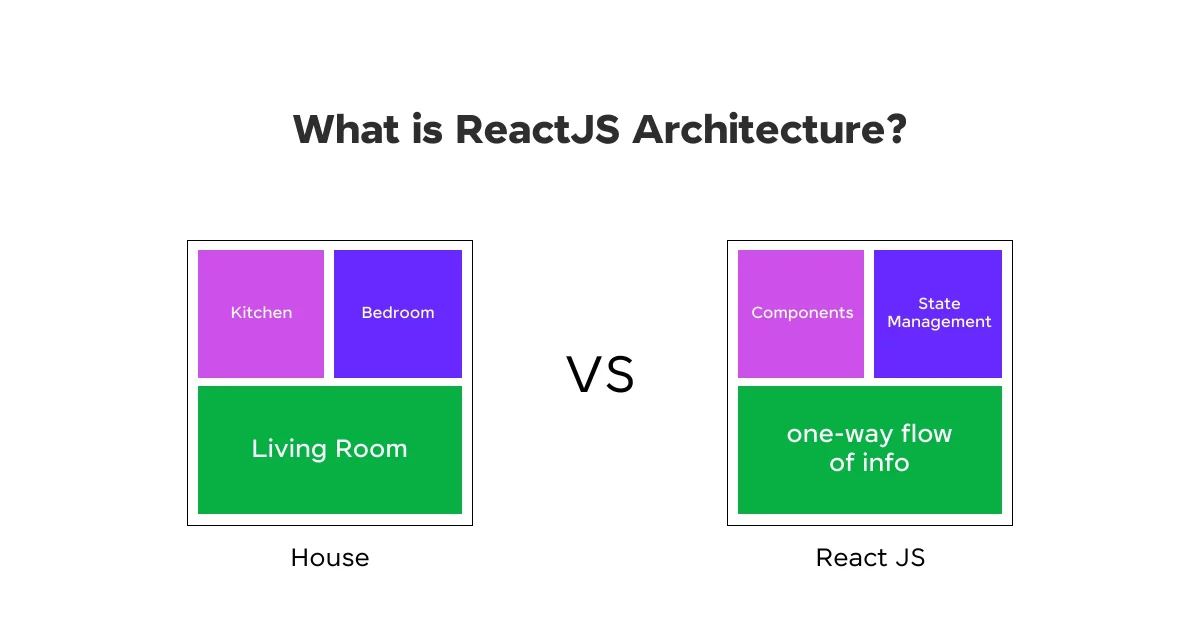
The foundation is stronger for any building to stand tall and mighty. So, we’ll be starting out learning the journey of ReactJS architecture right from its definition.
Imagine you’re building a house. You have different parts like the kitchen, living room, bedrooms, etc. Each part has its own purpose and can be designed separately, but they all come together to form a complete house.
ReactJS architecture is like this house. It’s a way of organizing and structuring a web application using React, a popular JavaScript library. In this “house,” we have components, state management, and a one-way/unidirectional flow of information.
When you combine components, state management, and one-way data flow, you get a clear and organized structure for building your web application. This is what we call ReactJS architecture. It helps developers create applications that are easy to build, maintain, and scale.
So, to put it simply, ReactJS architecture is like designing and building a house with different rooms (components), a smart home system (state management), and a simple plumbing system (one-way data flow) to keep everything running smoothly.
