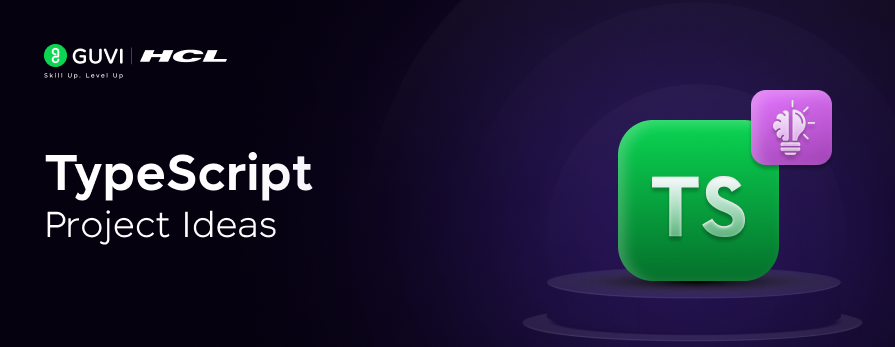
Top 15+ TypeScript Projects with Source Code
Nov 28, 2024 6 Min Read 73 Views
(Last Updated)
Have you ever wondered how to start building projects with TypeScript and take your programming skills to the next level? Learning by doing is one of the best ways to master TypeScript.
Hence, I present 18 TypeScript projects for beginners, intermediates, and experts in this article, covering diverse use cases with detailed explanations, source code links, and essential technical highlights. From simple projects like calculators and weather apps to advanced ones like blockchain explorers, these ideas will help you explore the versatility of TypeScript.
If you’re looking for TypeScript project ideas for beginners to build your portfolio or enhance your understanding of TypeScript, this curated list offers a variety of exciting options to choose from.
Table of contents
- The 18 Best TypeScript Project Ideas from Beginner to Expert [With Source Code]
- Pomodoro Timer
- Weather App
- Portfolio Website
- Text-Based Adventure Game
- Todo App
- Quiz App
- Currency Converter
- Photo Gallery
- Movie Search App
- Markdown Editor
- Real-Time Chat Application
- E-commerce Store
- Storybook
- Calculator
- Random Quote Generator
- Memory Game
- Expense Tracker
- Blockchain Explorer
- Final Words
- FAQs
- What are the easy TypeScript project ideas for beginners?
- Why are TypeScript projects important for beginners?
- What skills can beginners learn from TypeScript projects?
- Which TypeScript project is recommended for someone with no prior programming experience?
- How long does it typically take to complete a beginner-level TypeScript project?
The 18 Best TypeScript Project Ideas from Beginner to Expert [With Source Code]
These 18 TypeScript projects have been carefully selected to cover a wide range of complexity, from beginner-friendly tasks to advanced real-world applications.
Each project demonstrates TypeScript’s versatility, focusing on practical learning outcomes like type safety, scalable architecture, and seamless integration with modern frameworks and libraries.
1. Pomodoro Timer
The Pomodoro Timer project is a productivity tool built using TypeScript and React. It implements the Pomodoro Technique by managing work and break intervals, providing real-time countdowns, and supporting customizable session durations.
This project introduces event-driven programming, state management, and responsive UI design, making it an ideal entry-level project for mastering TypeScript in time-based applications.
Project Type: Web Application
Project Complexity: Beginner
Project Duration: 2–3 hours
Dependencies: React, TypeScript, CSS
Functionality Scope: A timer that alternates between work and rest periods based on the Pomodoro technique.
Tasks in Project:
- Implement a countdown timer with start, pause, and reset functionality.
- Add configurable work/rest intervals.
- Display a progress bar and notifications.
Technical Highlights:
- Timer logic implemented with TypeScript for type safety.
- Use of React hooks and state management.
Learning Outcomes:
- Managing states and props in React with TypeScript.
- Handling user interactions and time-based events.
Skills Acquired:
- TypeScript basics, DOM manipulation, and React integration.
