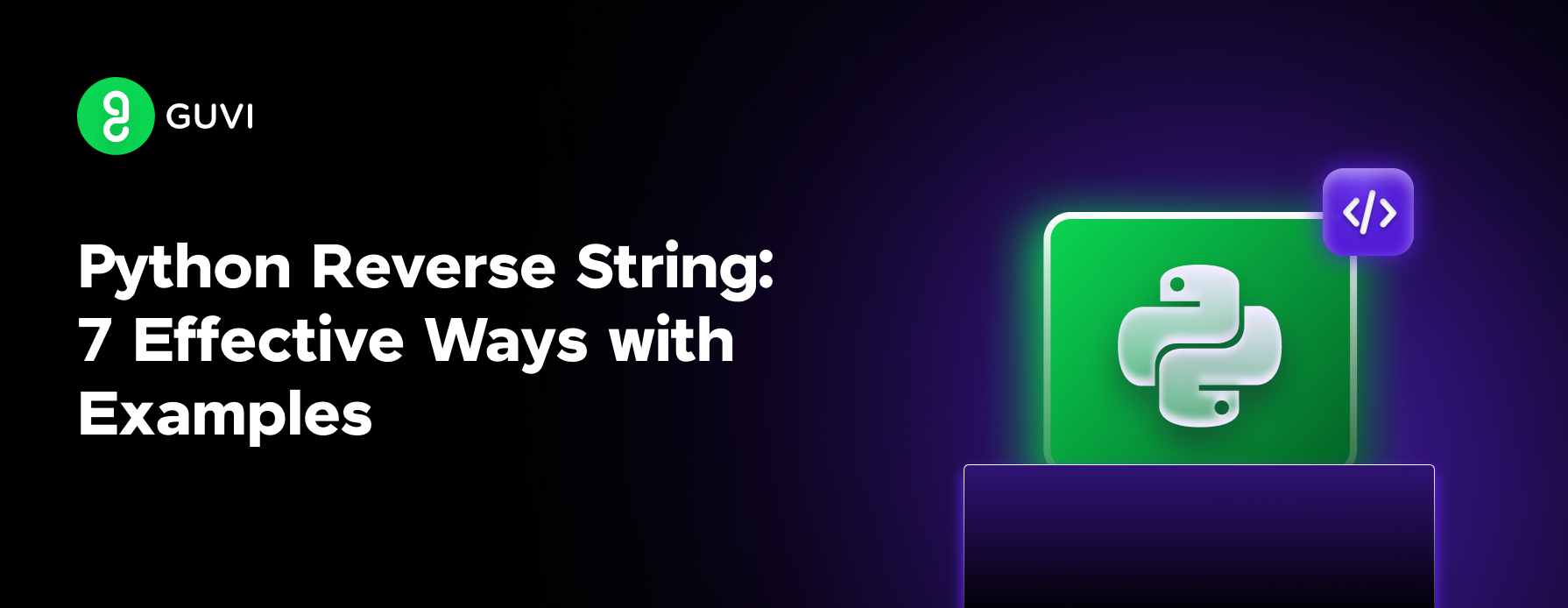
Python Reverse String: 7 Effective Ways with Examples
Oct 01, 2024 8 Min Read 3930 Views
(Last Updated)
In the versatile world of programming, the ability to reverse a string in Python stands as a fundamental yet crucial skill that every coder should master.
Understanding how to Python reverse string not only sharpens your problem-solving skills but also opens up a myriad of possibilities for manipulating data and implementing algorithms.
This article delves into seven effective methods for reversing strings in Python, providing you with a comprehensive guide to tackle this common problem.
