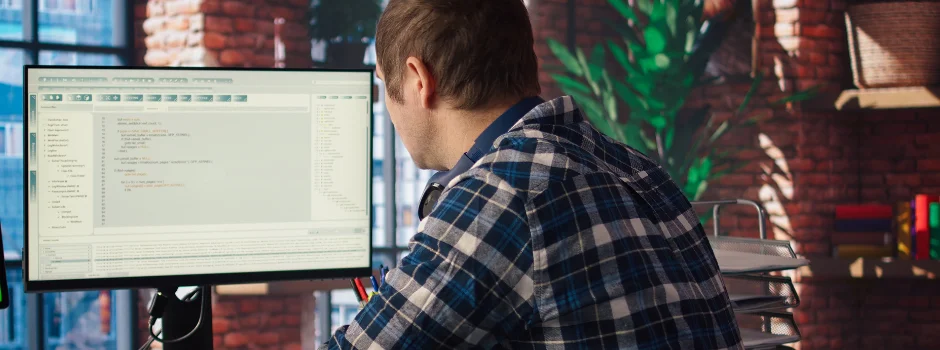
The Art of Monkey Testing Using Robot Framework
Mar 01, 2025 5 Min Read 446 Views
(Last Updated)
How do you ensure your software can handle unpredictable user behavior without breaking? In an era where applications must be robust and reliable, traditional testing methods often fail to capture the randomness of real-world usage.
Among the myriad testing techniques, Monkey Testing stands out for its unique approach to uncovering defects. This blog explores the concept of Monkey Testing and how it can be effectively implemented using the Robot Framework, a powerful tool for automated testing.
Table of contents
- What is Monkey Testing?
- The Robot Framework: A Brief Overview
- Setting Up the Environment
- Designing Monkey Test Cases
- Implementing Monkey Testing with Robot Framework
- Best Practices for Monkey Testing
- The Future of Monkey Testing
- Conclusion
What is Monkey Testing?
Monkey Testing, also known as Random Testing, is a type of automated testing where random inputs are provided to the application to explore unexpected behaviors and potential bugs.
The core idea is to simulate the actions of a monkey randomly interacting with the system, hence the name. Unlike structured testing techniques that follow predefined test cases, this testing thrives on randomness, which can lead to discovering edge cases and hidden issues that might otherwise go unnoticed.
The value of this testing technique lies in its ability to uncover rare and elusive bugs that traditional testing methods might miss. While systematic testing is crucial for verifying specific functionalities, this adds an extra layer of robustness by challenging the application with unexpected and often chaotic inputs.
It’s an excellent strategy for stress testing and for ensuring that the application can handle erratic user behavior without crashing or malfunctioning.
The Robot Framework: A Brief Overview
Before diving into the implementation of Monkey Testing, it’s essential to understand the tool we’ll be using—the Robot Framework. This is an open-source automation framework that supports both keyword-driven and behavior-driven development.
The Robot Framework’s key features include:
- Keyword-Driven Testing: Allows the creation of test cases using reusable keywords.
- Extensibility: Supports various libraries and can be integrated with other tools and frameworks.
- Easy Syntax: Utilizes a human-readable syntax, making it accessible even to non-programmers.
- Rich Reporting: Provides detailed logs and reports to analyze test outcomes.
Setting Up the Environment
To get started with Monkey Testing using the Robot Framework, you need to set up your testing environment. This involves installing the necessary tools and libraries. Here’s a step-by-step guide:
- Install Python: The Robot Framework is built on Python, so you’ll need Python installed on your system. You can download it from the official Python website.
- Install Robot Framework: You can install the Robot Framework using pip, Python’s package installer. Run the following command in your terminal:
3. Set Up Your Test Project: Create a new directory for your test project and set up the necessary files and folders.
Designing Monkey Test Cases
Designing test cases requires a different approach compared to traditional testing. Here are some key considerations:
- Random Input Generation: Develop mechanisms to generate random inputs, such as clicks, gestures, and text entries.
- Coverage and Duration: Decide on the coverage area and the duration of the test. This will determine how long the random actions will be performed.
- Logging and Monitoring: Ensure that all actions are logged and monitored to analyze the results and identify issues.