Learn More: 6 Emerging Programming Languages for Backend Development
Understanding ReactJS Architecture from Scratch
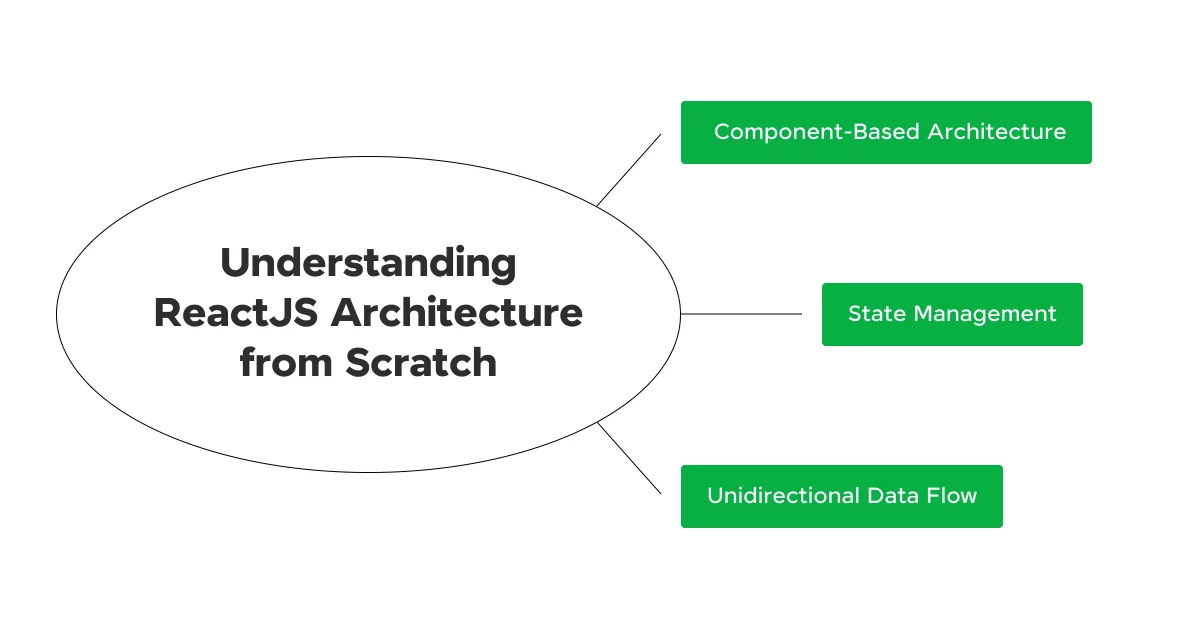
Now that you understand the basics and definition of ReactJS architecture, it is time to move on and learn in detail about the stuff that we saw previously.
But before we go any further, it is important that you have a basic understanding of JavaScript and full-stack development. If not, consider enrolling for a professionally certified online Full-stack Development Course that teaches you everything about backend frameworks and helps you get started as a developer.
Let us now dig deep into the ReactJS architecture:
1. Component-Based Architecture
As you have seen earlier, ReactJS architecture comprises three major architecture and the first one is component-based architecture.
At the core of ReactJS architecture is its component-based structure. Components are the building blocks of a React application. They encapsulate parts of the user interface (UI) into independent, reusable pieces, each managing its own state and rendering logic.
Think of components as the rooms in your house. Each component is a piece of the user interface (UI) that you can build separately.
For example, you might have a header component, a footer component, and a content component. These components are like the different rooms, each serving a specific purpose but coming together to form the whole application.
Functional and Class Components
React offers two types of components: functional and class components.
- Functional Components are stateless and primarily concerned with rendering UI. They are simpler and easier to test, making them ideal for presentational purposes.
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
- Class Components are stateful and can hold and manage state. They provide more features and lifecycle methods, allowing for more complex functionality.
class Greeting extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
With the introduction of hooks in React 16.8, functional components can now manage state and side effects, blurring the line between the two.
Must Explore: The Ultimate Guide to React Component Libraries in 2024
2. State Management
State management is crucial in ReactJS architecture to keep track of data changes over time. Each component can have its own state, which React efficiently updates in response to user interactions.
This helps in updating the right parts of your application when something changes.
useState and useEffect Hooks
React’s hooks, such as useState
and useEffect
, provide a cleaner and more functional approach to managing state and side effects in functional components.
- useState allows you to add state to functional components.
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
};
Know More: useState() Hook in React for Beginners | React Hooks 2024
- useEffect allows you to perform side effects in your components, such as fetching data or directly interacting with the DOM.
import React, { useState, useEffect } from 'react';
const DataFetcher = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []); // Empty dependency array means this effect runs once, similar to componentDidMount.
return (
<div>
{data ? <p>{data.message}</p> : <p>Loading...</p>}
</div>
);
};
3. Unidirectional Data Flow
ReactJS architecture promotes a unidirectional data flow, meaning data flows in one direction: from parent to child components. This makes it easier to debug and understand the state of your application.
