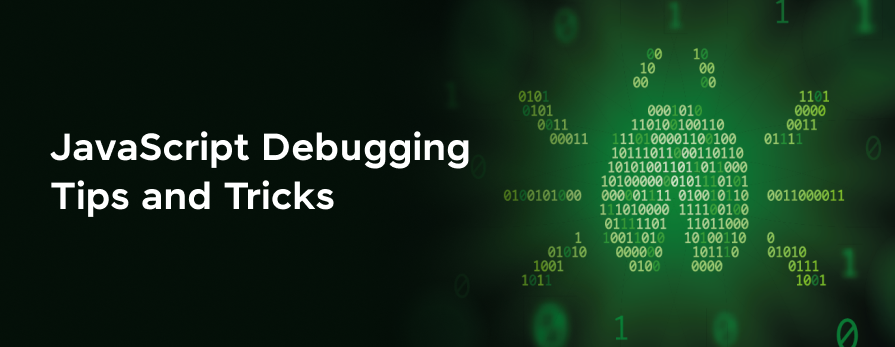
As a JavaScript developer, you’ve likely encountered your fair share of bugs and debugging challenges. Debugging can quickly become a daunting task, especially in complex applications. However, with the right tools and techniques, you can streamline the debugging process and learn the secrets hidden within your code.
In this blog, we’ll explore a wide range of JavaScript debugging tips and tricks that will enhance your debugging skills and help you tackle even the most stubborn bugs with confidence. From using built-in browser tools to mastering advanced debugging techniques, this blog post will equip you with the knowledge and strategies you need to become a true debugging expert.
Table of contents
- What is Debugging?
- JavaScript Debugging Tips and Tricks
- Setting Up the Debugging Environment
- Using Browser Developer Tools
- Utilizing Console Methods
- Debugging Techniques
- Handling Asynchronous Code
- Debugging Tools and Extensions
- Conclusion
- FAQs
- How do I start debugging my JavaScript code if it's not working?
- What's the best approach to debug asynchronous JavaScript code?
- What are some key practices for efficient JavaScript debugging?
What is Debugging?
Debugging is the process of identifying, locating, and fixing bugs or defects in computer software or hardware systems. A bug is an error, flaw, or fault in a computer program or system that causes it to produce unexpected or incorrect results, crash, or behave in an unintended way.
In the context of software development, debugging involves several steps:
- Problem Identification: The first step is to recognize that there is a problem or bug in the code. This can be done by observing unexpected behavior, error messages, or incorrect output during testing or actual use of the software.
- Reproduction: Once a bug has been identified, the next step is to reproduce it consistently. This involves creating a set of steps or conditions that reliably trigger the bug, making it easier to investigate and diagnose the root cause.
- Cause Identification: With the bug consistently reproducible, developers can start examining the code, variable values, execution flow, and other relevant information to pinpoint the specific cause of the issue. This often involves techniques like code tracing, logging, breakpoints, and step-through debugging.
- Solution Development: After identifying the root cause of the bug, developers can work on developing a solution or fix. This may involve modifying the existing code, refactoring portions of the codebase, or introducing new code to address the issue.
- Testing and Verification: Once a potential solution has been implemented, it must be thoroughly tested to ensure that the bug has been fixed and that no new issues have been introduced. This may involve running unit tests, integration tests, and other verification processes.
- Documentation and Knowledge Sharing: Finally, it’s important to document the bug, its cause, and the solution implemented. This documentation can serve as a reference for future debugging efforts and help prevent similar issues from occurring again. Additionally, sharing this knowledge with the development team can promote collective learning and improve overall coding practices.
Effective debugging requires a combination of technical skills, problem-solving abilities, attention to detail, and a deep understanding of the programming language, frameworks, and tools being used. It is an essential part of the software development lifecycle and contributes significantly to the overall quality and reliability of software applications.
Ready to master full-stack development? Enroll now and enhance your career with the IIT Kanpur Full Stack Development Certification course!
