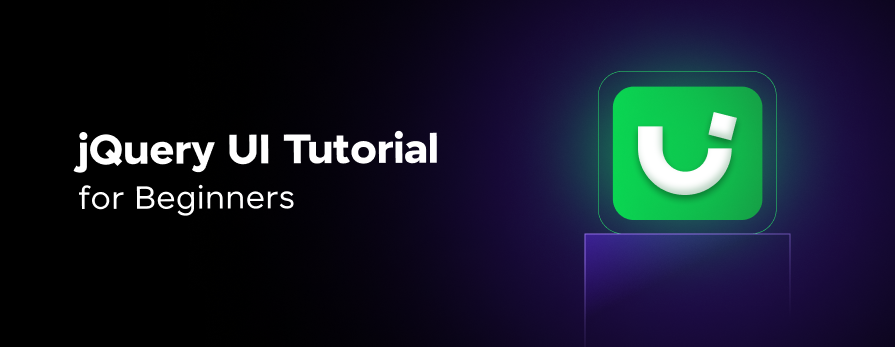
In the ever-evolving world of web development, creating engaging and user-friendly interfaces is crucial. Among the various tools and libraries available, jQuery has stood the test of time, providing developers with a powerful, yet easy-to-use, toolkit for enhancing web interfaces.
Despite the emergence of modern JavaScript frameworks like React and Vue.js, jQuery remains relevant, especially for quick enhancements and legacy projects. This blog on jQuery UI Tutorial for Beginners explores the benefits of using jQuery in UI development and provides practical examples to help you get started with it.
So, let’s get started.
Table of contents
- What is jQuery?
- Why Use jQuery for UI Development?
- Features of jQuery UI
- Getting Started with jQuery
- Practical Examples of jQuery in UI Development
- Conclusion
- FAQs
- What is jQuery UI used for?
- What is jQuery and its syntax?
- What are the advantages of jQuery?
- What are the two ways to use jQuery?
What is jQuery?
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies things like HTML document traversal and manipulation, event handling, and animation, making it much easier to use JavaScript on your website. Released in 2006 by John Resig, jQuery’s slogan is “Write less, do more,” reflecting its goal of simplifying the complex syntax of JavaScript.
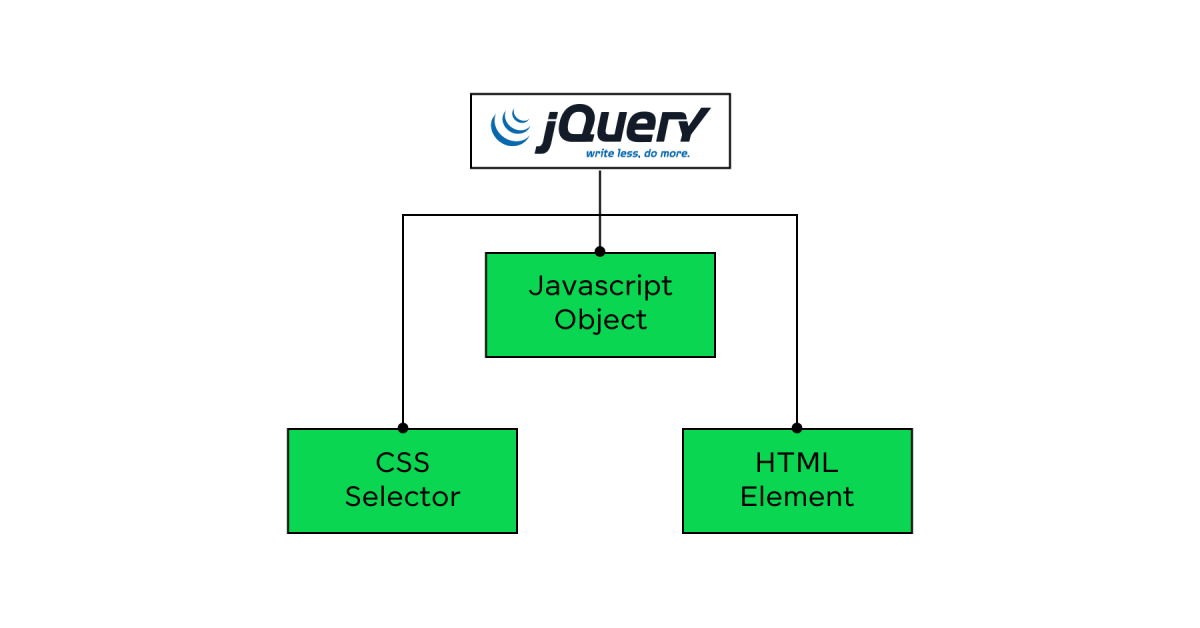
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Career Program with Placement Assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects. Additionally, if you want to explore jQuery through a self-paced course, try GUVI’s jQuery course.