React follows a one-way data flow, meaning data flows in one direction from parent to child components. It’s like water flowing through pipes in a house, from the main supply (parent) to the faucets (child components).
This makes it easier to understand and debug your application because you always know where the data is coming from and where it’s going.
Props
Props are used to pass data from parent to child components. They are read-only and cannot be modified by the child component.
const ChildComponent = (props) => {
return <p>{props.message}</p>;
};
const ParentComponent = () => {
return <ChildComponent message="Hello, world!" />;
};
Explore: The Greatest Challenges of Backend Developers
Advanced Concepts of ReactJS Architecture
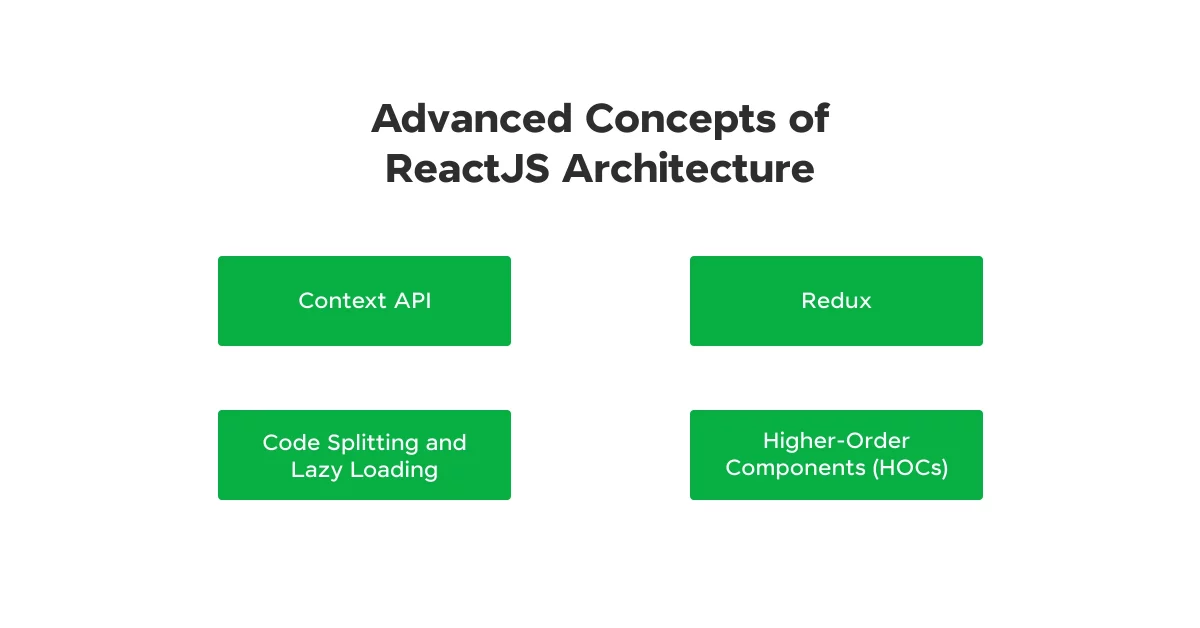
As your application grows, you may need to manage more complex states and side effects. Here are a few advanced concepts to consider in ReactJS architecture:
1. Context API
The Context API allows you to share the state across the entire app or part of it without passing props down manually at every level.
const MyContext = React.createContext();
const App = () => {
const [value, setValue] = useState("Hello from context!");
return (
<MyContext.Provider value={value}>
<ChildComponent />
</MyContext.Provider>
);
};
const ChildComponent = () => {
const value = useContext(MyContext);
return <p>{value}</p>;
};
Also Read: Use ReactJS to Fetch and Display Data from API – 5 Simple Steps
2. Redux
Redux is a popular state management library for React applications, offering a more structured and scalable approach to managing state.
import { createStore } from 'redux';
import { Provider } from 'react-redux';
const reducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
default:
return state;
}
};
const store = createStore(reducer);
const App = () => (
<Provider store={store}>
<Counter />
</Provider>
);
3. Code Splitting and Lazy Loading
Code splitting is a technique that allows you to split your code into smaller chunks, which can be loaded on demand. Lazy loading is a way to load these chunks only when they are needed.
