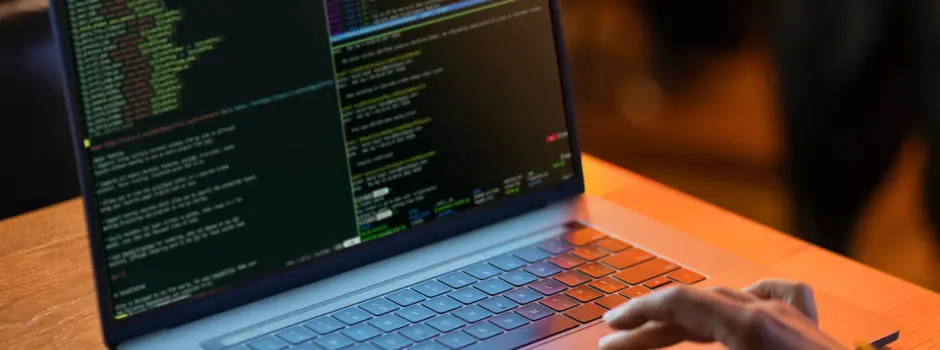
Working with dates and times is a fundamental part of many JavaScript applications, from scheduling events and logging activities to displaying timestamps and performing date calculations. JavaScript provides the Date
object as a built-in tool for creating, manipulating, and formatting dates and times.
In this article, we’ll take a comprehensive look at working with dates in JavaScript. We’ll start by exploring how to create and initialize dates using the Date
object, followed by common operations like formatting, parsing, and manipulating dates.
Table of contents
- Using Date object in JavaScript
- Creating Date objects: -
- Retrieving Date components: -
- Date using the Moment.js library
- Key Features of Moment.js
- Summary
Using Date object in JavaScript
One built-in datatype that facilitates working with dates and times is the JavaScript Date object. It offers several techniques for creating, modifying, and formatting dates.
Creating Date objects: –
Current Date and Time
const now = new Date(); console.log(now, typeof now) // 2024-10-03T02:43:23.173Z object |
Timestamp
const timestamp = Date.now(); // 1727923507074 const dateFromTimestamp = new Date(timestamp); console.log(dateFromTimestamp)// 2024-10-03T02:45:07.074Z |
Creating Date object using string
const dateString = Date(); console.log(dateString, typeof dateString) // Thu Oct 03 2024 08:18:56 GMT+0530 (India Standard Time) string const dateFromString = new Date(dateString); console.log(dateFromString, typeof dateFromString) // 2024-10-03T02:49:58.000Z object const dateStringTwo = “15 August 2024”; const dateFromStringTwo = new Date(dateStringTwo); console.log(dateFromStringTwo, typeof dateFromStringTwo); // 2024-08-15T00:00:00.000Z object |
Retrieving Date components: –
Once you have a Date object, you can retrieve multiple components using built-in methods
const dateObject = new Date(); // 2024-10-03T03:09:24.714Z console.log(dateObject.getFullYear()); // 2024 number console.log(dateObject.getMonth()); // 9 number console.log(dateObject.getDate()); // 3 number console.log(dateObject.getDay()); // 4 for Thursday (0 = Sunday) |
Date using the Moment.js library
A popular JavaScript library called Moment.js makes manipulating dates and times easier. Compared to the native JavaScript Date object, it offers a richer feature set that makes working with dates more straightforward.
Key Features of Moment.js
- Simplified Date Manipulation: Moment.js makes it simple to manipulate dates by providing methods for adding and subtracting time units (days, months, and years).
- Flexible Parsing: The library can parse dates from numerous formats, making managing user input or data from different sources easier.
- Formatting: Moment.js provides powerful formatting options to display dates in any desired format.
- Relative Time: With built-in methods, you may quickly represent relative time (e.g.: – 7 days ago).
- Validation: includes methods for validating date formats and checking if a date is valid.
Install the moment.js node package using – npm install moment
Creating moment instances
const date = moment(); console.log(date, typeof date) // Moment<2024-10-03T09:03:48+05:30> object |
const dateString = “2024-08-15”; const dateFromString = moment(dateString); console.log(dateFromString, typeof dateFromString); // Moment<2024-08-15T00:00:00+05:30> object const parsedDate = moment(“03/10/2024”, “DD/MM/YYYY”); console.log(parsedDate, typeof parsedDate); // Moment<2024-10-03T00:00:00+05:30> object |
Formatting dates
const formattedDateOne = moment().format(“DD-MM-YYYY”); console.log(formattedDateOne, typeof formattedDateOne); // 03-10-2024 string const formattedDateTwo = moment().format(“YYYY-MM-DD”); console.log(formattedDateTwo, typeof formattedDateTwo); // 2024-10-03 string |
Manipulating dates
const nextWeek = moment().add(7, “days”); console.log(nextWeek, typeof nextWeek); // Moment<2024-10-10T09:13:40+05:30> object const lastMonth = moment().subtract(1, “months”); console.log(lastMonth, typeof lastMonth); // Moment<2024-09-03T09:13:40+05:30> object |
Validation and Relative time
const isValidDate = moment(“2024-02-30”).isValid(); console.log(isValidDate); // false const relativeTime = moment(“2020-01-01”).fromNow(); console.log(relativeTime, typeof relativeTime); // 5 years ago string |
Chaining Methods
const now = moment(); console.log(now); // Moment<2024-10-03T09:26:07+05:30> object const resultOne = moment().add(5, “days”).subtract(2, “months”); console.log(resultOne, typeof resultOne); // Moment<2024-08-08T09:24:59+05:30> object const resultTwo = moment() .add(5, “days”) .subtract(2, “months”) .format(“DD-MM-YYY”); console.log(resultTwo, typeof resultTwo); // 08-08-242024 string |
In case, you want to learn more about “dates in JavaScript” and gain in-depth knowledge on full-stack development, consider enrolling for GUVI’s certified Full Stack Development Course that teaches you everything from scratch and make sure you master it!
Summary
Although the built-in JavaScript Date object is compact and a component of the language, Moment.js offers more flexible and user-friendly functionality. In applications where complex date manipulation, formatting, and time zone management are necessary, Moment.js can greatly reduce development time and improve the readability of code. Though Moment.js offers advantages to current codebases, it’s crucial to remember that as of September 2020, it is no longer actively supported. As such, developers should take into account more contemporary options, such as date-fns or Luxon, for new projects.
Did you enjoy this article?