Implementing Monkey Testing with Robot Framework
With your environment set up and test cases designed, it’s time to implement Monkey Testing using the Robot Framework. Here’s a basic example to get you started:-
*** Settings ***
Library SeleniumLibrary
Library Collections
Library BuiltIn
Library String
Library OperatingSystem
Suite Setup Setup Browser
Suite Teardown Close Browser
# Code Execution Command : robot -d Results TestCases/MonkeyTesting.robot
*** Variables ***
${URL} https://sevengramscaffe.com/
${SCROLLING_POINT} xpath://a[text()='Privacy Policy']
${FOOTER_URL} xpath://li[@class='Linklist__Item']/a[@class='Link Link--primary']
*** Test Cases ***
Perform Monkey Testing
[Documentation] Test case to perform Monkey Testing
Open Browser And Navigate To URL ${URL}
Scroll To Privacy Policy
Fetch Footer URLs
Execute Monkey Testing
*** Keywords ***
Setup Browser
[Documentation] Start Automation Testing
Open Browser ${URL} Chrome
Maximize Browser Window
Set Selenium Timeout 10 seconds
Open Browser And Navigate To URL
[Arguments] ${url}
Go To ${url}
Scroll To Privacy Policy
[Documentation] Scroll to the Privacy Policy section
Wait Until Element Is Visible ${SCROLLING_POINT}
Scroll Element Into View ${SCROLLING_POINT}
Fetch Footer URLs
[Documentation] Fetch all footer URLs from the page
@{footer_elements}= Get WebElements ${FOOTER_URL}
${footer_count}= Get Length ${footer_elements}
Log Total Footer Links: ${footer_count}
${footer_urls}= Create List
FOR ${element} IN @{footer_elements}
${href}= Get Element Attribute ${element} href
Append To List ${footer_urls} ${href}
END
Set Suite Variable ${FOOTER_URL_LIST} ${footer_urls}
Execute Monkey Testing
[Documentation] Perform Monkey Testing on all footer URLs
${run_count}= Set Variable 0
${random_time}= Set Variable 0
FOR ${url} IN @{FOOTER_URL_LIST}
Go To ${url}
${run_count}= Evaluate ${run_count} + 1
Log Running the Test: ${run_count} & URL: ${url}
${random_time}= Evaluate random.randint(0, 2) modules=random
Sleep ${random_time}
Go Back
END
Close Browser
[Documentation] Close the browser after execution
Close All Browsers
This Robot Framework script performs a basic form of “monkey testing” on the footer links of the website https://sevengramscaffe.com/. Let’s break down the code section by section:
1. Settings:
- Library SeleniumLibrary: Enables interaction with web browsers.
- Library Collections: Provides utilities for working with lists.
- Library BuiltIn: Offers built-in keywords for various tasks.
- Library String: Provides keywords for string manipulation.
- Library OperatingSystem: Provides access to operating system functionalities.
- Suite Setup Setup Browser: Runs the Setup Browser keyword before any test cases in the suite.
- Suite Teardown Close Browser: Runs the Close Browser keyword after all test cases in the suite have finished.
- # Code Execution Command : robot -d Results TestCases/MonkeyTesting.robot: This is a comment showing how to execute the test suite from the command line. robot is the test runner, -d Results specifies the output directory, and TestCases/MonkeyTesting.robot is the path to the test file.
2. Variables:
- ${URL}: Stores the base URL of the website.
- ${SCROLLING_POINT}: Stores the XPath locator for the “Privacy Policy” link. This is used to ensure the page is loaded sufficiently before interacting with the footer.
- ${FOOTER_URL}: Stores the XPath locator for all the footer links.
3. Test Cases:
- Perform Monkey Testing: This is the main test case.
- [Documentation] Test case to perform Monkey Testing: A brief description of the test case.
- Open Browser And Navigate To URL ${URL}: Opens the Chrome browser and navigates to the specified URL.
- Scroll To Privacy Policy: Scrolls the page to the “Privacy Policy” link. This acts as a check to ensure the page is loaded and to potentially bring the footer into view.
- Fetch Footer URLs: Retrieves all the URLs from the footer links and stores them in a list.
- Execute Monkey Testing: Iterates through the collected footer URLs, visits each one, waits a random small amount of time, and then goes back to the previous page.
