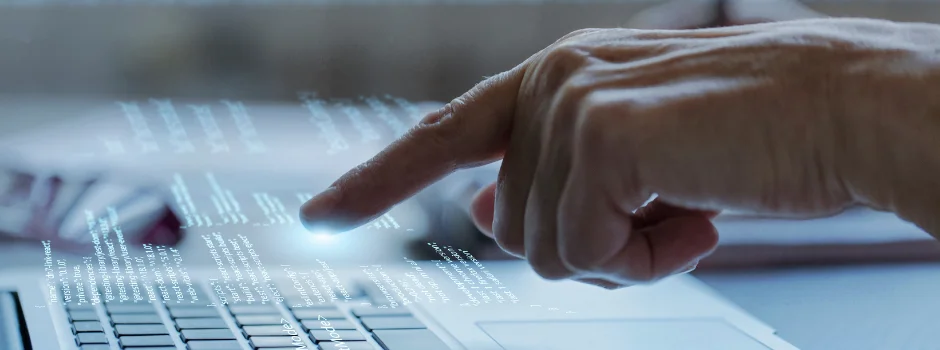
Working with Input Elements using Robot Framework
Jan 31, 2025 3 Min Read 721 Views
(Last Updated)
In this digital era, you must’ve visited thousands of websites, but at any point have you ever thought how websites handle user input automatically during testing? The answer lies in automation frameworks like Robot Framework, which streamline web testing by simulating real user interactions.
This article explores how to set up the environment, structure test cases, and execute automation scripts for interacting with input elements on a web page using Robot Framework.
Table of contents
- What is Robot Framework?
- Setting up Environment
- Project Structure
- Conclusion
What is Robot Framework?
Robot Framework is an open-source automation framework primarily aimed at acceptance testing and acceptance test-driven development (ATDD).
Known for its keyword-driven approach, it uses a simple, tabular syntax and is compatible with various libraries, allowing seamless integration with numerous automation tools and frameworks. One of its significant strengths lies in its extensive library support, which includes the popular Selenium for web testing.
Setting up Environment
Before you can start working with input elements on a web page, you need to set up the Robot Framework environment. This setup involves installing Robot Framework, SeleniumLibrary, and the appropriate WebDriver for your browser (e.g., ChromeDriver for Google Chrome).
- Install Robot Framework:
pip install robotframework
- Install SeleniumLibrary
pip install robotframework-seleniumlibrary
Project Structure
The project structure of the Test Suite will look like this which is given below :-
Reports Directory : This directory is designated for storing reports generated after test executions. In typical test automation projects, reports provide detailed information about test outcomes, including pass/fail statuses, logs, screenshots (if included), and other pertinent details. Having a separate Reports directory ensures that all test result documentation is organized in one location, making it easier to review and analyze the test outcomes.
TestCases Directory : This directory contains the test case files. Each file usually represents a suite of related test cases. In this structure, there is a single test suite file named Forms.robot. This is a Robot Framework test suite file. It contains test cases that likely focus on form-related functionalities on a web page. The .robot extension indicates that the file uses Robot Framework’s syntax and conventions for defining test cases.
The Code
The full-length code has been as given below :-
*** Settings ***
Documentation Working with Input Elements of a Web Page using Robot Framework
Library SeleniumLibrary
*** Variables ***
${URL} https://suman-dynamic-html-form.netlify.app/
${BROWSER} chrome
${FIRST_NAME} Suman
${LAST_NAME} Gangopadhyay
${ADDRESS} Bangalore
${PINCODE} 560068
*** Test Cases ***
TestCase-1
Start Automation Testing
TestCase-2
Fill Personal Details
TestCase-3
Click Radio Button
TestCase-4
Select Favorite Food
TestCase-5
Select State
TestCase-6
Select Country
TestCase-7
Click Submit Button
TestCase-8
Shutdown Automation Testing
*** Keywords ***
Start Automation Testing
[Documentation] Start the automation
[Tags] Suman-HTML-Form-1
open browser ${URL} ${BROWSER}
maximize browser window
set selenium implicit wait 10s
Shutdown Automation Testing
[Documentation] Start the automation
[Tags] Suman-HTML-Form-2
close all browsers
Fill Personal Details
[Documentation] Fill the First Name, Last Name, Address, Pincode
[Tags] Suman-HTML-Form-3
input text id=fname ${FIRST_NAME}
input text id=lname ${LAST_NAME}
input text id=address ${ADDRESS}
input text id=pin ${PINCODE}
Click Radio Button
[Documentation] Click the Radio Button
[Tags] Suman-HTML-Form-4
select radio button gender Male
Select Favorite Food
[Documentation] Select Favorite Food
[Tags] Suman-HTML-Form-5
select from list by value food Pizza
select from list by value food Pasta
Select State
[Documentation] Select Favorite Food
[Tags] Suman-HTML-Form-6
select from list by value state Karnataka
Select Country
[Documentation] Select Favorite Food
[Tags] Suman-HTML-Form-7
select from list by value country India
Click Submit Button
[Documentation] Select Favorite Food
[Tags] Suman-HTML-Form-8
click button xpath=//button[text()='Submit']
handle alert ACCEPT
sleep 5s
Let’s break down the Robot Framework script and understand its structure and functionality in detail. This script is designed to interact with a web form by performing actions like filling out personal details, selecting options, and submitting the form.
