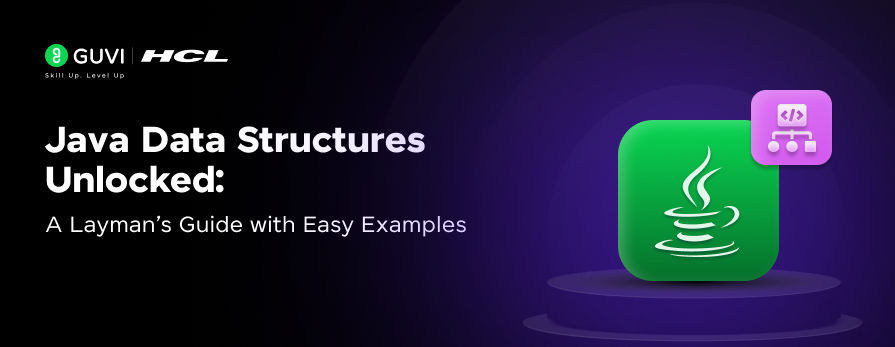
Java Data Structures Unlocked: A Layman’s Guide with Easy Examples
Oct 09, 2024 3 Min Read 116 Views
(Last Updated)
When we think about coding in Java, one thing that often runs behind the scenes but plays a crucial role is how Java stores and organizes data. These behind-the-scenes mechanics are known as data structures.
This article comprises everything related to Java Data Structures. Let’s break it down with simple stories and examples to help you understand the Java data structures.
Table of contents
- Understanding Java Data Structures
- Arrays: The Bookshelf Story
- ArrayList: The Expanding Closet
- LinkedList: The Train Cars
- HashMap: The Name-Tag Game
- Stack: The Plate Pile
- Queue: The Movie Line
- Conclusion
Understanding Java Data Structures
Let us understand everything related to Java Data Structures:
1. Arrays: The Bookshelf Story
Imagine you have a bookshelf with 10 slots. You can place one book in each slot. This is what an array is in Java. It’s like a shelf where each book (or data element) has its own place. You can reach for the 3rd or 7th book directly because you know their exact position.
Code Example:
int[] numbers = {10, 20, 30, 40, 50}; // A bookshelf with 5 books
System.out.println(numbers[2]); // Access the 3rd book (array index starts at 0)
Story Twist: What if you want to add more books? You can’t just stretch the shelf. That’s the downside of arrays—they have a fixed size. If you want more flexibility, you need something more adaptable.
2. ArrayList: The Expanding Closet
Now, imagine you get a magical closet. It starts small, but whenever you need more space, it automatically grows to fit more clothes. ArrayList in Java works similarly. Unlike arrays, ArrayLists can expand as needed, adding more items without worrying about a fixed size.
Code Example:
import java.util.ArrayList;
ArrayList<String> closet = new ArrayList<>();
closet.add("Shirt");
closet.add("Pants");
closet.add("Shoes");
System.out.println(closet); // See the expanding closet
closet.add("Jacket"); // Add more without worrying about space
Story Insight: An ArrayList is like having a never-ending closet—very useful when you don’t know exactly how much data you’ll need to store.
3. LinkedList: The Train Cars
Think of a train. Each car is connected to the next, and you can add or remove cars easily without affecting the rest of the train. This is exactly how LinkedLists works. Each element (or car) knows the next one in line. You can add or remove elements without shifting everything like you would need to with an array.
Code Example:
import java.util.LinkedList;
LinkedList<String> train = new LinkedList<>();
train.add("Engine");
train.add("Car1");
train.add("Car2");
System.out.println(train); // A train with three cars
train.addFirst("NewEngine"); // Adding a new car to the front
Story Insight: LinkedLists are great when you want to add or remove data frequently like train cars being added or removed without disturbing the whole sequence.
4. HashMap: The Name-Tag Game
Imagine you’re at a party and everyone has a unique name tag. When you meet someone, you can immediately know their name by looking at their tag. HashMaps in Java work in a similar way. Each data value (like a name at the party) is paired with a unique key (the tag). You can retrieve any value just by knowing its key.
Code Example:
import java.util.HashMap;
HashMap<String, String> nameTags = new HashMap<>();
nameTags.put("Alice", "Engineer");
nameTags.put("Bob", "Designer");
System.out.println(nameTags.get("Alice"));
Output: Engineer
Story Insight: HashMaps makes looking up data quick and easy, like finding out what job Alice does just by looking at her name tag.
5. Stack: The Plate Pile
Imagine a stack of plates in your kitchen. You can only take the top plate off or put a new one on top. This is how Stacks work. You can only add or remove the last thing you placed (think “Last In, First Out” or LIFO).
Code Example:
import java.util.Stack;
Stack<String> plates = new Stack<>();
plates.push("Plate1");
plates.push("Plate2");
plates.push("Plate3");
System.out.println(plates.pop()); // Remove the top plate (Plate3)
Story Insight: Stacks are great for scenarios where you need to reverse things, like going back to the previous webpage you visited.
6. Queue: The Movie Line
Let’s say you’re in line for a movie. The first person to get in line will be the first person to enter the theater. Queues work like this. It’s “First In, First Out” (FIFO)—just like waiting in a line.
Code Example:
import java.util.LinkedList;
import java.util.Queue;
Queue<String> movieLine = new LinkedList<>();
movieLine.add("Person1");
movieLine.add("Person2");
movieLine.add("Person3");
System.out.println(movieLine.poll()); // Person1 gets the ticket first
Story Insight: Queues are perfect for things like managing tasks or processes where the first thing in is the first thing handled.
In case, you want to learn more about Java Full stack development and how to become one, consider enrolling for GUVI’s Certified Java Full-stack Developer Course that teaches you everything from scratch and make sure you master it!
Conclusion
In conclusion, these internal data structures in Java are like the tools you use in everyday life—a bookshelf for organizing, a closet that grows, train cars that connect, name tags for easy identification, plate stacks in the kitchen, and waiting in line for a movie.
Each data structure has a specific job and shines in different scenarios. Understanding how they work can make your coding life much smoother, and knowing when to use each one will make your programs faster and more efficient.
Next time you code, think about which data structure fits your story!
Did you enjoy this article?