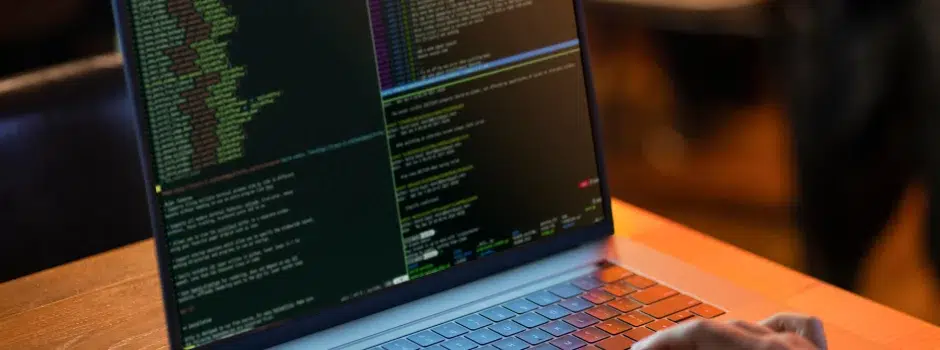
10 Java Performance Optimization Tips for Efficient Code
Jan 28, 2025 3 Min Read 433 Views
(Last Updated)
In high-load applications, optimizing performance is key to ensuring efficient and responsive Java code. One area where performance can significantly impact your application is in the handling of strings, memory management, data structures, and concurrent operations. By following practical tips like using StringBuilder for concatenation, leveraging primitive types over wrappers, choosing efficient data structures, utilizing lazy initialization, and employing caching strategies, you can greatly enhance the performance of your Java applications.
Additionally, optimizing loops, avoiding unnecessary object creation, and being mindful of synchronization will contribute to building smoother, faster, and more scalable Java solutions.
Optimizing performance in Java can be crucial, especially for high-load applications. Here are ten practical performance tips that can make your Java code more efficient and responsive:
Table of contents
- Optimize String Handling
- Leverage Primitives Over Wrappers
- Minimize Memory Usage with Efficient Data Structures
- Use Lazy Initialization
- Utilize Caching for Repeated Computations
- Avoid Unnecessary Object Creation
- Optimize Loops and Avoid Redundant Computations
- Use Streams and Lambdas Judiciously
- Minimize Synchronized Blocks and Use Concurrency Primitives
- Profile and Optimize Hot Spots
- Summary Table
- Final Thoughts
- Frequently Asked Questions
- Why is Java performance optimization important?
- How can I minimize object creation to improve performance?
- How can I optimize loops in Java for better performance?
1. Optimize String Handling
Use StringBuilder for Concatenation: String concatenation (+
) in loops can lead to a performance hit due to immutable String
objects. Use StringBuilder
or StringBuffer
for building strings in loops.
Avoid Repetitive toLowerCase
or toUpperCase
Calls: These methods can be expensive. Store precomputed values if you need case-insensitive comparisons.
java
Copy code
// Instead of
String result = "";
for (String s : strings) {
result += s; // creates multiple String objects
}
// Use
StringBuilder result = new StringBuilder();
for (String s : strings) {
result.append(s);
}
2. Leverage Primitives Over Wrappers
- Using primitive types (e.g.,
int, double
) instead of their wrapper classes (Integer, Double
) can reduce memory overhead and speed up operations.
- Wrappers introduce additional autoboxing/unboxing operations, which slow down performance and increase memory usage.
java
Copy code
// Prefer
int sum = 0;
// Over
Integer sum = 0; // Autoboxing/unboxing with each operation
3. Minimize Memory Usage with Efficient Data Structures
- Choose data structures that are optimal for the task. For instance, use an
ArrayList
when you need fast access by index, andLinkedList
only for frequent insertion/deletion operations.
- Avoid using
HashMap
with small collections if aList
orArray
will do the job, asHashMap
requires more memory.
java
Copy code
// If you have a small, fixed number of elements
int[] items = new int[3]; // Array is more memory-efficient than ArrayList
4. Use Lazy Initialization
- Only create objects or initialize variables when necessary. This can help avoid wasted memory and improve startup time.
- For expensive objects that may not always be used, consider lazy initialization to delay creation until actually needed.
java
Copy code
private ExpensiveObject obj = null;
public ExpensiveObject getObj() {
if (obj == null) { // Lazily initialize when first accessed
obj = new ExpensiveObject();
}
return obj;
}
5. Utilize Caching for Repeated Computations
- Cache results of expensive or frequently accessed computations to avoid recalculating them repeatedly.
