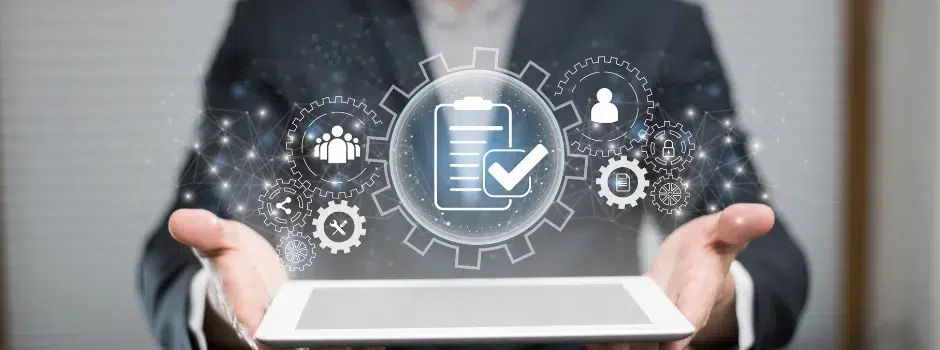
Table of contents
- Understanding the Role of iframes in Web Automation
- Identifying iframes on a Web Page
- Switching to an iframe with Selenium
- Methods to Switch to an iframe
- Interacting with Elements inside an iframe
- Switching Back to the Main Document
- Handling Nested iframes
- Best Practices for Handling iframes
- Wrapping Up: Best Practices for iframes in Selenium
Understanding the Role of iframes in Web Automation
When automating web applications, iframes often act as gatekeepers to essential content, posing unique challenges for test automation. An iframe, or inline frame, is a separate HTML document embedded within a parent HTML page, commonly used for integrating external widgets, ads, or third-party content. Since Selenium WebDriver operates on the main document by default, interacting with elements inside an iframe requires a deliberate switch of focus to the iframe. This guide delves into effective strategies for identifying, navigating, and managing iframes using Selenium WebDriver in Python. By understanding these techniques, you’ll gain the skills to handle even the most complex iframe scenarios in your automation projects seamlessly.
1. Identifying iframes on a Web Page
Before interacting with an iframe, you need to identify it on the page. You can usually find iframes by inspecting the HTML structure. Iframes are defined by the <iframe> tag and often have attributes like id, name, or src, which can help in locating them.
Example:
<iframe id=”iframe_id” src=”https://example.com/embedded_content”></iframe>
2. Switching to an iframe with Selenium
In Selenium, you can switch to an iframe using driver.switch_to.frame(). Selenium provides three primary ways to identify the iframe:
- By Index
- By Name or ID
- By WebElement
3. Methods to Switch to an iframe
a) Switching by Index
If you have multiple iframes on a page, you can switch by index. Note that index starts from 0.
from selenium import webdriver
driver = webdriver.Chrome()
driver.get(“https://example.com”)
# Switch to the first iframe on the page
driver.switch_to.frame(0)
b) Switching by Name or ID
If the iframe has a name or id attribute, you can switch to it directly by passing the name or id as a string.
# Switch to iframe using name or id
driver.switch_to.frame(“iframe_id”)
c) Switching by WebElement
If the iframe doesn’t have a reliable name or id, you can locate it as a WebElement and then switch.
# Locate the iframe element
iframe_element = driver.find_element(By.XPATH, “//iframe[@src=’https://example.com/embedded_content’]”)
# Switch to the iframe using WebElement
driver.switch_to.frame(iframe_element)
4. Interacting with Elements inside an iframe
Once inside the iframe, you can interact with its elements just like any other element. For example, let’s say you want to fill in a text field within the iframe.
# Locate the input element within the iframe and interact with it
driver.find_element(By.ID, “input_field”).send_keys(“Hello inside iframe!”)
5. Switching Back to the Main Document
After performing actions inside the iframe, it’s essential to switch back to the main document. You can do this using driver.switch_to.default_content().
# Switch back to the main content
driver.switch_to.default_content()
6. Handling Nested iframes
In cases where you have an iframe within another iframe (nested iframes), you need to switch to each iframe layer by layer.
# Switch to the outer iframe
driver.switch_to.frame(“outer_iframe”)
# Then switch to the inner iframe
driver.switch_to.frame(“inner_iframe”)
# Interact with elements inside the inner iframe
driver.find_element(By.ID, “inner_element”).click()
# Switch back to the outer iframe
driver.switch_to.parent_frame()
# Finally, switch back to the main document
driver.switch_to.default_content()
7. Best Practices for Handling iframes
- Use Explicit Waits: iframes may load slower than the main page. Use explicit waits to ensure the iframe and its elements are ready before interacting.
- Avoid Hardcoded Indexes: Indexes can be unreliable if the page structure changes. Using name, id, or WebElement is often more stable.
- Always Switch Back: Don’t forget to switch back to the main document when done. It’s easy to get stuck in an iframe, leading to errors when trying to locate elements outside of it.
Example: Full Workflow with iframes
Here’s a full example where we load a page, switch to an iframe, perform an action, and then switch back:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Initialize WebDriver
driver = webdriver.Chrome()
driver.get(“https://example.com”)
# Wait until iframe is available and switch to it
WebDriverWait(driver, 10).until(EC.frame_to_be_available_and_switch_to_it((By.ID, “iframe_id”)))
# Perform actions within the iframe
driver.find_element(By.ID, “button_inside_iframe”).click()
# Switch back to the main document
driver.switch_to.default_content()
# Continue interacting with main document elements
driver.find_element(By.ID, “main_page_element”).click()
Wrapping Up: Best Practices for iframes in Selenium
Handling iframes in Selenium is a critical skill for any automation engineer, especially when dealing with modern, dynamic web applications. From identifying and switching to iframes to interacting with nested frames and returning to the main document, mastering these processes ensures your scripts remain robust and versatile. By adopting explicit waits and avoiding hardcoded indexes, you can mitigate common pitfalls and build reliable automation workflows. With consistent practice and adherence to best practices, iframe management will become an integral part of your Selenium expertise, enabling you to confidently tackle even the most intricate test scenarios.
Mastering iframes is just one piece of the puzzle in Selenium automation. If you’re eager to sharpen your test automation expertise and gain real-world exposure, consider the industry-recognized Selenium Automation Testing Course. With hands-on training, expert mentorship, and industry-relevant projects, this course equips you with the skills to tackle complex automation challenges effectively.
Did you enjoy this article?