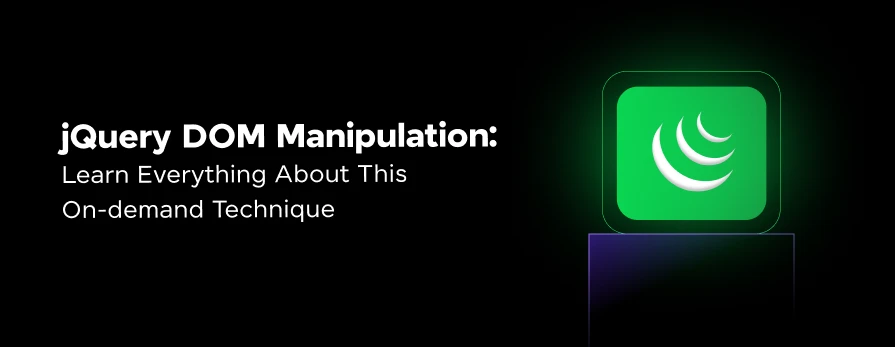
jQuery DOM Manipulation: Learn Everything About This On-demand Technique
Dec 31, 2024 6 Min Read 2622 Views
(Last Updated)
In full-stack development, knowing how to mess with the DOM (Document Object Model) is a game-changer. It lets you tweak the content, layout, and style of your web pages on the fly. This means you can make your site more interactive and fun for users.
Whether you’re updating text, changing attributes, or handling events, getting the hang of jQuery DOM manipulation is key to building cool, modern websites.
Table of contents
- Introduction to jQuery DOM Manipulation
- Why jQuery DOM Manipulation Matters in Full-Stack Development?
- Why Use jQuery for DOM Manipulation?
- Picking Elements in jQuery DOM Manipulation
- Using Selectors in jQuery
- Zeroing In on Specific Elements with jQuery
- Tweaking Elements with jQuery
- Changing Text Content
- Updating Attributes and Classes
- Jazzing Up Your Web Page with jQuery DOM Manipulation
- Tweaking CSS on the Fly
- Bringing Elements to Life with Animation
- Handling Events in jQuery DOM Manipulation
- Binding Event Handlers
- Responding to User Interactions
- Advanced jQuery DOM Manipulation Tricks
- Moving Around the DOM
- Making Dynamic Content with jQuery
- Conclusion
- FAQs
- Why is jQuery popular for DOM manipulation?
- What is the difference between $(this) and this in jQuery?
- What is the difference between .html() and .text() in jQuery?
- Is it possible to extend jQuery with plugins?
Introduction to jQuery DOM Manipulation
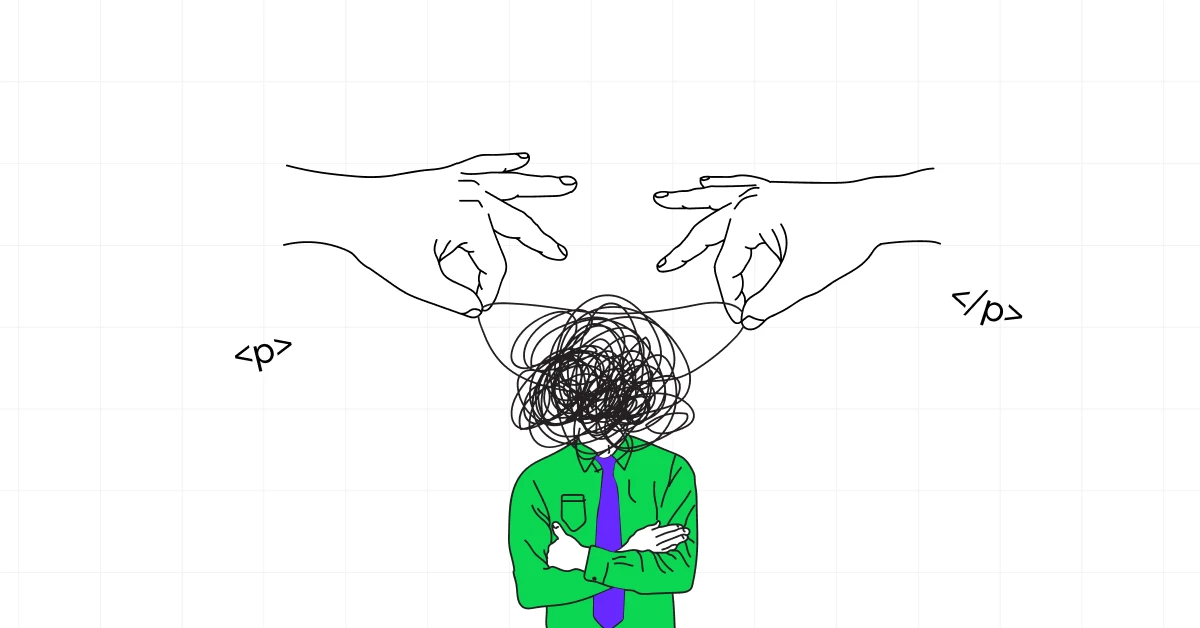
Let us first understand what jQuery DOM manipulation is. To put it simply, it is all about tweaking the structure, content, or style of a webpage using JavaScript. Think of the DOM as a tree where each branch is an HTML element.