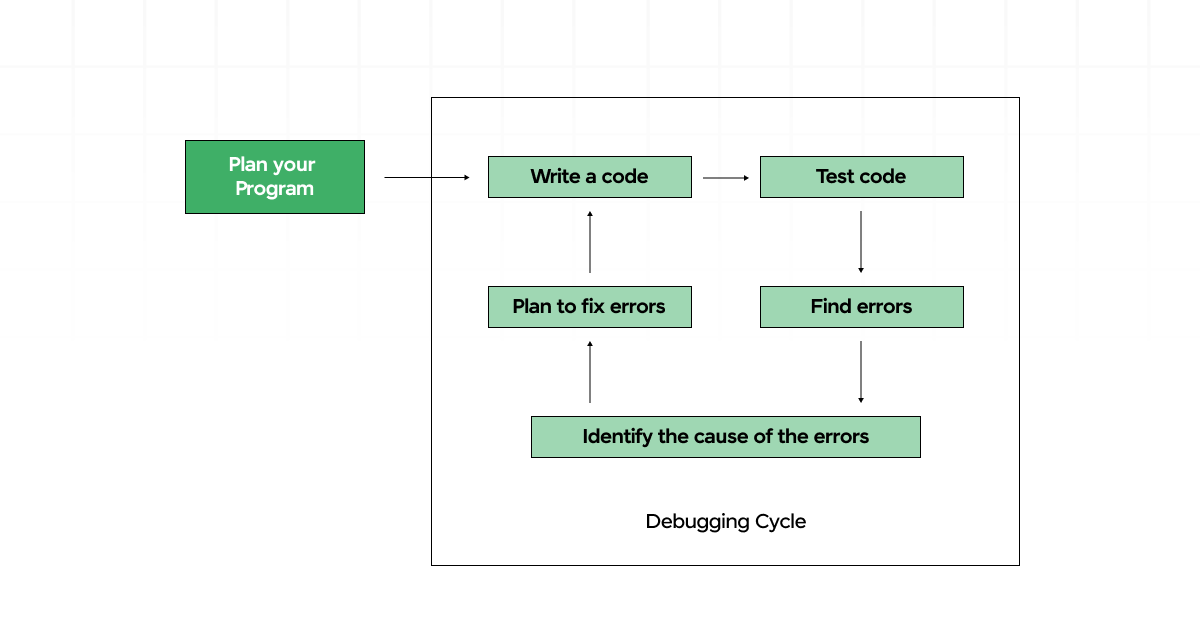
Now that we understand what debugging is, let’s learn some essential JavaScript debugging tips and tricks to help you get through coding challenges with ease.
JavaScript Debugging Tips and Tricks
Let’s explore some essential tips and tricks to enhance your JavaScript debugging skills:
1. Setting Up the Debugging Environment
Before debugging, it’s important to ensure your development environment is properly configured. This includes setting up your IDE, integrating debugging tools, and familiarizing yourself with relevant settings.
- IDE configuration for seamless debugging experience.
- Integration of debugging tools such as browser developer tools or IDE extensions.
- Customization of debugging settings to suit your workflow.
- Installation of necessary dependencies for debugging, such as linters or code validators.
- Documentation or resources to troubleshoot common setup issues.