This Robot Framework script automates filling out a web form and submitting it. Here’s a detailed breakdown:
Settings:
- Documentation: Describes the purpose of the script – working with input elements of a web page.
- Library: Imports the SeleniumLibrary which provides keywords for interacting with web elements using Selenium WebDriver.
Variables:
- URL : Stores the URL of the web page to be tested
- {BROWSER} : Defines the browser to be used for automation (chrome in this case).
- {FIRSTNAME} : Holds the value to be entered in the first name field (Suman).
- {LAST_NAME}: Holds the value to be entered in the last name field (Gangopadhyay).
- ADDRESS : Holds the value to be entered in the address field (Bangalore).
- {PINCODE}: Holds the value to be entered in the pincode field (560068).
Test Cases:
These are high-level test cases describing the steps involved in the automation process. They don’t directly interact with the web page, but call specific keywords for each action.
- TestCase-1: Starts the automation (calls Start Automation Testing keyword).
- TestCase-2: Fills personal details (calls Fill Personal Details keyword).
- TestCase-3: Clicks a radio button (calls Click Radio Button keyword).
- TestCase-4: Selects favorite foods (calls Select Favorite Food keyword).
- TestCase-5: Selects a state (calls Select State keyword).
- TestCase-6: Selects a country (calls Select Country keyword).
- TestCase-7: Clicks the submit button (calls Click Submit Button keyword).
- TestCase-8: Shuts down the automation (calls Shutdown Automation Testing keyword).
Keywords:
These are reusable blocks of code that perform specific actions on the web page.
- Start Automation Testing:
- Opens the provided URL in the specified browser (chrome).
- Maximizes the browser window.
- Sets an implicit wait of 10 seconds for Selenium to find elements.
- Shutdown Automation Testing:
- Closes all open browser windows.
- Fill Personal Details:
- Enters the values from variables ${FIRST_NAME}, ${LAST_NAME}, ${ADDRESS}, and ${PINCODE} into the corresponding form fields identified by their id attributes.
- Click Radio Button:
- Selects the radio button with the label “Male” (assuming there’s one with the value “Male”).
- Select Favorite Food:
- Selects both “Pizza” and “Pasta” from the “food” dropdown list using their values.
- Select State:
- Selects “Karnataka” from the “state” dropdown list using its value.
- Select Country:
- Selects “India” from the “country” dropdown list using its value.
- Click Submit Button:
- Click the button with text “Submit” using XPath.
- Accepts any alert that pops up (assuming it’s a confirmation alert).
- Waits for 5 seconds after clicking submit.
Overall Workflow:
- The script starts by opening the web form URL in Chrome and maximizing the window.
- It then fills the personal details form fields with predefined values.
- It selects the “Male” radio button.
- It selects both “Pizza” and “Pasta” as favorite foods.
- It selects “Karnataka” as the state.
- It selects “India” as the country.
- Finally, it clicks the “Submit” button, accepts any alert, and waits for 5 seconds.
- After all test cases are executed, the script closes all open browser windows.
In case you want to learn more about automation testing with Selenium, consider enrolling for GUVI’s certified Selenium Automation Testing Course online course that teaches you everything from scratch and also provides you with an industry-grade certificate!
Conclusion
In conclusion, this Robot Framework script provides a detailed example of how to interact with various input elements on a web page. It demonstrates how to open a browser, fill out a form, select options, submit the form, and handle alerts.
By using custom keywords, the script is modular and easy to read, making it an excellent template for automating similar web interactions.
Did you enjoy this article?