Here’s what you can do with DOM manipulation:
- Pick elements
- Change elements (like text or attributes)
- Tweak CSS (add/remove classes, change styles)
- Handle events (like clicks and hovers)
Sure, you can use plain JavaScript for this, but jQuery DOM Manipulation makes it a breeze.
Learn: 7 Best JavaScript Practices Every Developer Must Follow
Why jQuery DOM Manipulation Matters in Full-Stack Development?
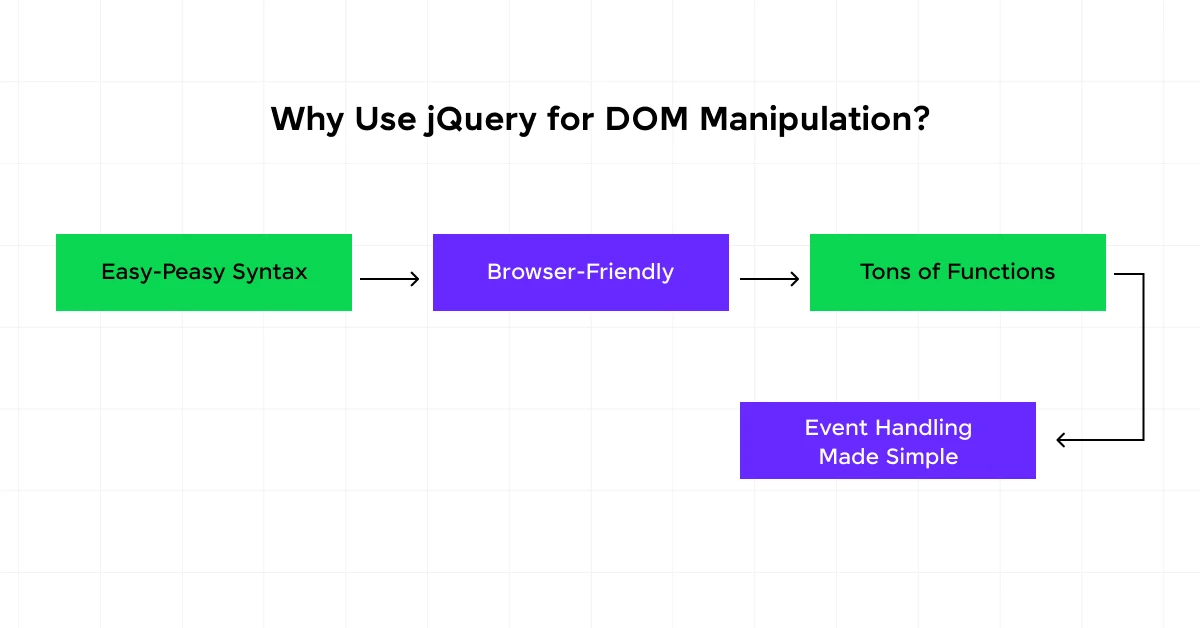
You learned the basics of jQuery DOM Manipulation in the previous section, now it is time for you to understand why it is important in full-stack development.