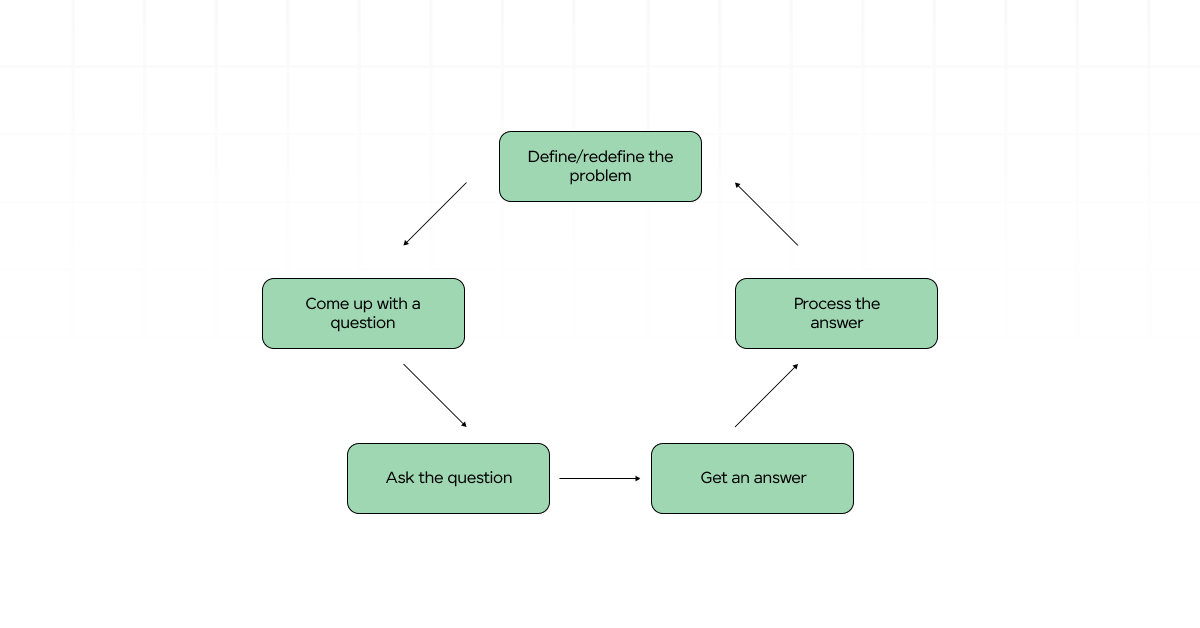
Also Read: 30 Best JavaScript Project Ideas For You [3 Bonus Portfolio Projects]
2. Using Browser Developer Tools
Browser developer tools offer features to aid in web development, including robust debugging capabilities. These tools allow you to inspect HTML, CSS, and JavaScript, debug code, analyze performance, and monitor network activity.
- Code inspection for identifying HTML, CSS, and JavaScript elements.
- JavaScript debugging tools such as breakpoints, step-through execution, and watch expressions.
- Performance profiling to identify bottlenecks and optimize code.
- Network monitoring to debug AJAX requests and track resource loading.
- Accessibility auditing and testing tools to ensure inclusive design practices.
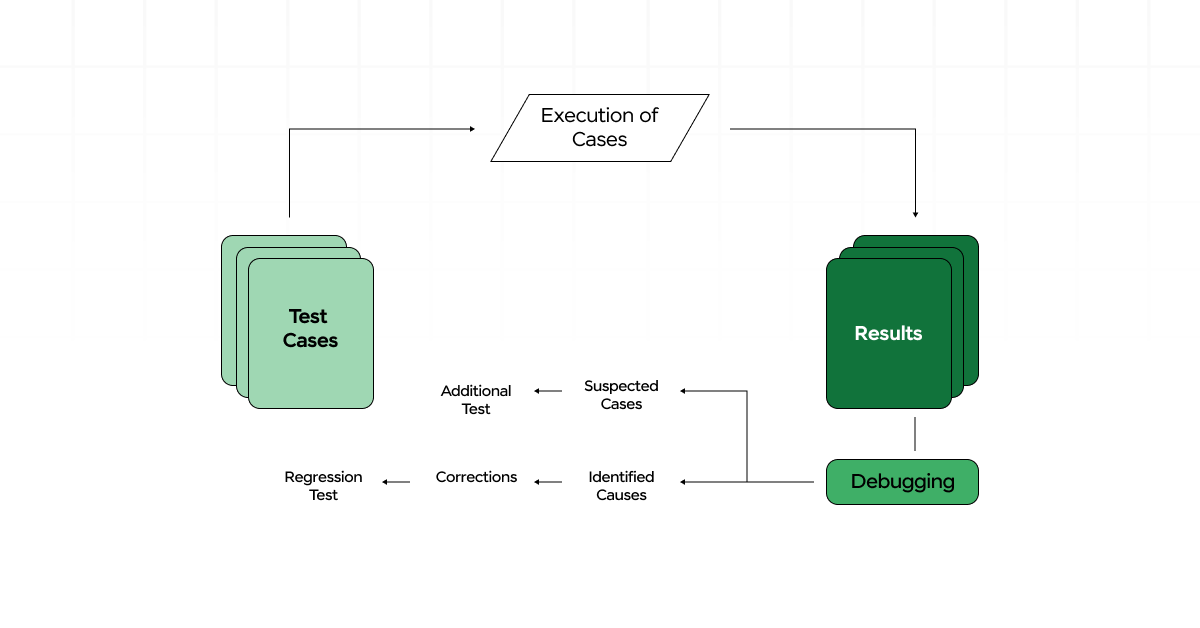
Also Read: 4 Key Differences Between == and === Operators in JavaScript
3. Utilizing Console Methods
The console object in JavaScript provides a range of methods for logging messages, errors, warnings, and other information to the browser console. These methods are important for understanding code behavior, tracking variable values, and identifying errors.