Source Code: Pomodoro Timer Code
2. Weather App
The Weather App uses TypeScript with APIs like OpenWeatherMap to fetch real-time weather data. It features dynamic UI updates, geolocation services, and responsive design for seamless performance across devices.
This project focuses on integrating TypeScript for API handling, data validation, and real-time DOM manipulation in a modern single-page application.
Project Type: Web Application
Project Complexity: Beginner to Intermediate
Project Duration: 4–6 hours
Dependencies: React, Axios, OpenWeather API
Functionality Scope: Displays real-time weather information for a searched location.
Tasks in Project:
- Fetch weather data using APIs.
- Build a responsive UI for search and display results.
Technical Highlights:
- Integrating REST APIs with TypeScript.
- Handling API errors gracefully.
Learning Outcomes:
- Mastering TypeScript interfaces for API data structures.
- Building API-driven apps.
Skills Acquired:
- TypeScript for API integration, async/await, and modular coding.
Source Code: Weather App Code
3. Portfolio Website
The Portfolio Website project combines TypeScript and React to create a visually engaging and responsive platform showcasing your personal projects and achievements. With modular component architecture, animation effects, and TypeScript for type safety, this project serves as a foundation for building scalable and customizable web applications.
Project Type: Static Web Application
Project Complexity: Beginner
Project Duration: 6–8 hours
Dependencies: React, Tailwind CSS
Functionality Scope: Showcase your projects, skills, and contact information.
Tasks in Project:
- Create reusable components for portfolio sections.
- Add animations and responsive designs.
Technical Highlights:
- Component-based design using TypeScript.
- Styling with Tailwind CSS and responsiveness.
Learning Outcomes:
- Building reusable components with TypeScript.
- Understanding TypeScript project structure for a real-world app.
Skills Acquired:
- Frontend development with TypeScript, CSS libraries, and design principles.
Source Code: Portfolio Website Code
4. Text-Based Adventure Game
This interactive Text-Based Adventure Game leverages TypeScript for game logic and state management. Players navigate through branching storylines, making choices that impact the game’s outcome. The project emphasizes TypeScript’s use in creating structured, reusable code for complex decision trees and event handling.
Project Type: Console Application
Project Complexity: Intermediate
Project Duration: 2–3 days
Dependencies: None (vanilla TypeScript)
Functionality Scope: A command-line game with interactive text-based choices.
Tasks in Project:
- Design a branching storyline.
- Handle user input and validate decisions.
Technical Highlights:
- Use of enums, interfaces, and classes for game logic.
- Implementing recursive functions for branching paths.
Learning Outcomes:
- Building logic-heavy applications.
- Working with TypeScript’s OOP features.
Skills Acquired:
- Problem-solving, recursion, and TypeScript OOP.
Source Code: Adventure Game Code
5. Todo App
The Todo App is a classic beginner project that demonstrates TypeScript’s capabilities in managing dynamic lists and stateful applications. With features like task creation, editing, and filtering, this project highlights core concepts such as type-safe state management, reusable components, and event-driven programming.
Project Type: Web Application
Project Complexity: Beginner
Project Duration: 4–6 hours
Dependencies: React, TypeScript
Functionality Scope: A task management app to add, edit, and delete tasks.
Tasks in Project:
- Implement CRUD operations for tasks.
- Persist tasks in local storage.
Technical Highlights:
- Use of TypeScript for state management.
- React hooks for CRUD functionality.
Learning Outcomes:
- Building simple and interactive applications.
- Using TypeScript interfaces for task objects.
Skills Acquired:
- React state management, DOM manipulation, and type safety.
Source Code: Todo App Code
6. Quiz App
The Quiz App is a dynamic application where TypeScript is used to create interactive quizzes with score tracking and timer-based challenges. This project emphasizes type-safe state management, real-time event handling, and dynamic content rendering for engaging user experiences.
