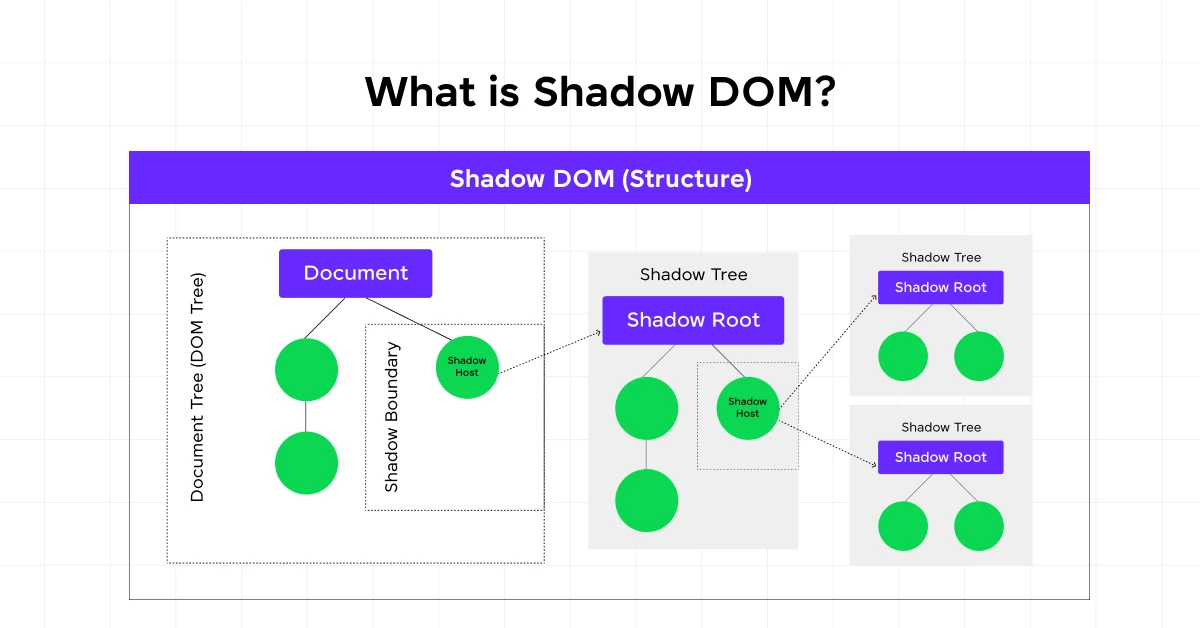
Mastering Shadow DOM Events: A Comprehensive Guide
Dec 31, 2024 5 Min Read 2972 Views
(Last Updated)
In the ever-evolving landscape of web development, the shadow DOM plays a pivotal role in encapsulating and structuring web components, offering a more modular and scalable approach to designing web applications.
By understanding and mastering shadow DOM events, web developers can significantly elevate the interactivity and user experience of web components while maintaining code separation and style encapsulation.
This depth of encapsulation not only streamlines the development process but also fortifies the application against common web vulnerabilities, making your knowledge of shadow DOM events an indispensable asset in the modern web development toolkit.
This article aims to guide you through the intricacies of shadow DOM events, starting with a fundamental understanding of the shadow DOM and encapsulation.
By the conclusion of this guide, you will possess a solid foundational knowledge of shadow DOM events, enabling you to effectively implement and manipulate DOM events within web components.
Table of contents
- Understanding Shadow DOM and Encapsulation
- 1) Definition and Importance
- 2) Style Scoping Enforcement
- Event Propagation in Shadow DOM
- 1) Bubbling vs. Composed Events
- 2) ShadowRoot Constructor
- Event Retargeting and composedPath in Shadow DOM
- 1) Retargeting Concept
- 2) Practical Examples
- Special Cases and Standard Events in Shadow DOM
- 1) Non-Composed Events
- 2) Bubbling and Non-Bubbling Behaviors
- Concluding Thoughts...
- FAQs
- What does shadow DOM do?
- What does the shadow DOM contain?
- How can we fire events up through the shadow?
- Is shadow DOM faster?