Also Read: jQuery DOM Manipulation
Why Use jQuery for UI Development?
Below are the reasons why you should use jQuery for UI development:
- Ease of Use: jQuery’s syntax is concise and easy to learn, making it accessible for developers of all skill levels. Its chainable methods allow for clean, readable code.
- Cross-Browser Compatibility: jQuery handles many of the cross-browser issues that developers face, providing a unified interface for different browsers.
- Rich Set of Features: From simple animations to complex effects, jQuery offers a vast array of features that can enhance the user experience.
- Extensive Plugin Library: jQuery has a rich ecosystem of plugins that extend its functionality, allowing developers to easily add features like sliders, carousels, and lightboxes.
- Active Community: With a large and active community, finding help and resources for jQuery is easy. The community constantly updates and improves the library.
Learn jQuery Plugins with the concepts, and common uses, and also learn how to create jQuery plugins.
Features of jQuery UI
jQuery UI is a curated set of user interface interactions, effects, widgets, and themes built on top of the jQuery JavaScript Library. Here are some of its key features:
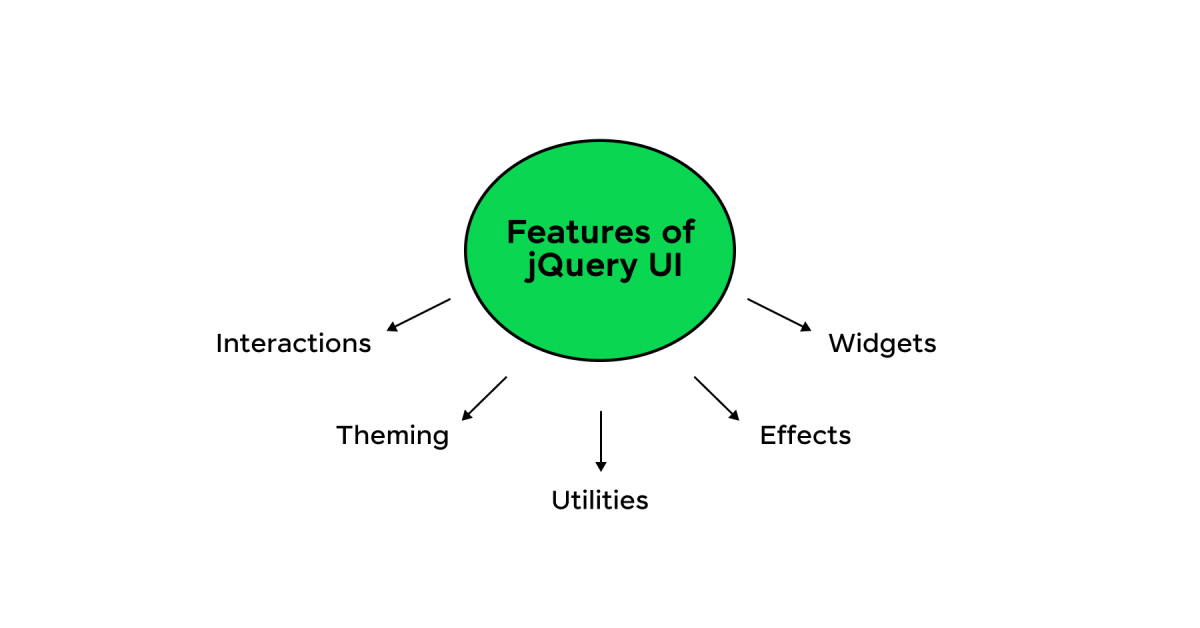
- Interactions: jQuery UI includes a variety of interaction plugins that help you build interactive web applications, including:
- Draggable: Makes any element draggable.