Project Type: Web Application
Project Complexity: Beginner
Project Duration: 4–6 hours
Dependencies: React, TypeScript
Functionality Scope: Multiple-choice quizzes with scoring functionality.
Tasks in Project:
- Create questions with multiple-choice answers.
- Track and display user scores.
Technical Highlights:
- TypeScript for defining question types.
- Conditional rendering based on quiz progress.
Learning Outcomes:
- Dynamic rendering with TypeScript.
- Understanding state and events in React.
Skills Acquired:
- TypeScript for logic-heavy components.
Source Code: Quiz App Code
7. Currency Converter
The Currency Converter project utilizes TypeScript and APIs like Exchange Rates API to handle real-time currency conversion. This project showcases TypeScript’s use in data validation, asynchronous API handling, and building feature-rich financial tools with responsive UIs.
Project Type: Web Application
Project Complexity: Beginner to Intermediate
Project Duration: 4–6 hours
Dependencies: REST APIs, TypeScript
Functionality Scope: Convert currencies using live exchange rates.
Tasks in Project:
- Fetch currency data from an API.
- Provide real-time conversion results.
Technical Highlights:
- Handling API responses with TypeScript interfaces.
- Calculating and formatting currency conversions.
Learning Outcomes:
- Mastering TypeScript for financial calculations.
Skills Acquired:
- API integration, async handling, and type management.
Source Code: Currency Converter Code
8. Photo Gallery
This Photo Gallery application uses TypeScript and React to create an interactive platform for displaying and organizing images. It focuses on TypeScript’s type-checking for image metadata, dynamic rendering for filtering and sorting, and modular UI design for scalable applications.
Project Type: Web Application
Project Complexity: Beginner
Project Duration: 3–5 hours
Dependencies: TypeScript, CSS
Functionality Scope: Display and organize images in a grid layout.
Tasks in Project:
- Create an interactive grid view for images.
- Add zoom and filter options.
Technical Highlights:
- TypeScript for managing image metadata.
- Dynamic rendering with responsive CSS.
Learning Outcomes:
- Working with TypeScript in UI-heavy projects.
Skills Acquired:
- TypeScript for image processing and DOM updates.
Source Code: Photo Gallery Code
9. Movie Search App
The Movie Search App integrates TypeScript and APIs like OMDB to deliver real-time movie searches with details like ratings and descriptions. It demonstrates TypeScript’s utility in fetching and managing large datasets while ensuring error-free operations through strong type-checking.
Project Type: Web Application
Project Complexity: Intermediate
Project Duration: 6–8 hours
Dependencies: React, OMDb API, TypeScript
Functionality Scope: Search and display movie details.
Tasks in Project:
- Fetch and display movie data from an API.
- Add pagination for search results.
Technical Highlights:
- API integration with TypeScript interfaces.
- Pagination logic with React state.
Learning Outcomes:
- Building feature-rich applications with TypeScript.
Skills Acquired:
- TypeScript for data-intensive apps.
Source Code: Movie Search App Code
10. Markdown Editor
The Markdown Editor is a browser-based tool built with TypeScript to parse and render Markdown syntax in real time. It highlights TypeScript’s capabilities in DOM manipulation, state management, and creating dynamic applications with syntax highlighting and type-safe operations.
Project Type: Web Application
Project Complexity: Intermediate
Project Duration: 8–10 hours
Dependencies: React, Marked.js, TypeScript
Functionality Scope: Convert markdown text to formatted HTML.
Tasks in Project:
- Implement a dual-pane editor.
- Provide real-time preview of markdown.
Technical Highlights:
- Leveraging libraries with TypeScript.
- State synchronization between editor and preview panes.
Learning Outcomes:
- Text parsing and transformation using TypeScript.
Skills Acquired:
- Markdown parsing, DOM updates, and UI design.
Source Code: Markdown Editor Code
11. Real-Time Chat Application
This real-time Chat Application uses TypeScript, Node.js, and WebSockets to enable user communication. It demonstrates advanced concepts like event-driven architecture, type-safe message handling, and live data synchronization, making it a crucial project for real-time application development.