- Logging messages with console.log() for debugging purposes.
- Highlighting errors and warnings using console.error() and console.warn().
- Conditional logging with console.assert() to validate assumptions.
- Formatting log messages with placeholders and string substitutions.
- Grouping related log messages for better organization using console.group().
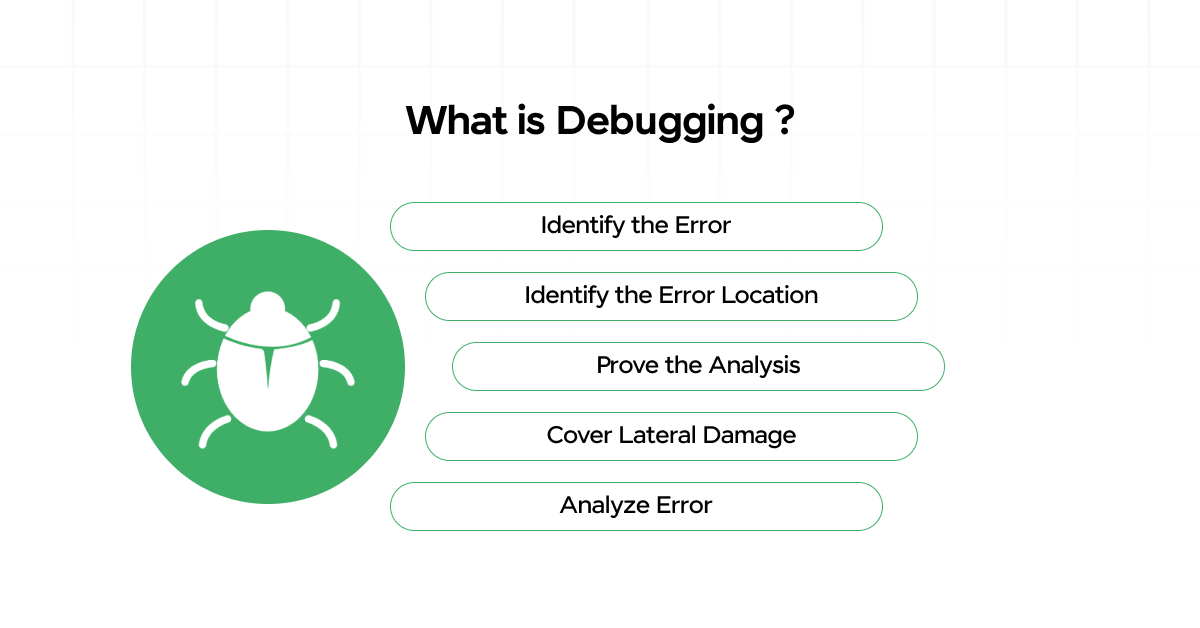
Also Read: Arrays in JavaScript: A Comprehensive Guide
4. Debugging Techniques
Effective debugging techniques involve strategies for isolating and resolving issues in code efficiently. These techniques include setting breakpoints, stepping through code execution, using watch expressions, and employing conditional breakpoints.