- Use caching libraries like Caffeine or Guava for large caches, or just a
Map
for simple cases.
java
Copy code
private Map<Integer, Long> factorialCache = new HashMap<>();
public long factorial(int n) {
if (factorialCache.containsKey(n)) return factorialCache.get(n);
long result = computeFactorial(n); // assume this is an expensive method
factorialCache.put(n, result);
return result;
}
6. Avoid Unnecessary Object Creation
- Reuse objects where possible instead of creating new ones repeatedly, especially in frequently called methods.
- For example, avoid creating new
DateFormat
orPattern objects
within a loop, as they are expensive. Consider creating them once and reusing.
java
Copy code
private static final Pattern PATTERN = Pattern.compile("pattern");
public boolean match(String input) {
return PATTERN.matcher(input).matches();
}
7. Optimize Loops and Avoid Redundant Computations
- Move invariant computations outside of loops.
- Minimize work done inside loops, and avoid redundant operations such as repeatedly checking conditions that don’t change during iteration.
java
Copy code
// Instead of recalculating list.size() in every iteration
for (int i = 0; i < list.size(); i++) { ... }
// Use
int size = list.size();
for (int i = 0; i < size; i++) { ... }
8. Use Streams and Lambdas Judiciously
- Streams and lambdas can be very convenient, but they may add overhead in performance-sensitive code, particularly in tight loops or hot paths.
- For simple tasks or small datasets, traditional loops are often faster than Streams.
java
Copy code
// Instead of using a stream for a simple sum
int sum = list.stream().mapToInt(Integer::intValue).sum();
// Consider a traditional loop
int sum = 0;
for (int i : list) sum += i;
9. Minimize Synchronized Blocks and Use Concurrency Primitives
- Synchronization can introduce thread contention and slow down performance. Keep synchronized blocks short, and use them only where absolutely necessary.
- For managing concurrency, consider alternatives like
ReadWriteLock
,Atomic
classes (likeAtomicInteger
), orConcurrentHashMap
for performance-sensitive code.
java
Copy code
// Instead of synchronized access
private final AtomicInteger counter = new AtomicInteger(0);
public int incrementAndGet() {
return counter.incrementAndGet(); // Non-blocking atomic increment
}
10. Profile and Optimize Hot Spots
- Use profiling tools (like VisualVM, JProfiler, or YourKit) to identify bottlenecks in your application rather than making assumptions about what might be slow.
- Focus on optimizing the “hot spots” that the profiler identifies as taking the most time. Often, only a small portion of the code needs optimization for significant performance gains.
Summary Table
Performance Tip | Summary |
String Handling | Use StringBuilder for concatenation, avoid repetitive case transformations |
Use Primitives | Favor primitives over wrappers to save memory and improve speed |
Efficient Data Structures | Select data structures best suited for the task (e.g., Array vs. ArrayList) |
Lazy Initialization | Initialize resources only when needed |
Caching | Cache results of expensive operations |
Avoid Unnecessary Objects | Reuse objects and avoid creating new ones repeatedly |
Optimize Loops | Minimize operations within loops; cache loop-invariant values |
Use Streams Judiciously | For high-performance code, consider avoiding streams in hot paths |
Minimize Synchronization | Keep synchronized blocks short and use atomic classes when possible |
Profile Code | Use a profiler to find real bottlenecks and optimize hotspots |
Unlock your potential as a Java Full-Stack Developer with our comprehensive Java Full-Stack development course! Dive deep into the world of Java, mastering front-end and back-end development to build powerful, dynamic web applications. Gain hands-on experience with essential tools and frameworks like Spring Boot, Hibernate, Angular, and React, all while learning best practices for performance optimization and scalable coding. Start your journey today and become the all-in-one developer every company is searching for!
Final Thoughts
Efficient Java code improves performance and ensures better scalability, maintainability, and user satisfaction. By adopting techniques such as using StringBuilder
, leveraging primitives, choosing optimal data structures, implementing lazy initialization, and employing effective caching, you can fine-tune your applications for better speed and responsiveness.
Furthermore, minimizing synchronization, optimizing loops, and profiling your code will help identify bottlenecks and make impactful performance improvements. With thoughtful optimization, your Java applications can perform at their best, delivering a seamless user experience and maintaining long-term efficiency.
Frequently Asked Questions
Optimizing Java performance enhances application efficiency, reduces resource consumption, and improves user experience. Efficient code leads to faster execution times and better scalability.
To enhance loop performance:
– Minimize computations within the loop.
– Use efficient looping constructs, such as iterating over collections using enhanced for-loops.
– Consider parallel processing for large datasets using the Streams API.
Did you enjoy this article?