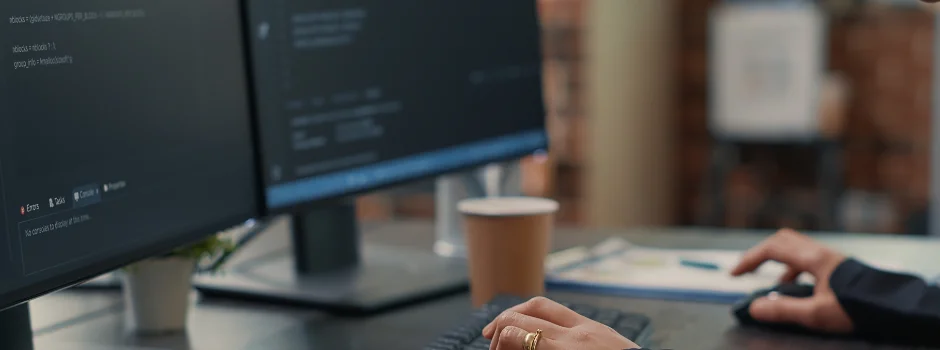
TestNG Annotations and Their Uses in Selenium Automation
Jan 30, 2025 4 Min Read 860 Views
(Last Updated)
What’s the secret behind making automation testing with Selenium not just effective but also seamless? It’s all about structure and strategy—and that’s where TestNG annotations come into play. Imagine running a marathon without clear checkpoints or a well-marked path. That’s how testing would feel without the structured guidance TestNG provides.
In this blog, we’ll take a closer look at TestNG annotations, unraveling their types and practical applications in Selenium. These annotations help simplify setting up test environments, managing workflows, and organizing test flows efficiently. Let’s explore how mastering these tools can transform your automation journey into a streamlined process.
Table of contents
- What Are TestNG Annotations?
- Commonly Used TestNG Annotations
- @BeforeSuite
- @BeforeTest
- @BeforeClass
- @Test
- @AfterClass
- @AfterTest
- @AfterSuite
- @DataProvider
- @BeforeMethod and @AfterMethod
- Key Uses of TestNG Annotations in Selenium Automation
- Conclusion
What Are TestNG Annotations?
TestNG annotations are special markers in Java that provide instructions to the TestNG framework about how to execute the test methods. These annotations define the life cycle of the test execution, such as before a test, after a test, or when the test should be skipped.
TestNG annotations are defined by the @TestNG library and help organize the execution sequence of test methods, facilitating modular and scalable automation testing.
Commonly Used TestNG Annotations
Below are the most commonly used TestNG annotations, their purposes, and how they can be leveraged in Selenium test scripts:
1. @BeforeSuite
Purpose: The @BeforeSuite annotation is executed once before any of the tests in the test suite are run. This is typically used to set up the environment for the entire suite, such as initializing WebDriver or reading configuration files.
Use Case:
- Initializing Selenium WebDriver before running all tests in a suite.
- Setting up test environment (e.g., creating a database connection).
Example:
@BeforeSuite
public void setupSuite() {
// Code to initialize WebDriver or setup suite-level configurations
System.out.println(“Before Suite: Setting up environment…”);
}
2. @BeforeTest
Purpose: This annotation is executed before any test methods inside a <test> tag are executed. It is useful for setting up preconditions for specific tests, like configuring a test database or logging into the application.
Use Case:
- Logging in to the application before a set of tests runs.
- Configuring test-specific settings.
Example:
@BeforeTest
public void setupTest() {
// Code to setup test-specific configurations
System.out.println(“Before Test: Setting up test environment…”);
}
3. @BeforeClass
Purpose: The @BeforeClass annotation is executed once before the first method in the current class is run. It is used for setting up resources needed specifically for the class.
Use Case:
- Setting up a browser window or WebDriver before running all tests within the class.
- Initializing a set of data or configurations that are required for all tests in the class.
Example:
@BeforeClass
public void beforeClass() {
// Code to initialize browser or setup configurations
System.out.println(“Before Class: Setting up browser…”);
}
4. @Test
Purpose: The @Test annotation marks a method as a test case. This is the most commonly used annotation in TestNG. You can have multiple test methods in a class, each marked with the @Test annotation.
Use Case:
- Writing actual test cases to validate functionalities like form submissions, login, navigation, etc.
- It can be used with parameters, assertions, and expected exceptions.
