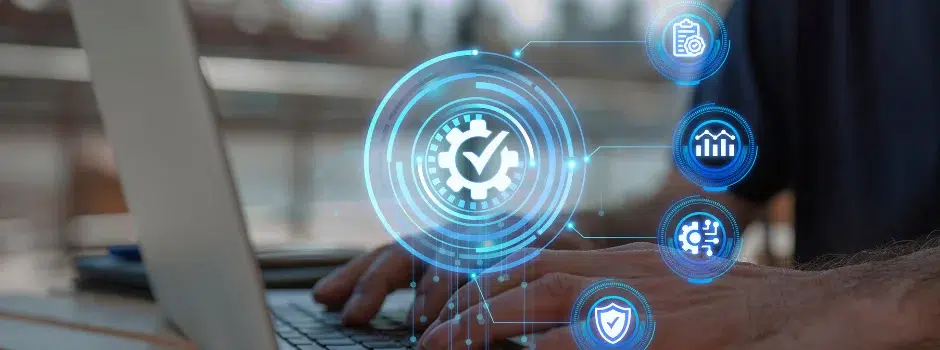
Simplifying Jenkins for Python Automation Testing
Feb 12, 2025 4 Min Read 715 Views
(Last Updated)
Table of contents
- The Role of Jenkins in Modern Python CI/CD
- Why Use Jenkins for Python CI/CD?
- Setting Up Jenkins for Python Automation Testing
- Configuring a Python Project in Jenkins
- Setting Up a Jenkins Pipeline for Python Automation Testing with pytest-html
- Viewing HTML Reports in Jenkins
- Key Takeaways for Python Testing with Jenkins
The Role of Jenkins in Modern Python CI/CD
In modern software development, automation isn’t a luxury—it’s a necessity. Continuous Integration and Continuous Deployment (CI/CD) have become the backbone of efficient and reliable software delivery. Jenkins, with its open-source flexibility and extensive plugin ecosystem, stands out as a powerful automation server for streamlining CI/CD processes. When combined with Python’s robust testing frameworks like pytest and enhanced with reporting tools like pytest-html, Jenkins becomes a comprehensive solution for building, testing, and deploying Python applications.
This guide explores how you can harness Jenkins to automate Python testing, ensure quality with every code change, and present test results in a readable format—all while simplifying the entire workflow.
1. Why Use Jenkins for Python CI/CD?
Jenkins provides several advantages for continuous integration and testing, including:
- Automated Testing: Execute tests automatically on every code change, identifying issues early.
- Plugin Support: Jenkins supports integration with popular tools like Git, Docker, and Slack.
- Customizable Pipelines: Jenkins pipelines allow you to automate tasks and create flexible workflows.
- Readable Reporting: With pytest-html, you can generate clear, detailed HTML reports that make test results easy to understand.
2. Setting Up Jenkins for Python Automation Testing
Step 1: Installing Jenkins
- Download and install Jenkins from the official Jenkins site.
- After installation, start Jenkins with:
java -jar jenkins.war - Access Jenkins at http://localhost:8080 in your browser and complete the setup.
Step 2: Installing Required Plugins
To fully integrate Jenkins with Git and notifications, install these plugins:
- Git Plugin: For connecting Jenkins to your Git repository.
- Notification Plugin: To receive notifications on build status.
- HTML Publisher Plugin: For displaying HTML reports generated by pytest-html.
Go to Manage Jenkins > Manage Plugins and install these plugins.
3. Configuring a Python Project in Jenkins
Step 1: Creating a New Job
- In the Jenkins dashboard, select New Item.
- Name your job, choose Freestyle project or Pipeline (recommended for CI/CD pipelines), and click OK.
Step 2: Setting Up Source Code Management
- In the Source Code Management section, choose Git.
- Enter the URL for your Git repository and add credentials if required.
Step 3: Setting Up the Build Environment
To run tests with pytest in Jenkins, make sure Python and any necessary dependencies are available on your Jenkins server.
4. Setting Up a Jenkins Pipeline for Python Automation Testing with pytest-html
Using a Jenkins Pipeline job type allows for flexible, customizable build scripts. Below is a Jenkinsfile for a Python automation project that uses pytest-html to generate reports.
Example Jenkinsfile:
pipeline {
agent any
stages {
stage(‘Clone Repository’) {
steps {
git ‘https://github.com/your-repository.git’
}
}
stage(‘Install Dependencies’) {
steps {
sh ”’
# Set up a virtual environment
python -m venv venv
source venv/bin/activate
# Install dependencies
pip install -r requirements.txt
”’
}
}
stage(‘Run Tests’) {
steps {
sh ”’
# Activate virtual environment
source venv/bin/activate
# Run tests with pytest-html to generate HTML report
pytest –html=report.html
”’
}
}
}
post {
always {
// Archive HTML report and display in Jenkins
publishHTML(target: [
reportName: ‘Test Report’,
reportDir: ”,
reportFiles: ‘report.html’,
alwaysLinkToLastBuild: true,
keepAll: true
])
}
success {
echo ‘Tests passed successfully.’
}
failure {
echo ‘One or more tests failed.’
}
}
}
Pipeline Breakdown
- Clone Repository: Pulls the latest code from the Git repository.
- Install Dependencies: Creates a virtual environment and installs dependencies listed in requirements.txt.
- Run Tests: Executes tests with pytest, generating an HTML report using pytest-html.
- Post Actions:
- publishHTML: Archives and displays the HTML test report, making it accessible directly in Jenkins.
- success and failure: Prints messages based on the test outcome, giving quick feedback on whether the build succeeded.
5. Viewing HTML Reports in Jenkins
With the pytest-html generated report archived in Jenkins, you can review test results directly from the Jenkins dashboard.
- Go to your Jenkins job and click on Last Successful Artifacts.
- Select Test Report to view the pytest-html report, which displays detailed information on test results, errors, and durations.
🚀 Want to take your automation testing skills to the next level? While Jenkins streamlines the CI/CD process, mastering Selenium can help you automate web testing like a pro. If you’re looking for a structured learning path, check out the Selenium Automation Testing Course designed to make you industry-ready with hands-on experience and expert guidance.
Key Takeaways for Python Testing with Jenkins
Integrating Jenkins into your Python automation testing strategy not only automates repetitive tasks but also ensures consistency and quality across every stage of your CI/CD pipeline. By leveraging the capabilities of pytest-html, you gain clear visibility into your test results, enabling teams to debug faster and maintain higher coding standards.
This approach doesn’t just improve workflows—it cultivates a development culture that prioritizes quality and efficiency. With Jenkins pipelines, Python developers can focus on building innovative solutions while leaving the intricacies of testing and deployment to a reliable automation process.
Did you enjoy this article?