But before we go any further, it is important that you have a basic understanding of backend and full-stack development. If not, consider enrolling for a professionally certified online Full-stack Development Course that teaches you everything about backend frameworks and helps you get started as a developer.
Let us see why jQuery DOM Manipulation is very important in full-stack development:
Why Use jQuery for DOM Manipulation?
jQuery is like an all-rounder of JavaScript libraries, making jQuery DOM manipulation a breeze. Here’s why jQuery rocks:
- Easy-Peasy Syntax: jQuery’s syntax is way simpler and shorter than plain old JavaScript. This makes your code easier to write and keep track of.
- Browser-Friendly: jQuery smooths out the quirks between different browsers, so your code works everywhere without you pulling your hair out.
- Tons of Functions: jQuery comes packed with functions for picking, changing, and styling DOM elements. This speeds up your development big time.
- Event Handling Made Simple: jQuery has solid methods for binding and managing events, so you can easily react to what users do on your site.
Using jQuery, you can quickly and efficiently mess with the DOM and build dynamic web apps.
Explore: jQuery Selectors: An Informative Guide On The Most Essential Framework
Picking Elements in jQuery DOM Manipulation
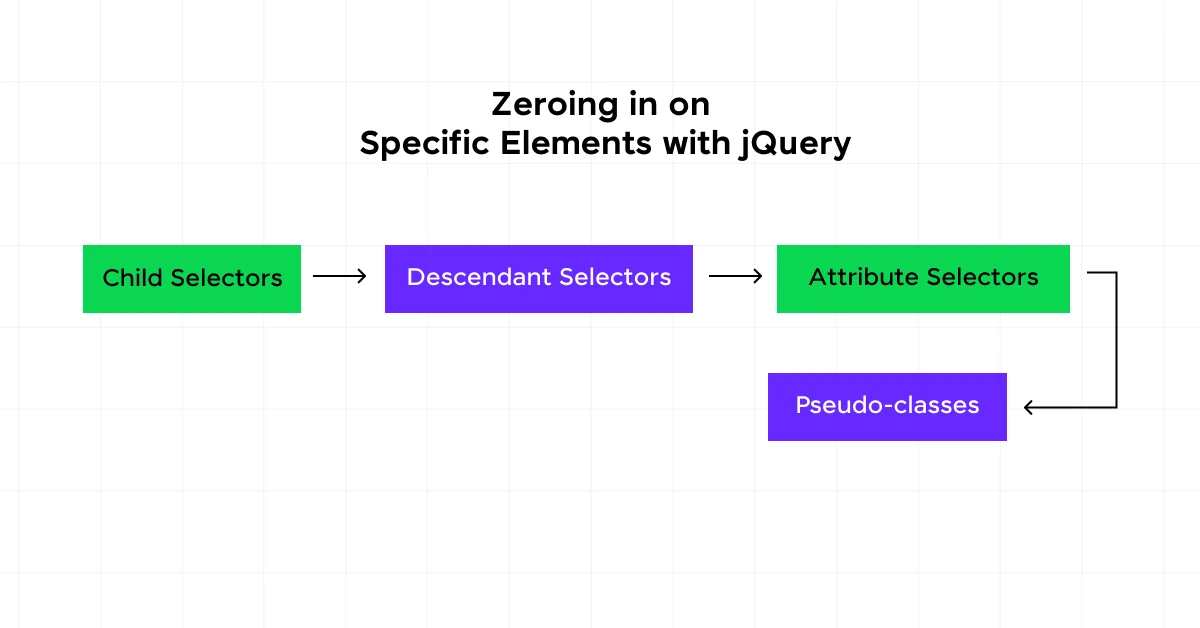
Picking elements is the first move in jQuery DOM manipulation. Knowing how to pick elements well can really boost your full-stack development game.