- Setting breakpoints at specific lines of code to pause execution.
- Stepping through code execution to understand flow and behavior.
- Watching variable values in real-time with watch expressions.
- Pausing execution based on specified conditions with conditional breakpoints.
- Utilizing debugging shortcuts and keyboard commands for faster navigation.
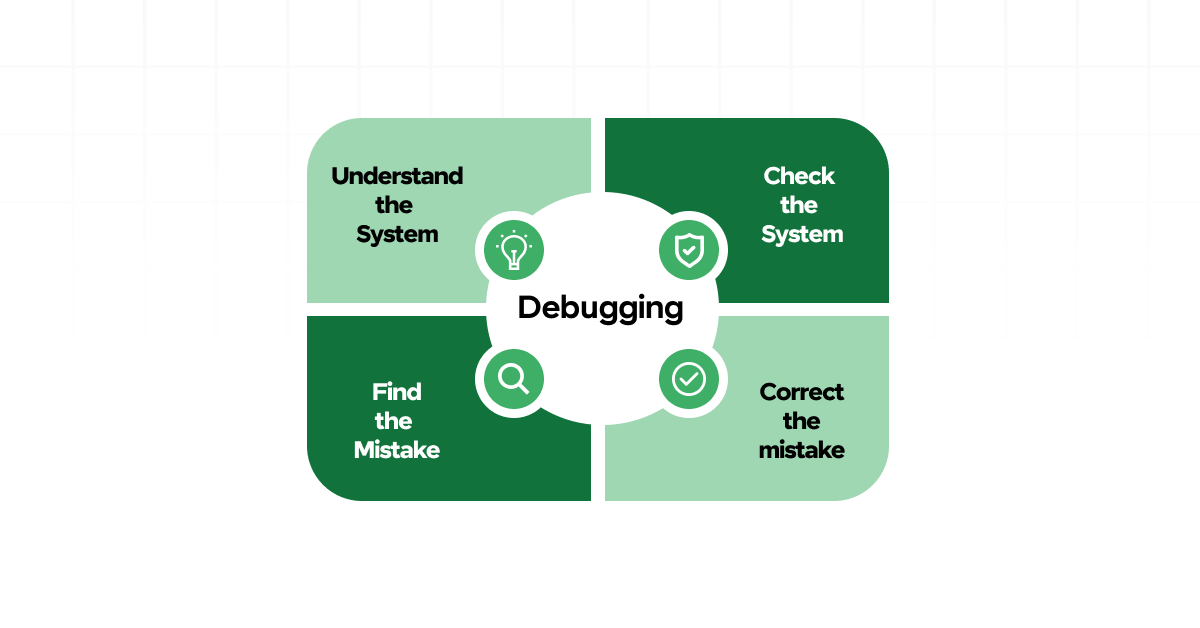
5. Handling Asynchronous Code
Asynchronous JavaScript code, such as promises, async/await functions, and event-driven code, introduces unique challenges for debugging. It’s essential to understand how to trace asynchronous execution, handle errors gracefully, and use tools for better insight into asynchronous behavior.
- Debugging promises and async/await functions with browser developer tools.
- Utilizing async stack traces for tracing asynchronous execution paths.
- Handling errors in asynchronous code using try-catch blocks or promise.catch().
- Debugging event-driven code with event listeners and callback functions.
- Identifying and resolving common pitfalls in asynchronous programming, such as race conditions and callback hell.