- Droppable: Allows an element to be a drop target.
- Resizable: Makes elements resizable by dragging.
- Selectable: Enables selection of multiple elements by dragging a box.
- Sortable: Enables sorting of elements through drag-and-drop.
- Widgets: jQuery UI provides a number of ready-to-use widgets that you can easily incorporate into your web applications, such as:
- Accordion: Creates collapsible content panels.
- Autocomplete: Adds a dropdown list of suggestions to a text input field.
- Button: Enhances HTML buttons with advanced features.
- Datepicker: Adds a datepicker to input fields.
- Dialog: Creates modal or non-modal dialog boxes.
- Menu: Creates a hierarchical menu.
- Progressbar: Displays a graphical progress bar.
- Slider: Adds a slider control for selecting values.
- Spinner: Adds a spinner to allow number input.
- Tabs: Creates a tabbed interface.
- Tooltip: Enhances the default browser tooltip.
- Effects: jQuery UI provides a set of effects for adding visual polish to your applications, such as:
- Color Animation: Animate changes in colors.
- Class Transitions: Animate changes in CSS class.
- Easing: Control the rate of animations.
- Show/Hide: Effects for showing and hiding elements.
- Toggle: Effects for toggling element visibility.
- Utilities: jQuery UI includes various utilities to simplify common tasks, including:
- Position: Align elements relative to the window, document, another element, or the cursor/mouse.
- Widget Factory: A base for creating stateful jQuery plugins by providing a consistent API and built-in functionality for managing options, state, and events.
- Theming: jQuery UI has a robust theming framework that allows developers to apply a consistent look and feel across all widgets. This includes:
- ThemeRoller: A web-based application to create custom themes by adjusting various parameters and downloading the resulting CSS.
- Predefined Themes: A set of predefined themes that can be used out-of-the-box or customized via ThemeRoller.
You can also optimize Web Development projects using jQuery for Responsive Design.
Getting Started with jQuery
To start using jQuery, you can either download the library and host it locally or include it via a CDN (Content Delivery Network). The latter is often preferred for its simplicity and faster loading times.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
There are two main ways to include jQuery in your web projects:
1. Using a CDN (Content Delivery Network).
2. Downloading and hosting jQuery locally.
Also, read about AJAX with jQuery for Dynamic Web Development
Basic jQuery Syntax: $(selector).action();
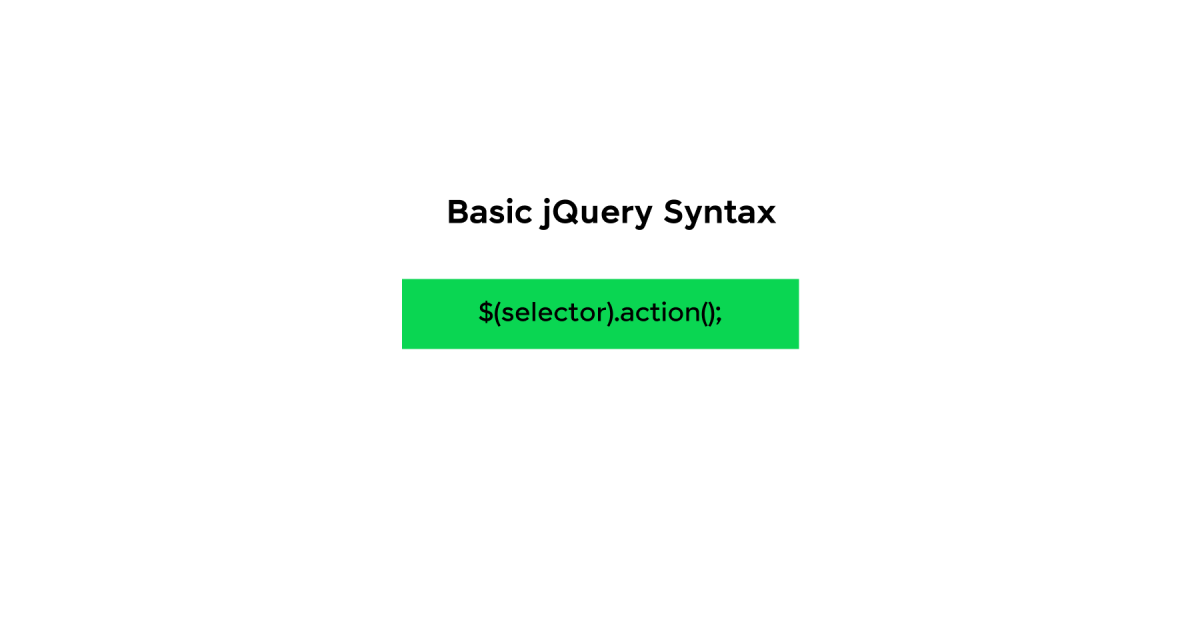
where,
$: This is the jQuery function, also known as the jQuery object.
