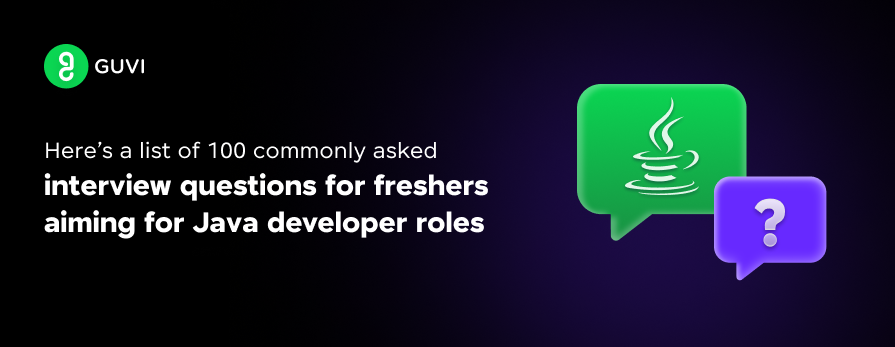
100 Commonly Asked Interview Questions for Java Developer Roles [Freshers]
Oct 09, 2024 4 Min Read 252 Views
(Last Updated)
Java developer roles have been of major importance since the dawn of the internet. Till today, there is a lot of demand for Java developer roles and to crack these interviews easily, we got you covered.
This article consists of 100 commonly asked interview questions for Java Developer roles that will prove to be useful and will give you the confidence to attend the interview! So, without further ado, let us get started!
Table of contents
- 100 Commonly Asked Interview Questions for Java Developer Roles
- Core Java and OOP Concepts
- Java Basics and Syntax
- Collections Framework
- Multithreading and Concurrency
- Exception Handling
- Java 8 Features
- SQL and Database Concepts
- Interview Preparation Tips:
- Conclusion
100 Commonly Asked Interview Questions for Java Developer Roles
Here’s a list of 100 commonly asked interview questions for Java developers which covers core Java concepts, OOP principles, collections, multithreading, exception handling, Java frameworks, database knowledge, and some advanced topics.
1. Core Java and OOP Concepts
- What is Java, and how does it work?
- What are the JVM, JRE, and JDK, and how do they differ?
- What are the four principles of Object-Oriented Programming (OOP)?
- What is the difference between public, private, protected, and default (package-private) access modifiers?
- Explain the concepts of class and object in Java.
- What is polymorphism? Give an example of runtime and compile-time polymorphism.
- What is method overloading and method overriding in Java?
- What is the purpose of the this keyword in Java?
- What is the difference between an interface and an abstract class?
- Explain the concept of inheritance in Java with an example.
- What are constructors, and how do they work in Java?
- What is the significance of the super keyword?
- What is encapsulation in Java? How is it achieved?
- What is an abstraction in Java? Provide an example.
- What is the use of the static keyword in Java?
- What is the difference between == and .equals() method in Java?
- What is the purpose of the final keyword in Java?
- What is the difference between a class and an object?
- What is the difference between an abstract class and a concrete class?
- What are the different types of constructors in Java?
2. Java Basics and Syntax
- What are primitive data types in Java?
- Explain autoboxing and unboxing in Java.
- What are wrapper classes in Java?
- What is the difference between String, StringBuffer, and StringBuilder?
- What is a final variable, method, and class in Java?
- What are the default values for instance variables in Java?
- What is a package in Java? Why is it used?
- What is the difference between import and static import in Java?
- What is the purpose of a main method in Java?
- What is a default constructor, and when is it used?
- Explain the use of a try-catch-finally block in Java.
- What are checked and unchecked exceptions in Java?
- What is the difference between throw and throws?
- What is the difference between break and continue in loops?
- What is an enhanced for-each loop, and when is it used?
- What is an enumeration (enum) in Java, and how is it used?
- What is the significance of the synchronized keyword in Java?
- What is the difference between shallow copy and deep copy in Java?
- What are static blocks, and when are they executed?
- Can we have multiple public classes in a single Java file? Why or why not?
3. Collections Framework
- What is the Java Collections Framework?
- What is the difference between ArrayList and LinkedList?
- What is the difference between HashMap and TreeMap?