This helps improve your application’s performance by reducing the initial load time and loading only the necessary parts of your application as the user navigates through it.
ReactJS architecture has built-in support for code splitting and lazy loading using React.lazy
and Suspense
.
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
const App = () => {
return (
<div>
<h1>My React App</h1>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
};
export default App;
In this example, LazyComponent
is loaded only when it is needed, and while it’s loading, a fallback loading message is shown.
Also Read: ReactJS Tutorial: Crafting a ToDo List Application in 5 Simple Steps (Code Included)
4. Higher-Order Components (HOCs)
Higher-order components (HOCs) are advanced techniques in React JS architecture for reusing component logic. A HOC is a function that takes a component and returns a new component with additional props or functionality.
HOCs allow you to reuse logic across multiple components without duplicating code, making your application more modular and easier to maintain.
const withLogging = (WrappedComponent) => {
return class extends React.Component {
componentDidMount() {
console.log('Component mounted:', WrappedComponent.name);
}
render() {
return <WrappedComponent {...this.props} />;
}
};
};
const Hello = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
const HelloWithLogging = withLogging(Hello);
const App = () => {
return <HelloWithLogging name="World" />;
};
export default App;
Understanding these advanced concepts in ReactJS architecture is fundamental to building efficient and scalable applications.
Know More: Best React Js Course Online with Certification
Common Issues and Best Practices in ReactJS Architecture
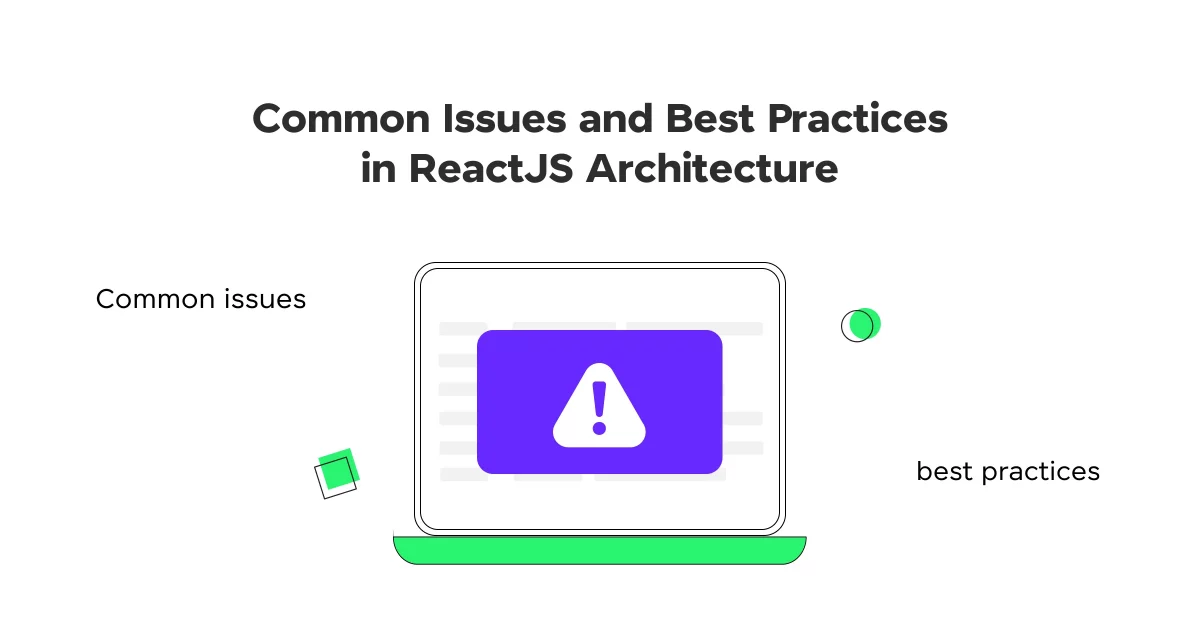
When you’re building an application with ReactJS architecture, it’s essential to understand some common issues you might encounter and the best practices to follow.
This will help you create a smooth, efficient, and maintainable application.
Common Issues in ReactJS Architecture
- Component Overloading
- Issue: You might find yourself putting too much logic into a single component. This makes the component complex and difficult to maintain.