Project Type: Web Application
Project Complexity: Intermediate
Project Duration: 12–15 hours
Dependencies: WebSockets, Node.js, TypeScript
Functionality Scope: Real-time chat functionality for one-on-one or group communication.
Tasks in Project:
- Set up a WebSocket server with Node.js.
- Implement a frontend with TypeScript for sending and receiving messages.
Technical Highlights:
- Real-time data flow using WebSockets.
- TypeScript for defining message and user types.
Learning Outcomes:
- Building real-time features with TypeScript.
- Understanding WebSocket integration.
Skills Acquired:
- Event-driven programming and type-safe messaging.
Source Code: Chat Application Code
Are you finding it difficult to learn TypeScript and build projects like this? GUVI’s Full Stack Development Course is an excellent choice for learners eager to master TypeScript and build robust projects. This live, mentor-led program specializes in the MERN Stack (MongoDB, Express.js, React, Node.js) while also incorporating TypeScript into practical, real-world applications.
Designed to transform beginners into professionals within 90 days, this course offers comprehensive placement support and builds skills in crafting scalable web applications.
12. E-commerce Store
The E-commerce Store project incorporates TypeScript, React, and Redux to create a full-fledged shopping app. With features like cart management, payment integration, and product filtering, this project focuses on scalable application design and state management using TypeScript.
Project Type: Web Application
Project Complexity: Intermediate to Advanced
Project Duration: 15–20 hours
Dependencies: React, Redux, TypeScript
Functionality Scope: Build an e-commerce platform with a product catalog, cart, and checkout.
Tasks in Project:
- Integrate a product API for fetching details.
- Implement cart management with Redux.
Technical Highlights:
- Complex state management using TypeScript.
- Component-based architecture with React.
Learning Outcomes:
- Building scalable and modular applications.
- TypeScript for data validation and API responses.
Skills Acquired:
- State management, API integration, and modular design.
Source Code: E-commerce Store Code
13. Storybook
Storybook is a TypeScript-powered tool for developing and documenting UI components. It allows developers to build reusable components in isolation, emphasizing TypeScript’s role in defining component properties and ensuring consistency in design systems.
Project Type: Component Development Tool
Project Complexity: Intermediate
Project Duration: 6–8 hours
Dependencies: Storybook, TypeScript
Functionality Scope: Create an interactive UI component library.
Tasks in Project:
- Develop reusable UI components.
- Document component usage with TypeScript.
Technical Highlights:
- TypeScript for defining reusable prop types.
- Interactive component testing with Storybook.
Learning Outcomes:
- Creating and managing design systems.
- Using TypeScript for component-based development.
Skills Acquired:
- UI library creation, reusable components, and documentation.
Source Code: Storybook Code
14. Calculator
The Calculator project showcases TypeScript’s utility in building type-safe mathematical applications. It handles user input dynamically, performing basic to advanced operations while ensuring precision and error-free execution with strict type definitions.
Project Type: Web Application
Project Complexity: Beginner
Project Duration: 3–4 hours
Dependencies: HTML, CSS, TypeScript
Functionality Scope: Perform basic arithmetic operations.
Tasks in Project:
- Implement buttons for operations.
- Display results dynamically.
Technical Highlights:
- TypeScript for handling numeric operations.
- UI event handling with TypeScript.
Learning Outcomes:
- Understanding TypeScript for event-driven apps.
Skills Acquired:
- Type-safe operations, UI design, and DOM updates.
Source Code: Calculator Code
15. Random Quote Generator
The Random Quote Generator fetches quotes from APIs and dynamically displays them using TypeScript for error handling and type-safe data processing. It demonstrates TypeScript’s simplicity in handling asynchronous data fetching and DOM updates.
Project Type: Web Application
Project Complexity: Beginner
Project Duration: 3–5 hours
Dependencies: TypeScript, Fetch API
Functionality Scope: Display random quotes fetched from an API.