- What is the difference between HashSet and LinkedHashSet?
- Explain the internal workings of a HashMap in Java.
- What is the difference between HashMap and Hashtable?
- What is the difference between List, Set, and Map interfaces?
- What is the difference between Iterator and ListIterator?
- How does the ConcurrentHashMap differ from a HashMap?
- What is the difference between fail-fast and fail-safe iterators in Java?
- How does the LinkedHashMap maintain insertion order?
- What are generics in Java? Explain with an example.
- What is the difference between ArrayList and Vector in Java?
- How does the TreeSet maintain sorted order?
- What is the difference between Array and ArrayList in Java?
- What is the role of the Comparator and Comparable interfaces in sorting collections?
- What is the difference between Queue and Deque?
- What is the difference between a PriorityQueue and a LinkedList in Java?
- What is the purpose of Collections.sort() in Java?
- What is the purpose of Collections.unmodifiableList() in Java?
4. Multithreading and Concurrency
- What is multithreading in Java, and why is it important?
- What is the difference between Thread class and Runnable interface?
- What are the different states of a thread in Java?
- What is synchronization, and why do we need it?
- What is a deadlock, and how can you avoid it?
- What is the difference between wait() and sleep() in Java?
- What is the difference between notify() and notifyAll() in Java?
- What is thread priority in Java, and how does it affect thread execution?
- What is the volatile keyword in Java, and how is it used?
- What is a thread pool, and how is it used in Java?
- What is the purpose of the ExecutorService framework in Java?
- Explain the difference between Callable and Runnable interfaces.
- What is synchronized block and method in Java?
- What is a CountDownLatch in Java, and when would you use it?
- What is the ForkJoinPool in Java?
5. Exception Handling
- What is an exception in Java? How is it different from an error?
- What are the main differences between checked and unchecked exceptions?
- What is the difference between throw and throws in Java?
- What is the purpose of a finally block?
- What is try-with-resources, and how does it work?
- What is a custom exception, and how do you create one in Java?
- What is NullPointerException, and how can you prevent it?
- What is the RuntimeException class in Java?
- Can we have multiple catch blocks in Java? How does it work?
- What is the Exception class in Java, and how is it related to the Throwable class?
6. Java 8 Features
- What are lambda expressions in Java 8, and how do they work?
- What is the purpose of the Stream API in Java 8?
- What are functional interfaces, and how are they used in Java 8?
- What is a default method in an interface, and why was it introduced?
- What is the Optional class in Java 8, and how is it used?
- What is the difference between map() and flatMap() in the Stream API?
- What is the purpose of the forEach() method in the Stream API?
- What is the Predicate interface, and how is it used in Java 8?
- Explain the difference between reduce() and collect() methods in the Stream API.
- What is method reference in Java 8, and how does it differ from a lambda expression?
- What is the Collectors class in Java 8?
7. SQL and Database Concepts
- What is SQL, and how is it used in Java applications?
- What is the difference between INNER JOIN and LEFT JOIN in SQL?
- What is the purpose of a PRIMARY KEY in a database?
- What is the difference between GROUP BY and ORDER BY in SQL?
Interview Preparation Tips:
- Revise core Java and OOP concepts.
- Practice coding using online platforms such as LeetCode, HackerRank, etc.
- Understand projects you’ve worked on and be ready to explain them.
- Mock interviews: Participate in practice interviews.
- Read: Keep yourself updated with the latest Java features and best practices.
This comprehensive set of questions will help you cover the essential topics required for a fresher Java developer interview.
In case, you want to learn more about Java Full stack development and how to become one, consider enrolling for GUVI’s Certified Java Full-stack Developer Course that teaches you everything from scratch and make sure you master it!
Conclusion
In conclusion, preparing for a Java developer role as a fresher requires not just technical know-how but also the ability to confidently answer a wide range of questions that reflect your understanding of core concepts, problem-solving skills, and adaptability.
By familiarizing yourself with these 100 commonly asked interview questions, you’ll be better equipped to tackle the challenges ahead and leave a positive impression on potential employers. Remember, practice and clarity are key, so review these questions thoroughly and continue refining your skills. Best of luck with your journey into the world of Java development!
Did you enjoy this article?