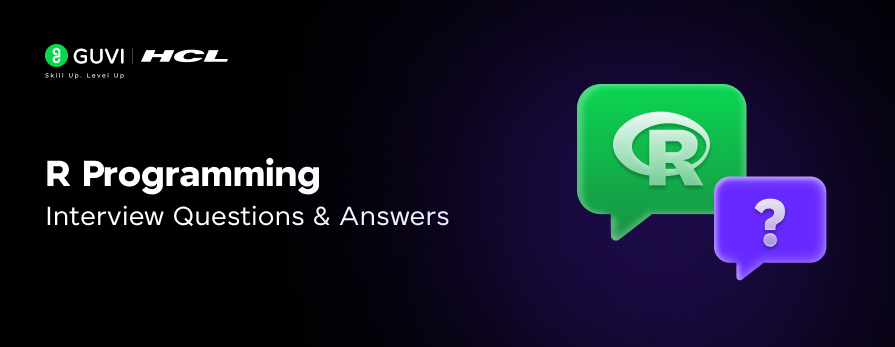
Top 45+ R Programming Interview Questions and Answers
Jan 09, 2025 11 Min Read 1161 Views
(Last Updated)
In the dynamic world of data science and analytics, R programming is one of the most powerful tools for statistical computing and data visualization. Whether you’re a budding data scientist or a seasoned professional aiming for top-tier roles, mastering R is crucial. Preparing for interviews requires theoretical understanding and practical expertise to tackle diverse scenarios confidently.
In this article, I have listed 48 must-know R programming interview questions and answers, thoughtfully categorized into beginner, intermediate, and advanced levels. These questions are designed to help you navigate the challenges of coding assessments and theoretical discussions, setting you apart as a standout candidate.
Table of contents
- Top R Programming Interview Questions and Answers (Section-Wise)
- Beginner Level
- Intermediate Level
- Advanced Level
- Concluding Thoughts…
Top R Programming Interview Questions and Answers (Section-Wise)
I have divided all these important R Programming interview questions and answers into various sections for your ease of learning, I recommend covering the beginner level questions as a must and then going through all the sections one by one so that you can gain a well-rounded knowledge of how these interviews are undertaken and how much and what you should prepare.
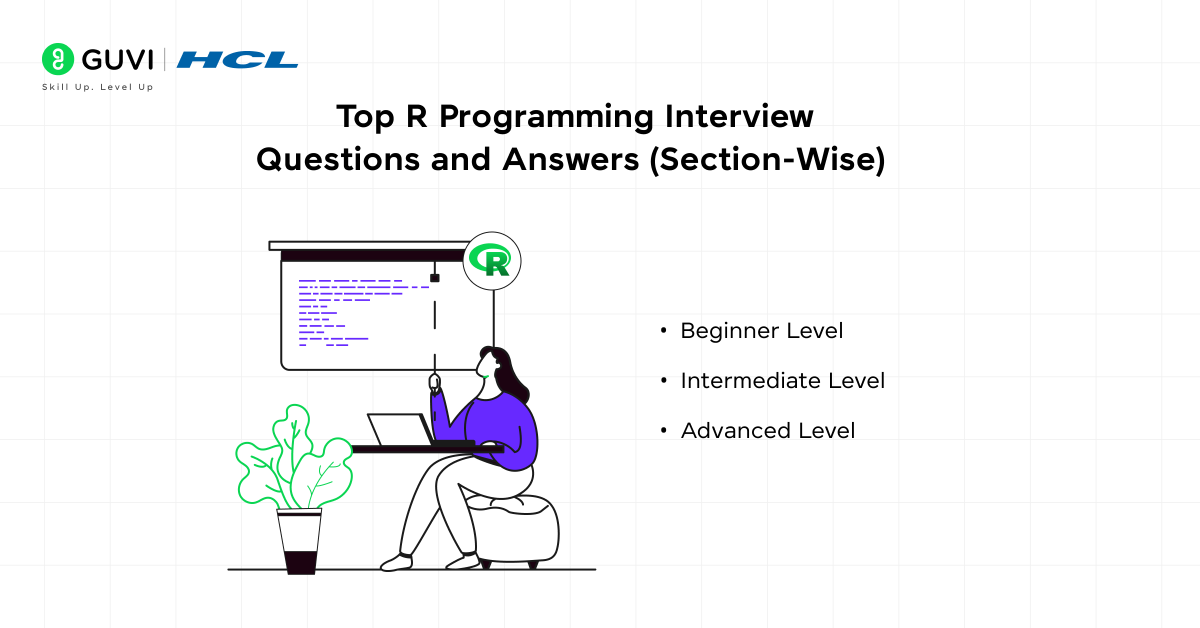
Beginner Level
1) What are the key features of R programming?