Table of contents
- 1) Python Reverse String Using Slicing
- 1) Basics of Slicing
- 2) Reversing with Negative Step
- 3) Practical Examples:
- 2) Reversing Strings Using the reversed() Function
- 1) Using reversed() and join()
- 2) Code Examples
- 3) Reversing Strings Using a Loop
- 1) Using for Loop
- 2) Using while Loop
- 3) Code Examples
- 4) Reversing Strings Using Recursion
- 1) Understanding Recursion
- 2) Base Case and Recursive Case
- 3) Code Examples
- 5) Reversing Strings Using a Stack
- 1) Stack Basics
- 2) Reversing String with Stack
- 3) Code Examples
- 6) Reversing Strings Using List Comprehension
- 1) List Comprehension Basics
- 2) Code Examples
- 7) Reversing Strings Using Functional Programming
- 1) Using reduce() Function
- 2) Code Examples
- Concluding Thoughts...
- FAQs
- Can you use reverse () on a string?
- How to reverse a string in Python without a reverse function?
- What is a reversed function in Python?
- What is reverse True in Python?
1) Python Reverse String Using Slicing
1.1) Basics of Slicing
- Slicing is a powerful technique in Python that allows you to extract portions of a sequence by specifying a start, stop, and step index.
- When applied to strings, this method enables you to access individual characters or substrates efficiently. To reverse a string using slicing, you utilize the syntax
a_string[start:stop:step]
.
- Here,
start
refers to the index where the slice begins,stop
is where it ends, andstep
determines the stride between each character in the slice.
1.2) Reversing with Negative Step
- The key to reversing a string with slicing lies in the
step
parameter. By settingstep
to-1
, you instruct Python to retrieve characters in reverse order.
- For instance, if you have a string
letters = "ABCDEF"
, executingletters[::-1]
would result in'FEDCBA'
.
- This technique leverages negative indexing to start from the end of the string and proceed backward to the beginning.
- It’s a succinct and efficient way to create a reversed copy of the string without modifying the original.
Find Out Top 12 Key Benefits of Learning Python in 2024
1.3) Practical Examples:
Let’s explore the practical applications of reversing strings using slicing. Consider the string txt = "Hello World"
.
To reverse it, you simply use the slice txt[::-1]
, which outputs "dlroW olleH"
. This method is not only fast but also easy to read and implement.
For scenarios where you need to reverse strings frequently, you can encapsulate this functionality within a function:
def reverse_string(s):
return s[::-1]
# Usage
reversed_txt = reverse_string("Hello World")
print(reversed_txt)
Output:
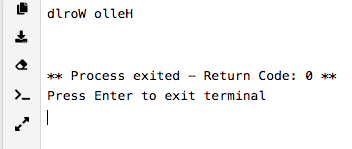
Moreover, if you require more control over the slicing, you can use the slice()
function. This built-in function allows you to define slicing behavior explicitly:
letters = "ABCDEF"
reversed_letters = letters[slice(None, None, -1)]
print(reversed_letters)
Output:
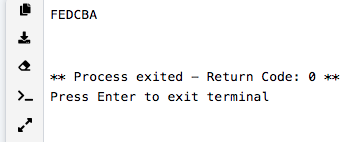
Passing None
for the start
and stop
parameters tells Python to use the default start and end indices, effectively slicing the entire string from end to start.
By mastering these slicing techniques, you enhance your ability to handle string manipulations in Python, making your code both efficient and elegant.
Before diving into the next section, ensure you’re solid on Python essentials from basics to advanced level. If you are looking for a detailed Python career program, you can join GUVI’s Python Career Program with placement assistance. You will be able to master the Multiple Exceptions, classes, OOPS concepts, dictionary, and many more, and build real-life projects.
Also, if you would like to explore Python through a Self-Paced course, try GUVI’s Python Self-Paced course.
2) Reversing Strings Using the reversed() Function
2.1) Using reversed() and join()
- One of the most Pythonic ways to reverse a string is by combining the
reversed()
function with thestr.join()
method.
- When you pass a string to
reversed()
, it doesn’t immediately return a reversed string. Instead, it provides a special reversed iterator object.
- This iterator yields characters from the string in reverse order, allowing you to access each character starting from the end of the original string.
