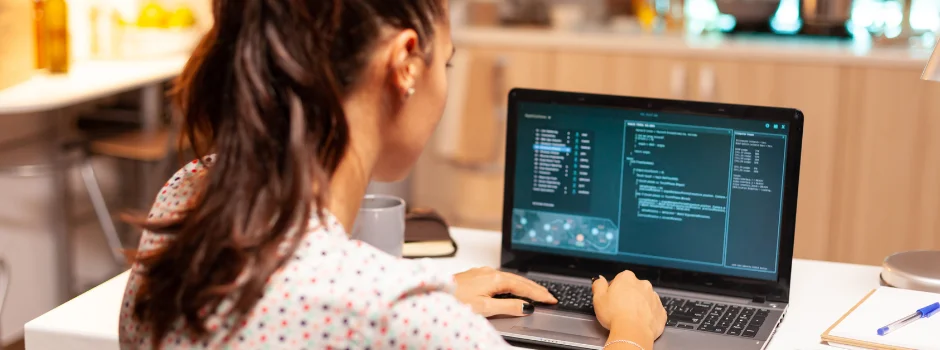
Mastering Monkey Testing with Python Selenium
Mar 01, 2025 6 Min Read 424 Views
(Last Updated)
How can you ensure your web application is truly robust and resilient against unpredictable user behavior? Traditional testing methods follow structured test cases, but real-world users don’t always interact with an application in a predictable manner.
This is where Monkey Testing comes into play, a technique that introduces randomness into the testing process to simulate unexpected user interactions. By using Python Selenium, testers can automate these chaotic interactions, pushing applications to their limits and uncovering hidden bugs that might go unnoticed with conventional testing.
In this article, we’ll explore how Monkey Testing works, why Python Selenium is an excellent choice for it, and how you can implement it effectively with a practical example. So, let us get started!
Table of contents
- Understanding Monkey Testing
- Python Selenium: A Powerful Combination
- Why Use Python Selenium for Monkey Testing?
- Getting Started with Monkey Testing Using Python Selenium
- Writing a Simple Monkey Testing Script
- Conclusion
Understanding Monkey Testing
Monkey Testing, also known as random testing, is a software testing technique where the tester enters random inputs into the system to check for any crashes, unexpected behaviors or performance issues.
The primary objective is to push the application to its limits and uncover hidden bugs that might not be discovered through conventional testing methods. This type of testing is especially useful for stress testing, load testing, and discovering edge cases.
Python Selenium: A Powerful Combination
Selenium, a popular open-source tool, is widely used for automating web browsers. It provides a robust framework for testing web applications across different browsers and platforms. Python with its simplicity and readability is a favored language among developers and testers alike.
Combining Python with Selenium creates a powerful toolset for automating web testing, including the execution of Monkey Testing.
Why Use Python Selenium for Monkey Testing?
- Ease of Use: Python’s syntax is easy to learn, making it an ideal choice for writing test scripts. Selenium’s well-documented APIs further simplify the automation process.
- Cross-Browser Testing: Selenium supports multiple browsers allowing testers to perform Monkey Testing across various environments and ensure consistent behavior.
- Integration Capabilities: Selenium can be easily integrated with other testing frameworks and tools, enhancing the overall testing process.
- Scalability: Python Selenium scripts can be scaled to perform extensive testing on large applications, ensuring thorough coverage.
Getting Started with Monkey Testing Using Python Selenium
To embark on your Monkey Testing journey, you need to set up your environment with Python and Selenium. Here are the steps to get started:
- Install Python: Ensure you have Python installed on your system. You can download it from the official Python website.
- Install Selenium: Use pip Python’s package installer to install Selenium. Run the following command in your terminal:
- Set Up WebDriver: Download the WebDriver for the browser you intend to test. For example, ChromeDriver for Google Chrome or GeckoDriver for Mozilla Firefox. Here, we will be using Python Selenium WebDriver Manager for the same as it will download and install the desired WebDriver Manager based on the web browser we will use for Python Automation Testing.
