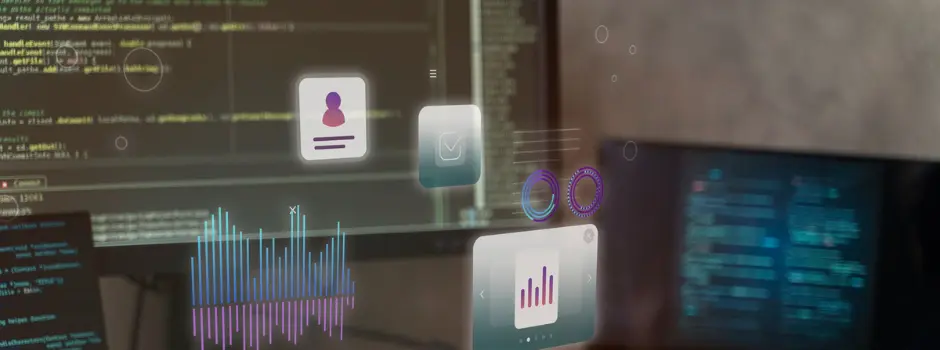
Boost Your Selenium Web Automation with Java 8+ Features
Sep 18, 2024 2 Min Read 64 Views
(Last Updated)
Selenium Web Automation testing is rapidly evolving, and with the introduction of Java 8, testers can leverage its new features to write cleaner, more efficient code in Selenium.
This blog will explore how Java 8+ features can enhance your Selenium web automation and simplify common tasks.
Table of contents
- Selenium Web Automation with Java 8+ Features
- Lambda Expressions: Simplifying Code
- Streams API: Efficient Data Handling
- Optional Class: Handling Null Values Gracefully
- Method References: Cleaner and Shorter Code
- Date and Time API: Improved Date Handling
- Default and Static Methods in Interfaces: Better Reusability
- Conclusion
Selenium Web Automation with Java 8+ Features
1. Lambda Expressions: Simplifying Code
One of the most powerful features of Java 8 is lambda expressions, which allow you to write concise and readable code. In Selenium, lambda expressions can simplify the way you interact with web elements and perform actions.
For example, iterating through a list of web elements and performing an action becomes much cleaner with lambdas:
List<WebElement> links = driver.findElements(By.tagName("a"));
links.forEach(link -> System.out.println(link.getText()));
This makes your code more readable and eliminates the need for traditional for loops.
2. Streams API: Efficient Data Handling
Java 8’s Streams API is another feature that shines in Selenium web automation testing. It allows you to process large collections of data with ease, whether you need to filter, map, or reduce data.
In Selenium, the Streams API can be used to filter web elements based on certain conditions, such as clicking on a specific button:
List<WebElement> buttons = driver.findElements(By.tagName("button"));
buttons.stream()
.filter(button -> button.getText().equals("Submit"))
.forEach(WebElement::click);
This approach reduces code verbosity and improves test efficiency, especially when dealing with large sets of elements.
3. Optional Class: Handling Null Values Gracefully
Dealing with NullPointerException is a common issue in automation testing. Java 8 introduced the Optional class, which helps avoid such exceptions by safely handling null values.
For instance, when searching for an element that might not exist on the page, you can use Optional:
Optional<WebElement> button = Optional.ofNullable(driver.findElement(By.id("submitButton")));
button.ifPresent(WebElement::click);
This ensures that your tests won’t fail due to a missing element, as the ifPresent method ensures that the click() action is only executed if the element is present.
4. Method References: Cleaner and Shorter Code
Method references allow you to further shorten lambda expressions when calling existing methods. Instead of passing a lambda that calls a method, you can directly refer to the method itself:
List<WebElement> links = driver.findElements(By.tagName("a"));
links.forEach(System.out::println);
This is particularly useful when working with reusable functions or libraries in your test framework.
5. Date and Time API: Improved Date Handling
Before Java 8, working with dates and times was cumbersome. With the new Date and Time API, you can now handle dates and times in a more structured way, which is crucial for tasks like timestamping test results or verifying date-related data on web pages.
Example usage:
LocalDateTime now = LocalDateTime.now();
System.out.println("Test executed at: " + now);
This makes date handling in tests more reliable and intuitive compared to the old Date and Calendar classes.
6. Default and Static Methods in Interfaces: Better Reusability
Java 8 introduced default and static methods in interfaces, which can be particularly useful for creating reusable utility methods for Selenium testing. These methods allow you to define default behaviors directly in interfaces without the need for separate utility classes.
For example, in an interface defining common actions, you could have:
public interface TestActions {
default void waitForElement(WebElement element) {
// wait logic
}
static void captureScreenshot(WebDriver driver) {
// screenshot logic
}
}
This promotes code reusability and simplifies test maintenance.
In case you want to learn more about Selenium web automation, consider enrolling for GUVI’s Automation Testing with Selenium which teaches you everything from scratch by providing an industry-grade certificate!
Conclusion
In conclusion, Java 8 has brought significant improvements to programming in general, but its impact on Selenium test automation is profound.
From lambda expressions and Streams to Optional handling and the new Date API, these features can simplify your test scripts and enhance your productivity as a test automation engineer.
By incorporating these features into your Selenium test suites, you can write cleaner, more maintainable, and more efficient tests.
Did you enjoy this article?