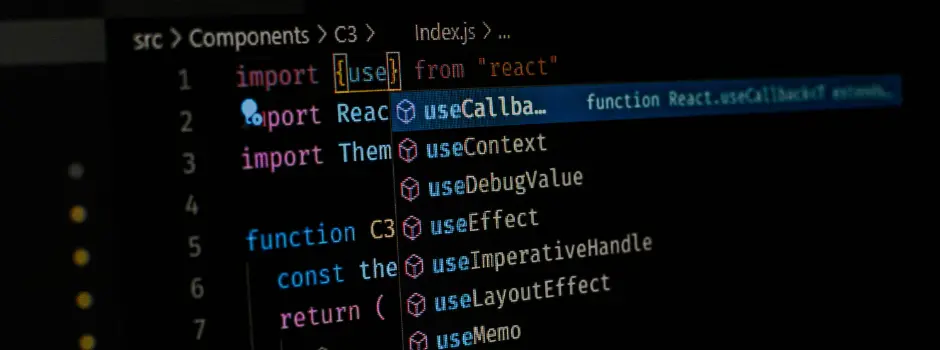
Understanding JavaScript Closures: A Deep Dive
Nov 06, 2024 3 Min Read 672 Views
(Last Updated)
JavaScript is a powerful and flexible language, that enables developers to craft complex, interactive applications. One of the essential yet sometimes puzzling concepts in JavaScript is closures. If you’ve worked with functions in JavaScript, you’ve likely encountered javascript closures, knowingly or unknowingly. This article will explore closures, providing clarity on what they are, how they work, and why they are so important in JavaScript.
Table of contents
- What is a Closure?
- Anatomy of a Closure
- Why are Closures Important?
- Real-world Use Cases for Closures
- Private Variables
- Function Factories
- Maintaining State in Asynchronous Operations
- Common Pitfalls with Closures
- Conclusion
What is a Closure?
A closure in JavaScript is essentially a function that “remembers” the environment in which it was created, even after that environment has disappeared. More formally, a closure is a function that retains access to its lexical scope, even when executed outside of that scope.
In simpler terms, a closure allows a function to access variables from its outer function even after the outer function has returned.
Anatomy of a Closure
Let’s break this down with a basic example:
function outerFunction() {
let outerVariable = ‘I am from the outer scope’;
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const closureFunction = outerFunction();
closureFunction(); // Output: “I am from the outer scope”
Here’s what happens step-by-step:
- outerFunction is called, and it declares a local variable outerVariable.
- Inside outerFunction, there’s another function called innerFunction that logs outerVariable to the console.
- outerFunction returns innerFunction but does not execute it.
- When we call closureFunction(), it has access to outerVariable, even though outerFunction has finished execution. This happens because of closure — innerFunction “closes over” its environment, preserving access to outerVariable.
Why are Closures Important?
Closures allow for some incredibly powerful programming patterns in JavaScript. Here are a few reasons why closures are valuable:
- Data Encapsulation: Closures enable you to hide variables from the outside scope, creating a kind of private variable. This is useful for controlling access to data.
- Maintaining State: A closure can maintain and update state across multiple function calls, which is key for scenarios like counters, memoization, or any situation where you want to retain information.
- Callbacks and Event Handlers: In asynchronous programming, closures are commonly used in callbacks, event handlers, and timers. They help preserve state at the time the callback was created, even when it’s executed much later.
Real-world Use Cases for Closures
Let’s look at some practical examples where closures shine.
1. Private Variables
Closures are often used to create private variables — variables that cannot be accessed or modified directly from outside a function.
function createCounter() {
let count = 0;
return {
increment: function () {
count++;
console.log(count);
},
decrement: function () {
count–;
console.log(count);
}
};
}
const counter = createCounter();
counter.increment(); // Output: 1
counter.increment(); // Output: 2
counter.decrement(); // Output: 1
Here, the count variable is private to the createCounter function. It can only be accessed and modified by the methods inside the returned object (increment and decrement), providing encapsulation.
2. Function Factories
Closures are useful when you want to generate a series of functions based on the same logic but with different parameters.
function createMultiplier(multiplier) {
return function (num) {
return num * multiplier;
};
}
const double = createMultiplier(2);
const triple = createMultiplier(3);
console.log(double(5)); // Output: 10
console.log(triple(5)); // Output: 15
Here, createMultiplier returns a function that “remembers” the value of multiplier. Each function created with createMultiplier has its own closure, preserving the specific multiplier passed when it was created.
3. Maintaining State in Asynchronous Operations
Closures are a common pattern in handling asynchronous operations like setTimeout or promises.
for (var i = 1; i <= 5; i++) {
setTimeout(function () {
console.log(i);
}, i * 1000);
}
// Output: 6 (five times)
In the above example, many developers expect the output to be 1, 2, 3, 4, 5. However, because var is function-scoped and the closure captures the reference to i, the value of i by the time the setTimeout callback runs is 6.
Using let instead of var solves the issue because let is block-scoped:
for (let i = 1; i <= 5; i++) {
setTimeout(function () {
console.log(i);
}, i * 1000);
}
// Output: 1, 2, 3, 4, 5
This happens because let creates a new binding for each iteration, thus preserving the expected value in the closure.
Common Pitfalls with Closures
Closures are immensely powerful, but they can introduce some challenges, especially for those new to JavaScript. Here are a couple of things to watch out for:
- Memory Leaks: Since closures hold onto references to their outer scope, they can inadvertently cause memory leaks if not used carefully. This usually happens when closures are used in long-running functions or objects, leading to variables never being garbage collected.
- Unintentional Retention of Variables: As seen in the setTimeout example, closures can sometimes retain unexpected values, especially when using var. Understanding block vs. function scoping is key here.
In case, you want to learn more about “JavaScript Closures” and gain in-depth knowledge on full-stack development, consider enrolling for GUVI’s certified Full-stack Development Course that teaches you everything from scratch and make sure you master it!
Conclusion
Closures are a fundamental concept in JavaScript that can be both elegant and tricky. They allow for powerful techniques such as data hiding, state preservation, and cleaner, more modular code. Understanding how closures work gives you the ability to write more robust and flexible applications.
The next time you create a function within a function, remember: you’ve likely just made a closure! Understanding this will not only enhance your JavaScript skills but also help you solve a wide range of programming challenges more effectively.
Did you enjoy this article?