Understanding Shadow DOM and Encapsulation
1.1) Definition and Importance
Shadow DOM is an essential part of the DOM that provides the capability to isolate JavaScript and CSS, similar to iframes
.
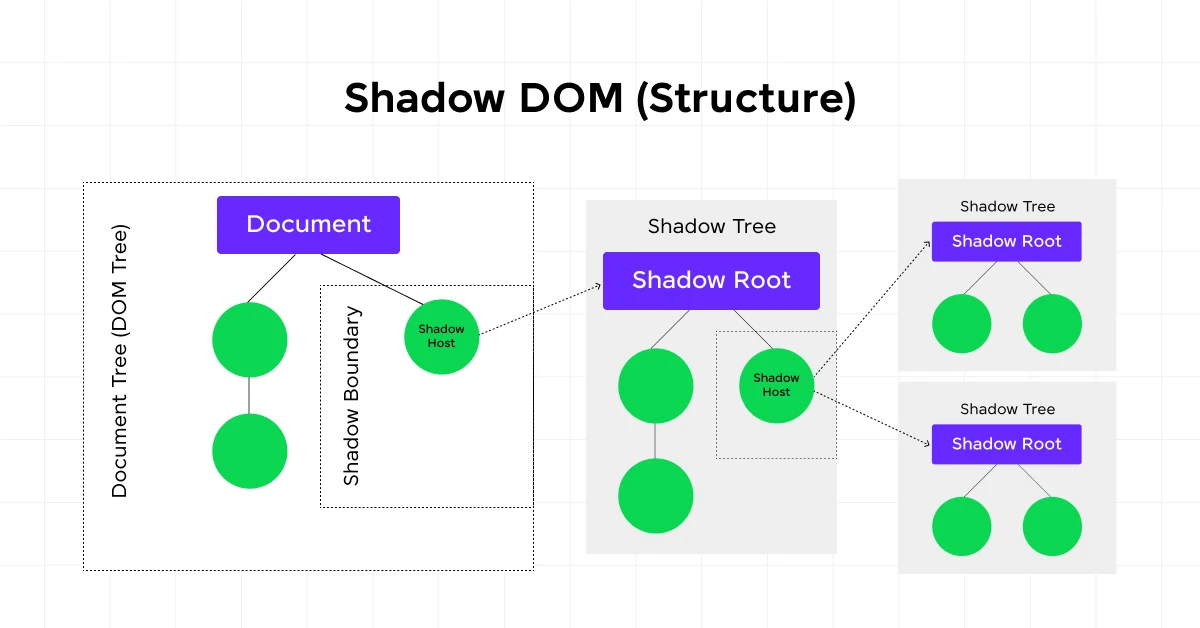
This isolation ensures that styles within a shadow DOM do not leak out, and external styles do not affect the internals of the shadow DOM.
This feature is crucial for creating self-contained, reusable components that are immune to style and naming conflicts when shared across different projects.
Also Read: What is DOM in Web Development?
1.2) Style Scoping Enforcement
- The enforcement of style scoping within the Shadow DOM is a vital feature that allows developers to maintain style encapsulation effectively.
- Globally scoped styles will apply to all matching elements in the document but will not interfere with the styles defined within the shadow trees.
- This scoping ensures that styles are confined to their respective DOMs, preventing unwanted style leakage and conflicts.
- In the context of web performance, shadow DOM’s style scoping offers significant advantages. By allowing browsers to handle smaller “sub-DOMs,” the computational overhead associated with style recalculations is reduced.
- This results in a more consistent and faster performance across different browsers and devices. For instance, benchmarks show that shadow DOM maintains steady performance irrespective of the DOM size or CSS complexity, with Chrome demonstrating particularly fast processing times compared to other browsers.
- Moreover, shadow DOM supports the use of
adoptedStyleSheets
, which lets developers define a single stylesheet that can be shared across multiple shadow DOMs.
- This approach not only optimizes style parsing and application across various components but also allows for dynamic style updates that are immediately reflected across all components using the shared stylesheet.
- By encapsulating styles within the shadow DOM, developers can ensure that their components remain stylistically independent and consistent, regardless of the external styles present on the page.
- This isolation is instrumental in building robust web applications that are both scalable and maintainable.