Answer:
R is a comprehensive statistical computing language known for:
- Data Manipulation and Visualization: Built-in support for robust data analysis and advanced graphical capabilities through packages like ggplot2 and lattice.
- Extensive Package Ecosystem: Thousands of CRAN packages extend R’s functionality for machine learning, time-series analysis, and more.
- Vectorized Operations: Optimized for efficient computation on vectors and matrices without explicit loops.
- Cross-Platform Compatibility: Runs seamlessly on Windows, macOS, and Linux.
- Open-Source: Freely available with an active community for support and contributions.
2) What is a vector in R? How do you create one?
Answer:
A vector in R is a one-dimensional array that holds elements of the same data type (numeric, character, logical, etc.). It is the simplest data structure in R and forms the basis of more complex structures like matrices and data frames.
Creation: Use the c() function. Example:
vector <- c(1, 2, 3, 4) # Numeric vector
3) Explain the difference between library() and require().
Answer:
- library(): Used to load a package and will throw an error if the package is not installed. Ideal for robust script execution.
require(): Loads the package but issues a warning instead of stopping the script if the package is unavailable. Typically used within functions to handle optional dependencies.
Example:
library(ggplot2) # Stops execution if ggplot2 is unavailable
if(require(dplyr)) # Continues with a warning if dplyr is unavailable
4) What is a factor in R? Why is it used?
Answer:
- Factors are categorical variables that store labels as levels, optimizing memory and computational efficiency.
- Use Case:
- Useful for statistical modeling, where categorical data needs to be distinguished from continuous variables.
Ensures consistent handling of categories in data frames and plotting.
Example:
factor_var <- factor(c("low", "medium", "high"), levels = c("low", "medium", "high"))
5) How do you check the structure of a dataset in R?
Answer:
- Use str() to display the dataset’s structure, including data types and dimensions.
Alternatively, use summary() for descriptive statistics or glimpse() (from dplyr) for a more compact view.
Example:
str(dataset) # Provides a concise structure summary
summary(dataset) # Gives statistical summaries for each column
6) Write a program to create a sequence of numbers from 5 to 15.
Answer:
In R, the seq() function is used to generate sequences with customizable steps. Here’s the program:
sequence <- seq(5, 15)
print(sequence)
Explanation:
- seq(5, 15) generates a sequence starting at 5 and ending at 15 with a default step of 1.
- For more control over the step size, you can use the by parameter, e.g., seq(5, 15, by=2).
7) What is the difference between data.frame and matrix in R?
Answer:
Feature | data.frame | matrix |
Data Types | Can hold different data types (e.g., numeric, character). | Homogeneous; all elements must be of the same type. |
Structure | Two-dimensional table with named rows/columns. | Two-dimensional array. |
Use Cases | Ideal for handling datasets, especially in data analysis. | Useful for mathematical operations. |
Access | Access via $ for columns (e.g., df$col). | Access by index only (e.g., matrix[1,1]). |
Example:
# Data Frame
df <- data.frame(name = c("A", "B"), score = c(90, 85))
# Matrix
mat <- matrix(1:4, nrow = 2, ncol = 2)
8) How do you read a CSV file in R?
Answer:
The read.csv() function is commonly used for reading CSV files into a data frame in R.
data <- read.csv("path_to_file.csv", header = TRUE, sep = ",")
head(data) # View the first few rows
- Key Parameters:
- header: Specifies whether the first row contains column names (default is TRUE).
- sep: Defines the field separator (default is a comma).
- For performance on large files, use the data.table package’s fread() function.
9) What is RStudio?
Answer:
RStudio is a popular integrated development environment (IDE) for R, enhancing the programming experience with tools for:
- Code Editing: Syntax highlighting, auto-completion, and debugging.
- Visualization: Integrated plotting windows for real-time data visualization.
