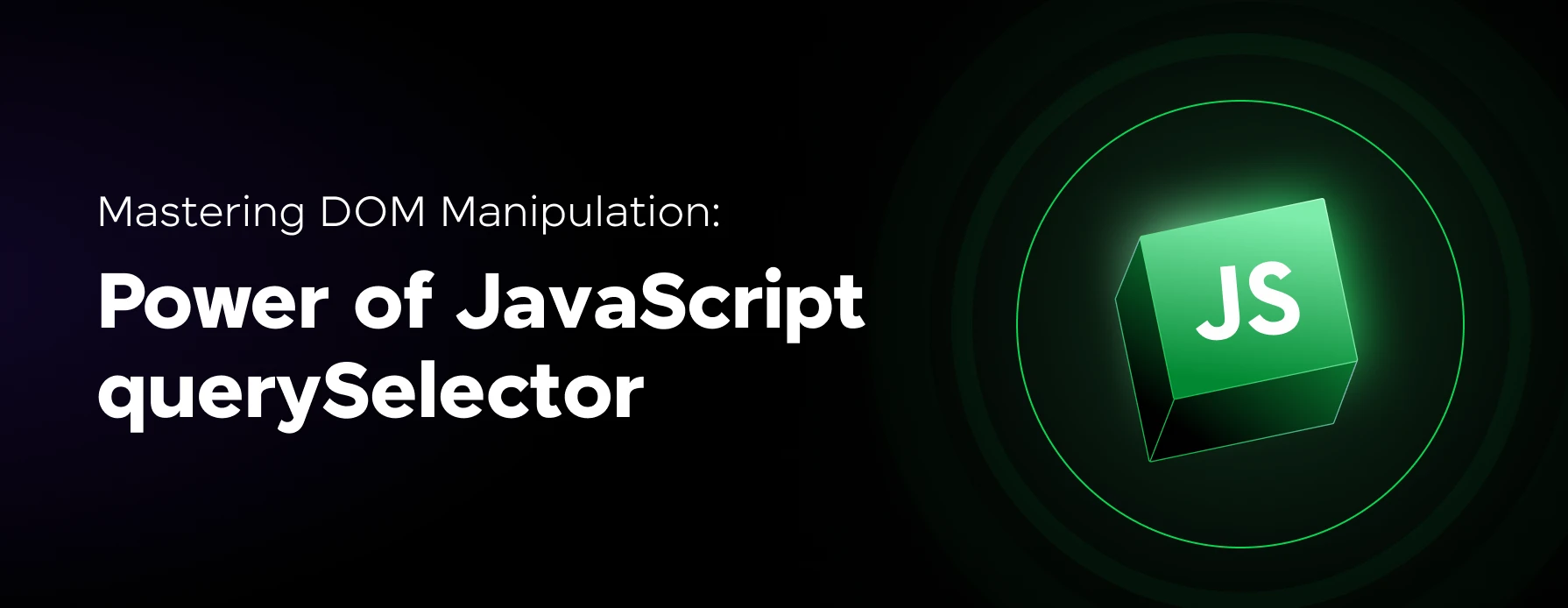
Mastering DOM Manipulation: Power of JavaScript querySelector
Mar 19, 2025 5 Min Read 3262 Views
(Last Updated)
In the world of web development, DOM (Document Object Model) manipulation plays a crucial role in creating dynamic and interactive websites. With DOM manipulation, you can access, modify, and manipulate the elements and content of a web page using JavaScript.
One powerful method that enables DOM manipulation is JavaScript querySelector
method. In this blog, we will explore the importance of DOM manipulation in web development and provide an overview of the querySelector
method in JavaScript.
Table of contents
- Importance of DOM Manipulation in Web Development
- Overview of JavaScript querySelector Method
- Understanding querySelector Basics
- Syntax and Usage of querySelector
- Leveraging querySelector for Dynamic Content
- Modifying Element Content with querySelector
- Manipulating Styles and Classes
- Event Handling with querySelector
- Adding Event Listeners with querySelector
- Responding to User Interactions
- Wrap Up
Importance of DOM Manipulation in Web Development
DOM manipulation is a fundamental skill that every web developer should possess. It allows you to dynamically update the content, structure, and styling of a web page, providing a seamless and interactive user experience. By manipulating the DOM, you can respond to user actions, modify elements on the fly, and create dynamic web applications.
Whether you want to update the text of a heading, change the background color of a button on click, or dynamically add new elements to a page, DOM manipulation is the key. It empowers you to create modern, interactive websites that engage and captivate users. Understanding and mastering DOM manipulation is essential for any developer looking to build robust and dynamic web applications.
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Course with Placement Assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Additionally, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript certification course.