Also Read: DOM Scripting Techniques for Modern Web Development
Before proceeding further, make sure you have a strong grasp of essential concepts in Java Full Stack Development, including front-end frameworks, back-end technologies, and database management. If you’re looking for a professional future in Java Full Stack Development, consider joining GUVI’s Java Full Stack Development Course. With placement assistance included, you’ll master the Java stack and build real-world projects to enhance your skills.
If you would like to explore JAVA through a self-paced course, you can take up GUVI’s JAVA course with certification.
Event Propagation in Shadow DOM
Understanding how events propagate in the Shadow DOM is crucial for managing interactions within your web components effectively.
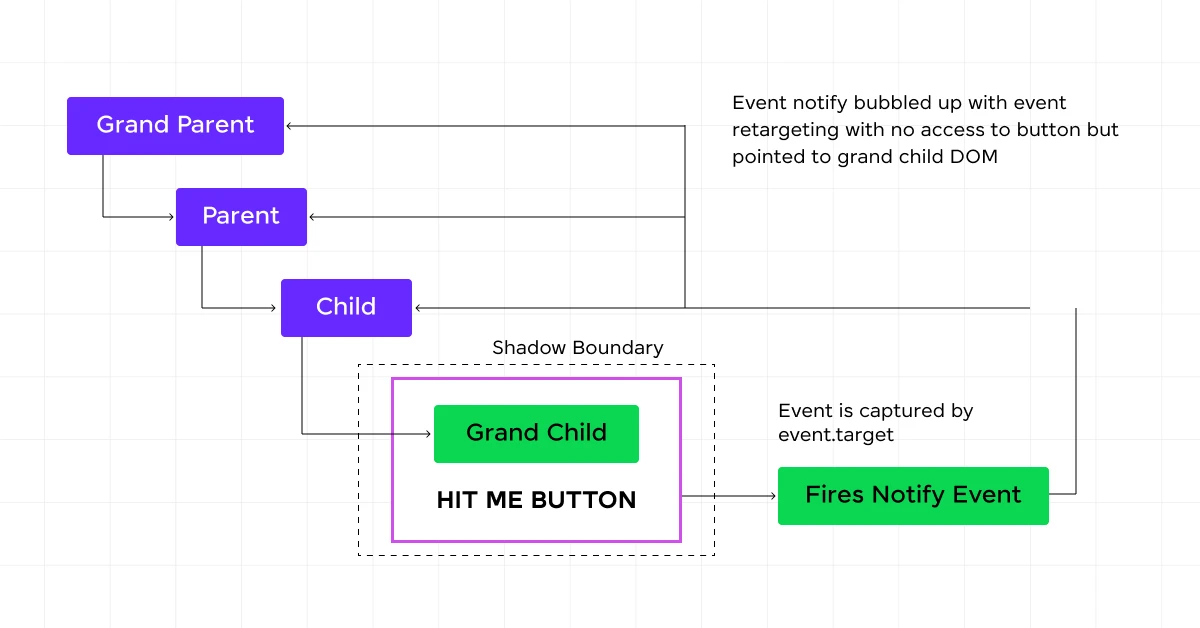
The ShadowRoot
constructor plays a fundamental role in this process as it extends EventTarget
, making it capable of dispatching and listening for events like other DOM nodes.
2.1) Bubbling vs. Composed Events
Events in the Shadow DOM behave slightly differently compared to the standard DOM. By default, events do not propagate outside the shadow trees, which prevents internal DOM events from inadvertently leaking into the document.
However, for an event to traverse the Shadow DOM boundaries, it must be configured with the composed: true
property.
Here’s a simple breakdown:
- Bubbling: Events will bubble up through the DOM hierarchy only if the
bubbles
property is set totrue
. - Composed: To allow events to cross Shadow DOM boundaries, the
composed
property must also be set totrue
.
For example, consider an event initialized with new Event('test', { bubbles: true, composed: true })
. This event will not only bubble within the shadow tree but also move beyond it, reaching potentially higher-level document nodes.
2.2) ShadowRoot Constructor
The ShadowRoot
is the root node for a shadow tree and is essential for event handling within the shadow DOM.