- Project Management: Organizing scripts and data files efficiently.
- Add-ons: Extensions for version control (Git), package building, and markdown reporting.
It simplifies R programming and is widely used in academia and industry for statistical modeling and data science.
10) How do you install a package in R?
Answer:
Use the install.packages() function to download and install R packages from CRAN:
install.packages("ggplot2") # Installing the ggplot2 package
library(ggplot2) # Loading the package
Key Notes:
Ensure internet connectivity.
Use dependencies = TRUE to install dependent packages as well:
install.packages("dplyr", dependencies = TRUE)
To install non-CRAN packages, use devtools::install_github() for GitHub-hosted libraries.
11. Explain R’s data types.
Answer:
R supports several basic data types that are fundamental to its operations. These include:
- Numeric: Represents both integer and real numbers. Example: x <- 3.14
- Integer: Whole numbers. Explicitly defined with an L suffix, e.g., x <- 5L.
- Character: Represents text (strings). Example: x <- “Hello”.
- Logical: Boolean values, either TRUE or FALSE. Example: x <- TRUE.
- Complex: Used for complex numbers. Example: x <- 3 + 4i. Additionally, R has compound data types like:
- Vector: A one-dimensional array of elements of the same type.
- Matrix: A two-dimensional array of elements of the same type.
- Data Frame: A table where each column can have different data types, commonly used for datasets.
- List: A generic vector that can contain elements of different types (e.g., numbers, strings, or even other lists).
12. What is the difference between a vector and a list in R?
Answer:
- Vector: A vector in R is a one-dimensional array where all elements must be of the same type (e.g., numeric, character). Vectors are used for storing and manipulating data of the same kind, and they can be indexed using integer positions. Example: vec <- c(1, 2, 3).
- List: A list can hold elements of different types, including vectors, data frames, and even other lists. This flexibility makes lists more versatile than vectors. Lists are typically used for more complex data structures. Example: lst <- list(a = 1, b = “hello”, c = 3.14).
13. How to import data in R?
Answer:
Data can be imported into R using several functions depending on the file type:
- CSV: read.csv(“file.csv”) or read.table(“file.csv”, sep = “,”) for comma-separated values.
- Excel: readxl::read_excel(“file.xlsx”) for reading Excel files.
- RData/RDS: load(“file.RData”) for RData or readRDS(“file.rds”) for RDS files.
- SQL databases: Use DBI::dbConnect() and DBI::dbReadTable() to interact with databases.
Intermediate Level
15) How do you filter rows in a data frame?
Answer:
Filtering rows in a data frame in R is done using logical indexing or functions provided by packages like dplyr. The process involves specifying conditions that rows must meet to be included in the output.
Base R Approach:
Using square brackets, rows can be filtered based on logical conditions applied to columns:
# Example: Filter rows where 'column_name' is greater than 10
filtered_data <- df[df$column_name > 10, ]
- The condition df$column_name > 10 evaluates to a logical vector (TRUE/FALSE).
- Rows corresponding to TRUE are selected.
dplyr Approach:
The filter() function from dplyr is preferred for readability and chaining:
library(dplyr)
# Example: Filter rows where 'column_name' is greater than 10
filtered_data <- filter(df, column_name > 10)
- Multiple conditions can be combined with logical operators (&, |).
- The filter() function can be seamlessly integrated with the %>% (pipe) operator for chaining operations.
Filtering is essential for data wrangling tasks like subsetting data for analysis or visualizations.
16) Explain the apply() function.
Answer:
The apply() function is part of base R and allows you to apply a function over the margins (rows or columns) of matrices or data frames. It’s particularly useful for matrix-like structures where operations on rows or columns are common.
Syntax:
apply(X, MARGIN, FUN, ...)
- X: The input object (matrix, array, or data frame).
- MARGIN: 1 for rows, 2 for columns.
- FUN: The function to apply.
- …: Additional arguments to pass to FUN.
Example:
Given a matrix:
matrix_data <- matrix(1:9, nrow = 3)
# Apply sum across rows
row_sums <- apply(matrix_data, 1, sum)
# Apply mean across columns
col_means <- apply(matrix_data, 2, mean)
- apply(matrix_data, 1, sum) calculates the row-wise sum.