Overview of JavaScript querySelector Method
The querySelector
method is a powerful tool in JavaScript that allows you to select and manipulate elements in the DOM using CSS selectors. With querySelector
, you can access individual elements or groups of elements that match a specified CSS selector.
One of the key advantages of querySelector
is its flexibility. It enables you to select elements based on their tag name, class name, or even specific attributes. This makes it incredibly versatile and allows you to target specific elements within the DOM with ease.
To use querySelector
, you simply pass in a CSS selector as a parameter, and it will return the first matching element it encounters. If you want to select multiple elements that match a selector, you can use the querySelectorAll
method instead.
// Selecting a single element using querySelector
const element = document.querySelector('.my-element');
// Selecting multiple elements using querySelectorAll
const elements = document.querySelectorAll('.my-elements');
By combining the power of JavaScript and the querySelector
method, you can manipulate the DOM to create dynamic and interactive web pages. In the following sections, we will explore different aspects of querySelector
and how it can be leveraged for various DOM manipulation tasks.
Now that we have established the importance of DOM manipulation in web development and introduced the querySelector
method, let’s delve deeper into its syntax and usage in the next section.
Understanding querySelector Basics
To harness the power of DOM manipulation in JavaScript, it’s essential to have a solid understanding of the querySelector method. This method allows you to select and manipulate elements in the Document Object Model (DOM) using CSS selectors. In this section, we will explore the syntax and usage of querySelector and how to select elements using CSS selectors.
Syntax and Usage of querySelector
The syntax for querySelector is straightforward. It takes a CSS selector as a parameter and returns the first element that matches the selector. Here’s the basic syntax:

const element = document.querySelector(selector);
document
refers to the Document object, which represents the web page itself.selector
is a valid CSS selector that specifies the element(s) you want to select.
The querySelector method returns the first element that matches the selector. If no elements are found, it returns null
.
Also Read: How to Master DOM in Selenium for Beginners
Leveraging querySelector for Dynamic Content
When working with dynamic web content, the JavaScript querySelector
method is a powerful tool for manipulating elements in the Document Object Model (DOM). It allows you to select and modify specific elements on a web page, making it easier to create interactive and engaging user experiences. In this section, we will explore how you can leverage querySelector
to modify element content and manipulate styles and classes.
Modifying Element Content with querySelector
With querySelector
, you can easily modify the content of HTML elements. Whether you want to update the text within a paragraph or change the value of an input field, querySelector
provides a flexible way to accomplish these tasks.
To modify the content of an element, you first need to select it using a CSS selector. Once selected, you can access the innerHTML
property of the element to change its content. For example, let’s say you have a <p>
element with the id “myParagraph” and you want to change its text:
const paragraph = document.querySelector("#myParagraph");
paragraph.innerHTML = "New text for the paragraph";
By assigning a new value to innerHTML
, you can update the content of the selected element dynamically. This technique is particularly useful when you want to display updated information or provide feedback to the user in real-time.
Manipulating Styles and Classes
In addition to modifying content, querySelector
enables you to manipulate the styles and classes of elements to enhance the visual presentation of your web page. You can change the appearance of elements, toggle classes, or add/remove specific styles based on user interactions or other events.
To manipulate styles, you can access the style
property of an element selected with querySelector
. This property allows you to modify individual CSS properties directly. For example, let’s say you have a button with the class “myButton” and you want to change its background color:
const button = document.querySelector(".myButton");
button.style.backgroundColor = "red";
By assigning a new value to the desired CSS property, you can dynamically update the style of the selected element.
Similarly, you can also manipulate classes using querySelector
. The classList
property provides methods to add, remove, or toggle classes on an element. This is particularly useful when you want to apply different styles or trigger specific behaviors based on user interactions. For example, let’s say you have a <div>
element with the id “myDiv” and you want to toggle a class named “highlight” when the user clicks on it:
const div = document.querySelector("#myDiv");
div.addEventListener("click", function() {
div.classList.toggle("highlight");
});
In this example, the toggle
method is used to add or remove the “highlight” class whenever the user clicks on the <div>
element. This allows you to create interactive effects and provide visual feedback to the user.
By leveraging querySelector
to modify element content, styles, and classes, you can dynamically update your web page and create engaging user experiences. Remember to use the appropriate CSS selectors to target the desired elements and explore other DOM manipulation techniques to expand your JavaScript skills.
Do check out Understanding and Preventing Memory Leaks in JavaScript Applications.
Event Handling with querySelector
When working with JavaScript and DOM manipulation, event handling is a crucial aspect that allows you to respond to user interactions and create dynamic and interactive web experiences. The querySelector
method in JavaScript provides a powerful tool for adding event listeners and responding to user interactions.