Using Selectors in jQuery
jQuery selectors are a magnet that lets you grab and tweak HTML elements easily. Here are some common jQuery selectors:
Selector | What It Does | Example |
---|---|---|
$("p") | Grabs all <p> elements | $("p").css("color", "blue"); |
$(".className") | Grabs all elements with the class className | $(".className").hide(); |
$("#idName") | Grabs the element with the id idName | $("#idName").show(); |
$("[attribute=value]") | Grabs all elements with the specified attribute and value | $("[type='text']").val(""); |
With these selectors, you can easily target specific elements and apply various jQuery methods to them.
Zeroing In on Specific Elements with jQuery
Zeroing in on specific elements in jQuery lets you make precise changes. Here are some tricks to target specific elements:
- Child Selectors: Grabs all direct children of a specified element.
$("ul > li").css("background-color", "yellow");
- Descendant Selectors: Grabs all descendants (kids, grandkids, etc.) of a specified element.
$("div p").css("font-size", "18px");
- Attribute Selectors: Grabs elements based on the presence or value of an attribute.
$("input[name='firstName']").val("John");
- Pseudo-classes: Grabs elements based on their state or position within the DOM.
$("li:first").css("font-weight", "bold");
$("li:last").css("font-weight", "bold");
These tricks help you accurately find and tweak elements in the DOM. By mastering these selection tricks, you can get really good at manipulating the DOM.
Learn More: 10 Best Node.js Libraries and Packages
Tweaking Elements with jQuery
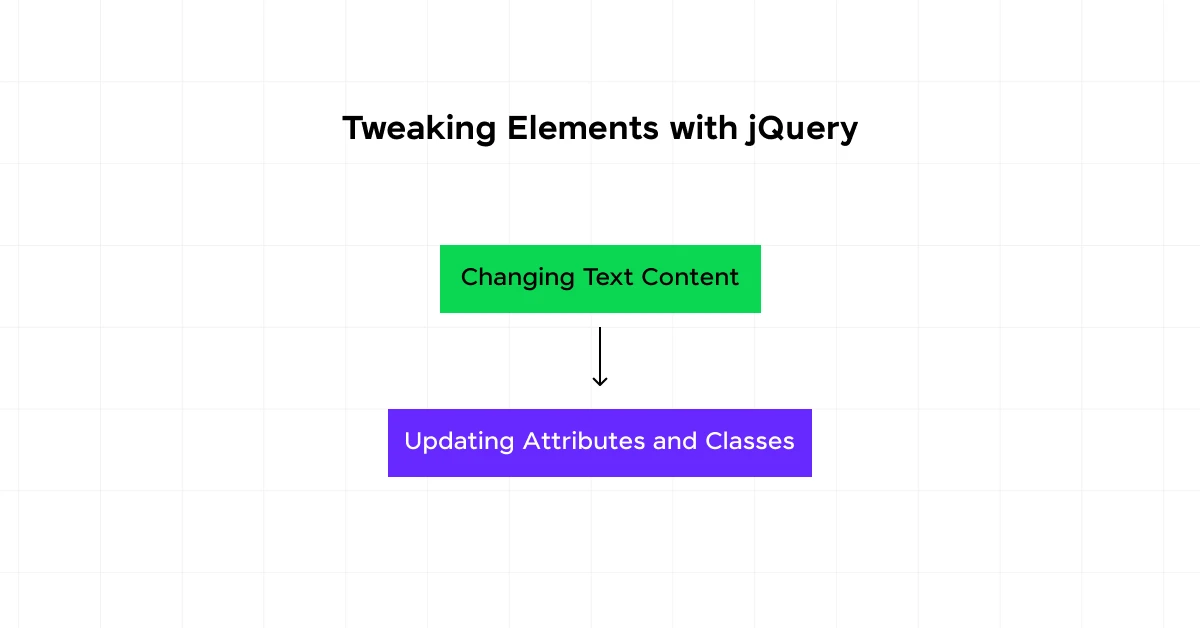
Messing around with jQuery to tweak elements? You’re in the right place. Let’s break down how you can change text, update attributes, and manage classes without breaking a sweat.
Changing Text Content
Need to change what an element says? jQuery makes it easy for you. The .text()
method is your go-to for updating text inside an HTML element. You can grab the current text or set new text with ease.