- apply(matrix_data, 2, mean) calculates the column-wise mean.
While versatile, apply() is slower for large datasets compared to vectorized alternatives.
17) What is the difference between lapply() and sapply()?
Answer:
Both lapply() and sapply() are used to apply a function over a list or vector, but they differ in their outputs.
lapply(): Returns the result as a list, regardless of the function’s output type.
Example:
lapply(1:5, function(x) x^2)
# Output: List of squared numbers
apply(): Tries to simplify the output. If all results are of the same type, it returns a vector or matrix; otherwise, it falls back to a list.
Example:
sapply(1:5, function(x) x^2)
# Output: Numeric vector of squared numbers
Key Difference:
- Use lapply() when you need a list.
- Use sapply() when you prefer simplified output (vector or matrix).
18) How do you handle missing values in R?
Answer:
Missing values in R are represented by NA and can impact analysis and computation. Handling them effectively involves identifying, removing, or imputing them.
Identify Missing Values:
is.na(df) # Logical matrix of TRUE/FALSE indicating NAs
sum(is.na(df)) # Count of missing values
Remove Rows with Missing Values:
clean_data <- na.omit(df)
# Removes rows with any NA
Replace Missing Values (Imputation):
Use the replace_na() function from tidyr for structured imputation:
library(tidyr)
df <- df %>% replace_na(list(column_name = 0))
Ignore NAs in Calculations:
Some functions have built-in options to handle missing values:
mean(df$column_name, na.rm = TRUE)
Proper handling depends on the dataset and context, as removing rows may lead to data loss, and imputation should align with domain knowledge.
19) Write a program to find the mean of a column in a data frame.
Answer:
Finding the mean of a column involves using the mean() function, which includes an option to handle missing values.
Code Example:
# Example Data Frame
df <- data.frame(column_name = c(1, 2, 3, NA, 5))
# Calculate mean of 'column_name' ignoring missing values
mean_value <- mean(df$column_name, na.rm = TRUE)
print(mean_value) # Output: 2.75
- na.rm = TRUE ensures that NA values are excluded from the calculation.
- Omitting this argument will result in NA if missing values are present.
20) How do you merge two data frames in R?
Answer:
Merging combines two data frames based on common columns or row indices. You can use merge() in base R or inner_join() in dplyr.
Base R Approach:
# Example Data Frames
df1 <- data.frame(ID = c(1, 2, 3), Value1 = c("A", "B", "C"))
df2 <- data.frame(ID = c(2, 3, 4), Value2 = c("X", "Y", "Z"))
# Merge by common column 'ID'
merged_data <- merge(df1, df2, by = "ID")
- merge() supports different types of joins:
- Inner Join: Default (only matching rows).
- Left Join: all.x = TRUE.
- Right Join: all.y = TRUE.
dplyr Approach:
library(dplyr)
merged_data <- inner_join(df1, df2, by = "ID")
The dplyr syntax is more readable and can easily handle other types of joins (left_join, right_join, full_join).
Efficient merging is critical when working with relational data across multiple datasets.
21) What is the difference between head() and tail()?
Answer:
head(): Displays the first n rows of a dataset (default is 6).
Example:
head(data, n = 10)
tail(): Displays the last n rows of a dataset (default is 6).
Example:
tail(data, n = 10)
Use these functions to inspect the start or end of data structures during exploratory data analysis (EDA).
22) What is the significance of ggplot2?
Answer:
ggplot2 is a data visualization package based on the Grammar of Graphics, enabling users to construct complex, layered plots.
Key Features:
- Supports aesthetics like color, shape, and size for mapping variables to plot elements.
- Facilitates multi-faceted visualizations with facet_wrap() and facet_grid().
Offers extensibility for custom themes and scales.
Example:
library(ggplot2)
ggplot(data, aes(x = var1, y = var2, color = group)) + geom_point() + theme_minimal()
23) Write a program to group and summarize data.
Answer:
Use the dplyr package for concise data manipulation:
library(dplyr)
data %>%
group_by(group_column) %>%
summarize(mean_value = mean(target_column, na.rm = TRUE),
count = n())
This groups the data by group_column and computes the mean and count for target_column.
24) How do you perform type conversion in R?