Writing a Simple Monkey Testing Script
Here’s an example of a basic Monkey Testing script using Python Selenium, which is given as below:-
"""
Program Description : Python program to perform Monkey Testing
Programmer : Suman Gangopadhyay
Email ID : suman@guvi.in
Version : 1.0
Code Library : Selenium
Prerequisites : Python, Selenium, ChromeDriver
Caveats : None
"""
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.common.exceptions import TimeoutException
from selenium.common.exceptions import NoSuchElementException
from selenium.common.exceptions import ElementNotVisibleException
from selenium.common.exceptions import ElementClickInterceptedException
from selenium.webdriver.common.action_chains import ActionChains
from time import sleep
from random import random
# Locators Class
class Locators:
SCROLLING_POINT = "//a[text()='Privacy Policy']"
FOOTER_URL = "//li[@class='Linklist__Item']/a[@class='Link Link--primary']"
# Monkey Testing Class
class MonkeyTesting(Locators):
"""
DESCRIPTION : Class to perform Monkey Testing
"""
def __init__(self, url):
self.url = url
self.driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
self.EXCEPTIONS = (TimeoutException, NoSuchElementException, ElementNotVisibleException, ElementClickInterceptedException)
self.wait = WebDriverWait(self.driver, 10, poll_frequency = 5, ignored_exceptions = self.EXCEPTIONS)
def monkey_testing(self):
"""
DESCRIPTION : Method to perform Monkey Testing
"""
try:
self.driver.maximize_window()
self.driver.get(self.url)
action = ActionChains(self.driver)
scrolling_point = self.wait.until(EC.visibility_of_element_located((By.XPATH, self.SCROLLING_POINT)))
action.move_to_element(scrolling_point).perform()
footer_list = self.wait.until(EC.visibility_of_all_elements_located((By.XPATH, self.FOOTER_URL)))
footer_count = len(footer_list)
"""Fetch URLs from Footer"""
footer_url = []
for data in footer_list:
footer_url.append(data.get_attribute('href'))
"""Monkey Testing"""
run_count = 0
for random_url in range(footer_count):
self.driver.get(footer_url[random_url])
run_count = run_count + 1
sleep(random())
self.driver.back()
print(f"Running the Test: {run_count} & URL: {footer_url[random_url]}")
sleep(random())
except self.EXCEPTIONS as error:
print(error)
finally:
self.driver.quit()
# Main Function
if __name__ == "__main__":
url = "https://sevengramscaffe.com/"
testing = MonkeyTesting(url)
testing.monkey_testing()
1. Imports and Setup
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.common.exceptions import TimeoutException
from selenium.common.exceptions import NoSuchElementException
from selenium.common.exceptions import ElementNotVisibleException
from selenium.common.exceptions import ElementClickInterceptedException
from selenium.webdriver.common.action_chains import ActionChains
from time import sleep
from random import random
The code begins by importing the necessary libraries and modules. It includes Selenium for browser automation webdriver_manager to manage browser drivers and several Selenium classes for handling web elements, exceptions and actions.
The time module is used to introduce delays, while the random module generates random numbers for simulating random interactions.
2. Locators Class
# Locators Class
class Locators:
SCROLLING_POINT = "//a[text()='Privacy Policy']"
FOOTER_URL = "//li[@class='Linklist__Item']/a[@class='Link Link--primary']"
The Locators class defines two XPath locators:
- SCROLLING_POINT : An XPATH locator for an element that the script will scroll to.
- FOOTER_URL : An XPATH locator for the footer links on the web page.
3. Monkey Testing Class
# Monkey Testing Class
class MonkeyTesting(Locators):
"""
DESCRIPTION : Class to perform Monkey Testing
"""
def __init__(self, url):
self.url = url
self.driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
self.EXCEPTIONS = (TimeoutException, NoSuchElementException, ElementNotVisibleException, ElementClickInterceptedException)
self.wait = WebDriverWait(self.driver, 10, poll_frequency = 5, ignored_exceptions = self.EXCEPTIONS)
The MonkeyTesting class inherits from the Locators class and includes the following:
- __init__ method : Initializes the class with the provided URL sets up the Chrome WebDriver defines the exceptions to be handled and creates a WebDriverWait instance for waiting on elements
4. Performing Monkey Testing
def monkey_testing(self):
"""
DESCRIPTION : Method to perform Monkey Testing
"""
try:
self.driver.maximize_window()
self.driver.get(self.url)
action = ActionChains(self.driver)
scrolling_point = self.wait.until(EC.visibility_of_element_located((By.XPATH, self.SCROLLING_POINT)))
action.move_to_element(scrolling_point).perform()
footer_list = self.wait.until(EC.visibility_of_all_elements_located((By.XPATH, self.FOOTER_URL)))
footer_count = len(footer_list)
"""Fetch URLs from Footer"""
footer_url = []
for data in footer_list:
footer_url.append(data.get_attribute('href'))
"""Monkey Testing"""
run_count = 0
for random_url in range(footer_count):
self.driver.get(footer_url[random_url])
run_count = run_count + 1
sleep(random())
self.driver.back()
print(f"Running the Test: {run_count} & URL: {footer_url[random_url]}")
sleep(random())
except self.EXCEPTIONS as error:
print(error)
finally:
self.driver.quit()
.
The monkey_testing method performs the actual Monkey Testing:
- Maximizes the browser window and navigates to the specified URL.
- Creates an ActionChains instance for performing actions.
- Waits for the scrolling point element to be visible and scrolls to it.
- Waits for all footer links to be visible and counts them.
- Fetches the URLs from the footer links and stores them in a list.
- Randomly selects URLs from the footer, navigates to them, introduces random delays, navigates back, and prints the current test run details.
5. Main Function
# Main Function
if __name__ == "__main__":
url = "https://sevengramscaffe.com/"
testing = MonkeyTesting(url)
testing.monkey_testing()
The main function initializes the MonkeyTesting class with the target URL and calls the monkey_testing method to start the testing process.
In case you want to learn more about Python Selenium, consider enrolling for GUVI’s Selenium Automation Testing Course which teaches you everything from scratch by providing an industry-grade certificate!
Conclusion
In conclusion, Monkey Testing using Python Selenium presents a unique and effective approach to ensuring the robustness and reliability of web applications. By introducing randomness and unpredictability into the testing process it simulates real-world usage scenarios that traditional testing methods might overlook. This technique is particularly valuable for uncovering hidden bugs, stress testing the application, and ensuring that it can handle unexpected user behaviors and inputs.
The provided code example demonstrates how to set up and perform Monkey Testing using Python Selenium. It includes essential components such as importing necessary libraries, defining locators, and creating a class for performing the testing.
The script navigates to the specified URL, interacts with web elements, and introduces random delays to simulate unpredictable user interactions. By repeatedly navigating to different URLs and performing actions, the script helps identify potential issues that might not be discovered through structured testing.
Did you enjoy this article?