- To convert this iterator into a string, you can use the
"".join()
method. This method takes an iterable as an argument and concatenates its elements separated by the string used before.join()
, which in this case is an empty string. Here’s how it works:
reversed_result = reversed("Hello, World!")
reversed_string = "".join(reversed_result)
print(reversed_string)
Output:
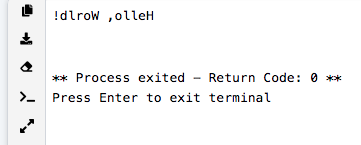
This method is particularly efficient because it reads characters directly from the original string without creating a new reversed string. This behavior minimizes memory usage, making it a desirable choice in scenarios where performance and efficiency are critical.
Also Read: How to remove an element from a list in Python? 4 Methods
2.2) Code Examples
Here’s a practical example of using the reversed()
function to reverse a string in Python:
def reverse_string(input_string):
# The reversed() function returns a reversed iterator
reversed_iterator = reversed(input_string)
# The "".join() method concatenates the elements of the iterator into a new string
reversed_string = "".join(reversed_iterator)
return reversed_string
# Example usage
original_string = "GUVI GEEK"
print("The original string is:", original_string)
reversed_string = reverse_string(original_string)
print("The reversed string(using reversed) is:", reversed_string)
Output:
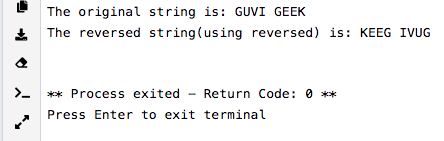
In this example, the reverse_string
function takes an input string, applies the reversed()
function to create an iterator, and then uses "".join()
to form the reversed string. This method is straightforward and leverages Python’s powerful built-in functions to perform the task efficiently.
By understanding and utilizing these techniques, you can effectively reverse strings in Python, enhancing both the readability and performance of your code.
3) Reversing Strings Using a Loop
Reversing strings using loops in Python is a straightforward method that can be accomplished either through a for
loop or a while
loop.
These approaches are particularly useful when you need a high degree of control over the string manipulation process.
3.1) Using for Loop
The for
loop method involves iterating over the string in reverse order and appending each character to a new string. Here’s a simple implementation:
def reverse_for_loop(s):
s1 = ''
for c in s:
s1 = c + s1 # appending chars in reverse order
return s1
input_str = 'Get extensive placement assistance with GUVI!'
print('Reverse String using for loop =', reverse_for_loop(input_str))
Output:
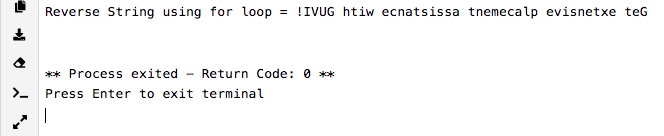
In this code, s1
starts as an empty string. Each iteration of the loop prepends the current character to s1
, effectively building the string in reverse order.
Must Explore: 16 Famous Tech Companies That Use Python In 2024
3.2) Using while Loop
Alternatively, you can use a while
loop to reverse a string by decrementing the index:
def reverse_while_loop(s):
s1 = ''
length = len(s) - 1
while length >= 0:
s1 += s[length]
length -= 1
return s1
input_str = 'Learn in your native language with GUVI!'
print('Reverse String using while loop =', reverse_while_loop(input_str))
Output:
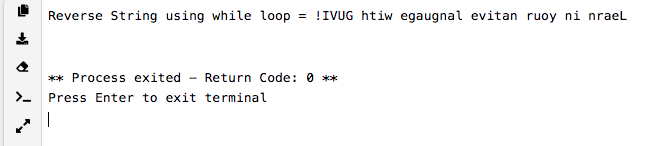
This method initializes s1
as an empty string and a length
variable set to the last index of the string s
. The loop continues until length
is less than 0, appending each character from the end of s
to s1
.