Check this out:
// Grab the current text
var currentText = $('#myElement').text();
// Set new text
$('#myElement').text('New Text Content');
If you need to include some HTML tags, switch to .html()
:
// Grab the current HTML
var currentHTML = $('#myElement').html();
// Set new HTML
$('#myElement').html('<strong>New HTML Content</strong>');
Also Read: AJAX with jQuery: A Comprehensive Guide for Dynamic Web Development
Updating Attributes and Classes
Changing attributes and classes is another jQuery superpower. Use .attr()
to get or set attribute values, and .addClass()
, .removeClass()
, or .toggleClass()
to manage CSS classes.
Here’s how you do it:
// Get an attribute value
var srcValue = $('#myImage').attr('src');
// Set a new attribute value
$('#myImage').attr('src', 'newImage.jpg');
And for classes:
// Add a class
$('#myElement').addClass('newClass');
// Remove a class
$('#myElement').removeClass('oldClass');
// Toggle a class
$('#myElement').toggleClass('activeClass');
Here’s a quick table to sum it up:
Method | What It Does |
---|---|
.attr(attributeName) | Gets the value of the specified attribute |
.attr(attributeName, value) | Sets the value of the specified attribute |
.addClass(className) | Adds the specified class to the selected elements |
.removeClass(className) | Removes the specified class from the selected elements |
.toggleClass(className) | Toggles the specified class on the selected elements |
Explore More: Best Javascript Course Online with Certification
Jazzing Up Your Web Page with jQuery DOM Manipulation
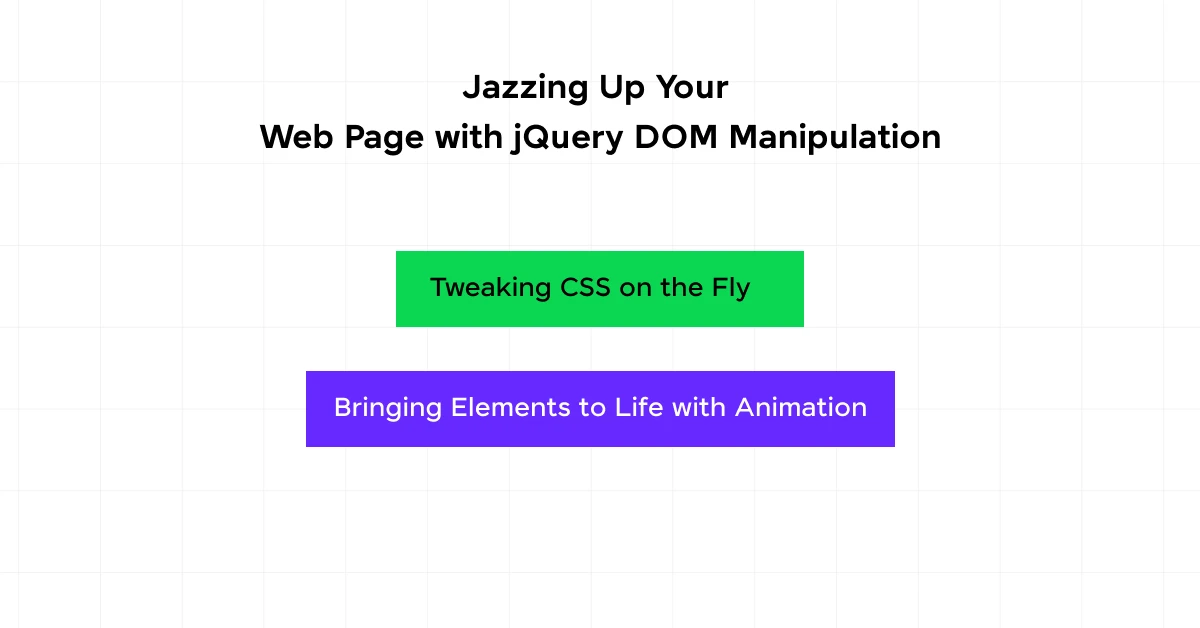
Want to make your website pop? jQuery’s got your back. Let’s dive into how you can tweak styles and animate elements to create a more lively user experience.