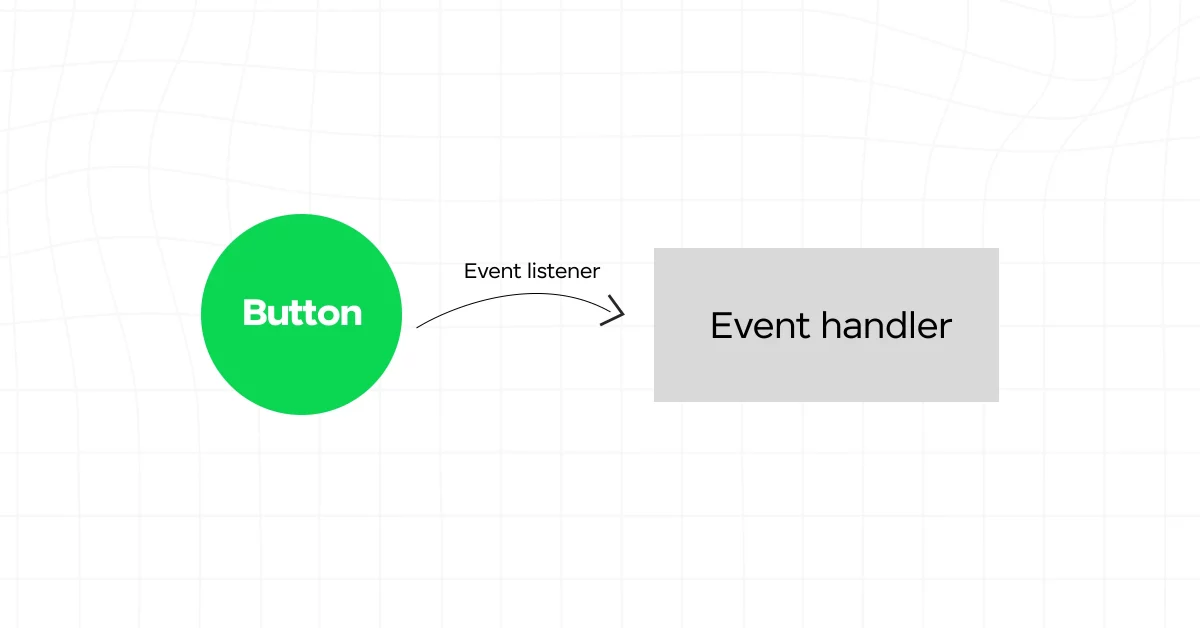
Adding Event Listeners with querySelector
To add an event listener using querySelector
, you first need to select the element(s) to which you want to attach the listener. The querySelector
method allows you to select elements based on CSS selectors, making it flexible and versatile.
Here’s an example of how you can add an event listener to a button element with the id “myButton” using querySelector
:
const button = document.querySelector("#myButton");
button.addEventListener("click", function() {
// Code to be executed when the button is clicked
});
In this example, the querySelector
method selects the button element with the id “myButton”. The addEventListener
method is then used to attach a “click” event listener to the button. When the button is clicked, the provided callback function will be executed.
Also, look at the JavaScript frontend development roadmap which gives you a detailed summary of how to understand the basics to advanced topics.
Responding to User Interactions
Once you have added an event listener using querySelector
, you can respond to various user interactions such as clicks, mouse movements, key presses, and more. The callback function you provide as the second argument to addEventListener
is where you define the actions you want to take when the event occurs.
For example, let’s say you have a form with an input field and a submit button. You can use querySelector
to select the form and attach an event listener to it to handle the form submission:
const form = document.querySelector("form");
form.addEventListener("submit", function(event) {
event.preventDefault(); // Prevents the default form submission
// Code to handle the form submission
});
In this case, when the form is submitted, the callback function will be executed. The preventDefault()
method is called to prevent the form from being submitted in the default manner. You can then write code to handle the form submission, such as validating input, making AJAX requests, or updating the DOM.
By leveraging the power of event handling with querySelector
, you can create dynamic and interactive web applications that respond to user interactions. Remember to use proper event delegation techniques and consider efficiency when adding multiple event listeners. For more information on DOM manipulation with JavaScript, you can refer to our article on DOM Manipulation with JavaScript.
In the next section, we will explore more advanced techniques with querySelector
and discuss how to traverse the DOM tree and work with parent and child elements.
Kickstart your Full Stack Development journey by enrolling in GUVI’s certified Full Stack Development Course with Placement Assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements.
Alternatively, if you want to explore JavaScript through a self-paced course, try GUVI’s JavaScript course.
Wrap Up
By taking these steps to ensure cross-browser compatibility, you can reach a wider audience and provide a seamless experience for users regardless of their chosen browser.
Remember, optimizing performance and ensuring cross-browser compatibility are crucial aspects of DOM manipulation using the querySelector
method. By following best practices, you can write efficient and reliable code that meets the needs of your users.
Did you enjoy this article?