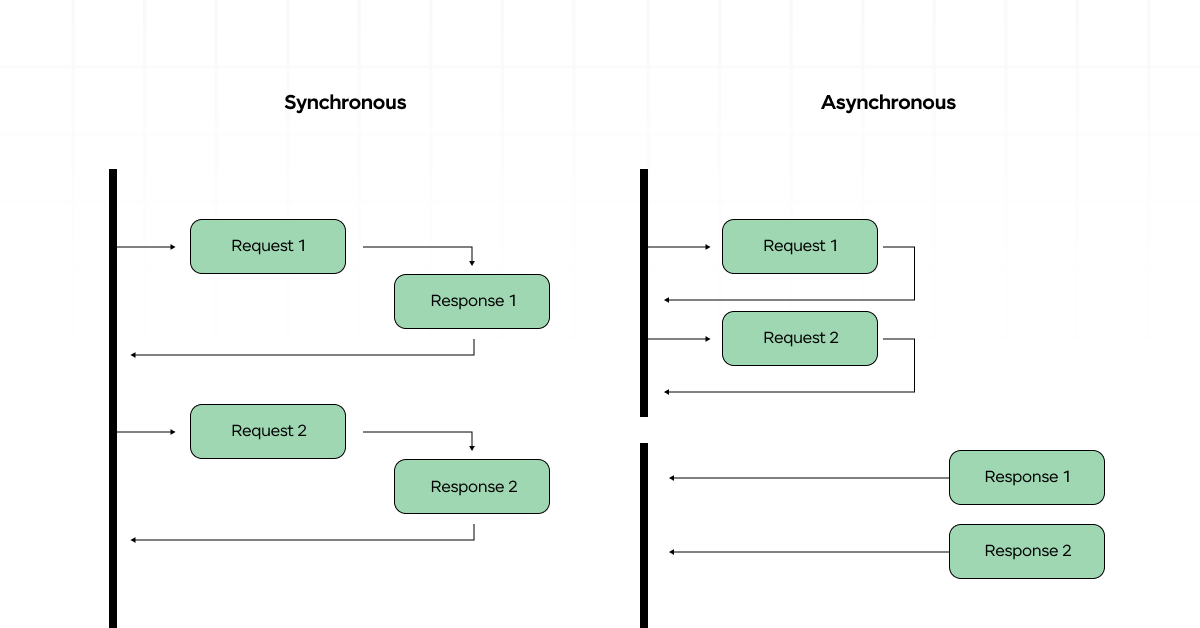
Also Read: Functions in JavaScript: Important Things To Know [2024]
6. Debugging Tools and Extensions
In addition to browser developer tools, there exists many third-party debugging tools and browser extensions specifically tailored for JavaScript development. These tools offer enhanced debugging features, code analysis, performance profiling, and workflow improvements.
- Integration with popular code editors such as Visual Studio Code or Sublime Text.
- Advanced debugging features including breakpoints, watch expressions, and call stack inspection.
- Performance profiling tools for identifying bottlenecks and optimizing code.
- Code analysis capabilities for detecting potential issues, such as syntax errors or unused variables.
- Workflow enhancements such as live reloading, code snippets, and Git integration for seamless collaboration.
By mastering these JavaScript debugging tips and tricks, you’ll be better equipped to tackle bugs and errors in your code, leading to smoother development cycles and higher-quality software products.
Don’t miss out on the opportunity to become a certified full-stack developer with the IIT Kanpur Full Stack Development Certification course. Join today and take your skills to the next level!
Conclusion
JavaScript debugging is ongoing and ever-evolving. Embrace the spirit of continuous learning and improvement by staying curious, experimenting with new techniques, and seeking out opportunities to enhance your debugging skills. There’s always more to discover and learn about JavaScript debugging.
FAQs
How do I start debugging my JavaScript code if it’s not working?
Begin by using browser developer tools to inspect for errors, utilize console logging, and set breakpoints to trace code execution and variable values.
What’s the best approach to debug asynchronous JavaScript code?
Debugging asynchronous code involves using browser tools to monitor promise resolution, employing try-catch blocks for error handling, and using async stack traces for insight into code behavior.
What are some key practices for efficient JavaScript debugging?
Write modular and well-documented code, utilize version control systems, collaborate with team members, and continuously improve debugging skills through practice and learning from resources.
Did you enjoy this article?