Answer:
- Type conversion ensures data compatibility for operations:
Numeric to character:
as.character(123)
Character to numeric:
as.numeric("123")
Factor to numeric:
as.numeric(as.character(factor_var))
List to data frame:
as.data.frame(list_obj)
- Always handle exceptions for non-convertible data to avoid runtime errors.
25) What are some methods to optimize R code?
Answer:
Vectorization: Replace loops with vectorized operations.
Example:
result <- vec1 + vec2
Efficient Libraries: Use data.table or dplyr for faster data manipulation.
Pre-allocate Memory: Avoid dynamic memory allocation in loops.
Example:
output <- numeric(length = n)
Parallel Computing: Leverage parallel or foreach packages for multi-core operations.
Profiling Tools: Use Rprof() or profvis to identify bottlenecks.
26) Explain what reshape2 does.
Answer:
reshape2 facilitates data reshaping between wide and long formats.
Key Functions:melt(): Converts wide-format data to long format.
Example:
melt(data, id.vars = "id")
dcast(): Converts long-format data back to wide format.
Example:
dcast(data, formula = variable1 ~ variable2, fun.aggregate = mean)
Useful in preparing data for statistical modeling or visualization.
27) What is R Markdown?
R Markdown is a file format for creating dynamic documents with embedded R code. It allows the creation of reports that integrate analysis and narrative text, including visualizations and tables. R Markdown can be compiled into various output formats such as HTML, PDF, and Word, making it a powerful tool for reproducible research and reports.
28) How to concatenate strings in R?
Strings can be concatenated in R using the paste() or paste0() functions:
- paste() adds a separator between strings (default is a space). Example: paste(“Hello”, “World”) outputs “Hello World”.
- paste0() concatenates without a separator. Example: paste0(“Hello”, “World”) outputs “HelloWorld”.
29) What types of data plots can be created in R?
R provides extensive functionality for creating various types of plots using base R functions and libraries such as ggplot2:
Base R: Bar plots (barplot()), histograms (hist()), scatter plots (plot()), boxplots (boxplot()), and line plots (lines()).
ggplot2: Highly customizable plots like scatter plots (geom_point()), line plots (geom_line()), bar plots (geom_bar()), and more complex plots like heatmaps and density plots. Example using ggplot2:
library(ggplot2)
ggplot(data = df, aes(x = var1, y = var2)) + geom_point()
30) What is the difference between the str() and summary() functions in R?
- str(): The str() function provides a compact, human-readable summary of an R object, displaying its structure, type, and the first few elements of the data. Example: str(df) gives information about the data frame’s structure.
- summary(): The summary() function provides a detailed statistical summary of the data, including measures like mean, median, min, max, and quantiles for numerical data, and frequency counts for categorical data. Example: summary(df) provides detailed summary statistics for each column of the data frame.
Master R with GUVI’s comprehensive R Programming Course designed for aspiring data scientists and those preparing for R programming interviews.
Gain hands-on expertise in data manipulation, visualization, and statistical modeling using top tools like ggplot2, dplyr, and RStudio. Learn from industry experts, work on real-world projects, and confidently crack interviews with dedicated guidance and resources!
Advanced Level
31) Explain the concept of lazy evaluation in R.
Answer:
Lazy evaluation in R refers to the delayed evaluation of function arguments until they are actually used in the body of the function. This behavior optimizes performance by avoiding unnecessary computations and allows flexible handling of invalid inputs if they are not utilized.
For example:
lazy_function <- function(x, y) {
print(x)
return(x^2)
}
lazy_function(4, stop("This won't be evaluated!")) # y is never evaluated
32) What are closures in R?
Answer:
A closure in R is a function paired with its defining environment. Closures allow the function to access variables from its creation environment, even when called outside of it. They are useful for encapsulating state and creating specialized functions dynamically.
For example:
closure_function <- function(a) {
return(function(b) a + b) # Retains 'a' from the environment
}
add_five <- closure_function(5)
add_five(3) # Output: 8
33) Explain the caret package.
Answer:
The caret (Classification And Regression Training) package in R streamlines the workflow of machine learning by providing functions for pre-processing, feature selection, model training, tuning, and evaluation. It supports cross-validation and integrates over 200 modeling algorithms. Key functions include train() for model training and createDataPartition() for splitting data.