Tweaking CSS on the Fly
With jQuery DOM Manipulation, changing the look of your webpage is a breeze. Whether it’s a quick color change or a full-on style overhaul, jQuery’s .css()
method has you covered.
Here’s how you can change a single CSS property:
// Change the background color of a div with the id 'example'
$('#example').css('background-color', 'blue');
Need to change more than one thing? No problem. Just pass an object with your desired properties:
// Change multiple CSS properties
$('#example').css({
'background-color': 'blue',
'font-size': '20px',
'margin-top': '10px'
});
Know More: A Complete Guide to HTML and CSS for Beginners
Bringing Elements to Life with Animation
Static pages are so last decade. jQuery DOM Manipulation makes it easy to add animations that can make your site feel more interactive. The .animate()
method lets you change CSS properties over time, creating smooth transitions.
Check out this example:
// Animate the width and height of a div with the id 'example'
$('#example').animate({
width: '200px',
height: '200px'
}, 1000); // The animation will take 1000 milliseconds (1 second)
jQuery also offers shorthand methods for common animations, making it even easier to add some flair:
.slideUp()
.slideDown()
.fadeIn()
.fadeOut()
Here’s how you can use .fadeIn()
and .fadeOut()
:
// Fade in a div with the id 'example'
$('#example').fadeIn(1000); // 1000 milliseconds (1 second) to fade in
// Fade out a div with the id 'example'
$('#example').fadeOut(1000); // 1000 milliseconds (1 second) to fade out
Combine these techniques with event handlers to make your site more responsive to user actions. Imagine a button click that changes the background color or a hover effect that animates an element’s size. The possibilities are endless.
Handling Events in jQuery DOM Manipulation
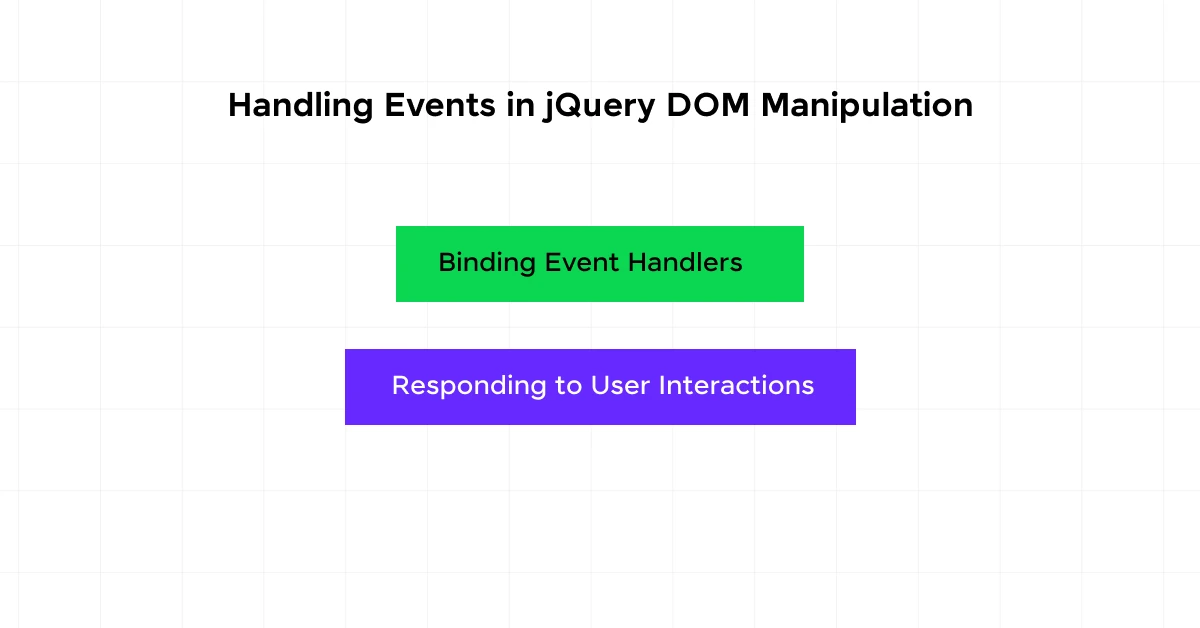
Handling events is a big deal in full-stack development. It lets you make your web pages come alive and react to what users do. With jQuery DOM Manipulation, you can easily set up event handlers and make your site interactive.
