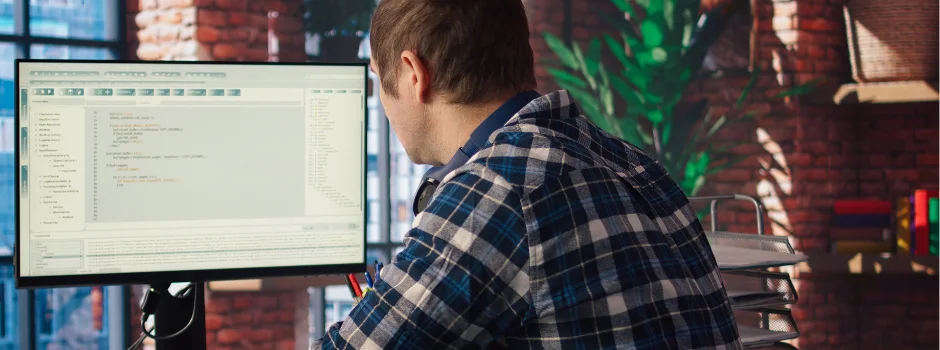
Mastering Selenium Web Automation with Python: Key Components and Best Practices
Jan 31, 2025 3 Min Read 804 Views
(Last Updated)
Web automation is crucial for modern software development, enabling faster, more reliable testing. If you’re looking to make your web testing efforts smarter and more efficient, Selenium web automation with Python is a powerful solution. Selenium, with its key components like WebDriver, Grid, IDE, and RC, provides a versatile framework for automating repetitive browser tasks and comprehensive web application tests. By pairing Selenium with Python, you can streamline your testing processes with the language’s simplicity and power. In this blog, we’ll explore the core components of Selenium and how Python enhances each of them, from the intuitive Selenium IDE for beginners to the robust WebDriver for complex tasks. We’ll also dive into Selenium Grid, a tool for parallel testing across multiple machines and browsers. Mastering these components will make your web automation more effective and efficient.
Table of contents
- Selenium WebDriver
- Overview
- Key Features
- Usage with Python
- Selenium Grid
- Overview
- Key Features
- Usage with Python
- Selenium IDE
- Overview
- Key Features
- Usage with Python
- Selenium RC (Remote Control)
- Overview
- Key Features
- Usage with Python
- Final Thoughts
1. Selenium WebDriver
Overview
Selenium WebDriver is the core component of the Selenium framework. It provides a programming interface to create and execute test scripts that interact directly with web browsers.
Key Features
- Browser Control: WebDriver allows you to launch and control different web browsers like Chrome, Firefox, Safari, and Edge.
- Element Interaction: It supports a wide range of interactions with web elements, such as clicking, typing, selecting, and more.
- Navigation: WebDriver can navigate between pages, refresh pages, and move forward or backward in the browser history.
- Wait Mechanisms: It includes explicit and implicit wait mechanisms to handle dynamic web content.
Usage with Python
To use Selenium WebDriver with Python, you need to install the selenium package via pip and set up the appropriate web driver for your browser.
from selenium import webdriver
from selenium.webdriver.common.by import By
# Set up the driver
driver = webdriver.Chrome(executable_path=’/path/to/chromedriver’)
# Open a website
driver.get(‘https://www.example.com’)
# Interact with elements
element = driver.find_element(By.ID, ‘element_id’)
element.click()
# Close the browser
driver.quit()
2. Selenium Grid
Overview
Selenium Grid allows you to distribute your test execution across multiple machines and browsers, facilitating parallel testing. This can significantly reduce the time required to run a large suite of tests.
Key Features
- Distributed Testing: Run tests on different machines with different browsers and operating systems.
- Parallel Execution: Execute multiple tests simultaneously, improving efficiency.
- Centralized Control: Manage multiple environments from a single hub.
Usage with Python
To use Selenium Grid, you need to set up a hub and nodes, then configure your WebDriver to interact with the grid.
from selenium import webdriver
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
# Set up remote WebDriver to connect to Selenium Grid
driver = webdriver.Remote(
command_executor=’http://localhost:4444/wd/hub’,
desired_capabilities=DesiredCapabilities.CHROME
)
# Open a website
driver.get(‘https://www.example.com’)
# Interact with elements
element = driver.find_element(By.ID,’element_id’)
element.click()
# Close the browser
driver.quit()
3. Selenium IDE
Overview
Selenium IDE (Integrated Development Environment) is a browser plugin that allows you to record and playback interactions with the web. It’s a great tool for beginners to get started with Selenium without writing code.
Key Features
- Recording and Playback: Record interactions with the web and play them back as automated tests.
- Exporting Scripts: Export recorded tests to different programming languages, including Python, to integrate with Selenium WebDriver.
- Easy Debugging: Built-in debugging tools to help troubleshoot test scripts.
Usage with Python
While Selenium IDE itself does not use Python, you can export the recorded tests as Python scripts and then run them using Selenium WebDriver.
But let’s be real – if you’re serious about mastering Selenium beyond just recording scripts, you need hands-on experience writing automation code, working with WebDriver, and understanding real-world test scenarios. That’s where structured learning can accelerate your progress.
If you’re looking for a practical, guided approach to Selenium automation with expert mentorship, check out GUVI’s Selenium Automation Testing Course. Designed for beginners and professionals alike, this program helps you build industry-ready automation skills with real-world projects and placement support.
4. Selenium RC (Remote Control)
Overview
Selenium Remote Control (RC) is the predecessor of Selenium WebDriver. It allows you to write automated web application UI tests in any programming language against any HTTP website using any mainstream JavaScript-enabled browser.
Key Features
- Language Support: Supports multiple programming languages, including Python.
- Cross-browser Testing: Allows testing across different browsers and platforms.
Usage with Python
Selenium RC is largely deprecated in favor of Selenium WebDriver, which offers a more modern and efficient approach to browser automation. However, for legacy systems, you might encounter Selenium RC scripts.
# Example (not recommended for new projects)
from selenium import selenium
sel = selenium(“localhost”, 4444, “*chrome”, “http://www.example.com”)
sel.start()
sel.open(“/”)
sel.click(“link=Login”)
sel.type(“username”, “test_user”)
sel.type(“password”, “password”)
sel.click(“submit”)
sel.stop()
Final Thoughts
Selenium’s versatility lies in its various components, each tailored to different automation scenarios—from the intuitive recording features of Selenium IDE to the robust scripting capabilities of Selenium WebDriver and the parallel testing power of Selenium Grid. Python brings an added layer of simplicity and efficiency, making it an excellent partner for Selenium. By understanding how these components work together, you’ll be better equipped to build scalable and effective automation solutions. With consistent practice and exploration, you can turn even the most complex testing challenges into manageable, streamlined processes.
Did you enjoy this article?