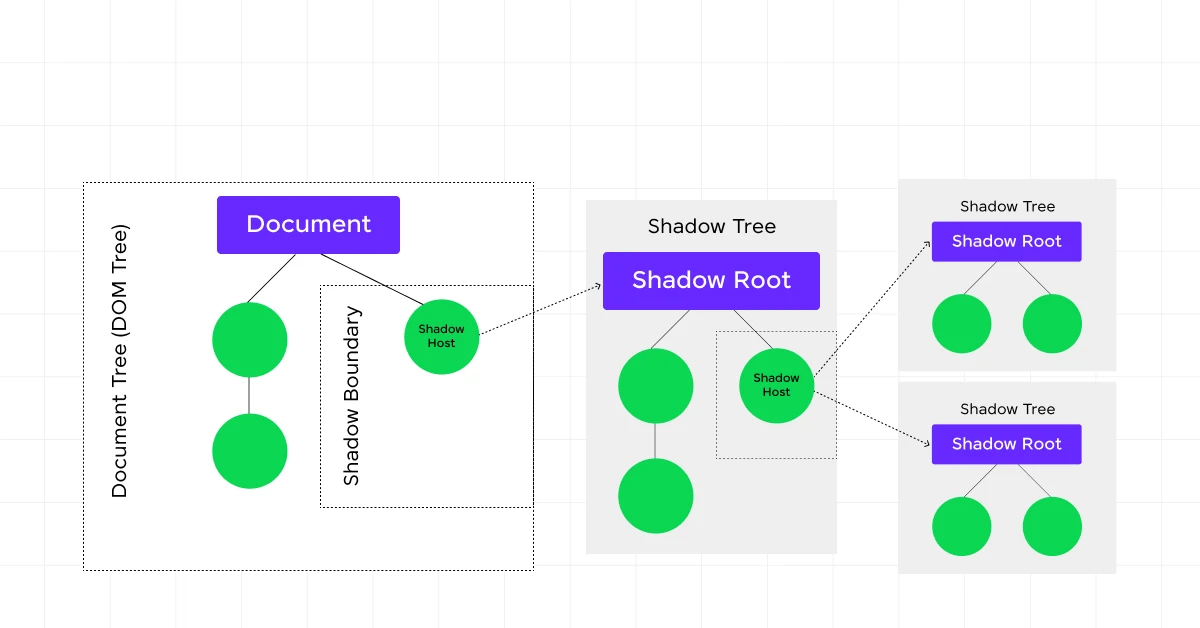
You can add event listeners directly to the shadow root, allowing you to manage events originating from any element within the shadow tree. Here’s how you might add a listener:
const shadowRoot = this.attachShadow({mode: 'open'});
shadowRoot.addEventListener('click', function(event) {
console.log('Click event triggered within shadow DOM');
});
This setup ensures that any click event within the shadow DOM triggers the specified function, maintaining encapsulation and control over the component’s internal behavior.
Event Propagation Example
Consider a scenario where an event is dispatched from a nested element within the shadow DOM:
let customEvent = new CustomEvent("myevent", { bubbles: true, composed: true });
this.shadowRoot.querySelector('span#example').dispatchEvent(customEvent);
In this case, the event will bubble up and cross the shadow boundary due to the composed: true
setting. The propagation path can be examined using the composedPath()
method, which returns the sequence of nodes the event will pass through.
Table: Event Properties and Their Effects
Property | Value | Effect on Propagation |
---|---|---|
bubbles | true | Propagates up through the DOM hierarchy |
composed | true | Crosses shadow boundaries |
bubbles | false | Contained within the initiating element |
composed | false | Contained within the shadow DOM |
Understanding these properties and how they influence event propagation is key to mastering interactions within your web components, ensuring both functionality and encapsulation are maintained.
Also Read: Mastering the DOM: A Guide to the HTML Document Object Model
Event Retargeting and composedPath in Shadow DOM
3.1) Retargeting Concept
Event retargeting is a fundamental mechanism within the Shadow DOM that ensures events appear as though they are coming from the host element, rather than from within the shadow tree.