Binding Event Handlers
Binding event handlers in jQuery DOM Manipulation means you tell your page what to do when something happens. You use the .on()
method to link actions to events. Here are some events you might deal with:
click
mouseover
keydown
submit
For example, if you want to show an alert when a button with the ID #myButton
is clicked, here’s how you do it:
$('#myButton').on('click', function() {
alert('Button clicked!');
});
This code pops up an alert whenever the button gets clicked. You can use the same idea for other events.
Also Read: Event Binding On Dynamically Created Elements
Responding to User Interactions
Making your site respond to users can really boost their experience. jQuery DOM Manipulation gives you lots of ways to change the DOM based on what users do.
For example, you can change the text of an element when someone clicks a button:
$('#myButton').on('click', function() {
$('#myText').text('You clicked the button!');
});
Or you can change the color of some text:
$('#myButton').on('click', function() {
$('#myText').css('color', 'red');
});
You can do more than just simple changes. You can show or hide elements, animate stuff, and more.
Here’s a quick table of some common jQuery event methods:
jQuery Method | What It Does |
---|---|
.on() | Binds an event handler to elements |
.off() | Removes an event handler |
.click() | Binds a click event handler |
.hover() | Binds handlers for mouseenter and mouseleave events |
Getting good at handling events with jQuery will help you make responsive and fun web apps.
Also Explore: Mastering jQuery Events: A Comprehensive Guide
Advanced jQuery DOM Manipulation Tricks
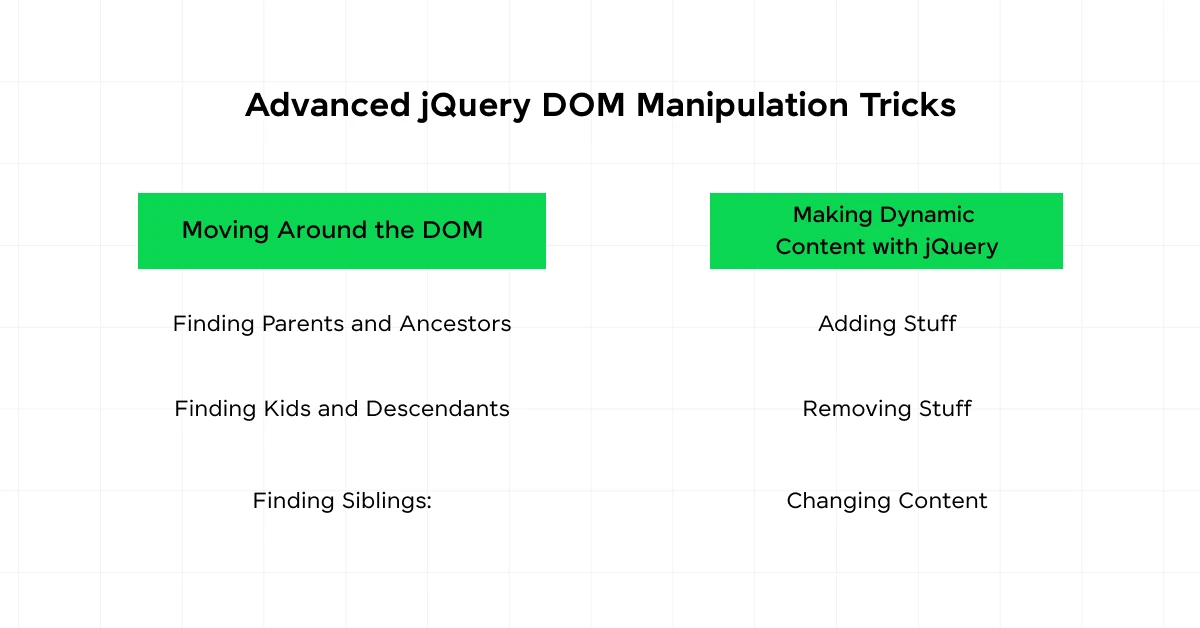
The basics are done now, it is time to move a step further and learn the advanced jQuery DOM Manipulation tricks.