selector: This is used to query or find HTML elements.
action(): This is the jQuery action that is to be performed on the elements.
Must Read:
Practical Examples of jQuery in UI Development
1. Creating a Simple Slider:
<div id="slider">
<div class="slide">Slide 1</div>
<div class="slide">Slide 2</div>
<div class="slide">Slide 3</div>
</div>
<button id="next">Next</button>
<script>
$(document).ready(function(){
let currentSlide = 0;
$("#next").click(function(){
currentSlide = (currentSlide + 1) % 3;
$("#slider .slide").hide();
$("#slider .slide").eq(currentSlide).show();
});
});
</script>
2. Form Validation:
<form id="myForm">
<input type="text" id="name" placeholder="Enter your name">
<input type="submit" value="Submit">
</form>
<div id="error"></div>
<script>
$(document).ready(function(){
$("#myForm").submit(function(event){
if($("#name").val() === ""){
$("#error").text("Name is required!");
event.preventDefault();
}
});
});
</script>
3. Interactive Content Filtering:
<input type="text" id="search" placeholder="Search items...">
<ul id="items">
<li>Apple</li>
<li>Banana</li>
<li>Cherry</li>
</ul>
<script>
$(document).ready(function(){
$("#search").keyup(function(){
let filter = $(this).val().toLowerCase();
$("#items li").filter(function(){
$(this).toggle($(this).text().toLowerCase().indexOf(filter) > -1);
});
});
});
</script>
Kickstart your Full Stack Development journey by enrolling in GUVI’s Certified Full Stack Development Career Program with Placement Assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements. Alternatively, if you want to explore jQuery through a self-paced course, try GUVI’s jQuery course.
Conclusion
jQuery remains a valuable tool in a web developer’s arsenal, particularly for quick, simple tasks and maintaining legacy projects. Its ease of use, extensive feature set, and active community make it a go-to choice for enhancing user interfaces. Whether you are building a new project or maintaining an old one, jQuery can help you create interactive and user-friendly web applications with minimal effort.
FAQs
What is jQuery UI used for?
jQuery UI is a collection of user interface interactions, effects, widgets, and themes built on top of the jQuery JavaScript Library. jQuery UI simplifies the process of adding complex, interactive features to web applications, making it easier to create a more polished and user-friendly interface.
What is jQuery and its syntax?
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies tasks such as HTML document traversal and manipulation, event handling, animation, and Ajax, with an easy-to-use API that works across a multitude of browsers.
Syntax: $(selector).action();
What are the advantages of jQuery?
1. Enables developers/programmers to write JavaScript faster and easier.
2. Works with multiple browsers, so the code is compatible regardless of whatever features the browser contains.
3. Compresses the most common JavaScript actions into fewer lines of code.
4. Helps you avoid common browser errors.
What are the two ways to use jQuery?
There are two main ways to include jQuery in your web projects:
1. Using a CDN (Content Delivery Network).
2. Downloading and hosting jQuery locally.
Did you enjoy this article?