Example:
@Test
public void testLoginFunctionality() {
// Selenium WebDriver code for testing login functionality
Assert.assertTrue(driver.findElement(By.id(“loginButton”)).isDisplayed());
System.out.println(“Login test passed”);
}
5. @AfterClass
Purpose: The @AfterClass annotation is executed after all the test methods in the current class have been run. It is used for cleanup tasks, such as closing the WebDriver or releasing any resources allocated for the class.
Use Case:
- Closing the browser window after all tests in a class are complete.
- Clearing test data or disconnecting resources.
Example:
@AfterClass
public void afterClass() {
// Code to close browser
driver.quit();
System.out.println(“After Class: Closing browser…”);
}
6. @AfterTest
Purpose: This annotation is executed after all test methods in the <test> tag have been executed. It is useful for test-specific cleanup, such as logging out or resetting configurations that were set up before the test.
Use Case:
- Logging out from an application after the tests are complete.
- Cleaning up or resetting configurations for the next test.
Example:
@AfterTest
public void afterTest() {
// Code to logout or clean up after the test
System.out.println(“After Test: Cleaning up…”);
}
7. @AfterSuite
Purpose: The @AfterSuite annotation is executed after all tests in the suite have been run. It is useful for performing cleanup tasks at the suite level, such as reporting or releasing suite-wide resources.
Use Case:
- Generating a test report after all tests in the suite have completed.
- Closing connections or releasing suite-level resources.
Example:
@AfterSuite
public void teardownSuite() {
// Code to generate reports or cleanup after the entire suite
System.out.println(“After Suite: Cleaning up after suite…”);
}
8. @DataProvider
Purpose: The @DataProvider annotation is used to provide data to a test method. It allows a test method to run multiple times with different inputs, which is useful for data-driven testing.
Use Case:
- Running the same test with different sets of data, such as validating form submissions with various input values.
Example:
@DataProvider(name = “loginData”)
public Object[][] loginDataProvider() {
return new Object[][] {
{ “user1”, “password1” },
{ “user2”, “password2” }
};
}
@Test(dataProvider = “loginData”)
public void testLogin(String username, String password) {
// Selenium WebDriver code to perform login with provided data
Assert.assertTrue(login(username, password));
}
9. @BeforeMethod and @AfterMethod
These annotations are executed before and after each individual test method in a class.
- @BeforeMethod: Used to set up any preconditions before each test method.
- @AfterMethod: Used for cleanup tasks after each test method.
Use Case:
- Setting up and tearing down individual tests, such as clearing cookies or logging in before each test.
Example:
@BeforeMethod
public void beforeMethod() {
// Code to initialize WebDriver or log in
System.out.println(“Before Method: Logging in before each test…”);
}
@AfterMethod
public void afterMethod() {
// Code to logout or clear browser cache
System.out.println(“After Method: Logging out after each test…”);
}
Key Uses of TestNG Annotations in Selenium Automation
- Test Organization and Setup: Annotations like @BeforeSuite, @BeforeClass, and @BeforeTest help organize the setup for a test suite, class, or individual test cases, allowing for better maintainability and structure.
- Efficient Test Execution: Annotations like @Test allow you to define multiple test methods, which can be run in parallel or sequentially. The use of @DataProvider further enhances test execution by running tests with different data sets.
- Resource Management: Annotations like @AfterSuite, @AfterTest, and @AfterClass make it easy to perform cleanup tasks such as closing WebDriver sessions or releasing any external resources after tests have run.
- Flexible and Scalable: TestNG allows you to group tests, run them in parallel, and apply different annotations depending on your needs. This makes it highly flexible and scalable for large test suites.
- Error Handling and Reporting: TestNG provides mechanisms for handling test failures, generating reports, and capturing logs. The annotations allow testers to structure their tests in a way that enhances visibility and debugging.
TestNG annotations streamline automation by managing test execution, parallel runs, and exception handling. But theory alone isn’t enough, real-world application is key.
Looking to master Selenium automation? Get hands-on with this Selenium Automation Testing Course and learn to implement TestNG effectively in scalable frameworks.
Conclusion
Mastering TestNG annotations isn’t just about ticking technical boxes—it’s about creating a well-oiled testing framework that can adapt to real-world complexities. By strategically using annotations like @BeforeSuite or @DataProvider, you’re not just managing tests but optimizing workflows for better results.
Think of TestNG as the backbone of your Selenium automation—helping you execute tests with precision while ensuring your framework remains robust and scalable. The more fluently you incorporate these annotations into your testing strategy, the more seamless and effective your automation efforts will become. Here’s to building better testing processes, one annotation at a time!
Did you enjoy this article?