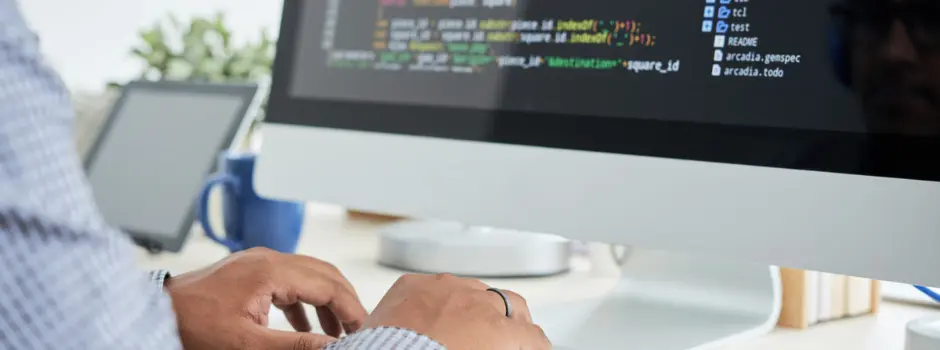
Understanding Polyfills: Bridging the Gap in Web Development
Nov 06, 2024 2 Min Read 567 Views
(Last Updated)
In the ever-evolving world of web development, the landscape of browsers and their support for modern JavaScript and CSS features is diverse. While new features are constantly being added to the web platform, not all browsers adopt these features at the same time. This can create challenges for developers who want to use the latest and greatest features while still supporting older browsers. This is where polyfills come into play.
Table of contents
- What is a Polyfill?
- Why Polyfills Are Important
- Common Use Cases for Polyfills
- How to Use Polyfills
- Popular Polyfill Libraries
- Best Practices for Using Polyfills
- Conclusion
What is a Polyfill?
A polyfill is a piece of code (usually JavaScript) that implements a feature on web browsers that do not natively support it. Essentially, it’s a script that “fills in” the gaps in a browser’s functionality, enabling developers to use modern features without having to worry about compatibility issues.
For example, if you want to use a JavaScript method like Array.prototype.includes() in your project, but need to support Internet Explorer, which does not have native support for this method, you can use a polyfill that provides a fallback implementation. This allows your code to work across all browsers, even those that do not support the method natively.
Why Polyfills Are Important
- Cross-Browser Compatibility: Not all users have access to the latest browser versions. Polyfills allow developers to write modern code without excluding users who are on older browsers.
- Progressive Enhancement: Polyfills enable progressive enhancement, a strategy where you build a baseline experience that works across all browsers, then add advanced features for browsers that support them.
- Future-Proofing: By using polyfills, you can start using new web standards immediately, knowing that your code will continue to work as browsers catch up.
Common Use Cases for Polyfills
- JavaScript Methods: Polyfills are often used to add support for newer JavaScript methods in older browsers. Examples include methods like Array.from(), Object.assign(), and Promise.
- HTML5 and CSS3 Features: As HTML5 and CSS3 introduced a range of new elements and styles, polyfills became essential to ensure that features like <canvas>, <video>, and CSS Grid work in older browsers.
- APIs: Newer APIs such as fetch for making network requests or IntersectionObserver for observing element visibility require polyfills for browsers that haven’t implemented these APIs yet.
How to Use Polyfills
Using a polyfill is generally straightforward. You include the polyfill script in your project, typically before your main JavaScript code runs. Here’s an example of how to include a polyfill for:
Array.prototype.includes():
if (!Array.prototype.includes) {
Array.prototype.includes = function(valueToFind, fromIndex) {
// Implementation of includes method
return this.indexOf(valueToFind, fromIndex) !== -1;
};
}
Alternatively, many polyfills are available as packages that can be installed via npm or included via a CDN, making it easier to manage and include them in your projects.
Popular Polyfill Libraries
- core-js: A comprehensive polyfill library that includes polyfills for many modern JavaScript features.
- polyfill.io: A service that automatically serves the polyfills needed by the user’s browser. This service is useful because it only delivers the polyfills required by the user’s browser, reducing the amount of unnecessary code.
- es5-shim: A polyfill for ECMAScript 5 features, which are essential for older browsers like Internet Explorer 8.
In case, you want to learn more about “this” and gain in-depth knowledge on full-stack development, consider enrolling for GUVI’s certified Full-stack Development Course that teaches you everything from scratch and make sure you master it!
Best Practices for Using Polyfills
- Conditional Loading: Avoid loading polyfills unnecessarily by using feature detection to check if a polyfill is needed before loading it.
- Consider Performance: Polyfills can add extra code to your application, which may affect performance. Always weigh the benefits against the cost, especially for performance-critical applications.
- Keep Up with Browser Support: Regularly review and update your polyfills as browser support evolves. Some polyfills may no longer be necessary as older browsers fall out of use.
Conclusion
Polyfills play a crucial role in modern web development by ensuring that the latest features of JavaScript, HTML5, and CSS3 can be used across all browsers. By understanding how and when to use polyfills, developers can create robust, future-proof applications that provide a consistent experience for all users, regardless of their browser choice. While the web is moving rapidly forward, polyfills help bridge the gap, making it possible to embrace new technologies without leaving anyone behind.
Did you enjoy this article?