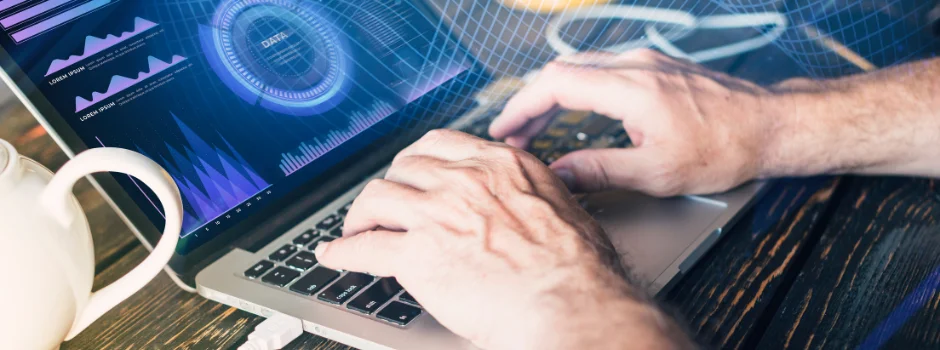
Understanding Selenium Waits: A Key to Effective Automation Testing
Jan 30, 2025 3 Min Read 426 Views
(Last Updated)
What’s more frustrating than a test script that fails unpredictably? You write a clean Selenium script, only for it to break because an element took a second longer to load. Modern web applications are dynamic – elements appear, disappear, and update asynchronously. If your script tries to interact with an element too soon, you’ll face exceptions that disrupt execution. This makes handling waits in Selenium a necessity not just a best practice.
Even a well-written test can fail without proper waits due to timing issues. This post will explore why selenium waits are critical, the different types available, and how to implement them effectively. If you’ve ever wondered why your test scripts behave inconsistently or fail intermittently, mastering Selenium waits might be the missing piece to achieving stable and reliable automation.
Table of contents
- Why Are Selenium Waits Important?
- Types of Waits in Selenium
- Implicit Waits
- Explicit Waits
- Fluent Waits
- Best Practices for Using Selenium Waits
- Use Explicit Waits for Dynamic Content
- Avoid Overusing Implicit Waits
- Avoid Using Thread.sleep()
- Set Reasonable Timeout Value
- Combine Different Types of Waits Wisely
- Conclusion
Why Are Selenium Waits Important?
In modern web applications, elements on a page often load dynamically after the initial page load. For instance, buttons might appear after an AJAX call, or an element might load asynchronously due to JavaScript execution. If your script tries to interact with an element before it is ready, you may encounter errors like:
- NoSuchElementException: When the element is not found.
- ElementNotVisibleException: When an element exists but is not visible or clickable.
- StaleElementReferenceException: When an element is no longer attached to the DOM.
To avoid these errors and ensure smooth execution, Selenium provides “waits,” which help the script to wait for a certain condition before interacting with elements. Without waits, your script might try to access elements before they are fully loaded or rendered.
Types of Waits in Selenium
Selenium provides three primary types of waits:
1. Implicit Waits
Implicit waits are used to instruct Selenium to wait for a certain amount of time before throwing an exception if an element is not found. Implicit waits are set globally and apply to all elements throughout the WebDriver session.
Syntax:
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
With an implicit wait, WebDriver will poll the DOM for the element every 500 milliseconds until the timeout is reached. If the element is found before the timeout, it will proceed immediately. If the timeout is reached and the element is not found, it will throw a NoSuchElementException.
Use Case: Implicit waits are useful when you know that elements are generally available, but they might take varying amounts of time to load (e.g., images, buttons, etc.).
Pros:
- Easy to implement and apply globally.
- Suitable for cases where elements typically appear after a delay.
Cons:
- It can slow down the test if used excessively, especially when dealing with multiple elements.
- Does not allow fine-grained control over waiting for specific conditions.
2. Explicit Waits
Explicit waits allow you to specify a condition to wait for before proceeding with the next step. These are more flexible than implicit waits and can be used for specific elements. Selenium’s WebDriverWait combined with expected conditions is the key to implementing explicit waits.
Syntax:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id(“someElement”)));
Here, WebDriverWait will wait until the specified element becomes visible. ExpectedConditions contains various conditions like visibilityOfElementLocated, elementToBeClickable, presenceOfElementLocated, etc.
Use Case: Explicit waits are used when you want to wait for a specific condition to occur (e.g., element visibility, text presence) before proceeding.
Pros:
- Allows you to wait for specific conditions rather than a fixed time.
- Provides better control over your test flow.
Cons:
- Requires more coding effort than implicit waits.
- Might introduce some complexity when dealing with multiple wait conditions in one script.
3. Fluent Waits
A Fluent Wait is similar to an explicit wait but with more fine-grained control over how frequently Selenium should check for a condition. Fluent waits can be customized to define the polling interval and the maximum wait time.
Syntax:
Wait<WebDriver> wait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(10))
.pollingEvery(Duration.ofMillis(500))
.ignoring(NoSuchElementException.class);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id(“someElement”)));
Here, the fluent wait will check for the element every 500 milliseconds and wait for up to 10 seconds for the condition to be met.
Use Case: Fluent waits are useful when you want to customize the polling frequency and ignore specific exceptions during the wait period.
Pros:
- Highly customizable for specific needs.
- Allows ignoring certain exceptions while waiting.
Cons:
- More complex than implicit or explicit waits.
- Should be used sparingly to avoid making the script too convoluted.
Best Practices for Using Selenium Waits
1. Use Explicit Waits for Dynamic Content
Dynamic content is a common scenario in modern web applications, so explicit waits are often the best choice to handle such situations. Use conditions like visibilityOfElementLocated or elementToBeClickable to wait until an element is ready for interaction.
2. Avoid Overusing Implicit Waits
While implicit waits can be useful, they can slow down your tests if set too long. Additionally, they might cause unexpected behavior when mixed with explicit waits, as implicit waits are global and apply to all elements.
3. Avoid Using Thread.sleep()
While Thread.sleep() can pause the execution for a specified time, it is not ideal for Selenium tests. It adds unnecessary delays and makes tests slower. Always prefer waits that check for conditions, rather than waiting for arbitrary periods.
4. Set Reasonable Timeout Value
When setting timeouts for explicit or fluent waits, make sure the values are reasonable. Too short of a timeout might cause elements to be missed, while too long may unnecessarily slow down your tests.
5. Combine Different Types of Waits Wisely
Sometimes, you might need to use a combination of waits for different parts of your test. For example, you might use an implicit wait for elements that generally load quickly but use explicit waits for more dynamic elements that need additional time to load.
Understanding Selenium waits is just one part of becoming a proficient automation tester. To truly master test automation and handle real-world challenges, structured training can make a difference. If you’re looking to gain hands-on experience and industry insights, check out the Selenium Automation Testing Course. The program is designed to help you write efficient, fail-proof test scripts while preparing you for a career in automation testing.
Conclusion
Selenium waits can be the difference between a stable test suite and one that’s riddled with intermittent failures. Without them, your script might attempt to interact with elements before they fully load, leading to avoidable errors. By strategically using implicit, explicit, and fluent waits, you ensure that your scripts synchronize seamlessly with real-world application behavior.
A well-structured automation script isn’t just about writing code, it’s about anticipating how web elements behave and adapting accordingly. Overusing or misusing waits can slow down tests, while the right balance improves efficiency and reliability. As you refine your test strategy, remember that mastering Selenium waits is key to building automation that runs smoothly across dynamic applications.
Did you enjoy this article?