Example of using caret:
library(caret)
data(iris)
train_control <- trainControl(method = "cv", number = 10)
model <- train(Species ~ ., data = iris, method = "rf", trControl = train_control)
print(model)
34) How do you perform logistic regression in R?
Answer:
Logistic regression is performed using the glm() function in R with the family argument set to binomial. This method models binary or multinomial response variables.
Example:
# Logistic regression with binary response
data(iris)
iris_binary <- subset(iris, Species != "setosa")
iris_binary$Species <- factor(iris_binary$Species)
logit_model <- glm(Species ~ Sepal.Length + Sepal.Width, data = iris_binary, family = binomial)
summary(logit_model)
35) Write a program to perform K-means clustering.
Answer:
K-means clustering partitions data into k clusters by minimizing within-cluster variance. Use the kmeans() function to perform clustering.
Example:
# Generate sample data
set.seed(123)
data <- matrix(rnorm(100), ncol = 2)
# Apply K-means clustering
kmeans_result <- kmeans(data, centers = 3, nstart = 25)
print(kmeans_result$centers) # Cluster centroids
# Visualize clusters
plot(data, col = kmeans_result$cluster, pch = 19, main = "K-means Clustering")
points(kmeans_result$centers, col = 1:3, pch = 8, cex = 2)
36) How do you detect multicollinearity in regression?
Answer:
Multicollinearity refers to the high correlation between independent variables in a regression model, which can distort coefficient estimates. Detection techniques include:
Variance Inflation Factor (VIF):
Calculate VIF using the car package:
library(car)
vif(model)
A VIF value greater than 5 or 10 indicates significant multicollinearity.
Correlation Matrix:
Analyze pairwise correlations:
cor(data)
High absolute values (>0.8) signify multicollinearity.
37) What is time-series analysis in R?
Answer:
Time-series analysis involves studying data points collected over time to identify trends, seasonal patterns, and cycles. R supports time-series through the ts object and packages like forecast and tseries.
Example: Create and plot a time series:
ts_data <- ts(data, start=c(2020, 1), frequency=12)
plot(ts_data)
Key models include ARIMA (auto.arima()), Exponential Smoothing (ets()), and decomposition methods.
38) How do you implement Random Forest in R?
Answer:
Random Forest is an ensemble learning method that builds multiple decision trees for classification or regression. Implement it using the randomForest package:
library(randomForest)
model <- randomForest(target ~ ., data=training_data, ntree=500, mtry=3)
predictions <- predict(model, newdata=test_data)
Key parameters:
- ntree: Number of trees.
- mtry: Number of features to consider at each split.
Evaluate performance using metrics like accuracy or RMSE.
39) What are survival models in R?
Answer:
Survival models analyze time-to-event data, accounting for censoring. Common methods include Kaplan-Meier estimation and Cox proportional hazards models, implemented using the survival package:
Kaplan-Meier Curve:
library(survival)
fit <- survfit(Surv(time, status) ~ group, data=survival_data)
plot(fit)
Cox Model:
cox_model <- coxph(Surv(time, status) ~ covariates, data=survival_data)
summary(cox_model)
40) Explain the caret::train() function.
Answer:
The train() function in the caret package standardizes the process of building machine learning models:
library(caret)
model <- train(target ~ ., data=training_data, method="rf", tuneGrid=data.frame(mtry=c(2, 3, 4)))
Key features:
- method: Specifies the algorithm (e.g., “rf”, “lm”).
- tuneGrid: Enables hyperparameter tuning.
- Cross-Validation: Use trainControl() to configure validation strategies.
41) What are eigenvalues and eigenvectors in R?
Answer:
Eigenvalues and eigenvectors decompose a square matrix to analyze its properties, used in PCA and linear transformations. Compute them using:
eigen_result <- eigen(matrix)
eigenvalues <- eigen_result$values
eigenvectors <- eigen_result$vectors
Eigenvalues represent the variance explained by each dimension, while eigenvectors define the directions.
42) Write a program for Principal Component Analysis (PCA).
Answer:
PCA reduces dimensionality by transforming correlated features into uncorrelated components. Example:
data <- scale(dataset) # Standardize data
pca_result <- prcomp(data, center=TRUE, scale.=TRUE)
summary(pca_result) # View explained variance
biplot(pca_result) # Visualize components
Key output:
- Principal Components: Linear combinations of original variables.