This process is crucial for maintaining the encapsulation of the component’s internal structure. When an event is dispatched from an element inside the shadow DOM, the event’s target is adjusted as it bubbles up to the main document, making the host element appear as the event target.
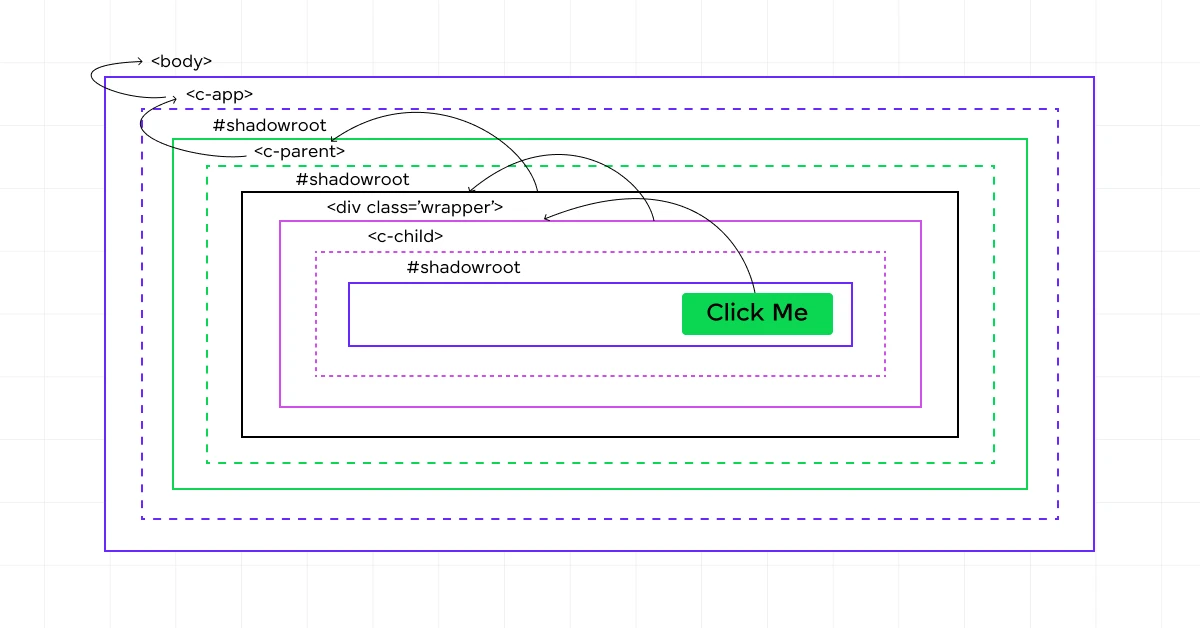
This adjustment is handled by setting the target to its host element once the event leaves the shadow tree.
This allows scripts outside the shadow DOM to interact with the shadow DOM as if it were a single element, without exposing its internal details.
3.2) Practical Examples
Consider a scenario where a click
event is triggered inside a shadow DOM:
// Adding an event listener inside the shadow DOM
const shadowRoot = this.attachShadow({mode: 'open'});
const button = document.createElement('button');
button.textContent = 'Click Me';
shadowRoot.appendChild(button);
button.addEventListener('click', function(event) {
alert('Clicked inside the shadow DOM');
});
// Adding an event listener outside the shadow DOM
document.addEventListener('click', function(event) {
console.log('Event target:', event.target.tagName);
});
In this example, even though the click occurs on the button inside the shadow DOM, the event target logged outside the shadow DOM will be the host element of the shadow DOM, not the button itself.
Table: Event Retargeting Effects
Event Origin | Original Target | Retargeted Event Target |
---|---|---|
Inside Shadow DOM | Button | Host Element |
Outside Shadow DOM | Div | Div |
The composedPath()
method provides a way to retrieve the full path of the event, showing all the nodes it passed through, including those within shadow trees:
button.addEventListener('click', function(event) {
console.log('Composed Path:', event.composedPath().map(node => node.tagName || node.nodeName));
});
This method will output an array of node names, offering insight into the actual path the event took through the DOM, which is crucial for debugging and complex event-handling scenarios in web applications involving shadow DOM.