- Solution: Break down your components into smaller, reusable ones. If a component is doing too much, it might be better to split it into multiple components.
- State Management Complexity
- Issue: Managing state can become complicated as your application grows. You might end up with a tangled web of state updates.
- Solution: Use state management libraries like Redux or Context API to handle state more predictably. Keep the state as close to where it’s needed as possible.
- Improper Use of Props
- Issue: Passing too many props through many layers of components (prop drilling) can make your code messy and hard to follow.
- Solution: Use the Context API to share state between components that are far apart in the component tree, avoiding the need to pass props through many levels.
- Performance Issues
- Issue: Your application might become slow if you don’t optimize your components and state updates.
- Solution: Use tools like React’s
shouldComponentUpdate
or React.memo to prevent unnecessary re-renders. Make sure to avoid heavy computations inside your render methods.
- Poor File Structure
- Issue: As your project grows, having an unorganized file structure can make it difficult to find and manage your code.
- Solution: Organize your files by feature or by type. Consistency is key, so choose a structure and stick to it.
Also, Know About Mistakes to Avoid When Starting the First React Project
Best Practices in ReactJS Architecture
- Component Design
- Practice: Design your components to be small, reusable, and focused on a single task. This makes your code easier to read, test, and maintain.
- Example: Instead of a single large component for a form, break it down into smaller components like
FormHeader
,FormBody
, andFormFooter
.
- Effective State Management
- Practice: Use hooks like
useState
anduseReducer
for managing state within components. For global state, consider using Context API or Redux. - Example: Use
useReducer
for complex state logic that involves multiple sub-values or when the next state depends on the previous state.
- Practice: Use hooks like
- Clear Prop Handling
- Practice: Pass only the necessary props to child components. Avoid passing down entire objects if only a few properties are needed.
- Example: Instead of passing a whole user object, pass only the necessary properties like
username
andemail
.
- Optimization Techniques
- Practice: Use React’s performance optimization techniques like React.memo and useCallback to prevent unnecessary re-renders.
- Example: Wrap components in
React.memo
to memoize the result and avoid re-rendering unless the props change.
- Consistent File Structure
- Practice: Adopt a consistent file structure for your components and other files. Group related files together and follow a naming convention.
- Use of PropTypes and TypeScript
- Practice: Use PropTypes or TypeScript to define the types of props your components should receive. This helps catch errors early and makes your code more readable.
- Documentation and Comments
- Practice: Keep your code well-documented with comments and README files. This helps others (and your future self) understand your code better.
- Example: Add comments to explain complex logic or important decisions in your code. Use README files to provide an overview of your project and instructions for setup and usage.
By keeping these common issues in mind and following these best practices, you can build scalable and robust applications using ReactJS architecture.
If you want to learn more about ReactJS architecture in full-stack development, then consider enrolling in GUVI’s Certified Full Stack Development Career Program which not only gives you theoretical knowledge but also practical knowledge with the help of real-world projects.
Also Read: Frontend vs Backend Development: Top 7 Differences
Conclusion
In conclusion, understanding ReactJS architecture is key to building efficient and scalable web applications. By utilizing components, effective state management, and unidirectional data flow, you can create robust and maintainable user interfaces.
Advanced concepts in ReactJS architecture further enhance your application’s performance and reusability. Adopting these best practices and addressing common issues will help you master ReactJS and develop applications that are both powerful and easy to manage.
Also Explore: React vs Angular vs Vue: Choosing the Right Framework [2024]
FAQs
1. What is the purpose of the useEffect hook in React?
The useEffect hook allows you to perform side effects in functional components, such as data fetching or interacting with the DOM, similar to lifecycle methods in class components.
2. What are some common performance optimization techniques in ReactJS?
Common techniques include using React.memo to memoize components, the useCallback and useMemo hooks to memoize functions and values, and code splitting with React.lazy and Suspense.
3. What are some best practices for organizing files in a React project?
Organize files by feature or type, maintain a consistent structure, use meaningful naming conventions, and group related files together for better maintainability.
4. How does the useContext hook work in React?
The useContext hook allows you to access the context value in functional components, making it easy to consume context data without prop drilling.
Did you enjoy this article?