3.3) Code Examples
Both looping methods are effective for reversing strings and can be utilized based on the specific requirements of your application. Here are some additional examples to illustrate the usage of loops for string reversal:
- For Loop Example:
def reverse_for_loop(s):
s1 = ''
for c in s:
s1 = c + s1 # appending chars in reverse order
return s1
original_string = "GUVI GEEK"
reversed_string = reverse_for_loop(original_string)
print("The original string is:", original_string)
print("The reversed string using a for loop is:", reversed_string)
Output:
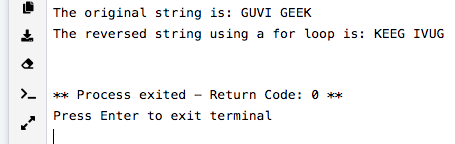
- While Loop Example:
def reverse_while_loop(s):
s1 = ''
length = len(s) - 1
while length >= 0:
s1 += s[length]
length -= 1
return s1
original_string = "Hello World!"
reversed_string = reverse_while_loop(original_string)
print("The original string is:", original_string)
print("The reversed string using a while loop is:", reversed_string)
Output:
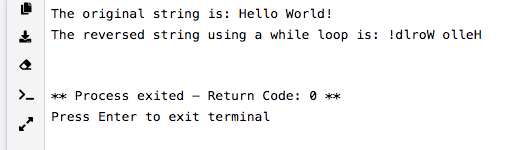
These examples demonstrate the flexibility of using loops to reverse strings in Python. Whether you choose a for
loop for its straightforward syntax or a while
loop for more control over the index, both methods provide robust solutions for string manipulation.
4) Reversing Strings Using Recursion
4.1) Understanding Recursion
- Recursion is a powerful programming paradigm where a function calls itself repeatedly to solve a problem by breaking it down into simpler versions of itself.
- In the context of reversing a string in Python, recursion involves taking the last character of the string and appending it to the reverse of the rest of the string.
- This process continues until a base case is reached.
4.2) Base Case and Recursive Case
- The base case in recursion is crucial as it determines when the recursive calls should stop. For reversing a string, the simplest base case is an empty string.
- If the string is empty, there’s nothing to reverse, and the function can simply return the empty string.
- The recursive case, on the other hand, involves taking the last character of the string and concatenating it with the reverse of the substring that excludes this character.
- This can be expressed with the following Python code:
def reverse_string(s):
if s == "":
return s
else:
return s[-1] + reverse_string(s[:-1])
This function checks if the string is empty. If not, it takes the last character (s[-1]
) and concatenates it with the result of a recursive call to reverse_string
on the rest of the string (s[:-1]
).
Must Read: Mastering Recursion in Python: A Comprehensive Guide [2024]
4.3) Code Examples
To illustrate recursion in action, let’s consider a practical example. Below is a Python function that uses recursion to reverse a string, along with a demonstration of its use:
def reverse_string(s):
if len(s) == 0:
return s
else:
return reverse_string(s[1:]) + s[0]
# Example usage
original_string = "hello"
reversed_string = reverse_string(original_string)
print("Original string:", original_string)
print("Reversed string:", reversed_string)
Output:
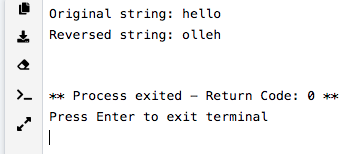
In this example, reverse_string
is called with the string “hello”. Each call to reverse_string
slices the string until it’s empty, at which point the concatenation of characters begins in reverse order, building up the reversed string as the recursion unwinds.
This method, while elegant and a good demonstration of recursion, is not the most efficient for reversing strings in Python due to the limitations of Python’s recursion depth and the overhead of multiple function calls.
However, it is a valuable technique to understand for its application in solving other recursive problems.
5) Reversing Strings Using a Stack
5.1) Stack Basics
- A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means the last element added to the stack is the first one to be removed.
- Utilizing a stack to reverse a string is an intuitive method because of this inherent characteristic.
- By pushing all characters of a string onto a stack and then popping them off, you inherently reverse the order due to the stack’s LIFO nature.
5.2) Reversing String with Stack
To reverse a string using a stack in Python, follow these steps:
- Create an empty stack: This will hold the characters of the string temporarily.
- Push all characters of the string to the stack: Iterate over the string and push each character onto the stack.
- Pop all characters from the stack and put them back into the string: By popping the characters from the stack and appending them back to an initially empty string, you effectively reverse the string.