- Proportion of Variance: Importance of each component.
43) Explain the Kaplan-Meier estimator.
Answer:
The Kaplan-Meier estimator is a non-parametric statistic used to estimate the survival function from lifetime data. It is particularly useful in survival analysis to estimate the probability of survival over time, considering censored data (cases where the event has not occurred by the end of the study).
In R, the Kaplan-Meier estimator can be implemented using the survival package, with the survfit() function. It generates a survival curve and handles censored data naturally.
Example:
library(survival)
fit <- survfit(Surv(time, status) ~ 1, data = dataset)
plot(fit)
Here, time represents survival time, and status indicates whether the event (e.g., death) occurred.
44) How do you use R for natural language processing (NLP)?
Answer:
R provides several packages to handle NLP tasks, such as text processing, tokenization, and sentiment analysis. Key libraries include tm for text mining and tidytext for text manipulation in a tidy format. To perform NLP, the typical workflow involves:
Text cleaning (removing stop words, punctuation, etc.) using tm:
library(tm)
corpus <- Corpus(VectorSource(text_data))
corpus <- tm_map(corpus, content_transformer(tolower))
Tokenization and sentiment analysis with tidytext:
library(tidytext)
data <- data_frame(text = c("R is great for NLP"))
data %>%
unnest_tokens(word, text) %>%
inner_join(get_sentiments("bing"))
This approach allows processing and analyzing large volumes of unstructured text efficiently.
45) Explain the purpose of cross-validation.
Answer:
Cross-validation is a technique used to assess the generalizability of a model. It splits the dataset into multiple subsets or “folds” and uses each fold as a test set while training the model on the remaining folds. The most common method is k-fold cross-validation, where the data is split into k equal parts.
It helps in reducing overfitting, ensuring that the model performs well on unseen data, and provides a more reliable estimate of model performance. In R, the caret package provides built-in cross-validation functionality:
library(caret)
train_control <- trainControl(method="cv", number=10)
model <- train(target ~ ., data = dataset, method="lm", trControl = train_control)
46) How do you automate reports in R?
Answer:
In R, automated reporting can be achieved using the rmarkdown package, which integrates R code with markdown for dynamic report generation. The process includes writing R code chunks within markdown documents that are rendered into HTML, PDF, or Word documents. This allows the automatic embedding of analysis results, plots, and tables directly from R code. Example:
library(rmarkdown)
render("report.Rmd", output_format = "html_document")
Additionally, knitr is often used to knit R code into reports. You can schedule report generation via scripts using cronR or RStudio Connect for automation.
47) What is the purpose of forecast::auto.arima()?
Answer:
The function auto.arima() in the forecast package is used to automatically select the best ARIMA (AutoRegressive Integrated Moving Average) model for time series forecasting. It performs model selection by evaluating combinations of different p, d, and q parameters (AR, differencing, and MA components), and uses AIC or BIC as the criteria for the best fit.
The function also handles seasonality by including seasonal ARIMA models if required. Example:
library(forecast)
fit <- auto.arima(time_series_data)
forecast(fit, h=10)
48) How do you build a Shiny app in R?
Answer:
A Shiny app in R allows the creation of interactive web applications with R code for both the backend (server) and frontend (user interface). To build a basic Shiny app, you need two components:
- UI: Defines the layout and elements (e.g., sliders, input fields, output plots).
- Server: Contains the logic and computation for rendering outputs based on inputs.
Example of a simple Shiny app:
library(shiny)
ui <- fluidPage(
sliderInput("num", "Choose a number:", min = 1, max = 100, value = 50),
textOutput("result")
)
server <- function(input, output) {
output$result <- renderText({
paste("You chose:", input$num)
})
}
shinyApp(ui = ui, server = server)
Shiny apps can also be deployed using shinyapps.io or RStudio Connect.
Concluding Thoughts…
R programming interviews are not just a test of your coding skills but also a deep dive into your analytical thinking and problem-solving abilities. By exploring this list of questions and answers, you’ve gained a robust toolkit to handle queries on core concepts, programming logic, and advanced statistical techniques.
I hope these questions have helped you start your preparation journey and if you have doubts about any of these questions or the article itself, reach out to me in the comments section below.
Did you enjoy this article?