Moving Around the DOM
Getting around the DOM tree is a key part of jQuery. It lets you pick and play with elements based on where they sit in the hierarchy. With jQuery DOM manipulation, you can hop up, down, and sideways in the DOM tree to grab parent, child, and sibling elements.
Finding Parents and Ancestors:
parent()
: Grabs the direct parent.parents()
: Grabs all ancestors.closest()
: Grabs the nearest matching ancestor.
// Grab the parent of an element with the class 'child'
$('.child').parent();
// Grab all ancestors of an element with the class 'child'
$('.child').parents();
// Grab the nearest ancestor with the class 'container'
$('.child').closest('.container');
Finding Kids and Descendants:
children()
: Grabs direct children.find()
: Grabs all descendants.
// Grab all direct children of an element with the class 'parent'
$('.parent').children();
// Grab all descendants with the class 'descendant'
$('.parent').find('.descendant');
Finding Siblings:
siblings()
: Grabs all siblings.next()
: Grabs the next sibling.prev()
: Grabs the previous sibling.
// Grab all siblings of an element with the class 'current'
$('.current').siblings();
// Grab the next sibling of an element with the class 'current'
$('.current').next();
// Grab the previous sibling of an element with the class 'current'
$('.current').prev();
Also Explore: How to Master DOM in Selenium for Beginners
Making Dynamic Content with jQuery
jQuery DOM Manipulation makes it easy to add, remove, or tweak elements and content on the fly.
Adding Stuff:
append()
: Adds content at the end.prepend()
: Adds content at the beginning.after()
: Adds content after.before()
: Adds content before.
// Add a new paragraph to a div with the id 'content'
$('#content').append('<p>New paragraph</p>');
// Add a new paragraph at the start of a div with the id 'content'
$('#content').prepend('<p>New paragraph</p>');
// Add a paragraph after a div with the id 'content'
$('#content').after('<p>New paragraph</p>');
// Add a paragraph before a div with the id 'content'
$('#content').before('<p>New paragraph</p>');
Removing Stuff:
remove()
: Deletes the selected element(s).empty()
: Deletes all child elements from the selected element.
// Delete an element with the class 'remove-me'
$('.remove-me').remove();
// Delete all child elements from a div with the id 'content'
$('#content').empty();
Changing Content:
html()
: Sets or gets the HTML content.text()
: Sets or gets the text content.
// Set the HTML content of a div with the id 'content'
$('#content').html('<p>Updated HTML content</p>');
// Set the text content of a div with the id 'content'
$('#content').text('Updated text content');
With these methods, you can tweak the DOM to make dynamic and interactive web pages.
If you want to learn more about jQuery DOM Manipulation in full-stack development, then consider enrolling in GUVI’s Certified Full Stack Development Course which not only gives you theoretical knowledge but also practical knowledge with the help of real-world projects.
Also Read: Full Stack Developer: Discover the Fastest Route to Becoming One
Conclusion
In conclusion, mastering jQuery DOM manipulation can significantly enhance your full-stack development skills. With jQuery, you can easily navigate and manipulate the structure of your web pages, making them more dynamic and interactive.
As you continue to explore and implement these techniques, you’ll find that jQuery is an invaluable tool in your full-stack development toolkit.
Know About The Future & Scope of Full-Stack Developers in India
FAQs
1. Why is jQuery popular for DOM manipulation?
jQuery is popular for DOM manipulation because it simplifies complex JavaScript operations, reduces the amount of code needed, and provides cross-browser compatibility.
2. What is the difference between $(this) and this in jQuery?
In jQuery, $(this) is a jQuery object that can call jQuery methods, while this is a plain JavaScript object. $(this) can be used to take advantage of jQuery’s extensive methods.
3. What is the difference between .html() and .text() in jQuery?
.html() sets or gets the HTML content of an element, while .text() sets or gets the text content without HTML tags.
4. Is it possible to extend jQuery with plugins?
Yes, jQuery can be extended with plugins to add new methods and functionality, making it a highly versatile tool for web development.
Did you enjoy this article?