Here’s a step-by-step breakdown:
- Initialization: Start with an empty stack.
- Pushing to Stack: Loop through the string and push each character onto the stack.
- Popping from Stack: Create a new empty string and append each character popped from the stack.
This method is efficient and easy to implement, making it a popular choice for string reversal tasks.
Also Read: 8 Beginner Python Projects to Kickstart Your Journey (with code)
5.3) Code Examples
Here is a Python code example demonstrating how to reverse a string using a stack:
def reverse_string_using_stack(input_string):
# Create an empty list to use as a stack
stack = []
# Push all characters of string to stack
for char in input_string:
stack.append(char)
# Pop all characters from stack and put them back to a new string
reversed_string = ''
while stack:
reversed_string += stack.pop()
return reversed_string
# Example usage
original_string = "hello"
reversed_string = reverse_string_using_stack(original_string)
print("Original string:", original_string , "Reversed string:", reversed_string)
Output:
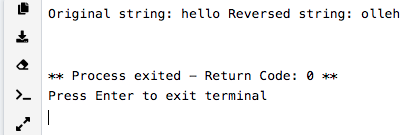
This function reverse_string_using_stack
takes a string as input, uses a list as a stack to reverse the string, and then returns the reversed string. The example demonstrates reversing the string “hello” to “olleh”.
By understanding and implementing these techniques, you can effectively utilize stacks for various string manipulation tasks, enhancing both the readability and functionality of your Python code.
6) Reversing Strings Using List Comprehension
6.1) List Comprehension Basics
- List comprehension in Python provides a concise way to apply an operation to each element in a list. This method is not only syntactically cleaner but also enhances code readability and efficiency.
- When reversing strings, list comprehension allows you to iterate over each string in a list and apply the reverse operation in a single line of code.
- The general syntax for a list comprehension that reverses strings is:
reversed_strings = [s[::-1] for s in original_list]
Here, s[::-1]
applies the slicing operation to each string s
in the original_list
, reversing each string. This approach is particularly useful when dealing with lists of strings that need to be reversed quickly and efficiently.
Also Read: Python: How To Iterate Through Two Lists In Parallel?
6.2) Code Examples
To demonstrate the effectiveness of list comprehension in reversing strings, consider the following practical examples:
- Reversing a List of Strings:
# Initializing list
test_list = ["GUVI", "GEEK", "is", "best", "for" , "students"]
# Printing original list
print("The original list is:", test_list)
# Using list comprehension to reverse all strings in the list
reversed_list = [item[::-1] for item in test_list]
# Printing reversed list
print("The reversed string list is:", reversed_list)
Output:
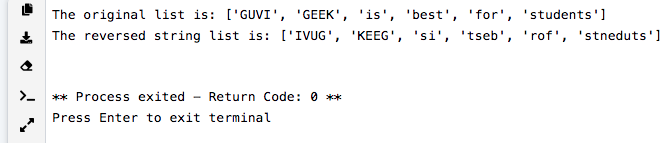
- Applying a Custom Reverse Function:
Sometimes, you might want to use a custom function to reverse strings. Here’s how you can integrate a custom reverse function within a list comprehension:
def reverseWord(word):
return word[::-1]
myList = ["string1", "string2", "string3"]
# Applying the reverseWord function on each word using a list comprehension
reversedWords = [reverseWord(word) for word in myList]
print("Reversed words:", reversedWords)
Output:
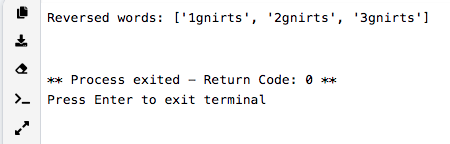
These examples illustrate the power and flexibility of list comprehensions for reversing strings in Python. By using this technique, you can streamline your code and focus on more complex logic while maintaining readability and performance.
Also Explore: 20 Best YouTube Channels to Learn Python in 2024
7) Reversing Strings Using Functional Programming
7.1) Using reduce() Function
- If you’re inclined towards a functional programming approach in Python, the
reduce()
function from thefunctools
module is a powerful tool you can utilize.