4. Keywords:
- Setup Browser:
- [Documentation] Start Automation Testing: A description.
- Open Browser ${URL} Chrome: Opens the Chrome browser and navigates to the URL.
- Maximize Browser Window: Maximizes the browser window.
- Set Selenium Timeout 10 seconds: Sets a timeout of 10 seconds for Selenium operations.
- Open Browser And Navigate To URL:
- [Arguments] ${url}: Takes the URL as an argument.
- Go To ${url}: Navigates to the specified URL.
- Scroll To Privacy Policy:
- [Documentation] Scroll to the Privacy Policy section: A description.
- Wait Until Element Is Visible ${SCROLLING_POINT}: Waits until the “Privacy Policy” element is visible.
- Scroll Element Into View ${SCROLLING_POINT}: Scrolls the page to bring the “Privacy Policy” element into the viewport.
- Fetch Footer URLs:
- [Documentation] Fetch all footer URLs from the page: A description.
- @{footer_elements}= Get WebElements ${FOOTER_URL}: Gets all the footer link elements using the XPath locator.
- ${footer_count}= Get Length ${footer_elements}: Gets the number of footer links.
- Log Total Footer Links: ${footer_count}: Logs the number of footer links.
- The loop iterates through each footer element, extracts the href attribute (the URL), and appends it to the footer_urls list.
- Set Suite Variable ${FOOTER_URL_LIST} ${footer_urls}: Makes the footer_urls list available to other test cases or keywords within the suite.
- Execute Monkey Testing:
- [Documentation] Perform Monkey Testing on all footer URLs: A description.
- The loop iterates through each URL in the FOOTER_URL_LIST.
- Go To ${url}: Navigates to the current URL.
- Log Running the Test: ${run_count} & URL: ${url}: Logs the test run number and the current URL.
- ${random_time}= Evaluate random.randint(0, 2) modules=random: Generates a random integer between 0 and 2 (inclusive) to simulate varying user interaction times.
- Sleep ${random_time}: Pauses the execution for the randomly generated time.
- Go Back: Navigates back to the previous page.
- Close Browser:
- [Documentation] Close the browser after execution: A description.
- Close All Browsers: Closes all open browser instances.
In summary: This script automates the process of visiting each footer link on the specified website. The “monkey testing” aspect is the random sleep time, which simulates a user clicking links at slightly different intervals.
Best Practices for Monkey Testing
While Monkey Testing is inherently random, there are best practices that can help guide its implementation:
- Define the Scope: Clearly define the areas of the application that will be subjected to Monkey Testing. This ensures that the testing efforts are focused and relevant.
- Monitor and Analyze: Continuously monitor the testing process and analyze the results. Detailed logs and reports are essential for identifying and understanding the issues that arise.
- Iterate and Improve: Use the insights gained to refine and improve the application. Treat each testing cycle as an opportunity to learn and enhance the software.
- Balance with Structured Testing: Maintain a balance between Monkey Testing and structured testing methods. Both approaches have their strengths, and together they provide a more comprehensive testing strategy.
The Future of Monkey Testing
As software development practices continue to evolve, so too will the methodologies and tools used for testing. Monkey Testing, with its emphasis on randomness and unpredictability, will remain a valuable technique for ensuring application robustness.
The Robot Framework, with its ongoing development and community support, will continue to be a versatile and powerful tool for implementing various testing strategies.
Looking ahead, we can expect to see further innovations in the field of automated testing. Advancements in artificial intelligence and machine learning, for example, have the potential to enhance Monkey Testing by making it even more intelligent and effective.
By leveraging these technologies, testers can develop more sophisticated algorithms for generating random inputs and predicting potential issues.
In case you want to learn more about automation testing techniques, consider enrolling for GUVI’s Selenium Automation Testing Course which teaches you everything from scratch by providing an industry-grade certificate!
Conclusion
In conclusion, Monkey Testing using the Robot Framework represents a dynamic and effective approach to software testing. By embracing the chaos of randomness, testers can uncover hidden vulnerabilities and ensure that applications are resilient and reliable.
As we continue to push the boundaries of what software can achieve Monkey Testing will remain a valuable tool for achieving excellence in software development.
Did you enjoy this article?