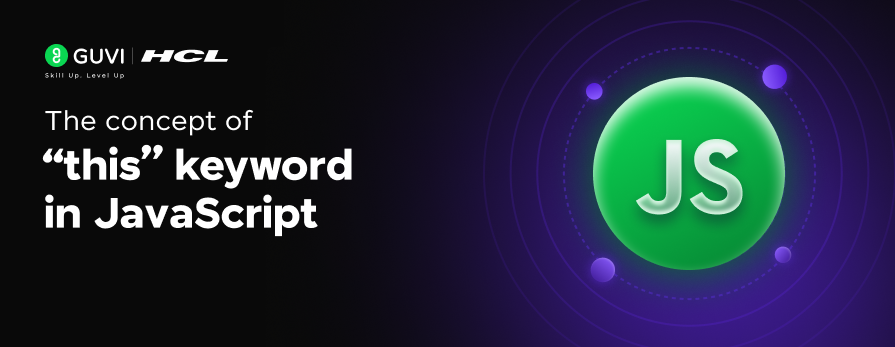
The concept of “this” keyword in Javascript
Oct 09, 2024 2 Min Read 880 Views
(Last Updated)
If you are starting out as a full-stack developer, you definitely come across one of the most popular languages which is JavaScript. There are various keywords like “this” keyword in JavaScript that play a vital role in backend development.
In those JavaScript keywords, the this keyword is something that every developer should know! If you don’t know what it is or how to use it, this article is right there to help you! So, let us get started!
Table of contents
- What is "this" Keyword? How to Use it?
- What is "this"?
- Global Context
- Function Context
- Explicit Binding
- Conclusion
What is “this” Keyword? How to Use it?
JavaScript, one of the most popular programming languages, is widely used for building dynamic web applications.
One of its unique and sometimes confusing features is the “this” keyword. Understanding how this works is essential for mastering JavaScript, as it behaves differently than in many other programming languages.
What is “this”?
In JavaScript, “this” refers to the context in which a function is executed. It is a reference to an object, and its value depends on where and how the function is called.
Global Context
When this is used outside of any function, in the global context, it refers to the global object. In browsers, the global object is “window”.
console.log(this); // window
Function Context
When “this” is used inside a regular function in JavaScript, its value depends on how the function is called.
- Simple Function Call
In a simple function call, this refers to the global object. Let us see about this with an example code:
function showThis() {
console.log(this);
}
showThis(); // window
- Method Call
When a function is called as a method of an object in JavaScript, this refers to the object that owns the method.
const obj = {
name: 'John',
greet: function() {
console.log(this.name);
}
};
obj.greet(); // John
- Constructor Call
When a function is used as a constructor with the new keyword, “this” refers to the newly created instance. Here is an example code to showcase this:
function Person(name) {
this.name = name;
}
const person = new Person('Guvi');
console.log(person.name); // Guvi
- Arrow Functions
Arrow functions behave differently. They do not have their own “this” context; instead, they inherit this from the surrounding non-arrow function. The below example helps you understand more about the arrow functions
const obj = {
name: 'Guvi',
greet: function() {
const arrowFunction = () => console.log(this.name);
arrowFunction();
}
};
obj.greet(); // Guvi
Explicit Binding
JavaScript provides methods to explicitly set the value of this: call(), apply(), and bind().
- call() and apply()
Both call() and apply() methods are used to call a function with a specified “this” value. The difference between them is that call() takes arguments separately, while apply() takes them as an array.
function introduce(language) {
console.log(`My name is ${this.name} and I speak ${language}.`);
}
const person = { name: 'Carlos' };
introduce.call(person, 'Spanish'); // My name is Carlos and I speak Spanish.
introduce.apply(person, ['Spanish']); // My name is Carlos and I speak Spanish.
- bind()
The bind() method creates a new function that, when called, has its this keyword set to the provided value. This is useful for creating functions with a specific “this” value.
const person = { name: 'Diana' };
function greet() {
console.log(this.name);
}
const boundGreet = greet.bind(person);
boundGreet(); // Diana
We hope you understand the ways in which “this” keyword is used in JavaScript frameworks. In case, you want to learn more about “this” and gain in-depth knowledge on full-stack development, consider enrolling for GUVI’s certified Full-stack Development Course that teaches you everything from scratch and make sure you master it!
Conclusion
In conclusion, understanding the “this” keyword in JavaScript is crucial for writing effective and bug-free code. By knowing how this behaves in different contexts, you can avoid common pitfalls and harness its power to create more dynamic and context-aware functions.
Whether you’re working with global context, object methods, constructors, or arrow functions, mastering this will significantly enhance your JavaScript skills.
Did you enjoy this article?