- Unlike other methods,
reduce()
takes a function and an iterable and applies the function cumulatively to the items of the iterable from left to right, reducing the iterable to a single value.
- This characteristic can be cleverly used to reverse strings.
Here’s a concise explanation of how reduce()
can be employed to reverse strings:
- Function Definition: You define a lambda function that takes two arguments,
a
andb
. The function placesb
beforea
, effectively reversing the order when applied repeatedly across a string. - Application of reduce(): The
reduce()
function iterates over the string, applying the lambda function to accumulate a final result which is the reversed string.
7.2) Code Examples
To put this into practice, consider the following Python code snippet:
from functools import reduce
def reversed_string(text):
return reduce(lambda a, b: b + a, text)
# Example usage
example_text = "Hello, World!"
print("Original text:", example_text)
print("Reversed text:", reversed_string(example_text))
In this example:
- The string
"Hello, World!"
is passed to thereversed_string
function. reduce()
applies the lambda function across the string. For each character in"Hello, World!"
, the lambda function places the current character before the accumulated characters.- The final output from
reduce()
is the string reversed:"!dlroW ,olleH"
.
Output:
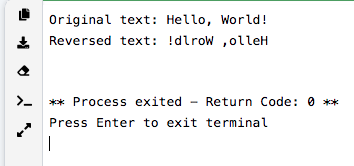
This method showcases not only the power of functional programming but also its elegance in executing tasks that might otherwise require more complex implementations.
Also Read: How To Use Global Variables Inside A Function In Python?
To further demonstrate the versatility of reduce()
in handling multiple strings, consider reversing a list of strings:
from functools import reduce
test_list = ["Go", "vernacular", "with", "GUVI"]
print("Original list:", test_list)
# Using reduce() to reverse each string in the list
reversed_list = [reduce(lambda x, y: y + x, string) for string in test_list]
print("Reversed string list:", reversed_list)
Output:
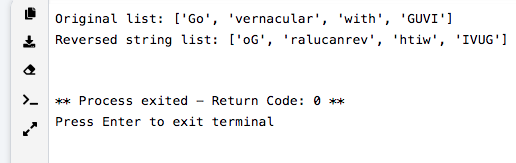
In this scenario, reduce()
is applied to each string in the list using a list comprehension. Each string is reversed individually, demonstrating the function’s ability to work with collections of strings efficiently.
This example not only reinforces the concept of functional programming but also illustrates how it can simplify operations on data structures, making your code cleaner and more Pythonic.
Kickstart your Programming journey by enrolling in GUVI’s Python Career Program where you will master technologies like multiple exceptions, classes, OOPS concepts, dictionaries, and many more, and build real-life projects.
Alternatively, if you would like to explore Python through a Self-Paced course, try GUVI’s Python Self-Paced course.
Concluding Thoughts…
Throughout this article, we’ve explored a variety of methods to reverse strings in Python, each with its unique approach and application.
From the simplicity of slicing to the technical prowess required for using recursion and the functional programming model with the reduce()
function, these techniques highlight the versatility of Python in handling common programming tasks such as string reversal.
As you move forward, remember that the best solution is one that balances efficiency, readability, and performance to meet the demands of your project. Let these examples inspire you to experiment and discover the most fitting string reversal technique for your next Python venture.
Also, Find Out 18 Famous Websites Built with Python in 2024
FAQs
Can you use reverse () on a string?
No, the reverse()
method cannot be used on a string in Python as it is specifically designed for lists.
How to reverse a string in Python without a reverse function?
You can reverse a string in Python using slicing: reversed_string = original_string[::-1]
.
What is a reversed function in Python?
The reversed()
function in Python returns an iterator that accesses the given sequence in the reverse order. It works with sequences like lists, tuples, and strings.
What is reverse True in Python?
In Python, reverse=True
is an argument used with sorting functions like sorted()
and list.sort()
. It sorts the elements in descending order.
Did you enjoy this article?