Tasks in Project:
- Fetch quotes from a public API.
- Display them with a button to generate new quotes.
Technical Highlights:
- TypeScript for API response handling.
Learning Outcomes:
- Integrating public APIs with TypeScript.
Skills Acquired:
- Data fetching, API integration, and basic app design.
Source Code: Random Quote Generator Code
16. Memory Game
This interactive Memory Game is built with TypeScript to manage game logic, state transitions, and animations. It provides an engaging way to understand state management, event handling, and responsive design using TypeScript.
Project Type: Web Application
Project Complexity: Beginner
Project Duration: 6–8 hours
Dependencies: HTML, CSS, TypeScript
Functionality Scope: Create a card-matching memory game.
Tasks in Project:
- Implement game logic for matching cards.
- Track and display scores.
Technical Highlights:
- TypeScript for game state management.
- Dynamic DOM updates for interactions.
Learning Outcomes:
- Using TypeScript for logic-heavy applications.
Skills Acquired:
- State management, UI updates, and basic animation.
Source Code: Memory Game Code
17. Expense Tracker
The Expense Tracker leverages TypeScript and React to build a financial tool that categorizes and visualizes expenses. It emphasizes type-safe state management, reusable components, and real-time data calculations for personal finance management.
Project Type: Web Application
Project Complexity: Intermediate
Project Duration: 6–8 hours
Dependencies: React, TypeScript
Functionality Scope: Track income and expenses with detailed categorization.
Tasks in Project:
- Create an input form for transactions.
- Display categorized expense summaries.
Technical Highlights:
- TypeScript for defining transaction types.
- Dynamic calculations for income and expenses.
Learning Outcomes:
- Working with TypeScript for financial data.
Skills Acquired:
- Data management, form handling, and financial logic.
Source Code: Expense Tracker Code
18. Blockchain Explorer
The Blockchain Explorer project uses TypeScript and Web3.js to fetch and display blockchain transactions, block data, and smart contract details. It highlights asynchronous programming, API integration, and type-safe data handling in blockchain environments.
Project Type: Web Application
Project Complexity: Advanced
Project Duration: 20–30 hours
Dependencies: Web3.js, TypeScript
Functionality Scope: Explore blockchain transactions and blocks.
Tasks in Project:
- Fetch blockchain data via Web3.js.
- Display transaction details dynamically.
Technical Highlights:
- Blockchain integration using TypeScript.
Learning Outcomes:
- Understanding blockchain data models.
Skills Acquired:
- Web3.js usage, async handling, and blockchain analysis.
Source Code: Blockchain Explorer Code
Final Words
Exploring these 18 TypeScript project ideas for beginners and advanced developers provides a clear path to mastering TypeScript.These TypeScript project ideas for beginners and experts not only help you learn key programming concepts but also make your portfolio more impressive.
With each project, you’ll gain practical knowledge, improve your coding confidence, and create portfolio-worthy applications. Reach out to us in the comments section if you have any doubts about any of these projects or the article. Good Luck!
FAQs
1. What are the easy TypeScript project ideas for beginners?
Beginner-friendly TypeScript project ideas include a to-do list app, weather app using APIs, a personal portfolio website, a simple calculator, or a note-taking app.
2. Why are TypeScript projects important for beginners?
TypeScript projects help beginners understand type safety, debugging, and the transition from JavaScript, building a strong foundation for scalable web applications.
3. What skills can beginners learn from TypeScript projects?
Beginners can learn type annotations, object-oriented programming, debugging, working with APIs, and creating reusable components.
4. Which TypeScript project is recommended for someone with no prior programming experience?
A simple to-do list or calculator app is recommended for absolute beginners to grasp the basics of TypeScript and programming.
5. How long does it typically take to complete a beginner-level TypeScript project?
Completing a beginner-level TypeScript project typically takes 1-2 days, depending on complexity and prior experience.
Did you enjoy this article?