Know About How To Boost DOM Rendering Performance Like a Pro
Special Cases and Standard Events in Shadow DOM
4.1) Non-Composed Events
Non-composed events, such as mouseenter
and mouseleave
, exhibit unique behavior within the Shadow DOM.
These events do not bubble and are not composed, meaning they do not cross the shadow boundary.
This containment within the originating shadow tree ensures that component internals remain encapsulated, preventing any leakage of implementation details.
4.2) Bubbling and Non-Bubbling Behaviors
Understanding the distinction between bubbling and non-bubbling behaviors in Shadow DOM events is crucial for effective event handling.
Most standard UI events like click
, keydown
, and touchstart
are both bubbling and composed, allowing them to propagate beyond the shadow boundary.
Table: Event Types and Their Characteristics
Event Type | Bubbles | Composed |
---|---|---|
mouseenter | No | No |
mouseleave | No | No |
click | Yes | Yes |
keydown | Yes | Yes |
touchstart | Yes | Yes |
For events that bubble and are composed, the event’s propagation path can include nodes outside the shadow tree, reaching the main document.
This is particularly important for events like click
and touchstart
, which are designed to interact seamlessly across document boundaries.
In contrast, non-bubbling, non-composed events are confined to the shadow tree where they were dispatched, as illustrated by mouseenter
and mouseleave
.
This behavior is vital for maintaining the isolation and security of web components, ensuring that interactions within a component do not inadvertently affect the surrounding document.
Also Explore More About Event Bubbling in DOM: An Interesting Technique To Know About
Code Example: Handling Bubbling and Non-Bubbling Events
// Adding event listeners within the Shadow DOM
const shadowRoot = this.attachShadow({mode: 'open'});
const innerDiv = document.createElement('div');
innerDiv.id = 'innerDiv';
shadowRoot.appendChild(innerDiv);
// Non-bubbling event
innerDiv.addEventListener('mouseenter', function(event) {
console.log('Mouse entered inner div');
});
// Bubbling and composed event
innerDiv.addEventListener('click', function(event) {
console.log('Click event within Shadow DOM');
});
// Event listener outside the Shadow DOM
document.addEventListener('click', function(event) {
console.log('Click event on document');
});
This code snippet demonstrates how different events are handled within and outside of the Shadow DOM.
The mouseenter
event, being non-bubbling and non-composed, will not trigger the document-level event listener, while the click
event will, due to its bubbling and composed nature.
Also Explore: DOM Event Flow: A Comprehensive Guide
Begin your career journey with GUVI’s Java Full Stack Development Course, providing placement assistance. Master essential technologies including Java, Maven, Eclipse, HTML, CSS, MongoDB, and more while working on practical real-world projects to enhance your expertise.
If you would like to explore JAVA through a self-paced course, you can take up GUVI’s JAVA course with certification.
Concluding Thoughts…
Throughout this article, we’ve understood the complexities of shadow DOM events, beginning with the basics of shadow DOM and its significance in web development to elucidate the nuanced mechanisms of event propagation, retargeting, and handling special cases.
The exploration of bubbling versus composed events, the functionality of the ShadowRoot
constructor and the critical concept of event retargeting has been complemented by practical code examples and explanations.
These segments have not only outlined how to effectively manage and utilize events within the shadow DOM but have also emphasized the importance of encapsulation in creating robust and maintainable web components.
Understanding these core topics equips developers with the knowledge to harness the full potential of the shadow DOM, improving the interactivity and user experience of web applications while maintaining a solid architectural foundation.
Must Read About Virtual DOM and Shadow DOM: Understanding the Key Differences and Benefits
FAQs
What does shadow DOM do?
Shadow DOM encapsulates a part of a webpage’s DOM tree to create a separate, isolated scope, improving modularity and preventing style and script conflicts.
What does the shadow DOM contain?
The shadow DOM contains its own HTML elements, styles, and scripts, which are isolated from the main document’s DOM.
How can we fire events up through the shadow?
To fire events up through the shadow DOM, use the composed: true
property in the event configuration. This allows the event to propagate across shadow DOM boundaries.
Is shadow DOM faster?
Yes, shadow DOM can improve performance by reducing style recalculations and enabling better component-based development.
Did you enjoy this article?